Top Atlassian Interview Questions: Ace Your Next Tech Interview
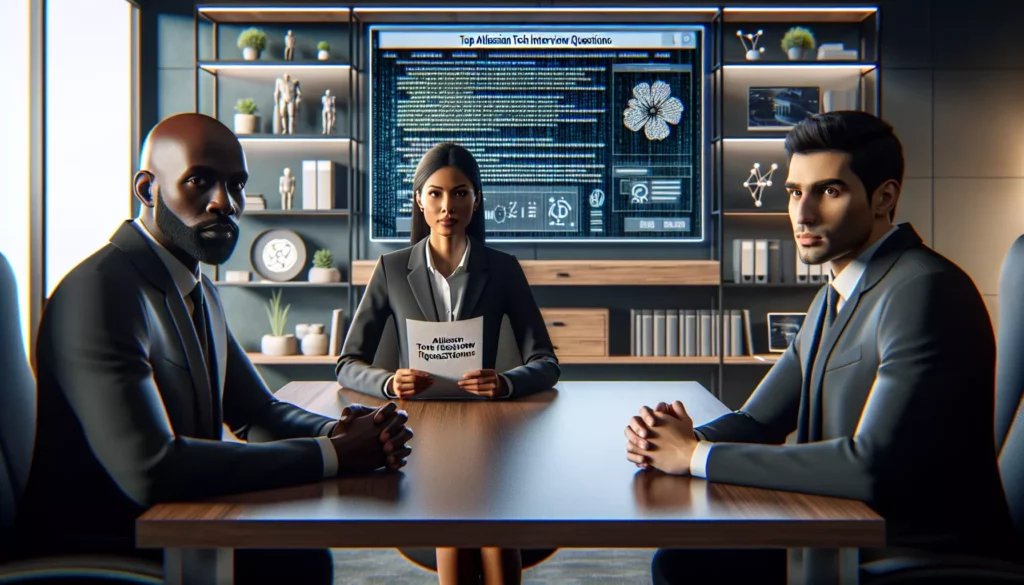
Are you gearing up for an interview with Atlassian, one of the leading software companies known for products like Jira, Confluence, and Trello? You’re in the right place! In this comprehensive guide, we’ll dive deep into the most common Atlassian interview questions, provide expert tips on how to answer them, and offer insights into the company’s hiring process. Whether you’re a seasoned developer or a fresh graduate, this article will help you prepare and boost your chances of landing that dream job at Atlassian.
Table of Contents
- Introduction to Atlassian Interviews
- Technical Interview Questions
- Behavioral Interview Questions
- Coding Challenges and Problem-Solving
- System Design Questions
- Culture Fit and Company Values
- The Atlassian Interview Process
- Preparation Tips and Resources
- Conclusion
1. Introduction to Atlassian Interviews
Atlassian is renowned for its collaborative software tools and innovative workplace culture. The company’s interview process is designed to assess not only your technical skills but also your problem-solving abilities, cultural fit, and potential for growth within the organization. Understanding the types of questions you might encounter is crucial for your success.
Atlassian’s interview process typically includes several stages:
- Initial phone screen or video call
- Technical coding interview
- System design discussion
- Behavioral and cultural fit interviews
- On-site interviews (or virtual equivalent)
Let’s explore each type of question you might face during these stages.
2. Technical Interview Questions
Technical questions form the core of Atlassian’s interview process for engineering roles. These questions are designed to assess your knowledge of programming languages, data structures, algorithms, and software development principles. Here are some common technical questions you might encounter:
2.1. Java-Specific Questions
Given Atlassian’s extensive use of Java, you’re likely to face Java-specific questions if you’re applying for a Java developer role:
- What are the main differences between an interface and an abstract class in Java?
- Explain the concept of Java’s garbage collection and how it works.
- What is the difference between “=” and “==” in Java?
- How does the HashMap work internally in Java?
- Explain the concept of multithreading in Java. How would you implement a thread-safe singleton?
2.2. Data Structures and Algorithms
Regardless of the specific programming language, you should be prepared to discuss various data structures and algorithms:
- Explain the difference between a stack and a queue. When would you use each?
- What is the time complexity of searching in a balanced binary search tree?
- Describe how you would implement a hash table from scratch.
- What is the difference between depth-first search (DFS) and breadth-first search (BFS)?
- Explain the concept of dynamic programming and provide an example of a problem that can be solved using this technique.
2.3. Web Development and APIs
For roles involving web development or API design, you might encounter questions like:
- What is RESTful API design, and what are its key principles?
- Explain the differences between GET and POST HTTP methods.
- What is Cross-Origin Resource Sharing (CORS), and why is it important?
- Describe the concept of server-side rendering versus client-side rendering.
- What are the main differences between SQL and NoSQL databases?
3. Behavioral Interview Questions
Behavioral questions are designed to assess your past experiences, problem-solving skills, and how you handle various workplace situations. Atlassian places a strong emphasis on teamwork and collaboration, so be prepared to discuss your experiences in these areas. Here are some common behavioral questions:
- Tell me about a time when you had to work on a challenging project with a tight deadline. How did you manage it?
- Describe a situation where you had a conflict with a team member. How did you resolve it?
- Can you share an example of a time when you had to learn a new technology quickly? How did you approach it?
- Tell me about a project you’re particularly proud of. What was your role, and what made it successful?
- Describe a time when you had to give constructive feedback to a colleague. How did you approach it, and what was the outcome?
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses. This approach helps you provide concise, relevant answers that showcase your skills and experiences effectively.
4. Coding Challenges and Problem-Solving
Atlassian’s interview process often includes live coding challenges or take-home assignments. These are designed to assess your problem-solving skills, coding ability, and how you approach complex tasks. Here are some tips for tackling coding challenges:
- Clarify the requirements before you start coding
- Think out loud and explain your thought process
- Consider edge cases and potential optimizations
- Write clean, well-commented code
- Be prepared to discuss your solution and potential alternatives
Here’s an example of a coding challenge you might encounter:
Problem: Implement a function to find the longest palindromic substring in a given string.
Input: A string s
Output: The longest palindromic substring in s
Example:
Input: s = "babad"
Output: "bab" or "aba" (both are valid)
Input: s = "cbbd"
Output: "bb"
Constraints:
1 <= s.length <= 1000
s consists of only lowercase English letters.
To solve this problem, you might implement a solution using dynamic programming or expand around center approach. Here’s a sample implementation in Java:
public class Solution {
public String longestPalindrome(String s) {
if (s == null || s.length() < 2) {
return s;
}
int start = 0, maxLength = 1;
for (int i = 0; i < s.length(); i++) {
int len1 = expandAroundCenter(s, i, i);
int len2 = expandAroundCenter(s, i, i + 1);
int len = Math.max(len1, len2);
if (len > maxLength) {
start = i - (len - 1) / 2;
maxLength = len;
}
}
return s.substring(start, start + maxLength);
}
private int expandAroundCenter(String s, int left, int right) {
while (left >= 0 && right < s.length() && s.charAt(left) == s.charAt(right)) {
left--;
right++;
}
return right - left - 1;
}
}
Be prepared to explain your approach, discuss its time and space complexity, and consider potential optimizations or alternative solutions.
5. System Design Questions
For more senior roles or positions involving architecture and scalability, you may encounter system design questions. These assess your ability to design large-scale systems and make architectural decisions. Some common system design questions include:
- Design a distributed task queue system like Atlassian’s Jira Service Management.
- How would you design a real-time collaboration feature for a document editing system like Confluence?
- Design a scalable notification system for a project management tool like Trello.
- How would you architect a system to handle millions of concurrent users for a chat application?
- Design a URL shortener service that can handle high traffic volumes.
When approaching system design questions, consider the following steps:
- Clarify requirements and constraints
- Outline the high-level architecture
- Dive into component design
- Discuss data models and storage
- Address scalability and performance considerations
- Identify potential bottlenecks and discuss solutions
Remember to think about Atlassian’s scale and the types of problems they solve when considering your design choices.
6. Culture Fit and Company Values
Atlassian places a strong emphasis on culture and values. They look for candidates who align with their company values and can contribute to their collaborative work environment. Be prepared to discuss how you embody Atlassian’s values:
- Open company, no bullshit
- Build with heart and balance
- Don’t #@!% the customer
- Play, as a team
- Be the change you seek
You might be asked questions like:
- How do you stay up-to-date with the latest technologies and industry trends?
- Describe a situation where you had to be transparent about a mistake you made. How did you handle it?
- How do you balance work and personal life?
- Tell me about a time when you went above and beyond for a customer or end-user.
- How do you contribute to creating a positive team environment?
When answering these questions, provide specific examples from your past experiences that demonstrate how you align with Atlassian’s values and culture.
7. The Atlassian Interview Process
Understanding the Atlassian interview process can help you prepare more effectively. Here’s a typical breakdown of the stages:
- Initial Application: Submit your resume and cover letter through Atlassian’s careers page.
- Phone Screen: A brief call with a recruiter to discuss your background and the role.
- Technical Phone Interview: A more in-depth discussion of your technical skills, often including coding questions.
- Take-Home Assignment: Some roles may require a coding assignment to be completed on your own time.
- On-Site Interviews: A series of interviews with team members, including technical, behavioral, and system design discussions. (Note: During the COVID-19 pandemic, these may be conducted virtually.)
- Final Decision: The hiring team reviews all feedback and makes a decision.
The entire process can take anywhere from a few weeks to a couple of months, depending on the role and current hiring needs.
8. Preparation Tips and Resources
To maximize your chances of success in an Atlassian interview, consider the following preparation tips:
- Review Core Computer Science Concepts: Brush up on data structures, algorithms, and system design principles.
- Practice Coding: Use platforms like LeetCode, HackerRank, or CodeSignal to practice coding problems.
- Study Atlassian Products: Familiarize yourself with Atlassian’s product suite and their use cases.
- Prepare Your Stories: Have specific examples ready for behavioral questions, using the STAR method.
- Mock Interviews: Practice with a friend or use services like Pramp for mock technical interviews.
- Stay Updated: Follow Atlassian’s blog and tech news to stay current on industry trends.
Useful resources for preparation include:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- Atlassian’s official blog and documentation
- Online courses on algorithms and system design from platforms like Coursera or edX
9. Conclusion
Preparing for an Atlassian interview requires a combination of technical skills, problem-solving ability, and cultural alignment. By thoroughly reviewing the types of questions you might encounter and practicing your responses, you’ll be well-equipped to showcase your talents and potential as an Atlassian team member.
Remember, the interview process is not just about Atlassian evaluating you—it’s also an opportunity for you to assess whether the company and role align with your career goals and values. Approach the interview with confidence, curiosity, and a genuine interest in the problems Atlassian is solving.
Good luck with your Atlassian interview! With thorough preparation and the right mindset, you’ll be well on your way to joining one of the most innovative companies in the tech industry.