Thinking Out Loud: How Explaining Your Thought Process Can Boost Your Coding Interview Performance
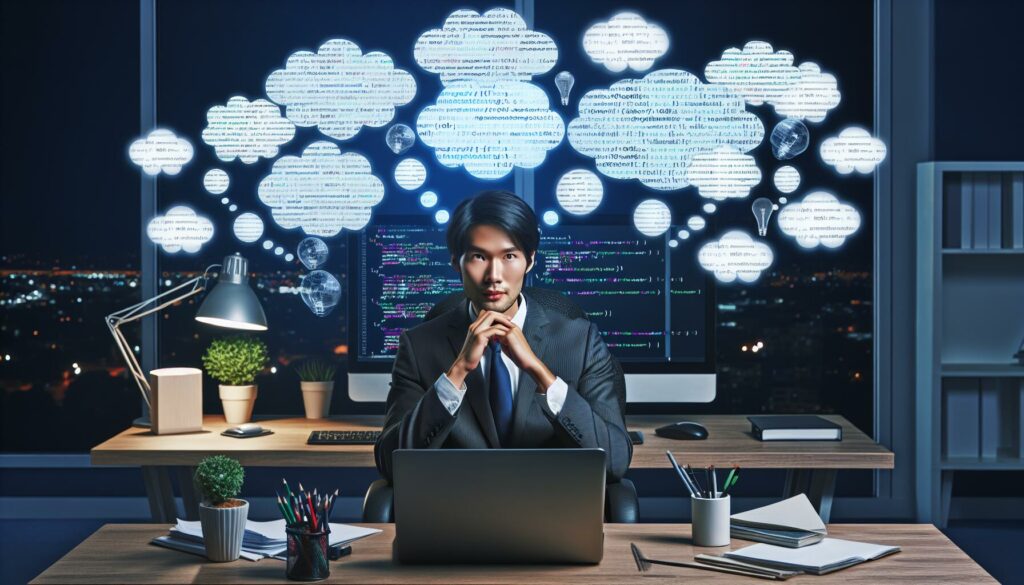
In the competitive world of tech hiring, coding interviews can be a make-or-break moment for aspiring developers. While your coding skills are undoubtedly crucial, there’s another often-overlooked aspect that can significantly impact your performance: verbalizing your thought process. This practice, commonly known as “thinking out loud,” can be a game-changer in demonstrating your problem-solving abilities and showcasing your logical thinking to potential employers. In this comprehensive guide, we’ll explore why articulating your thoughts during a coding interview is so important and provide you with practical tips to master this skill.
The Importance of Verbalizing Your Thought Process
When you’re in a coding interview, it’s easy to fall into the trap of silent concentration. After all, you’re there to solve complex problems, and it might seem natural to retreat into your own thoughts. However, by doing so, you’re missing out on a valuable opportunity to showcase your skills and engage with your interviewer. Here’s why thinking out loud is so crucial:
1. Demonstrates Problem-Solving Skills
By verbalizing your thought process, you’re giving the interviewer a window into your problem-solving approach. This allows them to see not just the end result, but how you arrived at your solution. It demonstrates your ability to break down complex problems, consider different approaches, and make informed decisions.
2. Showcases Logical Thinking
Thinking out loud reveals the logic behind your coding decisions. It shows the interviewer that you’re not just randomly trying solutions, but approaching the problem in a structured, thoughtful manner. This is particularly important in algorithmic problems where the reasoning behind your approach is often as important as the final code.
3. Facilitates Communication
In a real-world development environment, you’ll often need to explain your code and decisions to team members. By practicing verbal explanation during your interview, you’re demonstrating your ability to communicate technical concepts clearly – a highly valued skill in the tech industry.
4. Allows for Guidance and Collaboration
When you verbalize your thoughts, you give the interviewer the opportunity to provide hints or guide you if you’re heading in the wrong direction. This turns the interview into more of a collaborative problem-solving session, which can be less stressful and more productive.
5. Shows Your Personality
Thinking out loud allows your personality to shine through. It gives the interviewer a sense of what it would be like to work with you on a day-to-day basis. Are you methodical? Creative? Persistent? These traits can come across in how you verbalize your problem-solving process.
Tips for Effectively Articulating Your Approach
Now that we understand why verbalizing your thought process is so important, let’s dive into some practical tips on how to do it effectively:
1. Start with the Problem Statement
Begin by restating the problem in your own words. This serves two purposes: it ensures you’ve understood the question correctly, and it gives you a moment to gather your thoughts before diving into the solution.
For example:
Interviewer: "Can you write a function to reverse a string?"
You: "Certainly. So, if I understand correctly, we need to create a function that takes a string as input and returns a new string with all the characters in reverse order. For instance, if the input is 'hello', the output should be 'olleh'. Is that correct?"
2. Outline Your Approach
Before you start coding, explain your high-level approach to solving the problem. This shows that you’re thinking strategically rather than just diving in blindly.
For example:
You: "To solve this, I'm thinking of using a two-pointer approach. We'll have one pointer at the start of the string and another at the end. We'll swap the characters at these pointers and then move the pointers towards the center until they meet. This should efficiently reverse the string in place."
3. Discuss Trade-offs
If there are multiple ways to solve the problem, mention them and explain why you’re choosing a particular approach. This demonstrates your ability to consider different solutions and make informed decisions.
You: "Another approach could be to create a new string and populate it with characters from the original string in reverse order. While this would work, it would require additional space proportional to the length of the string. The two-pointer approach I mentioned earlier is more space-efficient as it reverses the string in place."
4. Think About Edge Cases
Show that you’re considering potential issues by thinking about edge cases out loud. This demonstrates thoroughness and attention to detail.
You: "Before we implement this, let's consider some edge cases. We should handle empty strings and strings with only one character. Also, we need to make sure our function works with strings containing spaces or special characters."
5. Explain Your Code as You Write It
As you’re coding, explain what each line or block of code does. This helps the interviewer follow your thought process and shows that you understand each step of your solution.
You: "First, I'll define our function:
def reverse_string(s):
# Convert string to a list of characters for in-place modification
chars = list(s)
# Initialize two pointers
left, right = 0, len(chars) - 1
# Swap characters until pointers meet in the middle
while left < right:
chars[left], chars[right] = chars[right], chars[left]
left += 1
right -= 1
# Convert list back to string and return
return ''.join(chars)
Now, let me walk you through this code..."
6. Discuss Time and Space Complexity
After implementing your solution, discuss its time and space complexity. This shows that you’re thinking about the efficiency of your code, which is crucial for scalable solutions.
You: "Let's analyze the time and space complexity of this solution. The time complexity is O(n), where n is the length of the string, because we're traversing each character once. The space complexity is O(n) as well, because we're creating a list of characters from the input string. However, if we were allowed to modify the input string directly, we could achieve O(1) space complexity."
7. Propose Improvements or Alternatives
If you can think of any improvements or alternative approaches after implementing your solution, mention them. This shows that you’re continuously thinking about optimization and different perspectives.
You: "While this solution works efficiently, if we were dealing with very large strings, we might want to consider using a more memory-efficient approach. For instance, in Python, we could use string slicing to reverse the string in one line: return s[::-1]. However, this creates a new string, so it's a trade-off between code simplicity and memory usage."
Engaging with the Interviewer
Thinking out loud isn’t just about explaining your thoughts; it’s also about engaging with your interviewer. Here are some tips to make this interaction more productive:
1. Ask Clarifying Questions
Don’t hesitate to ask questions if something about the problem is unclear. This shows that you’re detail-oriented and want to fully understand the requirements before diving in.
You: "Before we start, I just want to clarify - should the function handle Unicode characters, or can we assume it's just ASCII?"
2. Be Open to Hints
If the interviewer offers a hint, take it graciously. Use it as an opportunity to demonstrate how you can incorporate new information into your problem-solving process.
Interviewer: "Have you considered using a stack for this problem?"
You: "That's an interesting suggestion. Let me think about how we could use a stack here..."
3. Think Aloud When Stuck
If you find yourself stuck, don’t go silent. Instead, verbalize your thought process as you work through the problem. This shows perseverance and problem-solving skills even when faced with challenges.
You: "I'm not sure how to proceed here. Let me take a step back and think about what we're trying to achieve. We need to... [continue thinking out loud]"
4. Seek Feedback
Don’t be afraid to ask the interviewer for feedback on your approach. This shows that you’re open to criticism and eager to improve.
You: "Does this approach make sense to you? Is there anything you'd like me to explain further or approach differently?"
Common Pitfalls to Avoid
While thinking out loud is generally beneficial, there are some potential pitfalls to be aware of:
1. Rambling
While it’s good to explain your thoughts, be careful not to ramble or go off on tangents. Try to keep your explanations concise and relevant to the problem at hand.
2. Overexplaining Simple Concepts
If you’re interviewing for an advanced position, you don’t need to explain every basic programming concept. Focus on the parts of your solution that demonstrate your problem-solving skills and deep understanding.
3. Being Too Quiet During Implementation
Don’t fall completely silent when you start coding. Continue to explain what you’re doing, even if it’s just a brief comment for each step.
4. Ignoring the Interviewer
Remember that this is a two-way interaction. Pay attention to the interviewer’s reactions and be responsive to their questions or suggestions.
Practicing Your Verbalization Skills
Like any skill, thinking out loud during problem-solving takes practice. Here are some ways you can improve:
1. Solve Problems Aloud
When you’re practicing coding problems at home, pretend you’re in an interview setting and explain your thought process out loud. This might feel awkward at first, but it will become more natural with practice.
2. Record Yourself
Try recording yourself as you solve a problem and explain your thoughts. Then, listen back to identify areas where you can improve your verbalization.
3. Practice with a Friend
Ask a friend to act as an interviewer while you solve coding problems. This can help you get comfortable explaining your thoughts to another person and handling questions or interruptions.
4. Use Online Platforms
Platforms like AlgoCademy offer interactive coding tutorials and mock interview scenarios where you can practice verbalizing your thoughts in a realistic setting.
Conclusion
Mastering the art of thinking out loud during coding interviews can significantly boost your performance and set you apart from other candidates. It allows you to showcase not just your coding skills, but also your problem-solving approach, logical thinking, and communication abilities.
Remember, the goal is not just to solve the problem, but to demonstrate how you approach and think about complex coding challenges. By clearly articulating your thought process, you give the interviewer insight into your mental toolkit and show them what it would be like to have you on their team.
As you prepare for your next coding interview, make thinking out loud a key part of your practice routine. With time and effort, it will become second nature, allowing you to shine in your interviews and demonstrate your full potential as a developer.
Happy coding, and best of luck in your future interviews!