Top 15 Most Common Coding Interview Questions You Need to Master
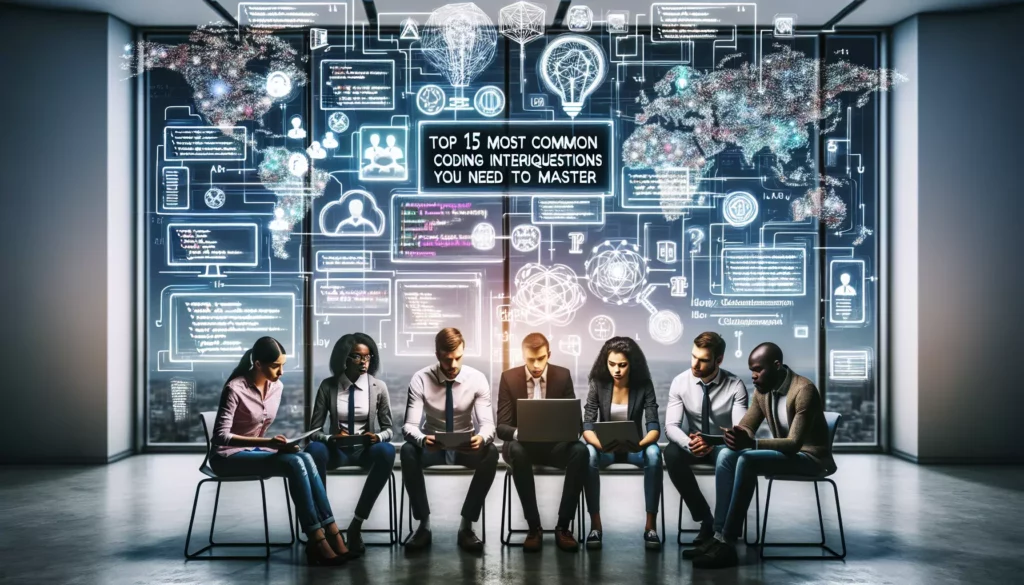
If you’re preparing for a technical interview at a major tech company, you’re likely feeling a mix of excitement and anxiety. The coding interview process can be daunting, but with the right preparation, you can approach it with confidence. In this comprehensive guide, we’ll explore the 15 most common coding interview questions you’re likely to encounter, along with strategies to tackle them effectively.
Why These Questions Matter
Before we dive into the specific questions, it’s important to understand why these particular problems are so frequently asked in coding interviews. These questions are designed to assess several key aspects of a candidate’s abilities:
- Problem-solving skills
- Algorithmic thinking
- Code efficiency and optimization
- Understanding of data structures
- Ability to communicate technical concepts
By mastering these common questions, you’ll not only be better prepared for your interviews but also develop a strong foundation in computer science principles that will serve you well throughout your career.
1. Two Sum
The Two Sum problem is a classic algorithmic question that tests your ability to work with arrays and hash tables.
Problem Statement:
Given an array of integers and a target sum, return indices of the two numbers in the array such that they add up to the target.
Example:
Input: nums = [2, 7, 11, 15], target = 9
Output: [0, 1]
Explanation: nums[0] + nums[1] = 2 + 7 = 9
Solution Approach:
The most efficient solution uses a hash table to store complements:
def two_sum(nums, target):
complement_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_map:
return [complement_map[complement], i]
complement_map[num] = i
return []
Time Complexity: O(n)
Space Complexity: O(n)
2. Reverse a Linked List
This problem tests your understanding of linked list data structures and your ability to manipulate pointers.
Problem Statement:
Given the head of a singly linked list, reverse the list and return the reversed list.
Example:
Input: 1 -> 2 -> 3 -> 4 -> 5
Output: 5 -> 4 -> 3 -> 2 -> 1
Solution Approach:
Use three pointers to reverse the links:
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Time Complexity: O(n)
Space Complexity: O(1)
3. Valid Parentheses
This problem tests your ability to work with stacks and handle string manipulation.
Problem Statement:
Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid. An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
Example:
Input: "()[]{}"
Output: true
Input: "([)]"
Output: false
Solution Approach:
Use a stack to keep track of opening brackets:
def is_valid_parentheses(s):
stack = []
bracket_map = {")": "(", "}": "{", "]": "["}
for char in s:
if char in bracket_map:
if not stack or stack[-1] != bracket_map[char]:
return False
stack.pop()
else:
stack.append(char)
return len(stack) == 0
Time Complexity: O(n)
Space Complexity: O(n)
4. Maximum Subarray
This problem tests your understanding of dynamic programming and your ability to optimize algorithms.
Problem Statement:
Given an integer array nums, find the contiguous subarray (containing at least one number) which has the largest sum and return its sum.
Example:
Input: nums = [-2,1,-3,4,-1,2,1,-5,4]
Output: 6
Explanation: [4,-1,2,1] has the largest sum = 6.
Solution Approach:
Use Kadane’s algorithm:
def max_subarray(nums):
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
Time Complexity: O(n)
Space Complexity: O(1)
5. Merge Two Sorted Lists
This problem tests your ability to work with linked lists and merge algorithms.
Problem Statement:
Merge two sorted linked lists and return it as a new sorted list. The new list should be made by splicing together the nodes of the first two lists.
Example:
Input: l1 = [1,2,4], l2 = [1,3,4]
Output: [1,1,2,3,4,4]
Solution Approach:
Use a dummy head and iterate through both lists:
def merge_two_lists(l1, l2):
dummy = ListNode(0)
current = dummy
while l1 and l2:
if l1.val < l2.val:
current.next = l1
l1 = l1.next
else:
current.next = l2
l2 = l2.next
current = current.next
current.next = l1 if l1 else l2
return dummy.next
Time Complexity: O(n + m)
Space Complexity: O(1)
6. Binary Search
This problem tests your understanding of divide-and-conquer algorithms and your ability to implement efficient search techniques.
Problem Statement:
Given a sorted array of integers, implement a function to search for a target value. If the target exists, return its index; otherwise, return -1.
Example:
Input: nums = [-1,0,3,5,9,12], target = 9
Output: 4
Explanation: 9 exists in nums and its index is 4
Solution Approach:
Implement the binary search algorithm:
def binary_search(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
return mid
elif nums[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Time Complexity: O(log n)
Space Complexity: O(1)
7. Longest Palindromic Substring
This problem tests your ability to work with strings and implement dynamic programming solutions.
Problem Statement:
Given a string s, return the longest palindromic substring in s.
Example:
Input: s = "babad"
Output: "bab"
Note: "aba" is also a valid answer.
Solution Approach:
Use the expand around center technique:
def longest_palindrome(s):
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left+1:right]
result = ""
for i in range(len(s)):
# Odd length palindromes
palindrome1 = expand_around_center(i, i)
# Even length palindromes
palindrome2 = expand_around_center(i, i+1)
result = max(result, palindrome1, palindrome2, key=len)
return result
Time Complexity: O(n^2)
Space Complexity: O(1)
8. Implement a Queue using Stacks
This problem tests your understanding of data structures and your ability to implement one structure using another.
Problem Statement:
Implement a first in first out (FIFO) queue using only two stacks. The implemented queue should support all the functions of a normal queue (push, pop, peek, empty).
Solution Approach:
Use two stacks, one for enqueue operations and one for dequeue operations:
class MyQueue:
def __init__(self):
self.stack1 = [] # For enqueue
self.stack2 = [] # For dequeue
def push(self, x: int) -> None:
self.stack1.append(x)
def pop(self) -> int:
self.peek()
return self.stack2.pop()
def peek(self) -> int:
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
return self.stack2[-1]
def empty(self) -> bool:
return not self.stack1 and not self.stack2
Time Complexity: O(1) amortized for all operations
Space Complexity: O(n)
9. Validate Binary Search Tree
This problem tests your understanding of binary search trees and recursive algorithms.
Problem Statement:
Given the root of a binary tree, determine if it is a valid binary search tree (BST).
Solution Approach:
Use recursive in-order traversal with range checking:
def is_valid_bst(root):
def validate(node, low=float('-inf'), high=float('inf')):
if not node:
return True
if node.val <= low or node.val >= high:
return False
return validate(node.left, low, node.val) and validate(node.right, node.val, high)
return validate(root)
Time Complexity: O(n)
Space Complexity: O(h), where h is the height of the tree
10. Climbing Stairs
This problem tests your ability to recognize and implement dynamic programming solutions.
Problem Statement:
You are climbing a staircase. It takes n steps to reach the top. Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?
Example:
Input: n = 3
Output: 3
Explanation: There are three ways to climb to the top.
1. 1 step + 1 step + 1 step
2. 1 step + 2 steps
3. 2 steps + 1 step
Solution Approach:
Use dynamic programming with bottom-up approach:
def climb_stairs(n):
if n <= 2:
return n
dp = [0] * (n + 1)
dp[1] = 1
dp[2] = 2
for i in range(3, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Time Complexity: O(n)
Space Complexity: O(n)
11. Find the Kth Largest Element in an Array
This problem tests your understanding of sorting algorithms and selection algorithms.
Problem Statement:
Given an unsorted array of integers nums and an integer k, return the kth largest element in the array.
Example:
Input: nums = [3,2,1,5,6,4], k = 2
Output: 5
Solution Approach:
Use QuickSelect algorithm:
import random
def find_kth_largest(nums, k):
def partition(left, right, pivot_index):
pivot = nums[pivot_index]
nums[pivot_index], nums[right] = nums[right], nums[pivot_index]
store_index = left
for i in range(left, right):
if nums[i] < pivot:
nums[store_index], nums[i] = nums[i], nums[store_index]
store_index += 1
nums[right], nums[store_index] = nums[store_index], nums[right]
return store_index
def select(left, right, k_smallest):
if left == right:
return nums[left]
pivot_index = random.randint(left, right)
pivot_index = partition(left, right, pivot_index)
if k_smallest == pivot_index:
return nums[k_smallest]
elif k_smallest < pivot_index:
return select(left, pivot_index - 1, k_smallest)
else:
return select(pivot_index + 1, right, k_smallest)
return select(0, len(nums) - 1, len(nums) - k)
Time Complexity: O(n) average case, O(n^2) worst case
Space Complexity: O(1)
12. Implement Trie (Prefix Tree)
This problem tests your ability to implement advanced data structures and understand their applications.
Problem Statement:
Implement a trie with insert, search, and startsWith methods.
Solution Approach:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word: str) -> None:
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def search(self, word: str) -> bool:
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end
def startsWith(self, prefix: str) -> bool:
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
Time Complexity: O(m) for all operations, where m is the length of the word
Space Complexity: O(n * m), where n is the number of words
13. Lowest Common Ancestor of a Binary Tree
This problem tests your understanding of tree structures and recursive algorithms.
Problem Statement:
Given a binary tree, find the lowest common ancestor (LCA) of two given nodes in the tree.
Solution Approach:
Use recursive depth-first search:
def lowest_common_ancestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
Time Complexity: O(n)
Space Complexity: O(h), where h is the height of the tree
14. Course Schedule (Detect Cycle in Directed Graph)
This problem tests your understanding of graph algorithms and cycle detection.
Problem Statement:
There are a total of numCourses courses you have to take, labeled from 0 to numCourses-1. Some courses may have prerequisites. Given the total number of courses and a list of prerequisite pairs, is it possible for you to finish all courses?
Example:
Input: numCourses = 2, prerequisites = [[1,0]]
Output: true
Explanation: There are a total of 2 courses to take.
To take course 1 you should have finished course 0. So it is possible.
Solution Approach:
Use depth-first search to detect cycles:
from collections import defaultdict
def can_finish(num_courses, prerequisites):
graph = defaultdict(list)
for course, prereq in prerequisites:
graph[course].append(prereq)
def is_cyclic(course, path):
if course in path:
return True
if course in visited:
return False
path.add(course)
visited.add(course)
for prereq in graph[course]:
if is_cyclic(prereq, path):
return True
path.remove(course)
return False
visited = set()
for course in range(num_courses):
if is_cyclic(course, set()):
return False
return True
Time Complexity: O(V + E), where V is the number of courses and E is the number of prerequisites
Space Complexity: O(V)
15. Serialize and Deserialize Binary Tree
This problem tests your ability to work with complex data structures and implement encoding/decoding algorithms.
Problem Statement:
Design an algorithm to serialize and deserialize a binary tree. There is no restriction on how your serialization/deserialization algorithm should work. You just need to ensure that a binary tree can be serialized to a string and this string can be deserialized to the original tree structure.
Solution Approach:
Use preorder traversal for serialization and a queue for deserialization:
class Codec:
def serialize(self, root):
def dfs(node):
if not node:
return "null"
return f"{node.val},{dfs(node.left)},{dfs(node.right)}"
return dfs(root)
def deserialize(self, data):
def dfs():
val = next(values)
if val == "null":
return None
node = TreeNode(int(val))
node.left = dfs()
node.right = dfs()
return node
values = iter(data.split(","))
return dfs()
Time Complexity: O(n) for both serialize and deserialize
Space Complexity: O(n) for both serialize and deserialize
Conclusion
Mastering these 15 common coding interview questions will significantly boost your confidence and preparedness for technical interviews. Remember, the key to success is not just knowing the solutions, but understanding the underlying principles and being able to explain your thought process clearly.
As you practice these problems, focus on:
- Understanding the problem thoroughly before coding
- Considering edge cases and potential optimizations
- Communicating your approach effectively
- Writing clean, readable code
- Analyzing time and space complexity
Keep in mind that interviewers are often more interested in your problem-solving approach than in whether you can recite a perfect solution from memory. Be prepared to discuss trade-offs between different solutions and to adapt your approach based on additional constraints or requirements that may be introduced during the interview.
Lastly, don’t forget to leverage resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you master these concepts and prepare effectively for your coding interviews. With dedicated practice and the right resources, you’ll be well-equipped to tackle even the most challenging technical interviews at top tech companies.