Time Series Analysis: Unlocking Insights from Temporal Data
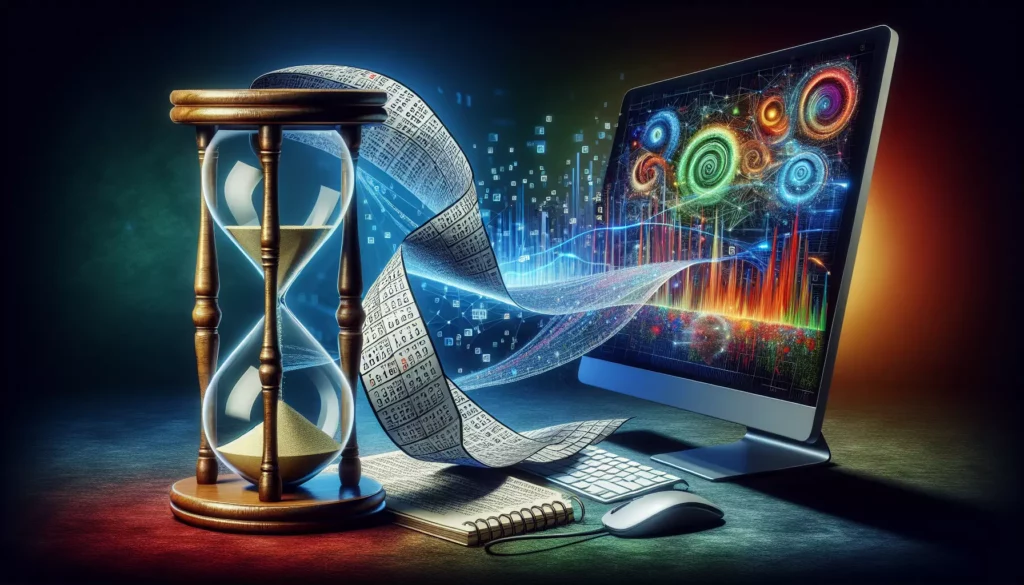
In the ever-evolving landscape of data science and programming, time series analysis stands out as a crucial skill for aspiring developers and data analysts. As part of AlgoCademy’s comprehensive approach to coding education and programming skills development, understanding time series analysis is essential for tackling real-world problems and preparing for technical interviews at major tech companies. In this extensive guide, we’ll dive deep into the world of time series analysis, exploring its fundamentals, applications, and implementation in various programming languages.
What is Time Series Analysis?
Time series analysis is a statistical technique used to analyze and interpret data points collected over time intervals. It involves examining the patterns, trends, and behaviors of data that are chronologically ordered. This method is particularly useful when dealing with datasets where time is a significant factor, such as stock prices, weather patterns, or website traffic.
The primary goals of time series analysis include:
- Identifying patterns and trends in the data
- Forecasting future values based on historical data
- Understanding the underlying factors influencing the data
- Detecting anomalies or outliers in the dataset
Components of Time Series Data
Before diving into the analysis techniques, it’s crucial to understand the four main components that make up time series data:
- Trend: The long-term movement or direction in the data.
- Seasonality: Repeating patterns or cycles at fixed intervals.
- Cyclical Patterns: Fluctuations that don’t have a fixed frequency.
- Irregular Variations: Random, unpredictable fluctuations in the data.
Time Series Analysis Techniques
There are several techniques used in time series analysis, each suited for different types of data and analysis goals. Let’s explore some of the most common methods:
1. Moving Average (MA)
The moving average is a simple yet effective technique for smoothing out short-term fluctuations and highlighting longer-term trends. It calculates the average of a subset of data points over a specific window of time.
Example implementation in Python:
import pandas as pd
import numpy as np
def moving_average(data, window):
return pd.Series(data).rolling(window=window).mean()
# Example usage
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
ma = moving_average(data, window=3)
print(ma)
2. Exponential Smoothing
Exponential smoothing is a forecasting method that gives more weight to recent observations. It’s particularly useful for data with no clear trend or seasonality.
Example implementation in Python:
def exponential_smoothing(data, alpha):
result = [data[0]] # First value is same as input
for n in range(1, len(data)):
result.append(alpha * data[n] + (1 - alpha) * result[n-1])
return result
# Example usage
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
smoothed = exponential_smoothing(data, alpha=0.3)
print(smoothed)
3. Autoregressive Integrated Moving Average (ARIMA)
ARIMA is a popular and more advanced technique that combines autoregression, differencing, and moving average components. It’s particularly useful for data with trends and seasonality.
Example implementation using the statsmodels library in Python:
from statsmodels.tsa.arima.model import ARIMA
import numpy as np
# Generate sample data
np.random.seed(1)
data = np.cumsum(np.random.randn(100))
# Fit ARIMA model
model = ARIMA(data, order=(1,1,1))
results = model.fit()
# Make predictions
forecast = results.forecast(steps=10)
print(forecast)
4. Seasonal Decomposition
Seasonal decomposition breaks down a time series into its constituent components: trend, seasonality, and residuals. This technique is useful for understanding the underlying patterns in the data.
Example implementation using the statsmodels library in Python:
from statsmodels.tsa.seasonal import seasonal_decompose
import pandas as pd
import numpy as np
# Generate sample data with trend and seasonality
date_rng = pd.date_range(start='1/1/2020', end='12/31/2020', freq='D')
data = np.sin(np.arange(len(date_rng))) + np.random.randn(len(date_rng)) * 0.1
ts = pd.Series(data, index=date_rng)
# Perform seasonal decomposition
result = seasonal_decompose(ts, model='additive', period=30)
# Plot the components
result.plot()
Applications of Time Series Analysis
Time series analysis has a wide range of applications across various industries and domains. Some common use cases include:
- Financial Forecasting: Predicting stock prices, currency exchange rates, and economic indicators.
- Weather Prediction: Analyzing historical weather data to forecast future conditions.
- Sales Forecasting: Predicting future sales based on historical data and seasonal patterns.
- Website Traffic Analysis: Understanding patterns in user behavior and predicting future traffic.
- Energy Consumption Prediction: Forecasting energy demand based on historical usage patterns.
- Internet of Things (IoT) Data Analysis: Analyzing sensor data collected over time for predictive maintenance and anomaly detection.
Challenges in Time Series Analysis
While time series analysis is a powerful tool, it comes with its own set of challenges that data scientists and programmers need to be aware of:
- Dealing with Missing Data: Time series often have gaps or missing values that need to be addressed.
- Handling Outliers: Extreme values can significantly impact the analysis and need to be properly managed.
- Seasonal Adjustments: Accounting for seasonal patterns can be complex, especially with multiple overlapping seasonalities.
- Non-Stationarity: Many real-world time series are non-stationary, requiring transformation before analysis.
- Model Selection: Choosing the appropriate model for a given dataset can be challenging and may require experimentation.
Best Practices for Time Series Analysis
To effectively work with time series data and prepare for technical interviews, consider the following best practices:
- Visualize Your Data: Always start by plotting your time series data to get a visual understanding of patterns and potential issues.
- Check for Stationarity: Many time series techniques assume stationarity. Use tests like the Augmented Dickey-Fuller test to check for stationarity and transform the data if necessary.
- Handle Missing Data Appropriately: Choose appropriate methods for handling missing data, such as interpolation or forward-filling, based on the nature of your dataset.
- Consider Multiple Models: Don’t rely on a single model. Try different approaches and compare their performance using appropriate metrics.
- Cross-Validation for Time Series: Use time series-specific cross-validation techniques, such as rolling window validation, to assess model performance.
- Feature Engineering: Create relevant features from your time series data, such as lag variables or rolling statistics, to improve model performance.
- Understand the Domain: Incorporate domain knowledge into your analysis to better interpret results and make informed decisions.
Advanced Topics in Time Series Analysis
As you progress in your understanding of time series analysis, consider exploring these advanced topics:
1. Vector Autoregression (VAR)
VAR is a multivariate time series model that captures the linear dependencies among multiple time series.
2. Long Short-Term Memory (LSTM) Networks
LSTM is a type of recurrent neural network architecture particularly well-suited for sequence prediction problems and complex time series analysis.
3. Prophet
Developed by Facebook, Prophet is a powerful forecasting tool that works well with time series data that have strong seasonal effects and multiple seasons of historical data.
4. Wavelet Analysis
Wavelet analysis is a technique for decomposing a signal into its frequency components, useful for analyzing non-stationary time series data.
Preparing for Technical Interviews
When preparing for technical interviews at major tech companies, especially those focusing on data science or machine learning roles, it’s crucial to have a solid understanding of time series analysis. Here are some tips to help you prepare:
- Practice Implementing Algorithms: Be comfortable implementing basic time series algorithms from scratch, such as moving averages and exponential smoothing.
- Understand the Math: Have a good grasp of the mathematical concepts underlying time series models, including autocorrelation and partial autocorrelation.
- Work on Real-World Datasets: Practice analyzing real-world time series datasets, such as stock prices or weather data, to gain practical experience.
- Be Familiar with Libraries: Know how to use popular time series libraries in Python, such as statsmodels, prophet, and pmdarima.
- Prepare for Conceptual Questions: Be ready to explain concepts like stationarity, seasonality, and the differences between various time series models.
- Solve Time Series Coding Challenges: Practice solving time series-related coding problems on platforms like LeetCode or HackerRank.
Conclusion
Time series analysis is a fundamental skill for anyone working with data that has a temporal component. By mastering the techniques and best practices outlined in this guide, you’ll be well-equipped to tackle real-world problems and excel in technical interviews at major tech companies.
Remember that time series analysis is just one part of the broader landscape of data science and machine learning. Continue to expand your knowledge in areas like statistical analysis, machine learning algorithms, and data visualization to become a well-rounded data scientist or programmer.
As you progress in your journey with AlgoCademy, take advantage of the interactive coding tutorials, AI-powered assistance, and step-by-step guidance to reinforce your understanding of time series analysis and other crucial programming concepts. With practice and dedication, you’ll be well on your way to mastering the art of working with temporal data and unlocking valuable insights from time series datasets.