Tips for Succeeding in a Machine Learning Interview
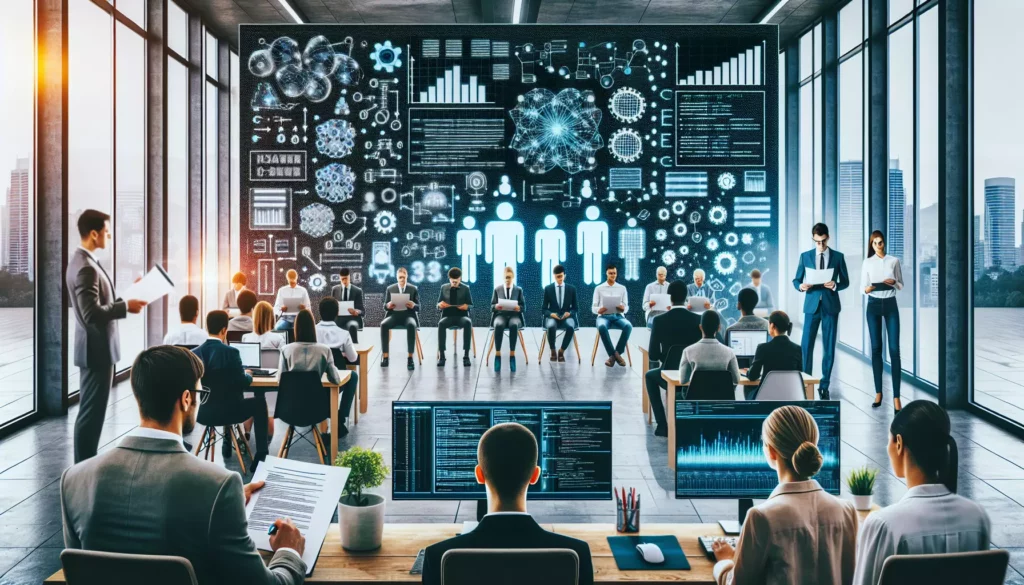
As the field of artificial intelligence continues to expand rapidly, machine learning engineers are in high demand. If you’re preparing for a machine learning interview, whether it’s with a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or another tech giant, you’ll need to be well-prepared. This comprehensive guide will provide you with valuable tips and strategies to help you succeed in your machine learning interview.
1. Master the Fundamentals
Before diving into advanced topics, ensure you have a solid grasp of the fundamentals. This includes:
- Linear algebra
- Probability and statistics
- Calculus
- Algorithms and data structures
- Basic machine learning concepts
Interviewers often start with basic questions to assess your foundation. Be prepared to explain concepts like gradient descent, backpropagation, and the difference between supervised and unsupervised learning.
2. Know Your Machine Learning Algorithms
Be familiar with a wide range of machine learning algorithms, including:
- Linear and logistic regression
- Decision trees and random forests
- Support Vector Machines (SVM)
- K-Means clustering
- Neural Networks and Deep Learning architectures
- Ensemble methods
For each algorithm, be prepared to discuss:
- How it works
- Its strengths and weaknesses
- Appropriate use cases
- How to evaluate its performance
3. Practice Coding Implementations
Many machine learning interviews include coding challenges. Practice implementing machine learning algorithms from scratch using Python or another preferred language. Be comfortable with popular libraries such as:
- NumPy
- Pandas
- Scikit-learn
- TensorFlow or PyTorch
Here’s a simple example of implementing linear regression using NumPy:
import numpy as np
def linear_regression(X, y):
X = np.column_stack((np.ones(X.shape[0]), X))
theta = np.linalg.inv(X.T.dot(X)).dot(X.T).dot(y)
return theta
# Example usage
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
theta = linear_regression(X, y)
print("Theta:", theta)
4. Understand Feature Engineering
Feature engineering is a crucial aspect of machine learning. Be prepared to discuss:
- Feature selection techniques
- Feature scaling and normalization
- Handling missing data
- Creating new features from existing ones
- Dimensionality reduction techniques (e.g., PCA)
Demonstrate your ability to think creatively about feature engineering in different scenarios.
5. Be Familiar with Model Evaluation Techniques
Know how to evaluate and compare different models. This includes understanding:
- Cross-validation techniques
- Metrics for classification (e.g., accuracy, precision, recall, F1-score)
- Metrics for regression (e.g., MSE, RMSE, R-squared)
- ROC curves and AUC
- Confusion matrices
Be prepared to discuss which evaluation metrics are most appropriate for different types of problems.
6. Understand Overfitting and Regularization
Overfitting is a common issue in machine learning. Be ready to explain:
- What overfitting is and how to detect it
- Techniques to prevent overfitting (e.g., cross-validation, regularization)
- Different types of regularization (L1, L2, Elastic Net)
- The bias-variance tradeoff
7. Be Prepared for Open-Ended Problems
Many machine learning interviews include open-ended problems or case studies. Practice approaching these types of questions by:
- Asking clarifying questions
- Breaking down the problem into smaller components
- Discussing your thought process out loud
- Considering multiple approaches and their tradeoffs
For example, you might be asked how you would build a recommendation system for an e-commerce platform. Walk through your approach, discussing data collection, feature engineering, algorithm selection, and evaluation metrics.
8. Stay Up-to-Date with Recent Developments
The field of machine learning is rapidly evolving. Stay current with recent developments by:
- Reading research papers and blog posts
- Following influential researchers and practitioners on social media
- Attending conferences or watching conference talks online
- Participating in Kaggle competitions or other machine learning challenges
Be prepared to discuss recent advancements and their potential impact on the field.
9. Understand the Ethical Implications of Machine Learning
As machine learning becomes more prevalent in society, it’s important to understand its ethical implications. Be prepared to discuss:
- Bias in machine learning models and how to mitigate it
- Privacy concerns and data protection
- Transparency and explainability of models
- The societal impact of AI and machine learning
10. Practice Explaining Complex Concepts Simply
A crucial skill for machine learning engineers is the ability to explain complex concepts to non-technical stakeholders. Practice explaining machine learning concepts in simple terms, using analogies and real-world examples.
11. Prepare for System Design Questions
Many machine learning interviews, especially for senior positions, include system design questions. Be prepared to discuss:
- How to design and implement machine learning systems at scale
- Batch vs. real-time processing
- Data pipelines and ETL processes
- Model deployment and monitoring
- A/B testing and experimentation frameworks
12. Demonstrate Your Project Experience
Be ready to discuss your past projects in detail. For each project, be prepared to explain:
- The problem you were trying to solve
- Your approach and why you chose it
- Challenges you faced and how you overcame them
- The results and impact of your work
- What you learned and what you might do differently next time
13. Understand the Business Context
Machine learning doesn’t exist in a vacuum. Show that you understand how machine learning fits into the broader business context. Be prepared to discuss:
- How machine learning can drive business value
- The tradeoffs between model performance and interpretability
- How to prioritize machine learning projects based on business impact
- The importance of collaboration between data scientists, engineers, and business stakeholders
14. Practice Whiteboard Coding
Many interviews involve coding on a whiteboard or in a shared online editor. Practice writing code without the help of an IDE or autocomplete. Focus on:
- Writing clean, readable code
- Explaining your thought process as you code
- Testing your code and considering edge cases
- Optimizing your solution for time and space complexity
15. Be Prepared for Behavioral Questions
In addition to technical questions, be ready for behavioral interviews. Common questions include:
- “Tell me about a time when you faced a challenging problem and how you solved it.”
- “How do you handle disagreements with team members?”
- “Describe a situation where you had to learn a new technology quickly.”
- “How do you prioritize tasks when working on multiple projects?”
Use the STAR method (Situation, Task, Action, Result) to structure your responses.
16. Understand Deep Learning Architectures
If you’re interviewing for a position that involves deep learning, be prepared to discuss various neural network architectures, such as:
- Convolutional Neural Networks (CNNs) for image processing
- Recurrent Neural Networks (RNNs) and Long Short-Term Memory (LSTM) for sequence data
- Transformer models for natural language processing
- Generative Adversarial Networks (GANs)
Be ready to explain how these architectures work and their appropriate use cases.
17. Know Your Tools and Frameworks
Be familiar with popular machine learning tools and frameworks, including:
- Version control systems (e.g., Git)
- Cloud platforms (e.g., AWS, Google Cloud, Azure)
- Big data processing frameworks (e.g., Apache Spark)
- Experiment tracking tools (e.g., MLflow, Weights & Biases)
- Model serving frameworks (e.g., TensorFlow Serving, TorchServe)
Understand the strengths and weaknesses of different tools and when to use them.
18. Practice Time Management
During the interview, manage your time effectively. If you’re stuck on a problem, don’t spend too much time on it. Ask for hints if needed, and be prepared to move on if necessary. Show that you can work efficiently under pressure.
19. Prepare Thoughtful Questions for Your Interviewers
At the end of the interview, you’ll likely have the opportunity to ask questions. Prepare thoughtful questions that demonstrate your interest in the role and the company. Some examples include:
- “What are some of the biggest challenges facing the machine learning team right now?”
- “How does the company approach the ethical considerations of AI and machine learning?”
- “Can you tell me about a recent project that had a significant impact on the business?”
- “How does the team stay up-to-date with the latest developments in machine learning?”
20. Follow Up After the Interview
After the interview, send a thank-you email to your interviewers. This is an opportunity to:
- Express your appreciation for their time
- Reiterate your interest in the position
- Briefly address any points you feel you could have explained better during the interview
- Provide any additional information they requested
Conclusion
Succeeding in a machine learning interview requires a combination of technical knowledge, problem-solving skills, and effective communication. By following these tips and dedicating time to preparation, you’ll be well-equipped to showcase your skills and land your dream job in machine learning.
Remember that the interview process is also an opportunity for you to evaluate the company and the role. Use the interview to gain insights into the team’s culture, the types of projects you’ll be working on, and the potential for growth and learning.
Lastly, don’t be discouraged if you don’t get an offer after your first interview. Each interview is a learning experience that will help you improve for future opportunities. Reflect on what went well and areas where you can improve, and use that knowledge to refine your preparation for the next interview.
With dedication, practice, and a growth mindset, you’ll be well on your way to succeeding in your machine learning interview and launching an exciting career in this rapidly evolving field.