Thinking in Edge Cases: How to Bulletproof Your Coding Solutions in Interviews
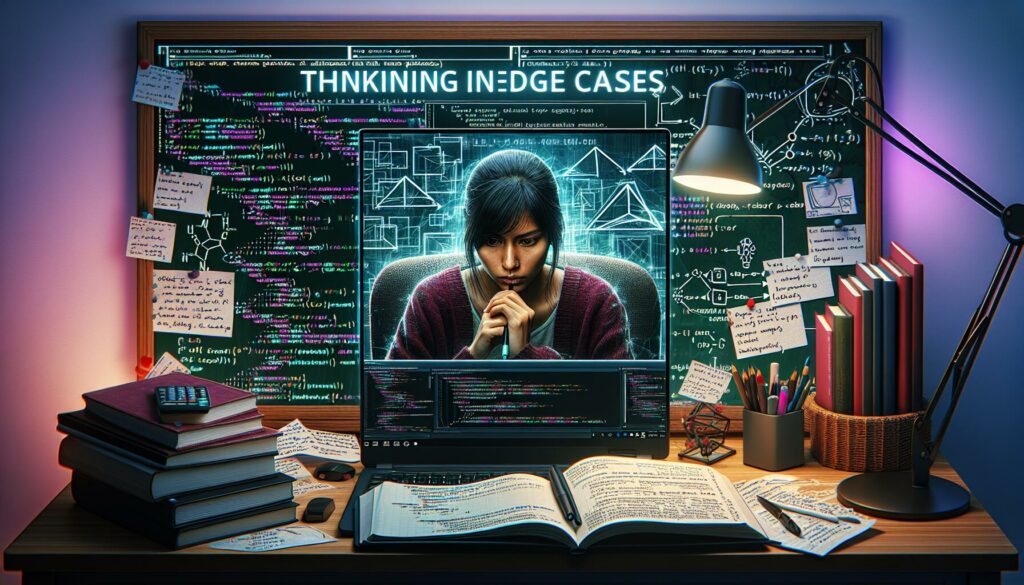
In the world of coding interviews, the difference between a stellar performance and a mediocre one often boils down to a single factor: the ability to think in edge cases. As aspiring developers and seasoned programmers alike navigate the challenging landscape of technical interviews, particularly those for coveted positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering the art of edge case analysis becomes crucial. This comprehensive guide will delve into the importance of considering edge cases, explore common scenarios you’re likely to encounter, and provide strategies to incorporate this critical thinking skill into your problem-solving approach.
Understanding Edge Cases: The Key to Bulletproof Solutions
Edge cases, in the context of programming and algorithm design, refer to input scenarios that push the boundaries of normal operating parameters. These are the extreme or unusual situations that might not occur frequently but can cause your code to behave unexpectedly or fail outright if not properly handled. In coding interviews, your ability to identify and address these edge cases can be the decisive factor in demonstrating your problem-solving skills and attention to detail.
Why Edge Cases Matter in Interviews
Interviewers at top tech companies are not just looking for candidates who can solve the happy path scenarios. They want to see how you approach problem-solving holistically. Here’s why edge cases are so crucial:
- Demonstrates thoroughness: By considering edge cases, you show that you think beyond the obvious and strive for robust solutions.
- Reveals problem-solving depth: Your ability to anticipate and handle edge cases reflects a deeper understanding of the problem domain.
- Mimics real-world scenarios: In production environments, edge cases often lead to critical bugs. Addressing them in interviews shows you’re ready for real-world challenges.
- Differentiates top candidates: While many candidates can solve the main problem, those who excel in handling edge cases stand out.
Common Edge Cases to Consider
As you prepare for coding interviews, familiarizing yourself with common edge cases can significantly improve your problem-solving approach. Let’s explore some of the most frequently encountered edge cases and how to address them:
1. Empty Collections
One of the most overlooked edge cases involves empty collections, such as arrays, strings, or lists. Always ask yourself: “What should happen if the input is empty?”
Example: Finding the Maximum Element in an Array
def find_max(arr):
if not arr: # Check for empty array
return None
max_val = arr[0]
for num in arr[1:]:
if num > max_val:
max_val = num
return max_val
# Test cases
print(find_max([1, 3, 5, 2, 4])) # Output: 5
print(find_max([])) # Output: None
In this example, we first check if the array is empty before proceeding with the main logic. This prevents errors when trying to access elements of an empty array.
2. Large Inputs
Consider how your solution will perform with extremely large inputs. This is especially important for problems involving arrays, strings, or numerical computations.
Example: Calculating Factorial
def factorial(n):
if n < 0:
return None # Handle negative numbers
if n > 20:
return "Result too large to compute" # Handle large inputs
result = 1
for i in range(1, n + 1):
result *= i
return result
# Test cases
print(factorial(5)) # Output: 120
print(factorial(-1)) # Output: None
print(factorial(100)) # Output: "Result too large to compute"
Here, we’ve added checks for negative numbers and very large inputs that could lead to integer overflow or excessive computation time.
3. Negative Numbers
When dealing with numerical problems, always consider how negative numbers might affect your solution. This is especially important in mathematical operations and array manipulations.
Example: Square Root Function
import math
def my_sqrt(x):
if x < 0:
return None # Handle negative numbers
return math.sqrt(x)
# Test cases
print(my_sqrt(16)) # Output: 4.0
print(my_sqrt(-4)) # Output: None
In this square root function, we explicitly handle negative numbers by returning None, as real square roots of negative numbers don’t exist.
4. Boundary Values
Pay attention to the limits of your input range, especially when dealing with specific constraints or data types.
Example: Leap Year Checker
def is_leap_year(year):
if year <= 0:
return False # Handle non-positive years
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
return True
return False
return True
return False
# Test cases
print(is_leap_year(2000)) # Output: True
print(is_leap_year(1900)) # Output: False
print(is_leap_year(2024)) # Output: True
print(is_leap_year(0)) # Output: False
This leap year function handles the edge case of non-positive years, which aren’t typically considered in the Gregorian calendar system.
5. Duplicate or Repeated Elements
In problems involving collections, consider how your solution handles duplicate or repeated elements.
Example: Finding the Mode of an Array
from collections import Counter
def find_mode(arr):
if not arr:
return None # Handle empty array
count = Counter(arr)
max_count = max(count.values())
modes = [k for k, v in count.items() if v == max_count]
return modes[0] if len(modes) == 1 else modes
# Test cases
print(find_mode([1, 2, 3, 2, 4, 2])) # Output: 2
print(find_mode([1, 2, 3, 3, 4, 4])) # Output: [3, 4]
print(find_mode([])) # Output: None
This function not only handles empty arrays but also accounts for the possibility of multiple modes when there are ties for the most frequent element.
Strategies for Incorporating Edge Case Thinking
Now that we’ve explored common edge cases, let’s discuss strategies to incorporate this thinking into your problem-solving approach during coding interviews:
1. Start with Edge Cases
Before diving into the main solution, take a moment to consider potential edge cases. This proactive approach can save you time and demonstrate your thoroughness to the interviewer.
Example: Reversing a String
def reverse_string(s):
# Edge cases
if not s:
return "" # Handle empty string
if len(s) == 1:
return s # Handle single character string
# Main logic
return s[::-1]
# Test cases
print(reverse_string("hello")) # Output: "olleh"
print(reverse_string("")) # Output: ""
print(reverse_string("a")) # Output: "a"
By addressing edge cases upfront, we ensure our solution works for all inputs, including empty strings and single-character strings.
2. Use Input Validation
Implement input validation at the beginning of your function to catch and handle unexpected inputs early.
Example: Calculating Average of an Array
def calculate_average(arr):
# Input validation
if not isinstance(arr, list):
raise TypeError("Input must be a list")
if not arr:
return None # Handle empty list
if not all(isinstance(x, (int, float)) for x in arr):
raise ValueError("All elements must be numbers")
# Main logic
return sum(arr) / len(arr)
# Test cases
print(calculate_average([1, 2, 3, 4, 5])) # Output: 3.0
print(calculate_average([])) # Output: None
try:
print(calculate_average("not a list"))
except TypeError as e:
print(f"Error: {e}")
try:
print(calculate_average([1, 2, "3"]))
except ValueError as e:
print(f"Error: {e}")
This function includes comprehensive input validation, ensuring that the input is a list of numbers and handling the empty list case.
3. Leverage Test-Driven Development (TDD)
While you may not have time to write full unit tests during an interview, thinking in terms of test cases can help you identify edge cases.
Example: Binary Search Implementation
def binary_search(arr, target):
if not arr:
return -1 # Handle empty array
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Test cases (thinking in TDD)
assert binary_search([], 5) == -1, "Empty array test failed"
assert binary_search([1, 3, 5, 7, 9], 5) == 2, "Normal case test failed"
assert binary_search([1, 3, 5, 7, 9], 6) == -1, "Target not in array test failed"
assert binary_search([1, 1, 1, 1, 1], 1) == 2, "All elements same test failed"
print("All tests passed!")
By thinking through various test cases, including edge cases, we ensure our binary search implementation is robust and handles all scenarios correctly.
4. Use Visualization and Examples
Sometimes, visualizing the problem or working through small examples can help you identify potential edge cases.
Example: Merging Two Sorted Arrays
def merge_sorted_arrays(arr1, arr2):
# Edge cases
if not arr1:
return arr2
if not arr2:
return arr1
# Main logic
result = []
i, j = 0, 0
while i < len(arr1) and j < len(arr2):
if arr1[i] <= arr2[j]:
result.append(arr1[i])
i += 1
else:
result.append(arr2[j])
j += 1
# Handle remaining elements
result.extend(arr1[i:])
result.extend(arr2[j:])
return result
# Test cases
print(merge_sorted_arrays([1, 3, 5], [2, 4, 6])) # Output: [1, 2, 3, 4, 5, 6]
print(merge_sorted_arrays([], [1, 2, 3])) # Output: [1, 2, 3]
print(merge_sorted_arrays([1], [])) # Output: [1]
print(merge_sorted_arrays([1, 2, 3], [4, 5, 6])) # Output: [1, 2, 3, 4, 5, 6]
By visualizing different scenarios, we’ve accounted for cases where one or both arrays might be empty, as well as when one array is completely smaller than the other.
The Impact of Edge Case Thinking on Problem-Solving
Incorporating edge case thinking into your problem-solving approach can have a profound impact on your performance in coding interviews and your overall development as a programmer. Here’s how:
1. Improved First-Try Success Rate
By considering edge cases early in your problem-solving process, you’re more likely to develop a comprehensive solution on your first attempt. This not only saves time during the interview but also demonstrates your ability to think critically and holistically about the problem at hand.
2. Enhanced Code Quality
Solutions that account for edge cases are inherently more robust and reliable. They’re less likely to break under unexpected inputs or conditions, which is a crucial quality in production-grade code.
3. Better Communication Skills
Discussing edge cases with your interviewer showcases your ability to think through problems thoroughly and communicate your thought process effectively. This is a valuable skill that interviewers at top tech companies highly appreciate.
4. Deeper Problem Understanding
Analyzing potential edge cases forces you to consider the problem from multiple angles, leading to a deeper understanding of the underlying concepts and potential pitfalls.
Practical Tips for Mastering Edge Case Thinking
To further develop your edge case thinking skills, consider the following practical tips:
1. Practice with Diverse Problems
Expose yourself to a wide range of coding problems, each with its own set of potential edge cases. Platforms like AlgoCademy offer a variety of challenges that can help you hone this skill.
2. Review and Analyze Failed Attempts
When you encounter a problem where your solution fails due to an edge case, take the time to understand why and how you could have anticipated it. This reflective practice will improve your ability to spot similar issues in the future.
3. Leverage AI-Powered Assistance
Tools like AlgoCademy’s AI-powered assistance can provide hints and guidance on potential edge cases you might have overlooked. Use these resources to supplement your learning and gradually improve your independent edge case identification skills.
4. Collaborate and Discuss
Engage in coding discussions with peers or in online forums. Explaining your approach and listening to others can often reveal edge cases you hadn’t considered.
5. Create Edge Case Checklists
Develop a personal checklist of common edge cases to review before considering your solution complete. This might include items like empty inputs, negative numbers, extremely large values, and type mismatches.
Conclusion: Elevating Your Coding Interview Performance
Mastering the art of thinking in edge cases is a powerful way to elevate your performance in coding interviews, especially when aiming for positions at major tech companies. By incorporating this skill into your problem-solving toolkit, you demonstrate not just your coding ability, but your capacity for thorough, production-ready thinking.
Remember, the goal in a coding interview isn’t just to solve the problem—it’s to showcase your comprehensive approach to software development. By consistently considering and addressing edge cases, you position yourself as a thoughtful, detail-oriented programmer capable of creating robust, reliable solutions.
As you continue your journey in programming and interview preparation, make edge case analysis an integral part of your problem-solving process. With practice and persistence, this skill will become second nature, significantly boosting your confidence and success rate in technical interviews.
Platforms like AlgoCademy offer the perfect environment to hone these skills, providing a wealth of problems, AI-assisted learning, and a supportive community to help you on your path to becoming a top-tier developer. Embrace the challenge of edge case thinking, and watch as your coding solutions become more bulletproof and your interview performances more impressive.