Time Management During a Coding Interview: How to Avoid Getting Stuck
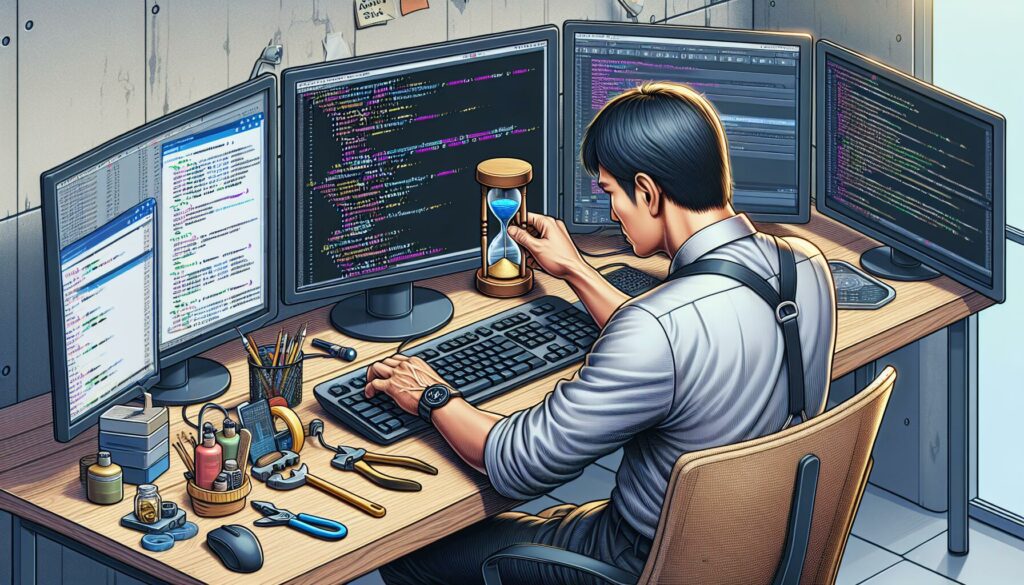
Coding interviews can be nerve-wracking experiences, especially when you’re up against the clock. One of the most crucial skills you can develop for these high-pressure situations is effective time management. In this comprehensive guide, we’ll explore strategies to help you navigate coding interviews efficiently, avoid getting stuck, and make the most of your limited time. Whether you’re a seasoned developer or just starting your journey into the tech world, these tips will help you approach coding interviews with confidence and composure.
Understanding the Importance of Time Management in Coding Interviews
Before we dive into specific strategies, it’s essential to understand why time management is so critical during coding interviews. Here are a few key reasons:
- Limited time frame: Most coding interviews last between 45 minutes to an hour, giving you a finite window to showcase your skills.
- Multiple components: You need to balance problem-solving, coding, and communication within this time frame.
- Pressure simulation: Time constraints simulate real-world pressure, allowing interviewers to assess how you perform under stress.
- Efficiency evaluation: Your ability to manage time effectively is itself a skill that employers value.
With these factors in mind, let’s explore strategies to help you make the most of your interview time.
Setting Time Benchmarks for Each Stage of Problem-Solving
One of the most effective ways to manage your time during a coding interview is to set clear benchmarks for each stage of the problem-solving process. Here’s a suggested breakdown for a 60-minute interview:
- Problem clarification and understanding (5-7 minutes): Use this time to thoroughly understand the problem, ask questions, and clarify any ambiguities.
- Brainstorming and planning (8-10 minutes): Discuss potential approaches, consider edge cases, and outline your solution strategy.
- Coding implementation (25-30 minutes): This is where you’ll spend the bulk of your time, actually writing the code to solve the problem.
- Testing and debugging (10-12 minutes): Run through test cases, identify and fix any bugs in your code.
- Optimization and discussion (5-7 minutes): If time allows, discuss potential optimizations or alternative approaches.
Remember, these are general guidelines and may need to be adjusted based on the specific problem and interview format. The key is to have a mental roadmap of how you’ll allocate your time.
Strategies for Avoiding and Overcoming Stuck Points
Getting stuck during a coding interview can be a major time sink and source of stress. Here are some strategies to help you avoid and overcome these roadblocks:
1. Start with a High-Level Approach
Before diving into coding, outline your approach in pseudocode or plain English. This can help you identify potential issues early and provide a roadmap for your implementation.
// Example pseudocode for a function to find the maximum element in an array
function findMax(array):
if array is empty:
return null
max = first element of array
for each element in array:
if element > max:
max = element
return max
2. Use the “Two-Minute Rule”
If you’re stuck on a particular part of the problem for more than two minutes, it’s time to reassess. You can:
- Ask the interviewer for a hint or clarification
- Explain your thought process and current roadblock
- Move on to another part of the problem and come back later
3. Break Down Complex Problems
If you’re facing a complex problem, break it down into smaller, manageable sub-problems. This approach, known as “divide and conquer,” can help you make progress even when the overall solution isn’t immediately clear.
4. Use Analogies and Visualizations
Sometimes, relating the problem to a real-world analogy or creating a visual representation can help unlock your thinking. Don’t hesitate to use the whiteboard or paper to sketch out your ideas.
5. Implement a Brute Force Solution First
If you’re struggling to find an optimal solution, start with a brute force approach. This accomplishes two things:
- It shows the interviewer that you can at least solve the problem, even if not optimally.
- It provides a starting point from which you can optimize.
// Example of a brute force solution to find pairs that sum to a target
function findPairs(array, target):
pairs = []
for i from 0 to length of array - 1:
for j from i + 1 to length of array:
if array[i] + array[j] == target:
pairs.append((array[i], array[j]))
return pairs
Prioritizing Partial Solutions
In some cases, you may not have time to implement a complete solution. In these situations, it’s crucial to prioritize partial solutions that demonstrate your problem-solving skills and coding ability. Here’s how to approach this:
1. Implement Core Functionality First
Focus on the main logic of the solution, even if you can’t handle all edge cases or optimizations. This shows that you understand the fundamental problem and can code a working solution.
2. Communicate Your Intentions
If you’re implementing a partial solution, clearly communicate to the interviewer:
- What parts of the problem your current solution addresses
- What limitations or edge cases it doesn’t handle
- How you would extend or optimize the solution given more time
3. Use Comments to Outline Unimplemented Parts
If you don’t have time to code certain parts of your solution, use comments to outline your approach. This demonstrates your thought process and planning skills.
function complexAlgorithm(input):
// Step 1: Preprocess input
preprocessedInput = preprocess(input)
// Step 2: Core algorithm implementation
result = coreAlgorithm(preprocessedInput)
// Step 3: Postprocessing (not implemented due to time constraints)
// TODO: Implement postprocessing logic
// - Handle edge cases
// - Optimize for space complexity
return result
function preprocess(input):
// Preprocessing logic here
function coreAlgorithm(data):
// Core algorithm implementation here
Time-Saving Techniques for Coding Interviews
In addition to the strategies mentioned above, here are some specific techniques to help you save time during coding interviews:
1. Master Your Development Environment
Whether you’re coding on a whiteboard, in a shared online editor, or on your local machine, make sure you’re comfortable with the environment. Practice coding without relying heavily on IDE features like auto-completion or syntax highlighting.
2. Use Built-in Functions and Data Structures
Don’t reinvent the wheel. Familiarize yourself with common built-in functions and data structures in your preferred programming language. Using these can save you valuable time during the interview.
// Instead of writing your own sorting function
array.sort()
// Use a set for efficient lookups
seen = set()
for item in array:
if item in seen:
return True
seen.add(item)
return False
3. Write Clean, Readable Code
While it might seem counterintuitive, writing clean, well-organized code from the start can save you time in the long run. It makes debugging easier and demonstrates your coding style to the interviewer.
4. Use Meaningful Variable Names
Choose clear, descriptive variable names. This makes your code more readable and can help you avoid confusion as you work through the problem.
// Instead of
for i in range(len(a)):
for j in range(len(a)):
if a[i] + a[j] == t:
return True
// Use
for index in range(len(numbers)):
for jndex in range(len(numbers)):
if numbers[index] + numbers[jndex] == target:
return True
5. Practice Active Recall
As you code, periodically step back and explain your approach to the interviewer. This serves two purposes:
- It helps you organize your thoughts and catch potential issues.
- It keeps the interviewer engaged and demonstrates your communication skills.
Handling Time Pressure and Stress
Even with excellent time management skills, the pressure of a coding interview can be intense. Here are some strategies to help you stay calm and focused:
1. Practice Under Timed Conditions
Regularly practice solving coding problems under time constraints. This will help you get comfortable with the pressure and improve your time management skills.
2. Use Deep Breathing Techniques
If you feel stress building up during the interview, take a moment to practice deep breathing. A few deep breaths can help calm your nerves and refocus your mind.
3. Maintain a Growth Mindset
Remember that the interview is not just about getting the perfect solution, but also about demonstrating your problem-solving process. Embrace challenges as learning opportunities.
4. Stay Hydrated and Well-Rested
Proper hydration and adequate rest before the interview can significantly impact your mental performance and ability to manage stress.
When to Move On vs. When to Persist
One of the trickiest aspects of time management in coding interviews is knowing when to move on from a problem and when to persist. Here are some guidelines:
When to Move On:
- You’ve been stuck on the same issue for more than 5-7 minutes without making progress.
- You’ve exhausted your ideas and can’t think of a new approach.
- You’re running out of time and haven’t addressed other important parts of the problem.
When to Persist:
- You’re making steady progress, even if it’s slow.
- You have a clear idea of what’s causing the issue and how to resolve it.
- The current problem is critical to the overall solution, and moving on would leave a significant gap.
Remember, it’s always better to have a partially working solution that demonstrates your skills than to get stuck on one part and not show your full capabilities.
Conclusion: Mastering Time Management for Coding Interview Success
Effective time management is a crucial skill for succeeding in coding interviews. By setting clear time benchmarks, employing strategies to avoid getting stuck, prioritizing partial solutions, and utilizing time-saving techniques, you can maximize your performance under pressure.
Remember that perfection is not the goal in most coding interviews. Interviewers are often more interested in your problem-solving approach, coding style, and ability to communicate your thoughts clearly. By managing your time effectively, you give yourself the best chance to showcase these skills.
As you prepare for your next coding interview, incorporate these time management strategies into your practice routine. With consistent application, you’ll find yourself better equipped to handle the time constraints and pressures of real interview situations.
Coding interviews may be challenging, but with the right approach to time management, you can turn them into opportunities to shine. Good luck with your preparations, and may your future interviews be productive, insightful, and successful!