Top Apple Interview Questions: A Comprehensive Guide for Aspiring Developers
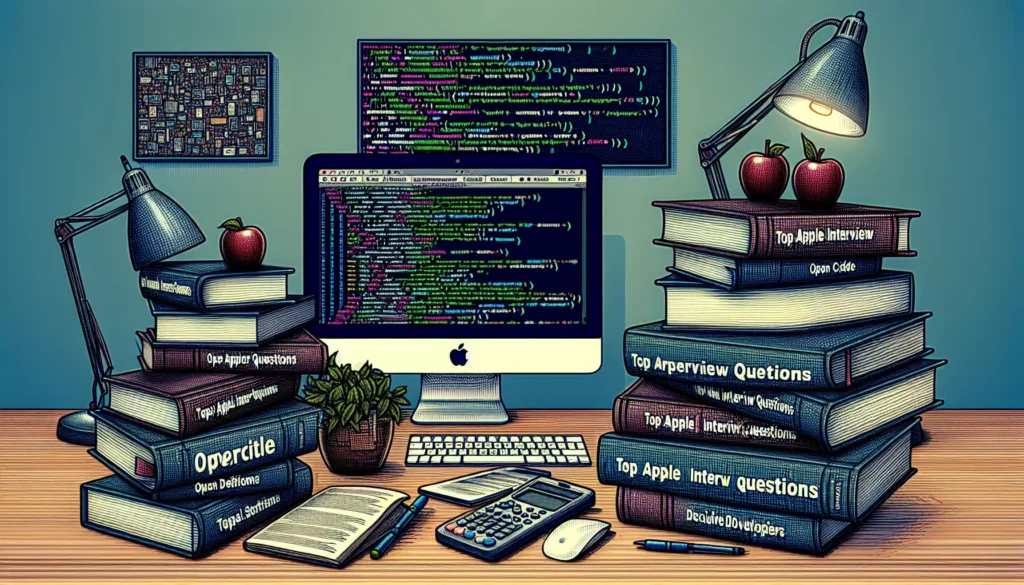
As one of the world’s most innovative and prestigious technology companies, Apple is a dream workplace for many software developers. However, landing a job at Apple is no easy feat. The interview process is known to be rigorous, testing candidates on various aspects of computer science, problem-solving skills, and cultural fit. In this comprehensive guide, we’ll explore some of the most common Apple interview questions, providing insights and tips to help you prepare for your big day.
Table of Contents
- Introduction to Apple’s Interview Process
- Technical Interview Questions
- Algorithmic and Data Structure Questions
- System Design Questions
- Behavioral and Cultural Fit Questions
- iOS-Specific Questions (for iOS Developer Positions)
- Tips for Success in Apple Interviews
- Conclusion
1. Introduction to Apple’s Interview Process
Before diving into specific questions, it’s essential to understand Apple’s interview process. Typically, it consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to assess your background and interest.
- Technical Phone Interview: One or more technical interviews conducted over the phone or video call.
- On-site Interviews: A series of face-to-face interviews (or virtual equivalents) with team members and potential colleagues.
- Final Evaluation: A decision-making process where feedback from all interviewers is considered.
Throughout these stages, you’ll encounter a mix of technical, algorithmic, system design, and behavioral questions. Let’s explore each category in detail.
2. Technical Interview Questions
Apple’s technical questions are designed to assess your fundamental knowledge of computer science and programming concepts. Here are some examples:
2.1. Object-Oriented Programming (OOP) Concepts
Q: Explain the difference between class and struct in Swift.
A: In Swift, classes and structs are both used to define custom types, but they have some key differences:
- Classes are reference types, while structs are value types.
- Classes support inheritance, while structs do not.
- Classes have a deinitializer, structs do not.
- Classes can be used with reference counting, structs cannot.
Q: What is polymorphism, and how is it implemented in Swift?
A: Polymorphism is the ability of objects of different classes to respond to the same method call. In Swift, polymorphism can be implemented through:
- Inheritance and method overriding
- Protocols and protocol extensions
- Generics
2.2. Memory Management
Q: Explain Automatic Reference Counting (ARC) in iOS development.
A: ARC is a memory management feature in Swift and Objective-C that automatically keeps track of strong references to objects. When an object’s reference count drops to zero, ARC deallocates it. This helps prevent memory leaks and makes memory management more efficient.
Q: What is a retain cycle, and how can you prevent it?
A: A retain cycle occurs when two objects hold strong references to each other, preventing ARC from deallocating them. To prevent retain cycles:
- Use weak or unowned references where appropriate
- Break strong reference cycles in deinitializers
- Use capture lists in closures to define capture semantics
2.3. Concurrency and Multithreading
Q: Explain the difference between synchronous and asynchronous operations.
A: Synchronous operations block the current thread until the operation completes, while asynchronous operations allow the current thread to continue execution without waiting for the operation to finish. Asynchronous operations are often used for tasks that may take a long time, such as network requests or file I/O.
Q: How do you prevent race conditions in multithreaded applications?
A: Race conditions can be prevented by:
- Using synchronization mechanisms like locks, semaphores, or dispatch queues
- Implementing atomic operations
- Using thread-safe data structures
- Applying the actor model in Swift for concurrent programming
3. Algorithmic and Data Structure Questions
Apple often asks candidates to solve algorithmic problems to assess their problem-solving skills and knowledge of data structures. Here are some examples:
3.1. Array Manipulation
Q: Given an array of integers, find two numbers such that they add up to a specific target number.
A: This problem can be solved efficiently using a hash table:
func twoSum(_ nums: [Int], _ target: Int) -> [Int] {
var dict = [Int: Int]()
for (i, num) in nums.enumerated() {
if let j = dict[target - num] {
return [j, i]
}
dict[num] = i
}
return []
}
3.2. String Manipulation
Q: Implement a function to determine if a string has all unique characters.
A: Here’s a solution using a Set:
func hasAllUniqueCharacters(_ str: String) -> Bool {
return Set(str).count == str.count
}
3.3. Linked Lists
Q: Reverse a linked list.
A: Here’s an iterative solution:
class ListNode {
var val: Int
var next: ListNode?
init(_ val: Int) {
self.val = val
self.next = nil
}
}
func reverseList(_ head: ListNode?) -> ListNode? {
var prev: ListNode? = nil
var current = head
while current != nil {
let nextTemp = current!.next
current!.next = prev
prev = current
current = nextTemp
}
return prev
}
3.4. Trees and Graphs
Q: Implement an in-order traversal of a binary tree.
A: Here’s a recursive solution:
class TreeNode {
var val: Int
var left: TreeNode?
var right: TreeNode?
init(_ val: Int) {
self.val = val
self.left = nil
self.right = nil
}
}
func inorderTraversal(_ root: TreeNode?) -> [Int] {
var result = [Int]()
inorderHelper(root, &result)
return result
}
func inorderHelper(_ node: TreeNode?, _ result: inout [Int]) {
guard let node = node else { return }
inorderHelper(node.left, &result)
result.append(node.val)
inorderHelper(node.right, &result)
}
4. System Design Questions
For more senior positions, Apple may ask system design questions to evaluate your ability to design scalable and efficient systems. Here are some examples:
4.1. Design a URL Shortener
Q: Design a system that shortens long URLs into shorter ones, like bit.ly.
A: Key considerations for this design include:
- Generating unique short codes
- Storing mappings between short and long URLs
- Handling redirects
- Scaling the system for high traffic
- Analytics and tracking
4.2. Design a Distributed Cache
Q: Design a distributed caching system to improve the performance of a large-scale web application.
A: Important aspects to consider:
- Cache eviction policies (e.g., LRU, LFU)
- Consistency and replication
- Sharding and partitioning
- Fault tolerance and recovery
- Monitoring and scaling
4.3. Design a News Feed System
Q: Design a news feed system similar to Facebook or Twitter.
A: Key components to discuss:
- Data model for users, posts, and relationships
- Feed generation and ranking algorithms
- Caching strategies for fast retrieval
- Real-time updates and push notifications
- Scaling for millions of users and posts
5. Behavioral and Cultural Fit Questions
Apple places great importance on cultural fit and soft skills. Here are some behavioral questions you might encounter:
5.1. Teamwork and Collaboration
Q: Describe a situation where you had to work with a difficult team member. How did you handle it?
A: When answering this question, focus on:
- Your approach to understanding the team member’s perspective
- Communication strategies you used to improve the working relationship
- How you maintained professionalism and focus on the project goals
- The positive outcome or lessons learned from the experience
5.2. Problem-Solving and Innovation
Q: Tell me about a time when you came up with an innovative solution to a complex problem.
A: In your response, highlight:
- The complexity of the problem and why traditional solutions weren’t sufficient
- Your creative thinking process and how you arrived at the innovative solution
- How you implemented the solution and measured its success
- Any lessons learned or insights gained from the experience
5.3. Adaptability and Learning
Q: Describe a situation where you had to quickly learn a new technology or skill to complete a project.
A: Focus on:
- Your approach to learning the new technology or skill
- How you managed your time and prioritized learning alongside project deadlines
- Any challenges you faced and how you overcame them
- The outcome of the project and how your new knowledge contributed to its success
6. iOS-Specific Questions (for iOS Developer Positions)
If you’re applying for an iOS developer role, you can expect questions specific to iOS development. Here are some examples:
6.1. UIKit and SwiftUI
Q: Compare and contrast UIKit and SwiftUI. When would you choose one over the other?
A: Key points to discuss:
- UIKit is the older, more established framework, while SwiftUI is newer and more declarative
- UIKit offers more fine-grained control, while SwiftUI provides faster development and better state management
- UIKit is necessary for supporting older iOS versions, while SwiftUI requires iOS 13+
- SwiftUI is better for rapid prototyping and simpler apps, while UIKit may be preferred for complex, custom UI
6.2. App Lifecycle
Q: Explain the app lifecycle in iOS and how you would handle background fetch.
A: Discuss the following:
- The main app states: Not Running, Inactive, Active, Background, and Suspended
- App delegate methods for handling state transitions
- Implementing background fetch using BGAppRefreshTask
- Best practices for preserving battery life while performing background tasks
6.3. Core Data
Q: Describe Core Data and its benefits. How would you implement data persistence in an iOS app?
A: Key points to cover:
- Core Data is Apple’s framework for managing the model layer objects in your application
- Benefits include automatic data validation, undo/redo support, and efficient memory management
- Implementing Core Data involves setting up a managed object context, defining entity models, and using NSFetchedResultsController for efficient data retrieval
- Alternatives to Core Data, such as Realm or SQLite, and when they might be preferred
7. Tips for Success in Apple Interviews
To increase your chances of success in Apple interviews, consider the following tips:
7.1. Prepare Thoroughly
- Review fundamental computer science concepts, data structures, and algorithms
- Practice coding problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Stay updated on the latest Apple technologies and developer tools
- Familiarize yourself with Apple’s design principles and user interface guidelines
7.2. Communicate Effectively
- Clearly explain your thought process while solving problems
- Ask clarifying questions when necessary
- Be open to feedback and willing to iterate on your solutions
- Demonstrate enthusiasm for technology and Apple’s products
7.3. Show Your Passion for Innovation
- Highlight any personal projects or contributions to open-source software
- Discuss how you stay current with emerging technologies
- Share examples of how you’ve applied creative problem-solving in your work
7.4. Practice Behavioral Scenarios
- Prepare stories that demonstrate your teamwork, leadership, and problem-solving skills
- Use the STAR method (Situation, Task, Action, Result) to structure your responses
- Be prepared to discuss how you handle failure and learn from mistakes
8. Conclusion
Preparing for an Apple interview can be challenging, but with the right approach and mindset, you can significantly increase your chances of success. By thoroughly reviewing the types of questions covered in this guide, practicing your problem-solving skills, and showcasing your passion for technology and innovation, you’ll be well-equipped to tackle the interview process.
Remember that Apple values not only technical expertise but also creativity, collaboration, and a commitment to excellence. As you prepare, focus on developing a well-rounded skill set that demonstrates your ability to contribute to Apple’s mission of creating products that enrich people’s lives.
Good luck with your Apple interview! With thorough preparation and a positive attitude, you’ll be well on your way to joining one of the world’s most innovative technology companies.