Thinking in Small Steps: The Power of Micro-Problems in Solving Larger Challenges
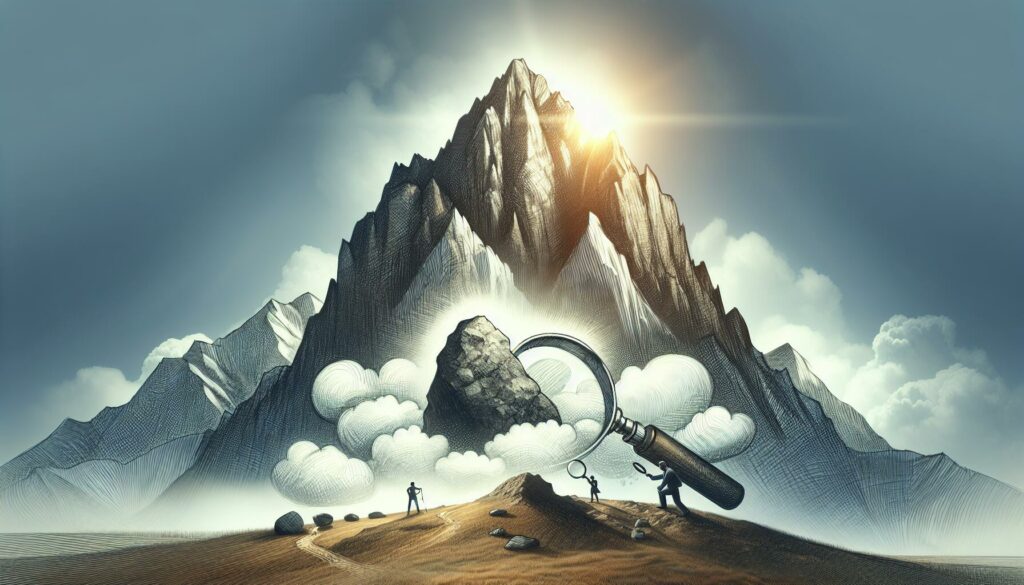
In the world of coding and software development, tackling complex problems is an everyday occurrence. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at major tech companies, the ability to break down large problems into smaller, more manageable pieces is an invaluable skill. This approach, often referred to as “thinking in small steps” or “micro-problem solving,” is not only a powerful technique for coding but also a fundamental principle that can be applied to various aspects of life and learning.
Understanding Micro-Problems
Micro-problems are small, focused challenges that are part of a larger, more complex problem. By breaking down a big task into these smaller components, we can approach seemingly insurmountable obstacles with greater clarity and confidence. This method aligns perfectly with the principles taught in coding education platforms like AlgoCademy, where learners are guided through interactive coding tutorials that emphasize algorithmic thinking and problem-solving skills.
The Benefits of Micro-Problem Solving
- Reduced Complexity: By focusing on smaller parts, the overall problem becomes less overwhelming.
- Increased Clarity: Each micro-problem has a clear objective, making it easier to understand and solve.
- Improved Progress Tracking: Solving micro-problems provides a sense of accomplishment and clear milestones.
- Enhanced Learning: Tackling smaller issues often reveals patterns and insights applicable to larger challenges.
- Better Resource Management: It’s easier to allocate time and effort effectively when working on bite-sized tasks.
Applying Micro-Problem Solving in Coding
In the context of coding and programming, thinking in small steps is particularly valuable. Let’s explore how this approach can be applied to various aspects of software development and problem-solving.
1. Algorithm Design
When designing algorithms, especially for complex problems, starting with a high-level approach and then breaking it down into smaller, implementable steps is crucial. This method is often taught in coding education platforms and is essential for technical interview preparation.
For example, consider the problem of implementing a binary search algorithm:
def binary_search(arr, target):
# Micro-problem 1: Initialize pointers
left, right = 0, len(arr) - 1
# Micro-problem 2: Implement the search loop
while left <= right:
# Micro-problem 3: Calculate mid-point
mid = (left + right) // 2
# Micro-problem 4: Compare and adjust pointers
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
# Micro-problem 5: Handle target not found
return -1
By breaking down the binary search algorithm into these micro-problems, we can focus on implementing and understanding each part individually, making the overall task more manageable.
2. Debugging
When faced with a bug in your code, applying the micro-problem approach can significantly streamline the debugging process. Instead of trying to fix everything at once, focus on isolating the issue and addressing it step by step:
- Identify the specific area where the bug occurs.
- Create a minimal reproduction of the issue.
- Check each variable and function involved in the problematic section.
- Use print statements or debugger to track the flow of execution.
- Make small, incremental changes and test after each modification.
This methodical approach helps in pinpointing the root cause of the bug more efficiently and reduces the chances of introducing new issues while fixing the original problem.
3. Feature Implementation
When tasked with implementing a new feature in a software project, the micro-problem approach can help in breaking down the work into manageable tasks. For instance, if you’re adding a user authentication system to a web application, you might break it down as follows:
- Design the database schema for user information
- Implement user registration functionality
- Create login and logout mechanisms
- Add password hashing and security measures
- Implement session management
- Create user profile pages
- Add password reset functionality
By addressing each of these micro-problems individually, you can make steady progress and ensure that each component is properly implemented before moving on to the next.
Micro-Problems in Learning and Skill Development
The concept of micro-problems isn’t limited to coding tasks; it’s equally valuable in the learning process itself. Platforms like AlgoCademy leverage this approach by breaking down complex programming concepts into smaller, more digestible lessons and interactive coding tutorials.
1. Incremental Learning
When learning a new programming language or framework, it’s often more effective to focus on mastering small concepts one at a time rather than trying to absorb everything at once. For example, when learning Python, you might structure your learning path like this:
- Basic syntax and data types
- Control structures (if statements, loops)
- Functions and modules
- Object-oriented programming concepts
- File I/O and exception handling
- Advanced topics (decorators, generators, etc.)
By tackling each of these areas as a micro-problem, you can build a solid foundation and gradually increase the complexity of your learning material.
2. Project-Based Learning
When working on coding projects to enhance your skills, applying the micro-problem approach can help you make consistent progress. Instead of getting overwhelmed by the entire project, break it down into smaller, achievable tasks. For instance, if you’re building a simple web application:
- Set up the development environment
- Create the basic HTML structure
- Style the page with CSS
- Implement core functionality with JavaScript
- Add interactivity and user input handling
- Implement data storage (local storage or backend integration)
- Optimize performance and responsiveness
- Add additional features and polish
This approach allows you to celebrate small victories along the way and maintain motivation throughout the project.
Micro-Problems in Technical Interview Preparation
For those aiming to land positions at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), mastering the art of micro-problem solving is crucial. Technical interviews often involve complex algorithmic challenges that can be intimidating at first glance. Here’s how to apply the micro-problem approach to your interview preparation:
1. Problem Analysis
When faced with a coding challenge during an interview, resist the urge to start coding immediately. Instead, break down the problem into smaller components:
- Understand the problem statement thoroughly
- Identify the input and expected output
- Consider edge cases and constraints
- Break down the solution into logical steps
- Think about potential data structures and algorithms that could be useful
By approaching the problem this way, you demonstrate to the interviewer your analytical skills and methodical thinking process.
2. Solution Implementation
Once you’ve broken down the problem, implement your solution step by step:
- Start with a basic implementation that handles the core logic
- Add functionality to handle edge cases
- Optimize the solution for better time or space complexity
- Refactor and clean up the code for readability
This approach not only helps you solve the problem more efficiently but also allows the interviewer to follow your thought process and provide guidance if needed.
3. Practice Strategies
When preparing for technical interviews, use the micro-problem approach to structure your practice sessions:
- Focus on one data structure or algorithm type at a time
- Start with easier problems and gradually increase difficulty
- Time yourself solving individual problems to improve speed
- Review and optimize your solutions after solving each problem
- Regularly revisit and reinforce previously learned concepts
This structured approach ensures that you cover all necessary topics and continuously improve your problem-solving skills.
Tools and Techniques for Micro-Problem Solving
To effectively apply the micro-problem approach in your coding and learning journey, consider using the following tools and techniques:
1. Task Management Tools
Utilize task management applications like Trello, Asana, or even a simple to-do list to break down larger projects into smaller, actionable items. This helps in visualizing progress and maintaining focus on individual micro-problems.
2. Pomodoro Technique
The Pomodoro Technique involves working in focused 25-minute intervals followed by short breaks. This method aligns well with the micro-problem approach, allowing you to tackle one small task or concept during each Pomodoro session.
3. Mind Mapping
Use mind mapping tools or even pen and paper to visually break down complex problems into smaller components. This can help in identifying relationships between different parts of the problem and planning your approach.
4. Version Control
When working on coding projects, use version control systems like Git to commit changes after solving each micro-problem. This not only provides a safety net but also allows you to track your progress and revert changes if needed.
5. AI-Powered Assistance
Leverage AI-powered coding assistants and platforms like AlgoCademy that provide step-by-step guidance. These tools can help break down complex problems into more manageable steps and offer targeted assistance when you’re stuck.
Overcoming Challenges in Micro-Problem Solving
While the micro-problem approach is powerful, it’s not without its challenges. Here are some common obstacles you might face and strategies to overcome them:
1. Losing Sight of the Big Picture
Challenge: When focusing on micro-problems, it’s easy to lose sight of the overall goal or solution.
Solution: Regularly step back and review how your current task fits into the larger context. Maintain a high-level outline or diagram of the entire problem to refer to as you work on individual components.
2. Over-Optimization
Challenge: Spending too much time perfecting individual micro-problems can lead to inefficient use of time and resources.
Solution: Set time limits for each micro-problem and focus on creating a working solution first. You can always come back to optimize after the core functionality is implemented.
3. Difficulty in Breaking Down Problems
Challenge: Some problems may seem too interconnected or complex to break down effectively.
Solution: Practice breaking down problems regularly, even for simple tasks. Start with high-level divisions and then further break down each section. Don’t be afraid to revise your breakdown as you gain more insight into the problem.
4. Motivation and Persistence
Challenge: Working on small, incremental steps can sometimes feel tedious or less rewarding than tackling big challenges head-on.
Solution: Celebrate small victories and track your progress visually. Set mini-milestones and reward yourself for completing sets of micro-problems. Remember that consistent small steps lead to significant progress over time.
Conclusion: Embracing the Power of Micro-Problems
Thinking in small steps and embracing the power of micro-problems is a transformative approach to problem-solving, learning, and personal growth. In the realm of coding and software development, this method aligns perfectly with the principles taught by platforms like AlgoCademy, emphasizing algorithmic thinking and practical coding skills.
By breaking down complex challenges into smaller, manageable tasks, we not only make progress more achievable but also enhance our understanding and retention of new concepts. This approach is particularly valuable in preparing for technical interviews at major tech companies, where the ability to analyze and solve complex problems efficiently is crucial.
As you continue your coding journey, whether you’re just starting out or preparing for advanced roles, remember that every large problem is simply a collection of smaller ones. By mastering the art of identifying and solving these micro-problems, you’ll be well-equipped to tackle any challenge that comes your way, both in coding and in life.
Embrace the power of small steps, celebrate incremental progress, and watch as your skills and confidence grow. With patience, persistence, and a methodical approach to problem-solving, there’s no limit to what you can achieve in the world of programming and beyond.