The Unexpected Similarities Between Coding and Stand-Up Comedy
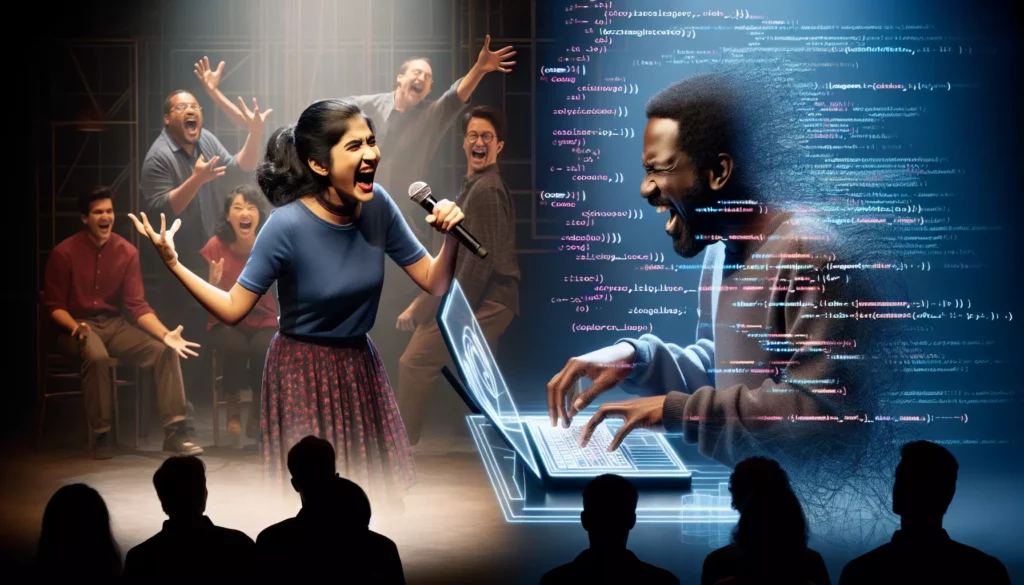
At first glance, coding and stand-up comedy might seem like two completely different worlds. One involves sitting in front of a computer, meticulously crafting lines of code, while the other involves standing on a stage, delivering punchlines to a live audience. However, as we delve deeper into these seemingly disparate fields, we discover a surprising number of similarities that highlight the creative and analytical nature of both pursuits.
1. The Art of Problem-Solving
Both coding and stand-up comedy require a keen ability to solve problems creatively. In coding, developers face challenges ranging from debugging complex algorithms to optimizing performance. Similarly, comedians must navigate the intricacies of crafting jokes that resonate with their audience, often addressing sensitive topics with tact and humor.
For instance, consider the following scenario:
// Coding Problem: Find the maximum sum subarray
function maxSubarraySum(arr) {
let maxSum = arr[0];
let currentSum = arr[0];
for (let i = 1; i < arr.length; i++) {
currentSum = Math.max(arr[i], currentSum + arr[i]);
maxSum = Math.max(maxSum, currentSum);
}
return maxSum;
}
// Comedy Problem: Crafting a joke about a sensitive topic
"I've been trying to lose weight, but it's hard when you're always hungry.
It's like my stomach is running its own weight gain algorithm, and I can't figure out how to debug it!"
In both cases, the individual must analyze the problem, consider various approaches, and implement a solution that achieves the desired outcome – whether it’s finding the maximum sum subarray or eliciting laughter from the audience.
2. Iterative Improvement
Both coders and comedians understand the importance of iteration and continuous improvement. In software development, this concept is often referred to as “refactoring” – the process of restructuring existing code without changing its external behavior. Similarly, comedians constantly refine their material, adjusting timing, word choice, and delivery based on audience reactions.
Consider the following examples:
// Initial code
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price;
}
return total;
}
// Refactored code
function calculateTotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
// Initial joke
"I tried to explain recursion to my friend, but he just didn't get it. So I tried to explain recursion to my friend, but he just didn't get it. So I tried to explain recursion to my friend..."
// Refined joke
"I tried to explain recursion to my friend, but he just didn't get it. So I told him to read this joke again."
In both cases, the initial version is functional but can be improved. Through iteration and refinement, both the code and the joke become more concise and effective.
3. Pattern Recognition
Recognizing patterns is crucial in both coding and comedy. Programmers often identify recurring structures in code, leading to the development of design patterns and reusable components. Similarly, comedians observe patterns in human behavior, societal trends, and everyday situations to craft relatable and humorous material.
For example:
// Coding pattern: Singleton
class DatabaseConnection {
private static instance: DatabaseConnection;
private constructor() {}
public static getInstance(): DatabaseConnection {
if (!DatabaseConnection.instance) {
DatabaseConnection.instance = new DatabaseConnection();
}
return DatabaseConnection.instance;
}
}
// Comedy pattern: Rule of Three
"I have a great memory. I remember my first day of school, my first kiss, and... uh... something else important."
In the coding example, the Singleton pattern ensures only one instance of the DatabaseConnection class is created. In the comedy example, the “Rule of Three” pattern sets up an expectation with two items and then subverts it with the third, creating a humorous effect.
4. Attention to Detail
Both coding and stand-up comedy require meticulous attention to detail. In programming, a single misplaced character can lead to syntax errors or logical bugs. Similarly, in comedy, the precise wording and timing of a joke can make the difference between a laugh and silence.
Consider these examples:
// Coding: Off-by-one error
for (let i = 0; i <= array.length; i++) {
console.log(array[i]); // Potential undefined access on last iteration
}
// Correct version
for (let i = 0; i < array.length; i++) {
console.log(array[i]);
}
// Comedy: Precise wording
"I used to be indecisive. Now I'm not so sure."
// Less effective version
"I was indecisive before. Now I don't know."
In both cases, small changes can significantly impact the outcome. Attention to detail ensures that both code and jokes perform as intended.
5. Audience Engagement
While it might not be immediately obvious, both coders and comedians must consider their audience. Programmers write code that will be read and maintained by other developers, requiring clear structure and documentation. Comedians, of course, tailor their material to resonate with their specific audience.
For instance:
// Code with poor readability
function x(a,b){return a.filter(i=>i>b).map(i=>i*2).reduce((s,i)=>s+i,0)}
// Code with better readability and documentation
/**
* Filters an array for values greater than a threshold,
* doubles each value, and returns the sum.
* @param {number[]} numbers - The input array of numbers
* @param {number} threshold - The minimum value to include
* @returns {number} The sum of doubled values above the threshold
*/
function sumDoubledValuesAboveThreshold(numbers, threshold) {
return numbers
.filter(num => num > threshold)
.map(num => num * 2)
.reduce((sum, num) => sum + num, 0);
}
// Comedy for a general audience
"Why don't scientists trust atoms? Because they make up everything!"
// Comedy for a tech-savvy audience
"Why do programmers prefer dark mode? Because light attracts bugs!"
In both cases, understanding and catering to the audience leads to more effective communication and engagement.
6. Handling Failure
Both coding and comedy involve a high risk of failure and require resilience in the face of setbacks. Programmers often encounter bugs, errors, and unexpected behavior in their code. Similarly, comedians face the possibility of jokes falling flat or audiences not responding as expected.
Consider these scenarios:
// Coding: Handling errors
try {
riskyOperation();
} catch (error) {
console.error("An error occurred:", error.message);
// Implement recovery logic or graceful degradation
}
// Comedy: Recovering from a joke that didn't land
"Well, that joke didn't work. I guess my comedy algorithm needs debugging. Anyone here know how to fix a null pointer exception in my humor module?"
In both cases, the ability to handle failure gracefully and learn from mistakes is crucial for long-term success.
7. Continuous Learning
The fields of technology and comedy are constantly evolving, requiring practitioners in both areas to engage in continuous learning. Programmers must stay updated with new languages, frameworks, and best practices. Comedians need to keep up with current events, cultural trends, and evolving societal norms to remain relevant and engaging.
For example:
// Learning a new programming paradigm: Functional Programming
// Traditional imperative approach
let numbers = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
// Functional approach
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((acc, curr) => acc + curr, 0);
// Adapting comedy to current events
"Remember when our biggest worry was Y2K? Now we're dealing with AI that can write better jokes than me. I'm not sure if I should be impressed or start looking for a new career in prompt engineering."
Both examples demonstrate the need to adapt and learn new approaches to stay effective in their respective fields.
8. Modularity and Reusability
In both coding and comedy, there’s a strong emphasis on creating modular, reusable components. Programmers strive to write functions and classes that can be easily reused across different projects. Similarly, comedians often develop versatile jokes or comedic techniques that can be adapted to various situations.
Consider these examples:
// Coding: Reusable utility function
function capitalizeString(str) {
return str.charAt(0).toUpperCase() + str.slice(1).toLowerCase();
}
console.log(capitalizeString("heLLo")); // Output: "Hello"
console.log(capitalizeString("wORLD")); // Output: "World"
// Comedy: Adaptable joke template
"Why don't {profession} use {tool/concept}? Because they prefer {humorous alternative}!"
// Examples:
// "Why don't programmers use Windows? Because they prefer to stay in their Linux comfort zone!"
// "Why don't chefs use recipe books? Because they prefer to wing it with a dash of panic!"
In both cases, the modular approach allows for efficient reuse and adaptation to different contexts.
9. Timing and Precision
Timing is critical in both coding and comedy. In programming, efficient algorithms and optimized code can significantly impact performance. In comedy, the precise timing of a punchline can make or break a joke.
For instance:
// Coding: Optimizing a sorting algorithm
// Inefficient bubble sort (O(n^2))
function bubbleSort(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = 0; j < arr.length - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
// More efficient quicksort (O(n log n) on average)
function quickSort(arr) {
if (arr.length <= 1) return arr;
const pivot = arr[arr.length - 1];
const left = [];
const right = [];
for (let i = 0; i < arr.length - 1; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
// Comedy: Timing in delivery
"I told my wife she was drawing her eyebrows too high. She looked surprised."
// The timing of the punchline ("She looked surprised") is crucial for the joke's effectiveness.
In both examples, precise timing and execution are essential for optimal results.
10. Abstraction and Simplification
Both coders and comedians often deal with complex ideas or systems, requiring the ability to abstract and simplify concepts for better understanding or impact. Programmers use abstraction to hide complex implementation details behind simple interfaces, while comedians distill complex societal issues into relatable, humorous observations.
For example:
// Coding: Abstraction in Object-Oriented Programming
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
start() {
// Complex engine startup logic abstracted away
console.log(`${this.make} ${this.model} is starting.`);
}
drive() {
// Complex driving mechanics abstracted away
console.log(`${this.make} ${this.model} is driving.`);
}
}
let myCar = new Car("Toyota", "Corolla");
myCar.start(); // Output: "Toyota Corolla is starting."
myCar.drive(); // Output: "Toyota Corolla is driving."
// Comedy: Simplifying complex ideas
"Trying to explain blockchain to my grandma is like trying to teach a cat calculus.
In the end, we both just stare at each other, confused and slightly annoyed."
In both cases, complex ideas are simplified and presented in a more accessible format.
Conclusion
As we’ve explored, the worlds of coding and stand-up comedy share numerous unexpected similarities. Both require creativity, problem-solving skills, attention to detail, and the ability to connect with an audience. They involve iterative improvement, pattern recognition, and the capacity to handle failure gracefully. Moreover, both fields demand continuous learning and adaptation to remain effective in rapidly changing environments.
These parallels highlight the interdisciplinary nature of creative and analytical thinking. Whether you’re debugging a complex algorithm or crafting the perfect punchline, the underlying cognitive processes often draw from the same well of skills and approaches.
For aspiring programmers and comedians alike, recognizing these similarities can provide valuable insights and potentially new approaches to honing their craft. It also underscores the importance of diverse thinking in problem-solving, regardless of the field.
So, the next time you’re stuck on a coding problem, why not try approaching it with the mindset of a comedian? Or if you’re working on your stand-up routine, consider applying some algorithmic thinking to your joke structure. You might just find that the intersection of these seemingly disparate fields leads to unexpected breakthroughs and innovations.
In the end, whether you’re aiming for clean code or clean comedy, remember: it’s all about connecting with your audience, solving problems creatively, and not being afraid to debug your approach when things don’t go as planned. And who knows? With the rise of AI, we might soon see a stand-up comedian bot telling jokes about its own code – now that would be a real test of both programming and comedic skills!