Tips for Dealing with Time Constraints During Coding Challenges
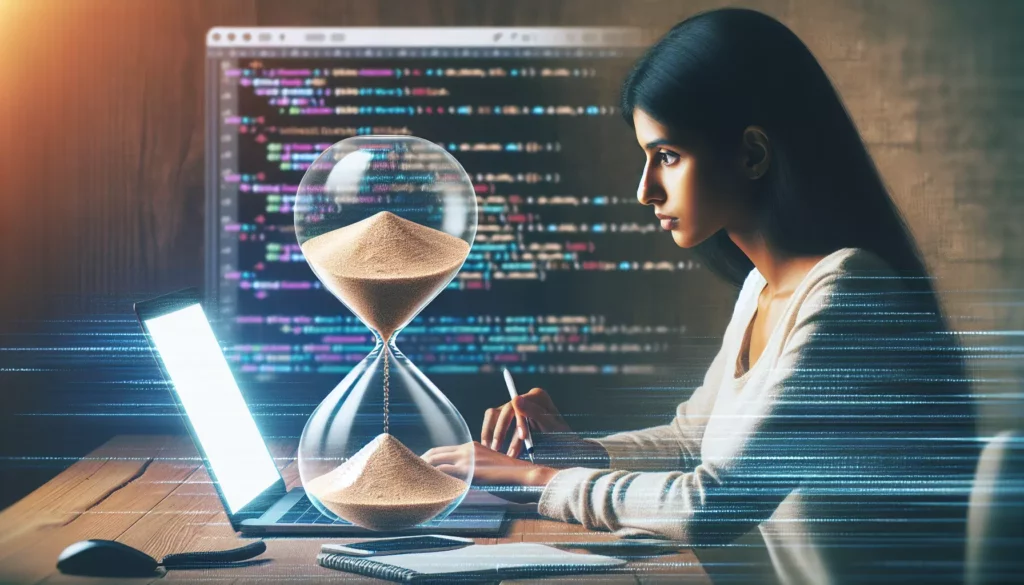
Time constraints are an inevitable part of coding challenges, whether you’re participating in a timed online assessment, a technical interview, or a competitive programming contest. The pressure of a ticking clock can be daunting, but with the right strategies and mindset, you can effectively manage your time and showcase your coding skills. In this comprehensive guide, we’ll explore various tips and techniques to help you navigate time constraints during coding challenges, allowing you to perform at your best when it matters most.
1. Understand the Challenge Requirements
Before diving into coding, take a moment to thoroughly read and understand the challenge requirements. This crucial step can save you valuable time and prevent unnecessary mistakes.
- Read the problem statement carefully: Make sure you grasp all the details, including input formats, output expectations, and any constraints.
- Identify the key elements: Determine what the challenge is asking you to do and what specific outputs are required.
- Note any time or space complexity requirements: Some challenges may have specific performance expectations that you need to meet.
By taking the time to fully comprehend the challenge, you’ll be better equipped to devise an efficient solution and avoid time-consuming misunderstandings later on.
2. Plan Your Approach
Once you understand the challenge, spend a few minutes planning your approach. This initial investment of time can pay off significantly in the long run.
- Outline your algorithm: Sketch out the high-level steps of your solution before writing any code.
- Consider edge cases: Think about potential edge cases or special scenarios that your solution needs to handle.
- Choose appropriate data structures: Select the most suitable data structures for your solution, considering time and space efficiency.
A well-thought-out plan can help you avoid getting stuck or having to backtrack later, saving you precious time during the challenge.
3. Start with a Brute Force Solution
If you’re struggling to come up with an optimal solution immediately, don’t hesitate to start with a brute force approach.
- Implement a working solution first: Focus on getting a functional solution, even if it’s not the most efficient.
- Optimize later: Once you have a working solution, you can refine and optimize it if time permits.
- Ensure correctness: A correct brute force solution is better than an incomplete optimized one.
Remember, having a working solution, even if it’s not optimal, is better than having no solution at all when time runs out.
4. Use Pseudocode to Organize Your Thoughts
Pseudocode can be a powerful tool for organizing your thoughts and planning your implementation without getting bogged down in syntax details.
- Write high-level logic: Use pseudocode to outline the main steps of your algorithm.
- Focus on the problem-solving aspect: Concentrate on the logic and flow of your solution rather than specific programming language syntax.
- Translate to code: Once you’re satisfied with your pseudocode, translate it into actual code.
Here’s an example of how you might use pseudocode to plan a solution for finding the maximum element in an array:
// Pseudocode for finding the maximum element in an array
initialize max_element to first element of array
for each element in array:
if element > max_element:
max_element = element
return max_element
Using pseudocode can help you clarify your thinking and spot potential issues before you start writing actual code, potentially saving you time in the long run.
5. Leverage Built-in Functions and Libraries
Most programming languages come with a rich set of built-in functions and libraries that can significantly speed up your coding process.
- Familiarize yourself with common libraries: Know the standard libraries and their capabilities in your chosen programming language.
- Use built-in sorting and searching algorithms: Instead of implementing these from scratch, use the language’s built-in methods when possible.
- Utilize data structure implementations: Take advantage of pre-implemented data structures like heaps, sets, or maps.
For example, in Python, instead of writing your own sorting algorithm, you can use the built-in sort()
method:
numbers = [5, 2, 8, 1, 9]
sorted_numbers = sorted(numbers) # Returns a new sorted list
numbers.sort() # Sorts the list in-place
By leveraging these built-in tools, you can focus more on solving the core problem rather than reinventing the wheel.
6. Practice Time Management
Effective time management is crucial when dealing with time constraints. Here are some strategies to help you manage your time efficiently:
- Allocate time for each phase: Divide your available time among reading, planning, coding, and testing phases.
- Set checkpoints: Establish time checkpoints to assess your progress and adjust your approach if needed.
- Know when to move on: If you’re stuck on a particular part of the problem, consider moving on and returning to it later if time allows.
For example, in a 60-minute coding challenge, you might allocate your time as follows:
- 5 minutes for reading and understanding the problem
- 10 minutes for planning and pseudocoding
- 30 minutes for implementation
- 10 minutes for testing and debugging
- 5 minutes for final review and optimization (if possible)
Remember, these allocations are flexible and may vary depending on the specific challenge and your personal working style.
7. Write Clean and Readable Code
Even under time pressure, it’s important to write clean and readable code. This practice can save you time in the long run by making your code easier to debug and modify.
- Use meaningful variable and function names: Choose names that clearly indicate the purpose or content of the variable or function.
- Maintain consistent indentation: Proper indentation makes your code structure clear at a glance.
- Add comments for complex logic: While you shouldn’t overdo it, brief comments can help explain tricky parts of your code.
Here’s an example of clean, readable code versus messy code:
// Clean, readable code
function calculateAverage(numbers) {
if (numbers.length === 0) {
return 0;
}
const sum = numbers.reduce((acc, num) => acc + num, 0);
return sum / numbers.length;
}
// Messy, hard-to-read code
function calc(n) {
if(n.length===0)return 0;
let s=0;for(let i=0;i<n.length;i++)s+=n[i];
return s/n.length;
}
Clean code is not only easier for others to understand but also makes it simpler for you to spot and fix errors quickly.
8. Test Your Code Incrementally
Rather than writing all your code at once and then testing it, consider testing incrementally as you go. This approach can help you catch errors early and save time on debugging.
- Test each function or module separately: Verify that individual components work correctly before integrating them.
- Use print statements or debuggers: Add print statements or use debugging tools to check intermediate values and execution flow.
- Start with simple test cases: Begin with basic inputs and gradually move to more complex scenarios.
Here’s an example of how you might test a function incrementally:
function reverseString(str) {
return str.split('').reverse().join('');
}
// Test with a simple case
console.log(reverseString('hello')); // Should output: 'olleh'
// Test with an empty string
console.log(reverseString('')); // Should output: ''
// Test with a palindrome
console.log(reverseString('racecar')); // Should output: 'racecar'
// Test with a string containing spaces
console.log(reverseString('hello world')); // Should output: 'dlrow olleh'
By testing your code in stages, you can identify and fix issues more quickly, potentially saving valuable time during the challenge.
9. Know When to Optimize
While optimization is important, it’s crucial to balance the time spent on optimization with the need to complete the challenge. Here are some guidelines:
- Focus on correctness first: Ensure your solution works correctly before attempting to optimize it.
- Identify performance bottlenecks: If you have time, use profiling tools or your own analysis to find the parts of your code that are slowing things down.
- Consider the trade-offs: Weigh the time it will take to optimize against the potential performance gains.
Remember, a working solution that meets the basic requirements is often better than an incomplete optimization attempt when time is running out.
10. Stay Calm and Focused
Maintaining a calm and focused mindset is crucial when dealing with time constraints. Here are some strategies to help you stay composed:
- Take deep breaths: If you feel stress building up, take a moment to breathe deeply and reset your focus.
- Stay positive: Maintain a positive attitude, even if you encounter difficulties. Remember that challenges are opportunities to learn and improve.
- Focus on what you can control: Instead of worrying about the time limit, concentrate on solving the problem step by step.
Remember, everyone faces challenges with time constraints. Your ability to stay calm and think clearly can be a significant advantage.
11. Practice, Practice, Practice
The most effective way to improve your performance under time constraints is through consistent practice. Here are some ways to incorporate practice into your routine:
- Solve timed coding challenges regularly: Use platforms like AlgoCademy, LeetCode, or HackerRank to practice solving problems under time pressure.
- Simulate real interview conditions: Set up mock interviews with friends or use online platforms that offer interview simulations.
- Review and learn from each attempt: After each practice session, review your performance and identify areas for improvement.
Consistent practice will help you become more comfortable with time constraints and improve your problem-solving speed and efficiency.
12. Learn to Recognize Common Patterns
Many coding challenges follow common patterns or can be solved using well-known algorithms. Familiarizing yourself with these patterns can save you significant time during a challenge.
- Study common algorithms: Learn and practice implementing algorithms like binary search, depth-first search, dynamic programming, etc.
- Recognize problem types: Learn to quickly identify whether a problem is a sorting issue, a graph problem, a dynamic programming challenge, etc.
- Build a mental toolkit: Develop a repertoire of go-to solutions for common problem types.
For example, if you recognize a problem as a classic “two-pointer” scenario, you might quickly implement a solution like this:
function twoSum(numbers, target) {
let left = 0;
let right = numbers.length - 1;
while (left < right) {
const sum = numbers[left] + numbers[right];
if (sum === target) {
return [left + 1, right + 1]; // Adding 1 for 1-based indexing
} else if (sum < target) {
left++;
} else {
right--;
}
}
return []; // No solution found
}
Recognizing and quickly implementing these patterns can significantly speed up your problem-solving process.
13. Use Effective Debugging Techniques
When time is limited, efficient debugging becomes crucial. Here are some techniques to help you identify and fix errors quickly:
- Use strategic print statements: Place print statements at key points in your code to track variable values and execution flow.
- Leverage debugging tools: Familiarize yourself with the debugging features of your IDE or code editor.
- Apply binary search to debugging: If you’re not sure where an error is occurring, use a binary search approach to narrow down the problematic section of code.
Here’s an example of using print statements for debugging:
function processData(data) {
console.log("Input data:", data);
const processedData = data.map(item => item * 2);
console.log("After processing:", processedData);
const sum = processedData.reduce((acc, val) => acc + val, 0);
console.log("Sum:", sum);
return sum;
}
const result = processData([1, 2, 3, 4, 5]);
console.log("Final result:", result);
These print statements can help you quickly identify where a potential issue might be occurring in your code.
14. Know When to Ask for Help
In some coding challenges, especially during interviews, it’s acceptable and sometimes encouraged to ask for clarification or hints. Knowing when and how to ask for help can be valuable:
- Ask for clarification on ambiguous requirements: If any part of the problem statement is unclear, don’t hesitate to ask for more details.
- Request hints if you’re completely stuck: If you’ve been stuck for a while and can’t make progress, it’s often better to ask for a hint than to waste too much time.
- Communicate your thought process: Even if you’re not sure about the solution, explaining your thinking can demonstrate your problem-solving skills.
Remember, asking thoughtful questions can show that you’re engaged with the problem and are thinking critically about the solution.
15. Review and Optimize if Time Permits
If you finish implementing your solution before the time is up, use the remaining time wisely:
- Review your code for correctness: Double-check that your solution meets all the requirements and handles edge cases.
- Look for optimization opportunities: See if there are any parts of your code that could be made more efficient.
- Consider alternative approaches: If you have time, think about whether there might be a completely different (and potentially better) way to solve the problem.
Here’s an example of how you might optimize a simple function:
// Original function
function findDuplicates(arr) {
const duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// Optimized function
function findDuplicatesOptimized(arr) {
const seen = new Set();
const duplicates = new Set();
for (const num of arr) {
if (seen.has(num)) {
duplicates.add(num);
} else {
seen.add(num);
}
}
return Array.from(duplicates);
}
The optimized version uses a Set to improve time complexity from O(n^2) to O(n).
Conclusion
Dealing with time constraints during coding challenges can be stressful, but with the right strategies and mindset, you can navigate these pressures effectively. Remember to understand the problem thoroughly, plan your approach, start with a working solution, and manage your time wisely. Practice regularly to build your skills and confidence, and don’t forget to stay calm and focused during the challenge.
By applying these tips and continuously refining your approach, you’ll be better equipped to handle time-constrained coding challenges, whether in interviews, competitions, or real-world projects. Remember, the goal is not just to solve the problem quickly, but to demonstrate your problem-solving skills, coding ability, and grace under pressure. With persistence and practice, you’ll find yourself becoming more adept at tackling these challenges efficiently and effectively.