Top Asana Interview Questions: Ace Your Technical Interview
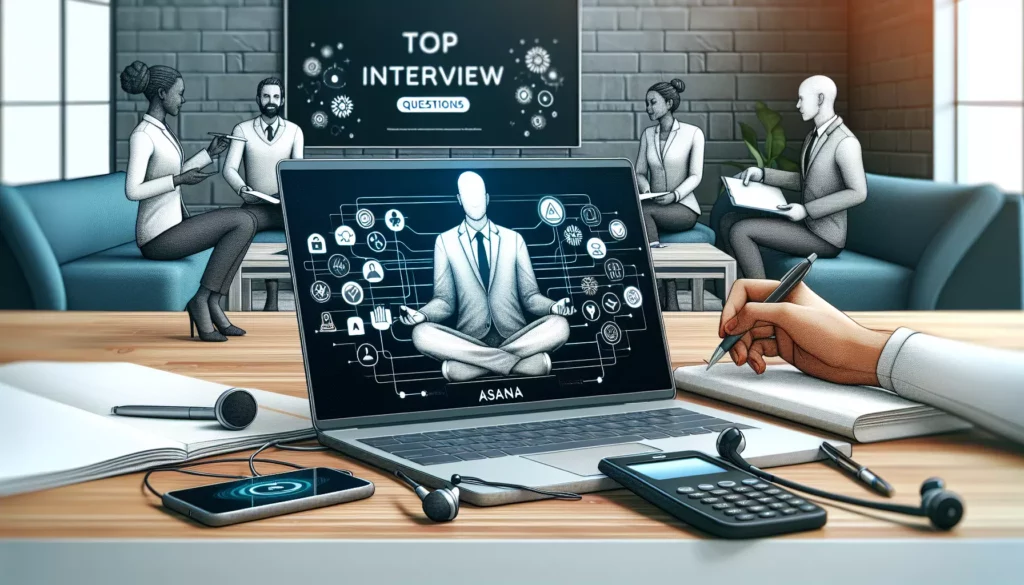
Are you preparing for a technical interview at Asana? You’ve come to the right place! In this comprehensive guide, we’ll explore the most common Asana interview questions, provide insights into the company’s interview process, and offer tips to help you succeed. Whether you’re a seasoned developer or just starting your career in tech, this article will equip you with the knowledge and confidence to tackle your Asana interview head-on.
Table of Contents
- Understanding Asana: Company Culture and Values
- The Asana Interview Process: What to Expect
- Technical Interview Questions
- Behavioral Interview Questions
- System Design Questions
- Coding Challenges and Problem-Solving
- Tips for Interview Success
- Preparation Resources
- Conclusion
Understanding Asana: Company Culture and Values
Before diving into the specific interview questions, it’s crucial to understand Asana’s company culture and values. Asana is a leading work management platform that helps teams organize, track, and manage their work. The company prides itself on its mission to help humanity thrive by enabling all teams to work together effortlessly.
Key aspects of Asana’s culture include:
- Emphasis on mindfulness and work-life balance
- Commitment to diversity, equity, and inclusion
- Focus on transparency and open communication
- Encouragement of continuous learning and growth
Understanding these values will help you align your responses and demonstrate cultural fit during the interview process.
The Asana Interview Process: What to Expect
The Asana interview process typically consists of several stages:
- Initial phone screen with a recruiter
- Technical phone interview or take-home coding challenge
- On-site interviews (or virtual equivalent)
- Final decision and offer
The on-site interviews usually include:
- Coding interviews
- System design discussions
- Behavioral interviews
- Culture fit assessments
Now, let’s explore the types of questions you might encounter during your Asana interview.
Technical Interview Questions
Asana’s technical interviews aim to assess your programming skills, problem-solving abilities, and understanding of fundamental computer science concepts. Here are some common technical questions you might face:
1. Data Structures and Algorithms
Question: “Implement a function to reverse a linked list.”
This question tests your understanding of linked list operations and your ability to manipulate pointers. Here’s a sample solution in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
2. Time and Space Complexity Analysis
Question: “What is the time and space complexity of the following function? Can you optimize it?”
def find_duplicate(arr):
seen = set()
for num in arr:
if num in seen:
return num
seen.add(num)
return None
This question assesses your ability to analyze algorithmic efficiency. The current implementation has a time complexity of O(n) and a space complexity of O(n). You could optimize the space complexity to O(1) using Floyd’s cycle-finding algorithm (tortoise and hare).
3. Object-Oriented Programming
Question: “Design a class hierarchy for a task management system, including classes for tasks, projects, and users.”
This question evaluates your understanding of OOP principles such as inheritance, encapsulation, and polymorphism. Here’s a basic structure to get you started:
class User:
def __init__(self, name, email):
self.name = name
self.email = email
self.tasks = []
self.projects = []
class Task:
def __init__(self, title, description, due_date, assignee):
self.title = title
self.description = description
self.due_date = due_date
self.assignee = assignee
self.status = "To Do"
class Project:
def __init__(self, name, owner):
self.name = name
self.owner = owner
self.tasks = []
self.members = [owner]
def add_task(self, task):
self.tasks.append(task)
def add_member(self, user):
self.members.append(user)
4. Database Design and SQL
Question: “Design a database schema for a social media platform, including tables for users, posts, and comments. Write a SQL query to fetch all comments for a specific post.”
This question tests your understanding of database design principles and SQL querying. Here’s a sample schema and query:
CREATE TABLE Users (
user_id INT PRIMARY KEY,
username VARCHAR(50) UNIQUE,
email VARCHAR(100) UNIQUE,
created_at TIMESTAMP
);
CREATE TABLE Posts (
post_id INT PRIMARY KEY,
user_id INT,
content TEXT,
created_at TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES Users(user_id)
);
CREATE TABLE Comments (
comment_id INT PRIMARY KEY,
post_id INT,
user_id INT,
content TEXT,
created_at TIMESTAMP,
FOREIGN KEY (post_id) REFERENCES Posts(post_id),
FOREIGN KEY (user_id) REFERENCES Users(user_id)
);
-- Query to fetch all comments for a specific post
SELECT c.comment_id, u.username, c.content, c.created_at
FROM Comments c
JOIN Users u ON c.user_id = u.user_id
WHERE c.post_id = ?
ORDER BY c.created_at DESC;
5. Web Technologies and APIs
Question: “Explain the differences between REST and GraphQL. When would you choose one over the other?”
This question assesses your understanding of modern web technologies and API design. Some key points to mention:
- REST uses multiple endpoints for different resources, while GraphQL uses a single endpoint
- REST often leads to over-fetching or under-fetching of data, while GraphQL allows clients to request exactly what they need
- REST is simpler to implement and has better caching mechanisms, while GraphQL offers more flexibility and reduces the number of network requests
Behavioral Interview Questions
Asana places a strong emphasis on cultural fit and teamwork. Here are some behavioral questions you might encounter:
1. Collaboration and Teamwork
Question: “Describe a situation where you had to work with a difficult team member. How did you handle it, and what was the outcome?”
This question assesses your interpersonal skills and ability to navigate challenging team dynamics. Focus on your communication strategies, problem-solving approach, and the positive results you achieved.
2. Handling Pressure and Deadlines
Question: “Tell me about a time when you had to meet a tight deadline. How did you prioritize your tasks and ensure timely delivery?”
This question evaluates your time management skills and ability to perform under pressure. Highlight your organizational methods, ability to focus on critical tasks, and any strategies you used to optimize your workflow.
3. Learning and Growth
Question: “Describe a technically challenging project you worked on recently. What did you learn from it, and how did you apply that knowledge to subsequent projects?”
This question assesses your ability to learn from experiences and apply new knowledge. Emphasize your problem-solving process, the specific technical skills you gained, and how you’ve used those skills in other contexts.
4. Conflict Resolution
Question: “Give an example of a time when you disagreed with a colleague’s approach to solving a technical problem. How did you resolve the disagreement?”
This question evaluates your communication skills and ability to handle conflicts professionally. Focus on your approach to understanding different perspectives, your ability to find common ground, and how you worked towards a mutually beneficial solution.
5. Adaptability and Innovation
Question: “Tell me about a time when you had to adapt to a significant change in project requirements or technology stack. How did you handle the transition?”
This question assesses your flexibility and ability to embrace change. Highlight your willingness to learn new technologies, your problem-solving skills in the face of unexpected challenges, and your ability to maintain productivity during transitions.
System Design Questions
For more senior positions, you may encounter system design questions that test your ability to architect scalable and efficient solutions. Here are some examples:
1. Design a Task Management System
Question: “Design a scalable task management system similar to Asana. Consider features like task creation, assignment, due dates, and real-time updates.”
Key points to address:
- Data model for tasks, projects, and users
- API design for CRUD operations
- Scalability considerations (e.g., sharding, caching)
- Real-time update mechanism (e.g., WebSockets, long polling)
- Search functionality and indexing
2. Design a Notification System
Question: “Design a notification system that can handle millions of users and support various notification types (email, push, in-app).”
Key points to address:
- Message queuing system for handling high volume
- Strategies for handling delivery failures and retries
- User preference management for notification types
- Scaling considerations for different notification channels
- Analytics and monitoring for notification delivery and engagement
3. Design a Collaborative Document Editing System
Question: “Design a real-time collaborative document editing system similar to Google Docs.”
Key points to address:
- Conflict resolution strategies (e.g., Operational Transformation, CRDTs)
- Real-time synchronization mechanism
- Version control and history tracking
- Scalability considerations for concurrent edits
- Offline editing and sync capabilities
Coding Challenges and Problem-Solving
Asana may present you with coding challenges to solve during the interview or as a take-home assignment. These challenges are designed to assess your problem-solving skills, coding ability, and attention to detail. Here are some tips for tackling coding challenges:
- Read the problem statement carefully and ask clarifying questions if needed.
- Consider edge cases and potential input variations.
- Start with a brute-force solution, then optimize if time allows.
- Write clean, well-documented code with meaningful variable and function names.
- Test your solution with various inputs, including edge cases.
- Be prepared to explain your thought process and justify your design decisions.
Here’s an example of a coding challenge you might encounter:
Challenge: “Implement a function that takes a string as input and returns the length of the longest palindromic substring within it.”
Here’s a sample solution using the expanding around center technique:
def longest_palindrome_substr(s):
if not s:
return 0
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
max_length = 0
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
max_length = max(max_length, len1, len2)
return max_length
# Test the function
print(longest_palindrome_substr("babad")) # Output: 3 ("bab" or "aba")
print(longest_palindrome_substr("cbbd")) # Output: 2 ("bb")
Tips for Interview Success
To increase your chances of success in your Asana interview, consider the following tips:
- Research the company: Familiarize yourself with Asana’s products, recent news, and company culture.
- Practice coding: Solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy to sharpen your skills.
- Review computer science fundamentals: Brush up on data structures, algorithms, and system design principles.
- Prepare questions: Have thoughtful questions ready to ask your interviewers about the role, team, and company.
- Use the STAR method: For behavioral questions, structure your responses using the Situation, Task, Action, Result format.
- Think aloud: During technical interviews, verbalize your thought process to give interviewers insight into your problem-solving approach.
- Be collaborative: Engage with your interviewers, ask for clarification when needed, and be open to feedback.
- Show enthusiasm: Demonstrate your passion for technology and your interest in Asana’s mission and products.
- Follow up: Send a thank-you email to your interviewers, reiterating your interest in the position.
Preparation Resources
To help you prepare for your Asana interview, consider using the following resources:
- AlgoCademy: Use our interactive coding tutorials and AI-powered assistance to improve your algorithmic thinking and problem-solving skills.
- LeetCode: Practice coding problems, particularly those tagged as “Asana” or similar companies.
- System Design Primer: A comprehensive GitHub repository covering system design concepts and examples.
- Asana Blog and Engineering Blog: Read about Asana’s technology stack, engineering challenges, and company culture.
- Glassdoor: Review interview experiences shared by other candidates who have interviewed at Asana.
- Cracking the Coding Interview: A popular book covering essential algorithms, data structures, and interview strategies.
Conclusion
Preparing for an Asana interview requires a combination of technical skills, problem-solving abilities, and cultural alignment. By familiarizing yourself with the types of questions you might encounter and following the tips provided in this guide, you’ll be well-equipped to showcase your talents and make a strong impression during the interview process.
Remember that interviews are also an opportunity for you to evaluate whether Asana is the right fit for your career goals and work style. Don’t hesitate to ask questions and engage in meaningful discussions with your interviewers about the role, team dynamics, and growth opportunities within the company.
With thorough preparation and a positive attitude, you’ll be well on your way to acing your Asana interview and potentially joining one of the most innovative companies in the work management space. Good luck!