The Unexpected Similarities Between Coding and Writing Poetry
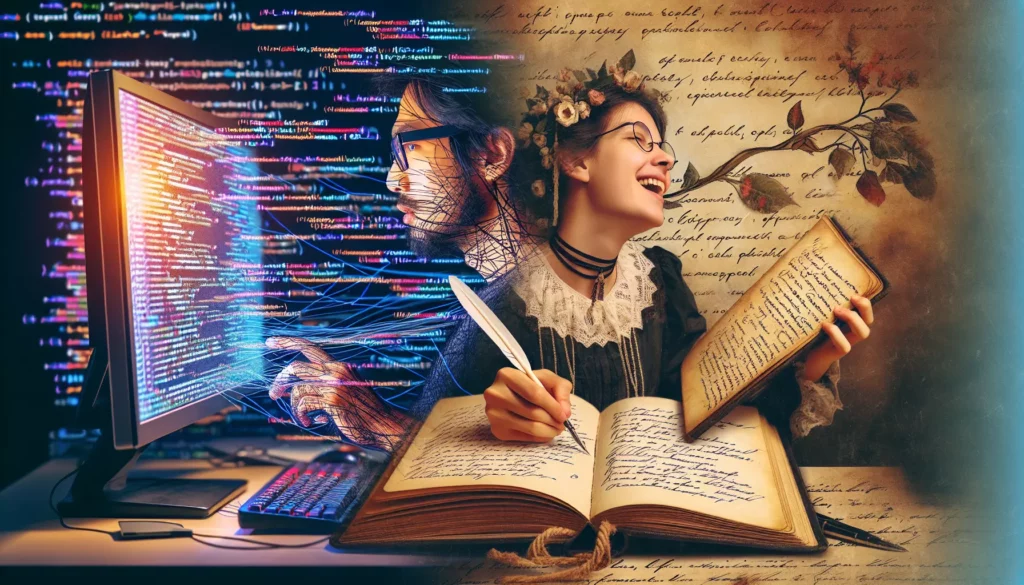
At first glance, coding and poetry might seem like two completely different worlds. One is associated with logic, algorithms, and the cold precision of machines, while the other is often seen as a realm of emotion, creativity, and human expression. However, as we delve deeper into these seemingly disparate disciplines, we uncover a surprising number of similarities that challenge our preconceptions and reveal the artistry inherent in both.
In this exploration, we’ll examine the unexpected parallels between coding and poetry, shedding light on how these two forms of expression share more common ground than meets the eye. By understanding these connections, we can gain valuable insights into both fields and perhaps even improve our skills in each.
1. The Power of Conciseness
One of the most striking similarities between coding and poetry is the emphasis on conciseness. In both disciplines, practitioners strive to express complex ideas in the most efficient and elegant way possible.
Coding: The Art of Brevity
In programming, writing clean, concise code is a hallmark of expertise. Developers aim to solve problems with the least amount of code necessary, avoiding redundancy and maximizing readability. This principle is often referred to as the DRY (Don’t Repeat Yourself) principle or writing “elegant” code.
Consider this example of a function to calculate the factorial of a number:
function factorial(n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
This concise recursive function accomplishes its task in just one line of code, demonstrating the power of brevity in programming.
Poetry: Economy of Words
Similarly, poets strive to convey profound emotions and complex ideas using carefully chosen words and compact structures. Haiku, a Japanese form of poetry, is an excellent example of this principle in action. With just 17 syllables, a haiku can paint a vivid picture or evoke deep emotions.
Consider this haiku by Matsuo Basho:
An old silent pond…
A frog jumps into the pond,
splash! Silence again.
In just a few words, Basho captures a moment in time, creating a powerful image and evoking a sense of tranquility and sudden disruption.
2. Structure and Syntax
Both coding and poetry rely heavily on structure and syntax to convey meaning and ensure proper execution or interpretation.
Coding: The Language of Computers
In programming, syntax refers to the set of rules that define how code must be written to be understood by the computer. Each programming language has its own syntax, and adhering to these rules is crucial for the code to function correctly.
For example, in Python, indentation is a critical part of the syntax that defines code blocks:
def greet(name):
if name:
print(f"Hello, {name}!")
else:
print("Hello, World!")
The indentation in this code snippet is not just for readability; it’s an essential part of the language’s syntax that determines the structure and execution of the program.
Poetry: The Language of Emotion
Poetry also has its own set of rules and structures, though they can be more flexible and open to interpretation. Poetic forms like sonnets, villanelles, or even free verse have specific structural requirements that poets work within to create their art.
Consider the structure of a Shakespearean sonnet:
- 14 lines
- Iambic pentameter
- Rhyme scheme: ABAB CDCD EFEF GG
Just as a programmer must work within the constraints of a programming language’s syntax, a poet crafting a sonnet must adhere to these structural rules while still expressing their ideas and emotions effectively.
3. Abstraction and Metaphor
Both coding and poetry make extensive use of abstraction and metaphor to represent complex ideas in more manageable or relatable forms.
Coding: Abstraction for Simplification
In programming, abstraction is a fundamental concept that allows developers to hide complex implementation details behind simpler interfaces. This makes code more manageable and easier to understand at a higher level.
For example, consider the concept of a “stack” in programming:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
This class abstracts the concept of a stack, providing a simple interface (push, pop, is_empty) that hides the underlying implementation details.
Poetry: Metaphor for Understanding
Poets use metaphors and other figurative language to represent abstract concepts or emotions in more concrete, relatable terms. This allows readers to connect with complex ideas on a more intuitive level.
Consider these lines from Emily Dickinson’s poem “Hope is the thing with feathers”:
“Hope” is the thing with feathers –
That perches in the soul –
And sings the tune without the words –
And never stops – at all –
Here, Dickinson uses the metaphor of a bird to represent the abstract concept of hope, making it more tangible and easier to grasp.
4. Problem-Solving and Creativity
Both coding and poetry involve creative problem-solving, albeit in different contexts.
Coding: Algorithmic Thinking
Programmers constantly face challenges that require creative solutions. They must break down complex problems into smaller, manageable parts and devise algorithms to solve them efficiently.
For instance, consider the classic problem of finding the longest palindromic substring in a given string. A creative solution might involve dynamic programming:
def longest_palindrome(s):
n = len(s)
dp = [[False] * n for _ in range(n)]
start, max_length = 0, 1
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
# Check for substrings of length 2
for i in range(n - 1):
if s[i] == s[i + 1]:
dp[i][i + 1] = True
start = i
max_length = 2
# Check for lengths greater than 2
for length in range(3, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j] and dp[i + 1][j - 1]:
dp[i][j] = True
if length > max_length:
start = i
max_length = length
return s[start:start + max_length]
This solution demonstrates the creativity involved in developing an efficient algorithm to solve a complex problem.
Poetry: Emotional Problem-Solving
Poets, on the other hand, often grapple with emotional or philosophical problems, seeking to express complex feelings or ideas in a way that resonates with readers. They must find creative ways to use language, rhythm, and imagery to convey their message effectively.
Consider how Robert Frost addresses the concept of choice and regret in his poem “The Road Not Taken”:
Two roads diverged in a yellow wood,
And sorry I could not travel both
And be one traveler, long I stood
And looked down one as far as I could
To where it bent in the undergrowth;
Frost creatively uses the metaphor of diverging roads to explore the complex emotions surrounding life-altering decisions.
5. Iteration and Refinement
Both coding and poetry involve processes of iteration and refinement to achieve the desired outcome.
Coding: Debugging and Optimization
In software development, the initial version of a program is rarely perfect. Developers spend considerable time debugging, refactoring, and optimizing their code to improve its functionality, efficiency, and readability.
For example, consider this initial implementation of a function to find the nth Fibonacci number:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
While this recursive solution works, it’s inefficient for large values of n. Through iteration and refinement, a developer might optimize it to use dynamic programming:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i - 1] + fib[i - 2]
return fib[n]
This refined version is much more efficient, demonstrating the importance of iteration in coding.
Poetry: Revision and Polishing
Similarly, poets often go through multiple drafts and revisions of their work, refining their language, adjusting rhythm and meter, and honing their imagery to create the most impactful version of their poem.
Consider these two versions of a stanza from W.H. Auden’s poem “September 1, 1939”. The first is from an early draft:
Uncertain and afraid
As the clever hopes expire
Of a low dishonest decade:
And the final, published version:
Uncertain and afraid
As the clever hopes expire
Of a low dishonest decade:Waves of anger and fear
Circulate over the bright
And darkened lands of the earth,
The addition of the second stanza adds depth and imagery to the poem, illustrating how revision and refinement can enhance the power of poetry.
6. The Importance of Flow
Both coding and poetry place a high value on flow, though in slightly different contexts.
Coding: Logical Flow and Readability
In programming, “flow” refers to the logical progression of a program and how easily other developers can follow and understand the code. Good code should have a natural flow that makes its purpose and functionality clear.
Consider this example of a function that calculates the average of a list of numbers:
def calculate_average(numbers):
if not numbers:
return 0
total = sum(numbers)
count = len(numbers)
average = total / count
return average
This function has a clear, logical flow: it checks for an empty list, calculates the sum and count, computes the average, and returns the result. The flow of the code makes it easy to understand and maintain.
Poetry: Rhythm and Cadence
In poetry, flow refers to the rhythm and cadence of the words, how they sound when read aloud, and how they carry the reader through the poem. A well-crafted poem has a natural flow that enhances its impact and meaning.
Consider the flow in these lines from Edgar Allan Poe’s “The Raven”:
Once upon a midnight dreary, while I pondered, weak and weary,
Over many a quaint and curious volume of forgotten lore—
While I nodded, nearly napping, suddenly there came a tapping,
As of some one gently rapping, rapping at my chamber door.
The rhythm and repetition in these lines create a hypnotic flow that draws the reader into the poem’s eerie atmosphere.
7. The Joy of Creation
Perhaps the most fundamental similarity between coding and poetry is the joy of creation that practitioners in both fields experience.
Coding: Building Something from Nothing
Programmers often describe the satisfaction of seeing their code come to life, solving real-world problems or creating new experiences. There’s a unique thrill in transforming abstract ideas into functional software.
For example, imagine the satisfaction of creating a simple game like Tic-Tac-Toe:
def print_board(board):
for row in board:
print(" | ".join(row))
print("---------")
def check_winner(board):
# Check rows, columns, and diagonals
for i in range(3):
if board[i][0] == board[i][1] == board[i][2] != " ":
return board[i][0]
if board[0][i] == board[1][i] == board[2][i] != " ":
return board[0][i]
if board[0][0] == board[1][1] == board[2][2] != " ":
return board[0][0]
if board[0][2] == board[1][1] == board[2][0] != " ":
return board[0][2]
return None
def play_game():
board = [[" " for _ in range(3)] for _ in range(3)]
current_player = "X"
while True:
print_board(board)
row = int(input(f"Player {current_player}, enter row (0-2): "))
col = int(input(f"Player {current_player}, enter column (0-2): "))
if board[row][col] == " ":
board[row][col] = current_player
winner = check_winner(board)
if winner:
print_board(board)
print(f"Player {winner} wins!")
break
if all(board[i][j] != " " for i in range(3) for j in range(3)):
print_board(board)
print("It's a tie!")
break
current_player = "O" if current_player == "X" else "X"
else:
print("That spot is already taken. Try again.")
play_game()
Creating this game from scratch and seeing it work can be incredibly rewarding for a programmer.
Poetry: Expressing the Inexpressible
Poets often speak of the satisfaction of capturing a fleeting emotion or complex idea in words, of creating something beautiful and meaningful from the raw material of language.
Consider the sense of accomplishment a poet might feel after crafting a powerful piece like Maya Angelou’s “Still I Rise”:
You may write me down in history
With your bitter, twisted lies,
You may trod me in the very dirt
But still, like dust, I’ll rise.
The ability to express resilience and defiance so eloquently is a source of profound satisfaction for many poets.
Conclusion: The Intersection of Art and Science
As we’ve explored, coding and poetry share many unexpected similarities. Both require precision, creativity, and a deep understanding of structure and flow. Both involve problem-solving, iteration, and the joy of creation. And both have the power to profoundly impact how we understand and interact with the world around us.
Recognizing these parallels can enrich our appreciation of both disciplines. For coders, embracing the poetic aspects of programming can lead to more elegant, expressive code. For poets, understanding the logical structures underlying language can provide new avenues for creativity.
Moreover, this intersection of art and science reminds us that these two realms are not as separate as we often believe. In both coding and poetry, we find a beautiful synthesis of logic and creativity, of structure and expression. By appreciating this synthesis, we can become better programmers, better poets, and perhaps even better thinkers overall.
As we continue to explore the frontiers of technology and art, let’s remember that innovation often happens at these unexpected intersections. The next great breakthrough in AI might be inspired by a poem, or the next literary masterpiece might be structured like an algorithm. By keeping our minds open to these connections, we open ourselves to new possibilities in both fields and beyond.
So whether you’re debugging a complex program or crafting a sonnet, remember that you’re part of a rich tradition that spans both art and science. Embrace the precision of a well-structured algorithm and the beauty of a perfectly chosen word. In doing so, you’ll not only improve your skills in your chosen field but also gain a deeper appreciation for the incredible complexity and beauty of human expression in all its forms.