Top Amazon Interview Questions: Ace Your Technical Interview
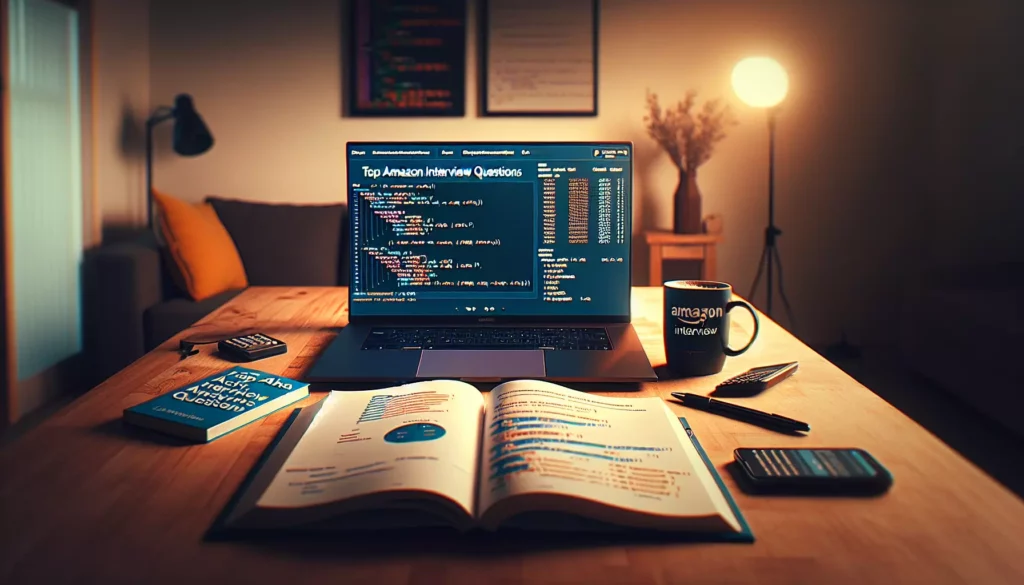
Are you preparing for a technical interview at Amazon? You’re in the right place! In this comprehensive guide, we’ll dive deep into the most common Amazon interview questions, provide expert tips on how to tackle them, and share strategies to help you stand out from other candidates. Whether you’re a seasoned software engineer or a fresh graduate, this article will equip you with the knowledge and confidence to excel in your Amazon interview.
Table of Contents
- Introduction to Amazon’s Interview Process
- Coding Questions
- System Design Questions
- Behavioral Questions
- Amazon’s Leadership Principles
- General Interview Tips
- Preparation Strategies
- Common Mistakes to Avoid
- Post-Interview Steps
- Conclusion
1. Introduction to Amazon’s Interview Process
Before we dive into specific questions, it’s essential to understand Amazon’s interview process. Typically, it consists of several rounds:
- Online Assessment: This may include coding challenges and aptitude tests.
- Phone Screens: One or two technical interviews conducted over the phone or video call.
- On-site Interviews: A series of face-to-face interviews (or virtual equivalents) covering technical, behavioral, and system design aspects.
Throughout these stages, Amazon evaluates candidates based on their technical skills, problem-solving abilities, and alignment with the company’s leadership principles. Let’s explore the types of questions you might encounter in each category.
2. Coding Questions
Coding questions form the backbone of Amazon’s technical interviews. These questions assess your ability to write efficient, clean, and correct code. Here are some common types of coding questions you might encounter:
2.1. Data Structures and Algorithms
Amazon frequently asks questions related to fundamental data structures and algorithms. Some popular topics include:
- Arrays and Strings
- Linked Lists
- Trees and Graphs
- Stacks and Queues
- Hash Tables
- Dynamic Programming
- Sorting and Searching
Let’s look at a sample question:
Question: Two Sum
Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Here’s a Python solution to this problem:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(result) # Output: [0, 1]
This solution uses a hash table (dictionary in Python) to achieve O(n) time complexity.
2.2. Object-Oriented Programming
Amazon also values strong object-oriented programming skills. You might be asked to design classes, implement inheritance, or showcase your understanding of OOP concepts. Here’s a sample question:
Question: Design a Parking Lot
Design a parking lot system that can handle multiple levels, different types of vehicles, and track available spaces.
Here’s a basic implementation in Python:
class ParkingSpot:
def __init__(self, level, spot_number, vehicle_type):
self.level = level
self.spot_number = spot_number
self.vehicle_type = vehicle_type
self.is_occupied = False
class Vehicle:
def __init__(self, vehicle_type, license_plate):
self.vehicle_type = vehicle_type
self.license_plate = license_plate
class ParkingLot:
def __init__(self, levels, spots_per_level):
self.levels = levels
self.spots_per_level = spots_per_level
self.spots = self._initialize_spots()
def _initialize_spots(self):
spots = []
for level in range(self.levels):
for spot in range(self.spots_per_level):
vehicle_type = 'car' if spot % 3 != 0 else 'truck'
spots.append(ParkingSpot(level, spot, vehicle_type))
return spots
def park_vehicle(self, vehicle):
for spot in self.spots:
if not spot.is_occupied and spot.vehicle_type == vehicle.vehicle_type:
spot.is_occupied = True
return f"Vehicle parked at level {spot.level}, spot {spot.spot_number}"
return "No available spots"
def remove_vehicle(self, level, spot_number):
for spot in self.spots:
if spot.level == level and spot.spot_number == spot_number:
if spot.is_occupied:
spot.is_occupied = False
return "Vehicle removed successfully"
else:
return "No vehicle at this spot"
return "Invalid spot"
# Example usage
parking_lot = ParkingLot(3, 10)
car = Vehicle('car', 'ABC123')
truck = Vehicle('truck', 'XYZ789')
print(parking_lot.park_vehicle(car))
print(parking_lot.park_vehicle(truck))
print(parking_lot.remove_vehicle(0, 1))
This implementation demonstrates basic OOP concepts like classes, inheritance, and encapsulation.
3. System Design Questions
For more senior positions, Amazon often includes system design questions to assess your ability to architect large-scale systems. These questions test your knowledge of distributed systems, scalability, and trade-offs in design decisions.
Common System Design Topics:
- Load Balancing
- Caching
- Database Sharding
- Microservices Architecture
- API Design
- Content Delivery Networks (CDNs)
Sample Question: Design a URL Shortener
Design a system that takes a long URL as input and returns a shortened URL. The system should also be able to take a shortened URL and redirect to the original long URL.
Here’s a high-level approach to this system design:
- API Endpoints:
- POST /shorten – Accept a long URL and return a shortened URL
- GET /{short_code} – Redirect to the original long URL
- Database:
- Use a relational database to store mappings between short codes and long URLs
- Table schema: (id, short_code, long_url, created_at, expiration_date)
- URL Shortening Algorithm:
- Generate a unique short code (e.g., 6-8 characters)
- Use a combination of timestamp and random characters
- Alternatively, use a base62 encoding of an auto-incrementing ID
- Caching:
- Implement a cache (e.g., Redis) to store frequently accessed URL mappings
- This reduces database load and improves response times
- Load Balancer:
- Distribute incoming requests across multiple application servers
- Analytics:
- Track click counts and other metrics for each shortened URL
Remember to discuss scalability, potential bottlenecks, and how you’d handle them in your design.
4. Behavioral Questions
Amazon places a strong emphasis on behavioral questions to assess your past experiences and how well you align with their leadership principles. These questions often follow the STAR (Situation, Task, Action, Result) format.
Sample Behavioral Questions:
- Tell me about a time when you had to work on a project with tight deadlines.
- Describe a situation where you had to deal with a difficult team member.
- Give an example of a time when you took a calculated risk.
- How do you handle criticism or feedback on your work?
- Tell me about a time when you had to make a decision without all the necessary information.
When answering these questions, be specific, provide context, and focus on your individual contributions and learnings from each experience.
5. Amazon’s Leadership Principles
Amazon’s 16 Leadership Principles are fundamental to their culture and decision-making process. Familiarize yourself with these principles and be prepared to demonstrate how you’ve embodied them in your past experiences.
Amazon’s Leadership Principles:
- Customer Obsession
- Ownership
- Invent and Simplify
- Are Right, A Lot
- Learn and Be Curious
- Hire and Develop the Best
- Insist on the Highest Standards
- Think Big
- Bias for Action
- Frugality
- Earn Trust
- Dive Deep
- Have Backbone; Disagree and Commit
- Deliver Results
- Strive to be Earth’s Best Employer
- Success and Scale Bring Broad Responsibility
For each principle, think of specific examples from your professional or personal life where you’ve demonstrated these qualities.
6. General Interview Tips
To make the most of your Amazon interview, keep these general tips in mind:
- Think Aloud: Communicate your thought process as you work through problems. This gives interviewers insight into your problem-solving approach.
- Ask Clarifying Questions: Don’t hesitate to ask for more information or clarification on problems. This shows you’re thorough and detail-oriented.
- Consider Edge Cases: When solving coding problems, always consider and discuss potential edge cases.
- Optimize Your Solutions: After providing an initial solution, discuss potential optimizations or trade-offs.
- Be Prepared for Follow-ups: Interviewers may ask you to extend or modify your solutions, so be ready to adapt.
- Show Enthusiasm: Demonstrate genuine interest in the role and the company.
- Have Questions Ready: Prepare thoughtful questions about the team, projects, and company culture.
7. Preparation Strategies
To maximize your chances of success, consider the following preparation strategies:
- Practice Coding: Use platforms like LeetCode, HackerRank, or AlgoCademy to practice coding problems regularly.
- Review Computer Science Fundamentals: Brush up on data structures, algorithms, and system design concepts.
- Mock Interviews: Conduct mock interviews with friends or use services that offer practice interviews with experienced professionals.
- Study Amazon’s Products and Services: Familiarize yourself with Amazon’s various products, services, and recent technological advancements.
- Behavioral Preparation: Prepare specific examples that demonstrate your skills and alignment with Amazon’s leadership principles.
- Time Management: Practice solving problems within time constraints to improve your efficiency.
- System Design Practice: For senior roles, practice designing large-scale systems and discussing trade-offs.
8. Common Mistakes to Avoid
Be aware of these common pitfalls during Amazon interviews:
- Jumping to Code Too Quickly: Take time to understand the problem and discuss your approach before coding.
- Neglecting Time Complexity: Always analyze and discuss the time and space complexity of your solutions.
- Ignoring Amazon’s Culture: Failing to demonstrate alignment with Amazon’s leadership principles can be a significant drawback.
- Being Vague in Behavioral Responses: Provide specific, detailed examples when answering behavioral questions.
- Not Testing Your Code: Always test your code with sample inputs and discuss potential edge cases.
- Overcomplicating Solutions: Start with a simple, working solution before optimizing or adding complexity.
- Poor Communication: Clearly explain your thought process and actively engage with the interviewer.
9. Post-Interview Steps
After your interview, consider these steps:
- Send a Thank-You Note: Express your appreciation for the interviewer’s time and reiterate your interest in the position.
- Reflect on Your Performance: Identify areas where you excelled and areas for improvement.
- Follow Up: If you haven’t heard back within the expected timeframe, don’t hesitate to follow up politely.
- Continue Learning: Regardless of the outcome, use the interview experience as a learning opportunity to improve your skills.
10. Conclusion
Preparing for an Amazon interview can be challenging, but with the right approach and mindset, you can significantly increase your chances of success. Remember to focus on strong problem-solving skills, clear communication, and alignment with Amazon’s leadership principles. By thoroughly preparing for coding questions, system design challenges, and behavioral interviews, you’ll be well-equipped to showcase your talents and secure that dream position at Amazon.
Good luck with your interview preparation, and don’t forget to leverage resources like AlgoCademy to enhance your coding skills and interview readiness. With dedication and the right preparation, you’ll be well on your way to joining one of the world’s most innovative companies. Happy coding, and best of luck in your Amazon interview!