Top Adobe Interview Questions: Your Ultimate Guide to Ace the Technical Interview
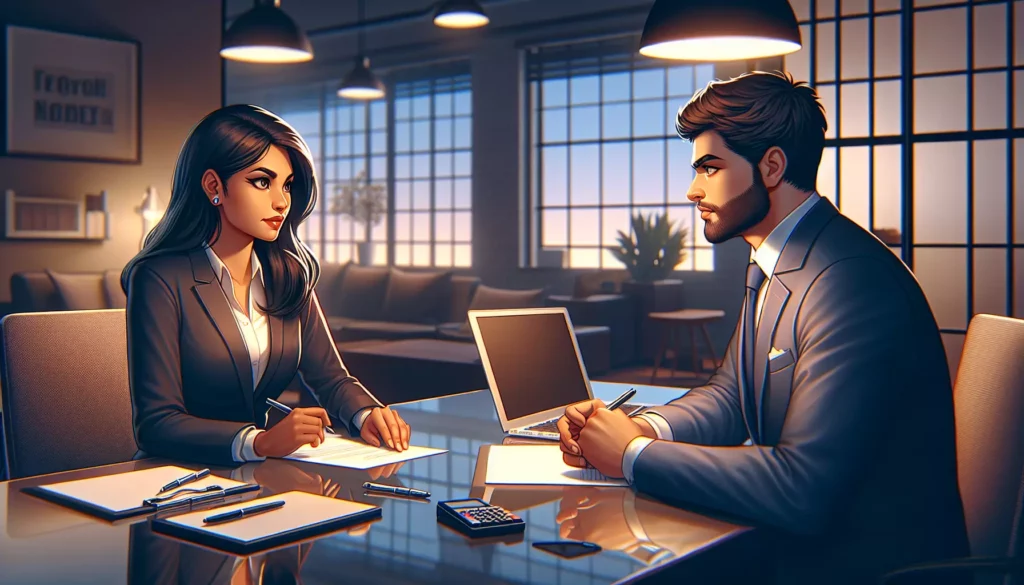
Are you preparing for an interview at Adobe? Congratulations on making it this far in your job search! Adobe is known for its innovative software products and creative solutions, making it an attractive destination for many tech professionals. However, their interview process can be challenging, especially when it comes to technical questions. In this comprehensive guide, we’ll explore some of the most common Adobe interview questions, provide insights into what interviewers are looking for, and offer tips to help you succeed.
Table of Contents
- Understanding Adobe’s Interview Process
- General Interview Questions
- Technical Interview Questions
- Coding Challenges and Problem-Solving Questions
- Behavioral and Situational Questions
- Adobe-Specific Questions
- Tips for Interview Success
- Conclusion
Understanding Adobe’s Interview Process
Before diving into specific questions, it’s essential to understand Adobe’s interview process. Typically, candidates go through several stages:
- Initial Screening: This may involve a phone call or online assessment to gauge your basic qualifications and interest.
- Technical Phone Interview: You’ll likely have one or more technical phone interviews with engineers or hiring managers.
- On-site Interviews: If you pass the initial stages, you’ll be invited for on-site interviews, which usually consist of multiple rounds with different team members.
- Final Decision: After the on-site interviews, the hiring team will make a decision based on your overall performance.
Throughout this process, Adobe looks for candidates who not only have strong technical skills but also align with their company culture and values. They prioritize creativity, innovation, and collaboration, so keep these qualities in mind as you prepare.
General Interview Questions
While technical skills are crucial, Adobe also wants to get to know you as a person and understand your motivations. Here are some general questions you might encounter:
- Why do you want to work at Adobe?
- What do you know about our company and products?
- Can you describe a challenging project you’ve worked on and how you overcame obstacles?
- How do you stay updated with the latest technologies and industry trends?
- Where do you see yourself in five years?
- What are your strengths and weaknesses as a software developer?
- How do you handle criticism or feedback on your work?
- Can you give an example of a time when you had to learn a new technology quickly?
When answering these questions, be honest and provide specific examples from your experience. Show enthusiasm for Adobe’s products and mission, and demonstrate how your skills and goals align with the company.
Technical Interview Questions
Adobe’s technical questions can vary depending on the specific role you’re applying for, but they often cover fundamental computer science concepts and programming skills. Here are some common technical topics and sample questions:
Data Structures and Algorithms
- Explain the difference between an array and a linked list. When would you use one over the other?
- How does a hash table work, and what is its time complexity for insertion and lookup operations?
- Describe the quicksort algorithm and its average-case time complexity.
- What is a binary search tree, and how would you implement an in-order traversal?
- Explain the concept of dynamic programming and provide an example of a problem that can be solved using this technique.
Object-Oriented Programming
- What are the four main principles of object-oriented programming?
- Explain the difference between composition and inheritance. When would you use one over the other?
- What is polymorphism, and how can it be implemented in your preferred programming language?
- Describe the SOLID principles of object-oriented design.
- What is the difference between an abstract class and an interface?
Web Development
- Explain the differences between GET and POST HTTP methods.
- What is CORS, and how does it work?
- Describe the concept of responsive design and how you would implement it.
- What are the main differences between HTTP/1.1 and HTTP/2?
- Explain the concept of server-side rendering and its benefits.
Database Systems
- What is normalization in database design, and why is it important?
- Explain the difference between INNER JOIN and LEFT JOIN in SQL.
- What is an index in a database, and how does it improve query performance?
- Describe the ACID properties of database transactions.
- What are the main differences between SQL and NoSQL databases?
System Design
- How would you design a URL shortening service like bit.ly?
- Explain the architecture of a distributed cache system like Memcached or Redis.
- How would you design a real-time chat application that can support millions of users?
- Describe the components and architecture of a content delivery network (CDN).
- How would you design a system to handle file synchronization across multiple devices (similar to Dropbox)?
When answering these technical questions, it’s important to not only provide the correct answer but also to explain your thought process. Interviewers are often more interested in how you approach problems than in whether you have memorized every detail.
Coding Challenges and Problem-Solving Questions
Adobe often includes coding challenges or problem-solving questions as part of their technical interviews. These are designed to assess your ability to write clean, efficient code and solve algorithmic problems. Here are some examples of the types of challenges you might encounter:
1. String Manipulation
Question: Write a function to determine if two strings are anagrams of each other.
Solution:
def are_anagrams(str1, str2):
# Remove spaces and convert to lowercase
str1 = str1.replace(" ", "").lower()
str2 = str2.replace(" ", "").lower()
# Check if lengths are equal
if len(str1) != len(str2):
return False
# Create dictionaries to store character counts
char_count1 = {}
char_count2 = {}
# Count characters in both strings
for char in str1:
char_count1[char] = char_count1.get(char, 0) + 1
for char in str2:
char_count2[char] = char_count2.get(char, 0) + 1
# Compare character counts
return char_count1 == char_count2
# Test the function
print(are_anagrams("listen", "silent")) # True
print(are_anagrams("hello", "world")) # False
2. Array Manipulation
Question: Given an array of integers, find the contiguous subarray with the largest sum.
Solution: This problem can be solved using Kadane’s algorithm.
def max_subarray_sum(arr):
max_sum = float('-inf')
current_sum = 0
for num in arr:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
print(max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4])) # Output: 6
3. Tree Traversal
Question: Implement an in-order traversal of a binary tree without using recursion.
Solution:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
result.append(current.val)
current = current.right
return result
# Test the function
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # Output: [4, 2, 5, 1, 3]
4. Dynamic Programming
Question: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
Solution:
def min_coins(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for i in range(1, amount + 1):
for coin in coins:
if coin <= i:
dp[i] = min(dp[i], dp[i - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
# Test the function
print(min_coins([1, 2, 5], 11)) # Output: 3 (5 + 5 + 1)
print(min_coins([2], 3)) # Output: -1 (not possible)
When solving these coding challenges, remember to:
- Clarify any ambiguities in the problem statement before starting.
- Think out loud and explain your approach as you work through the problem.
- Consider edge cases and handle them appropriately.
- Analyze the time and space complexity of your solution.
- If time allows, optimize your initial solution or discuss potential optimizations.
Behavioral and Situational Questions
Adobe values soft skills and cultural fit as much as technical abilities. You’re likely to encounter behavioral and situational questions that assess how you work in a team, handle challenges, and align with Adobe’s values. Here are some examples:
- Describe a time when you had to work on a project with a tight deadline. How did you manage your time and ensure the project was completed successfully?
- Tell me about a situation where you had a disagreement with a team member. How did you resolve it?
- Can you share an example of a time when you took initiative to improve a process or solve a problem at work?
- How do you handle working on multiple projects with competing priorities?
- Describe a situation where you had to explain a complex technical concept to a non-technical stakeholder. How did you approach this?
- Tell me about a time when you received constructive criticism. How did you respond, and what did you learn from it?
- Can you give an example of how you’ve contributed to fostering an inclusive work environment?
- Describe a situation where you had to adapt to a significant change at work. How did you handle it?
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses. Provide specific examples from your past experiences, and focus on demonstrating qualities such as leadership, teamwork, adaptability, and problem-solving skills.
Adobe-Specific Questions
To stand out in your Adobe interview, it’s crucial to demonstrate knowledge of the company’s products, technologies, and industry position. Here are some Adobe-specific questions you might encounter:
- Which Adobe products are you most familiar with, and how have you used them in your work or personal projects?
- How do you think Adobe’s Creative Cloud has changed the landscape for creative professionals?
- What do you think are the biggest challenges facing Adobe in the current tech landscape?
- Can you explain the concept of Digital Experience Platforms and how Adobe Experience Cloud fits into this category?
- How do you think AI and machine learning are impacting Adobe’s products and the creative industry as a whole?
- What are your thoughts on Adobe’s acquisition strategy (e.g., Figma, Magento, Marketo)? How do you think these acquisitions benefit the company?
- How do you see Adobe’s role in the future of web development, particularly with technologies like WebAssembly?
- What do you know about Adobe’s open-source initiatives, such as Apache Flex or PhoneGap?
To prepare for these questions, spend time researching Adobe’s products, recent news, and industry trends. Familiarize yourself with their annual reports, blog posts, and technology showcases to gain insights into their strategic direction and innovations.
Tips for Interview Success
To increase your chances of success in an Adobe interview, consider the following tips:
- Practice, practice, practice: Use platforms like LeetCode, HackerRank, or AlgoCademy to sharpen your coding skills and practice solving algorithmic problems.
- Review computer science fundamentals: Ensure you have a solid understanding of data structures, algorithms, and system design principles.
- Stay up-to-date with industry trends: Follow tech news, particularly in areas relevant to Adobe’s products and services.
- Prepare your own questions: Have thoughtful questions ready to ask your interviewers about the role, team, and company.
- Use the STAR method: When answering behavioral questions, structure your responses using the Situation, Task, Action, Result format.
- Show enthusiasm: Demonstrate genuine interest in Adobe’s products and mission throughout the interview process.
- Be honest: If you don’t know something, admit it and explain how you would go about finding the answer.
- Practice mock interviews: Conduct mock interviews with friends or mentors to get comfortable with the interview format and receive feedback.
- Highlight your unique experiences: Emphasize any relevant projects, internships, or contributions that set you apart from other candidates.
- Follow up: After the interview, send a thank-you email to your interviewers, reiterating your interest in the position.
Conclusion
Preparing for an Adobe interview can be challenging, but with the right approach and mindset, you can increase your chances of success. Remember that the interview process is not just about demonstrating your technical skills but also about showcasing your problem-solving abilities, communication skills, and cultural fit.
By thoroughly preparing for the types of questions outlined in this guide, practicing your coding skills, and staying informed about Adobe and the tech industry, you’ll be well-equipped to make a strong impression during your interview.
Good luck with your Adobe interview! Remember to stay calm, be yourself, and let your passion for technology and creativity shine through. With proper preparation and a positive attitude, you’ll be one step closer to joining one of the world’s most innovative software companies.