Top 100 Frontend Interview Questions: Your Ultimate Guide to Acing Technical Interviews
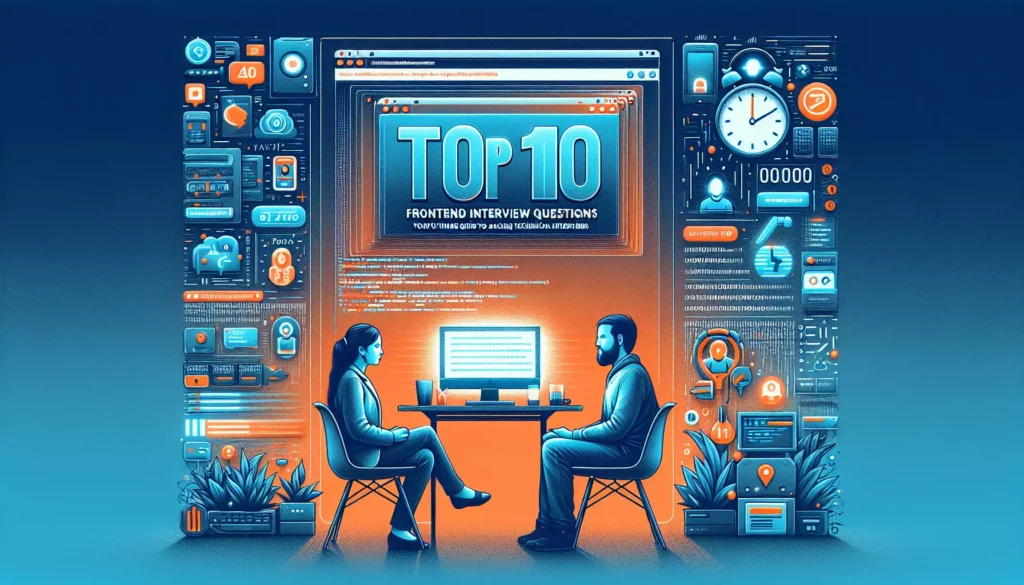
Are you gearing up for a frontend developer interview? Whether you’re a seasoned pro or just starting your journey in web development, being well-prepared for technical interviews is crucial. In this comprehensive guide, we’ll explore the top 100 questions frequently asked in frontend interviews, covering a wide range of topics from HTML and CSS to JavaScript, frameworks, and beyond.
Table of Contents
- HTML Questions
- CSS Questions
- JavaScript Questions
- Framework-specific Questions
- Performance Optimization Questions
- Accessibility Questions
- Testing and Debugging Questions
- Version Control Questions
- General Web Development Questions
- Conclusion
HTML Questions
1. What is the purpose of the DOCTYPE declaration?
The DOCTYPE declaration specifies the document type and version of HTML being used. It helps browsers render the page correctly by determining which rendering mode to use.
2. Explain the difference between <div> and <span> tags.
The <div> tag is a block-level element used for grouping and structuring content, while the <span> tag is an inline element used for applying styles to specific portions of text within a larger block of content.
3. What are semantic HTML tags? Give examples.
Semantic HTML tags are elements that carry meaning about the structure and content of a web page. Examples include <header>, <nav>, <article>, <section>, <aside>, and <footer>.
4. How do you create a hyperlink that opens in a new tab?
You can use the target attribute with the value “_blank” in the <a> tag:
<a href="https://example.com" target="_blank">Link Text</a>
5. What is the purpose of the <meta> tag?
The <meta> tag provides metadata about the HTML document, such as character encoding, viewport settings for responsive design, and description for search engines.
6. Explain the difference between <script>, <script async>, and <script defer>.
<script> blocks parsing and executes immediately. <script async> downloads asynchronously and executes as soon as it’s available. <script defer> downloads asynchronously but executes only after the document has finished parsing.
7. What is the purpose of the <canvas> element?
The <canvas> element is used to draw graphics, animations, and other visual images on the fly using JavaScript.
8. How do you embed video in HTML5?
You can use the <video> tag to embed video in HTML5:
<video width="320" height="240" controls>
<source src="movie.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
9. What is the purpose of the <figure> and <figcaption> elements?
The <figure> element is used to encapsulate media content (like images, diagrams, or videos) along with its caption. The <figcaption> element is used within <figure> to provide a caption for the content.
10. Explain the concept of HTML5 form validation.
HTML5 introduced built-in form validation features using attributes like required, pattern, min, max, and type. These allow for client-side validation without the need for JavaScript.
CSS Questions
11. What is the box model in CSS?
The box model is a fundamental concept in CSS that describes how elements are rendered. It consists of content, padding, border, and margin.
12. Explain the difference between margin and padding.
Margin is the space outside an element, creating distance from other elements. Padding is the space inside an element, creating distance between the content and the element’s border.
13. What is the purpose of the z-index property?
The z-index property controls the stacking order of elements on a web page. Elements with a higher z-index value appear on top of elements with lower values.
14. How do you center an element horizontally and vertically?
One way to center an element both horizontally and vertically is using flexbox:
.parent {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
15. What is the difference between display: none and visibility: hidden?
display: none removes the element from the document flow and hides it completely. visibility: hidden hides the element but keeps its space in the layout.
16. Explain the concept of CSS specificity.
CSS specificity is a set of rules that determines which styles are applied to an element when there are conflicting rules. It’s calculated based on the selector’s components (inline styles, IDs, classes, elements).
17. What are pseudo-classes and pseudo-elements?
Pseudo-classes select elements based on a specific state (e.g., :hover, :focus). Pseudo-elements allow you to style a specific part of an element (e.g., ::before, ::after).
18. How do you implement a responsive design?
Responsive design can be implemented using techniques like fluid grids, flexible images, and media queries to adapt the layout and styling based on the device’s screen size.
19. What is the purpose of the @media rule?
The @media rule is used to apply different styles for different media types/devices. It’s a key component in creating responsive designs.
20. Explain the concept of CSS Grid.
CSS Grid is a two-dimensional layout system that allows you to create complex grid-based layouts with rows and columns. It provides powerful control over the sizing and positioning of grid items.
JavaScript Questions
21. What is the difference between let, const, and var?
var has function scope and is hoisted. let and const have block scope. const creates a read-only reference to a value, while let allows reassignment.
22. Explain closure in JavaScript.
A closure is a function that has access to variables in its outer (enclosing) lexical scope, even after the outer function has returned.
23. What is the purpose of the ‘use strict’ directive?
‘use strict’ enables strict mode in JavaScript, which catches common coding errors and prevents the use of certain error-prone features.
24. How does prototypal inheritance work in JavaScript?
In JavaScript, objects can inherit properties and methods from other objects through their prototype chain. Each object has an internal link to another object called its prototype.
25. Explain the concept of event bubbling.
Event bubbling is a mechanism where an event triggered on a nested element “bubbles up” through its parent elements in the DOM tree.
26. What is the purpose of the ‘this’ keyword in JavaScript?
The ‘this’ keyword refers to the object that is executing the current function. Its value is determined by how a function is called.
27. How do you handle asynchronous operations in JavaScript?
Asynchronous operations can be handled using callbacks, Promises, or async/await syntax. These allow non-blocking execution of code.
28. What is the difference between == and === operators?
== performs type coercion before comparison, while === compares both value and type without coercion.
29. Explain the concept of hoisting in JavaScript.
Hoisting is JavaScript’s default behavior of moving declarations to the top of their respective scopes during the compilation phase.
30. What are arrow functions and how do they differ from regular functions?
Arrow functions provide a concise syntax for writing function expressions. They have a lexical ‘this’ binding and cannot be used as constructors.
Framework-specific Questions
31. What is the virtual DOM in React?
The virtual DOM is a lightweight copy of the actual DOM. React uses it to improve performance by minimizing direct manipulation of the DOM.
32. Explain the concept of components in modern frontend frameworks.
Components are reusable, self-contained pieces of UI that encapsulate HTML, CSS, and JavaScript. They help in building modular and maintainable applications.
33. What is state management and why is it important?
State management refers to the handling of an application’s data. It’s crucial for maintaining data consistency across components and managing complex application states.
34. How does two-way data binding work in Angular?
Two-way data binding in Angular allows automatic synchronization of data between the model and the view. Changes in either are reflected in the other.
35. What are hooks in React and how do they work?
Hooks are functions that allow you to use state and other React features in functional components. They provide a more direct API to React concepts.
36. Explain the concept of directives in Vue.js.
Directives in Vue.js are special attributes with the v- prefix. They apply special reactive behavior to the rendered DOM.
37. What is the purpose of NgModules in Angular?
NgModules in Angular are containers for a cohesive block of code dedicated to an application domain, a workflow, or a closely related set of capabilities.
38. How does React handle form submissions?
React typically uses controlled components for form handling, where form data is controlled by React state.
39. What is the Vue.js lifecycle?
The Vue.js lifecycle consists of several hooks (like created, mounted, updated, destroyed) that allow you to run code at specific stages of a component’s lifecycle.
40. Explain the concept of dependency injection in Angular.
Dependency injection in Angular is a design pattern where a class receives its dependencies from external sources rather than creating them itself.
Performance Optimization Questions
41. What techniques can you use to improve website loading speed?
Techniques include minifying CSS/JS, optimizing images, using a Content Delivery Network (CDN), implementing lazy loading, and leveraging browser caching.
42. How do you minimize reflows and repaints?
Minimize reflows and repaints by batching DOM changes, using CSS transforms for animations, and avoiding frequent style changes.
43. What is code splitting and how does it improve performance?
Code splitting is the practice of splitting your code into smaller chunks which can be loaded on demand, reducing the initial load time of the application.
44. How can you optimize images for the web?
Optimize images by using appropriate formats (JPEG for photographs, PNG for graphics with transparency), compressing them, and using responsive images.
45. What is the purpose of lazy loading?
Lazy loading defers the loading of non-critical resources until they are needed, improving initial load time and saving bandwidth.
46. How do you handle memory leaks in JavaScript?
Handle memory leaks by properly managing event listeners, avoiding circular references, and using weak references when appropriate.
47. What is the role of a service worker in performance optimization?
Service workers can improve performance by enabling offline functionality, background sync, and push notifications, as well as caching resources for faster subsequent loads.
48. How can you optimize the critical rendering path?
Optimize the critical rendering path by minimizing the number of critical resources, reducing the critical path length, and lowering the number of critical bytes.
49. What is tree shaking and how does it improve performance?
Tree shaking is a technique used in modern JavaScript build tools to eliminate dead code, reducing the final bundle size.
50. How do you implement infinite scrolling efficiently?
Implement efficient infinite scrolling by using virtual scrolling techniques, loading data in chunks, and unloading off-screen elements to manage memory usage.
Accessibility Questions
51. What is ARIA and how is it used?
ARIA (Accessible Rich Internet Applications) is a set of attributes that define ways to make web content and applications more accessible to people with disabilities.
52. How do you ensure keyboard accessibility in web applications?
Ensure keyboard accessibility by providing focus indicators, using proper tab order, and ensuring all interactive elements can be accessed and operated using only a keyboard.
53. What are some common color contrast issues and how do you address them?
Common color contrast issues include insufficient contrast between text and background. Address them by ensuring sufficient contrast ratios and avoiding color as the only means of conveying information.
54. How do you make images accessible?
Make images accessible by providing descriptive alt text, using appropriate text alternatives for complex images, and ensuring decorative images are properly marked.
55. What is the role of semantic HTML in accessibility?
Semantic HTML plays a crucial role in accessibility by providing meaning and structure to content, which helps assistive technologies interpret and navigate the page.
56. How do you create accessible forms?
Create accessible forms by using proper labels, providing clear instructions, using fieldsets and legends for grouping, and ensuring error messages are clear and accessible.
57. What are skip links and why are they important?
Skip links are hidden links that allow keyboard users to bypass repetitive content and navigate directly to the main content. They improve navigation efficiency for keyboard and screen reader users.
58. How do you ensure accessibility of dynamic content?
Ensure accessibility of dynamic content by using ARIA live regions, managing focus appropriately, and providing clear feedback for user actions.
59. What is the purpose of the lang attribute in HTML?
The lang attribute specifies the language of the element’s content, which is crucial for screen readers to pronounce content correctly and for search engines to identify the language of the page.
60. How do you make video and audio content accessible?
Make video and audio content accessible by providing captions, transcripts, and audio descriptions, as well as ensuring media players have keyboard-accessible controls.
Testing and Debugging Questions
61. What is unit testing and why is it important?
Unit testing involves testing individual components or functions in isolation. It’s important for catching bugs early, improving code quality, and facilitating refactoring.
62. How do you debug JavaScript code?
Debug JavaScript using browser developer tools, console.log statements, breakpoints, and debugging tools in IDEs.
63. What is the purpose of end-to-end testing?
End-to-end testing verifies that the entire application works as expected from start to finish, simulating real user scenarios.
64. How do you handle cross-browser testing?
Handle cross-browser testing by using browser compatibility libraries, automated testing tools, and manual testing on different browsers and devices.
65. What is the difference between mocks and stubs in testing?
Mocks are pre-programmed objects that verify behavior, while stubs provide canned answers to calls made during the test.
66. How do you test asynchronous code?
Test asynchronous code using callbacks, Promises, or async/await syntax in combination with testing framework features designed for asynchronous testing.
67. What is Test-Driven Development (TDD)?
TDD is a development process where tests are written before the actual code. The cycle involves writing a failing test, writing code to pass the test, and then refactoring.
68. How do you ensure code coverage in your tests?
Ensure code coverage by using code coverage tools, setting coverage thresholds, and writing tests that cover different code paths and edge cases.
69. What are some common frontend performance testing tools?
Common frontend performance testing tools include Lighthouse, WebPageTest, Chrome DevTools Performance tab, and GTmetrix.
70. How do you debug CSS issues?
Debug CSS issues using browser developer tools to inspect elements, modify styles in real-time, and analyze the CSS cascade and specificity.
Version Control Questions
71. What is Git and why is it used?
Git is a distributed version control system used to track changes in source code during software development. It facilitates collaboration and maintains a history of code changes.
72. Explain the difference between Git and GitHub.
Git is the version control system, while GitHub is a web-based hosting service for Git repositories that provides additional collaboration features.
73. What is a branch in Git and how is it used?
A branch in Git is a lightweight movable pointer to a commit. It’s used to develop features isolated from each other and to manage different versions of code.
74. How do you resolve merge conflicts in Git?
Resolve merge conflicts by manually editing the conflicting files, choosing which changes to keep, and then committing the resolved files.
75. What is the purpose of a .gitignore file?
The .gitignore file specifies intentionally untracked files that Git should ignore, such as build artifacts, temporary files, or sensitive information.
76. Explain the concept of rebasing in Git.
Rebasing is the process of moving or combining a sequence of commits to a new base commit. It’s used to maintain a linear project history.
77. What is a pull request?
A pull request is a method of submitting contributions to a project. It allows developers to notify team members about changes pushed to a branch in a repository.
78. How do you undo the last commit in Git?
To undo the last commit while keeping the changes, use:
git reset --soft HEAD~1
To completely discard the last commit and its changes, use:
git reset --hard HEAD~1
79. What is Git stash and when would you use it?
Git stash temporarily shelves changes you’ve made to your working copy so you can work on something else, and then come back and re-apply them later.
80. How do you create and apply a patch in Git?
Create a patch using:
git diff > changes.patch
Apply a patch using:
git apply changes.patch
General Web Development Questions
81. What is the difference between HTTP and HTTPS?
HTTP is the standard protocol for transmitting data over the internet. HTTPS is the secure version that encrypts the data during transmission.
82. Explain the concept of RESTful APIs.
RESTful APIs are web services that use HTTP methods (GET, POST, PUT, DELETE) to perform CRUD operations on resources, following the principles of Representational State Transfer (REST).
83. What is CORS and how does it work?
Cross-Origin Resource Sharing (CORS) is a security mechanism that allows a web page from one domain to request resources from a different domain.
84. What are Web Workers and how are they used?
Web Workers allow scripts to run in background threads, separate from the main execution thread of a web application, enabling better performance for CPU-intensive tasks.
85. Explain the concept of Progressive Web Apps (PWAs).
PWAs are web applications that use modern web capabilities to deliver an app-like experience to users, including offline functionality, push notifications, and home screen installation.
86. What is the purpose of a Content Delivery Network (CDN)?
A CDN distributes content from a website across multiple, geographically diverse servers to reduce latency and improve load times for users.
87. How do you implement client-side routing in a single-page application?
Client-side routing in SPAs is typically implemented using JavaScript libraries or frameworks that manipulate the browser’s history API to change the URL without triggering a full page reload.
88. What is the role of webpack in modern frontend development?
Webpack is a module bundler that helps manage dependencies, optimize assets, and bundle various resources (JavaScript, CSS, images) for deployment.
89. Explain the concept of server-side rendering (SSR).
Server-side rendering involves generating the full HTML for a page on the server in response to a user’s request, which can improve initial load time and SEO.
90. What are microservices and how do they relate to frontend development?
Microservices are an architectural style where an application is composed of small, independent services. In frontend development, this often relates to consuming APIs from multiple microservices.
91. How do you handle state persistence in web applications?
State persistence can be handled using techniques like local storage, session storage, cookies, or server-side storage depending on the requirements and security considerations.
92. What is the role of TypeScript in modern frontend development?
TypeScript adds static typing to JavaScript, providing better tooling, earlier error detection, and improved code maintainability in large-scale applications.
93. Explain the concept of JAMstack.
JAMstack (JavaScript, APIs, Markup) is an architecture designed to make the web faster, more secure, and easier to scale by pre-rendering pages and serving them directly from a CDN.
94. What are Web Components?
Web Components are a set of web platform APIs that allow you to create reusable custom elements with their functionality encapsulated away from the rest of your code.
95. How do you handle internationalization (i18n) in web applications?
Handle internationalization by separating text content from code, using libraries for managing translations, and adapting layouts for different languages and text directions.
96. What is the purpose of a package manager like npm or Yarn?
Package managers automate the process of installing, updating, configuring, and removing third-party dependencies in your projects.
97. How do you implement authentication and authorization in a web application?
Implement authentication and authorization using techniques like JWT (JSON Web Tokens), OAuth, or session-based authentication, often in combination with server-side logic and secure storage of credentials.
98. What are some strategies for optimizing web fonts?
Optimize web fonts by using font subsetting, leveraging font-display CSS property, preloading critical fonts, and using system fonts when possible.
99. How do you handle error boundaries in React applications?
Error boundaries in React are components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed.
100. What are some best practices for writing clean and maintainable code?
Best practices include following consistent coding standards, writing self-documenting code, using meaningful variable and function names, keeping functions small and focused, and regularly refactoring code.
Conclusion
Mastering these top 100 frontend interview questions will significantly boost your confidence and preparedness for technical interviews. Remember, the key to success is not just memorizing answers, but understanding the underlying concepts and being able to apply them in real-world scenarios.
As you continue your journey in frontend development, keep in mind that the field is constantly evolving. Stay curious, keep learning, and practice regularly. Platforms like AlgoCademy can be invaluable resources for honing your skills, particularly in areas like algorithmic thinking and problem-solving, which are crucial for excelling in technical interviews.
Good luck with your interviews, and may your frontend development career be filled with exciting challenges and rewarding experiences!