The Unexpected Parallels Between Coding and Ancient Philosophy
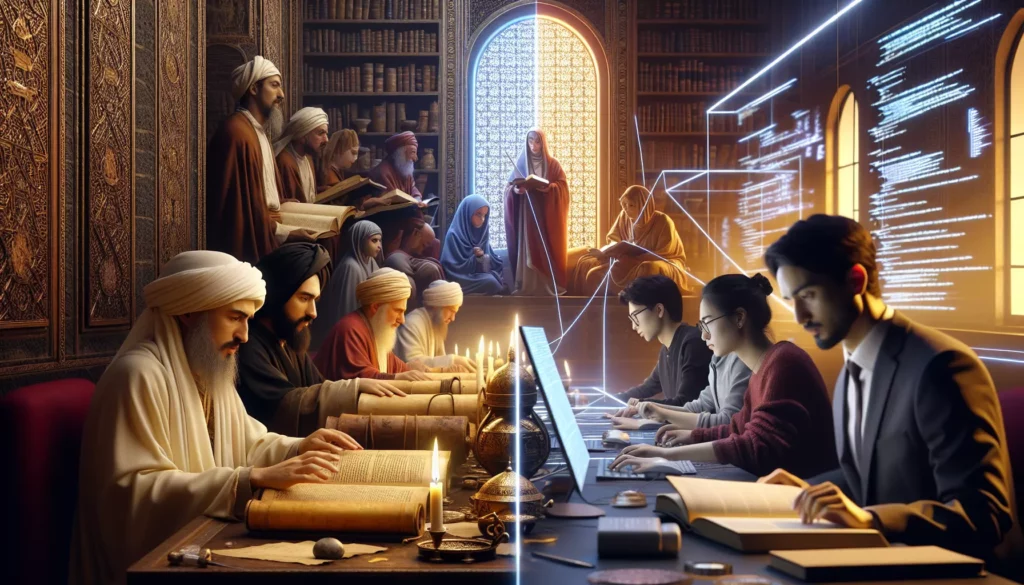
In the fast-paced world of technology, where lines of code shape our digital landscape, it might seem counterintuitive to draw parallels with ancient philosophy. Yet, as we delve deeper into the realms of programming and the timeless wisdom of philosophers, we uncover striking similarities that bridge millennia. This exploration not only enriches our understanding of coding but also provides a fresh perspective on the age-old teachings that continue to influence our thinking today.
The Foundations of Logical Thinking
At the core of both coding and ancient philosophy lies the art of logical thinking. The great philosophers of antiquity, such as Aristotle, laid the groundwork for formal logic, which is essentially the bedrock of computer science and programming.
Aristotelian Logic and Boolean Algebra
Aristotle’s syllogisms, a form of deductive reasoning, find their modern counterpart in Boolean algebra, which is fundamental to computer programming. Just as Aristotle used premises to reach logical conclusions, programmers use Boolean operators to create conditions and make decisions in their code.
For instance, consider this simple syllogism:
All men are mortal.
Socrates is a man.
Therefore, Socrates is mortal.
Now, let’s look at how this translates to a simple Boolean condition in code:
if (is_man && is_mortal) {
console.log("The subject is mortal.");
}
This simple example demonstrates how ancient logical structures continue to inform modern programming practices.
The Socratic Method and Debugging
The Socratic method, named after the philosopher Socrates, involves asking probing questions to stimulate critical thinking and illuminate ideas. This method bears a striking resemblance to the debugging process in programming.
When debugging, programmers ask a series of questions about their code:
- What is the expected output?
- What is the actual output?
- Where could the discrepancy be occurring?
- What assumptions am I making about the code’s behavior?
This systematic questioning approach mirrors the Socratic method, leading developers to uncover truths about their code and resolve issues effectively.
The Quest for Elegance and Simplicity
Both ancient philosophers and modern programmers share a common goal: to distill complex ideas into their simplest, most elegant forms.
Occam’s Razor in Philosophy and Code
While not strictly from ancient philosophy, the principle of Occam’s Razor, attributed to the 14th-century philosopher William of Ockham, states that the simplest explanation is often the correct one. This principle finds its echo in the world of coding through the DRY (Don’t Repeat Yourself) principle and the pursuit of clean, maintainable code.
Consider this verbose code snippet:
function calculateArea(shape) {
if (shape === "square") {
return side * side;
} else if (shape === "circle") {
return Math.PI * radius * radius;
} else if (shape === "triangle") {
return (base * height) / 2;
}
}
Now, compare it with a more elegant solution using object-oriented principles:
const shapes = {
square: (side) => side * side,
circle: (radius) => Math.PI * radius * radius,
triangle: (base, height) => (base * height) / 2
};
function calculateArea(shape, ...params) {
return shapes[shape](...params);
}
The second approach embodies the philosophical principle of simplicity, making the code more maintainable and easier to extend.
Zen and the Art of Coding
Zen philosophy, with its emphasis on simplicity and mindfulness, has much to offer the world of programming. The concept of “less is more” in Zen finds its programming counterpart in minimalist code that achieves maximum effect with minimal complexity.
Consider the Zen-like elegance of Python’s list comprehensions:
squares = [x**2 for x in range(10)]
This single line of code creates a list of squares, embodying the Zen principle of achieving more with less.
The Ethical Dimensions
Ancient philosophy placed great emphasis on ethics and the pursuit of virtue. Similarly, in the world of coding, ethical considerations play a crucial role, especially as technology’s impact on society grows.
The Hippocratic Oath for Programmers
Just as ancient Greek physicians swore by the Hippocratic Oath to uphold ethical standards in their practice, modern programmers are increasingly adopting ethical codes of conduct. The ACM Code of Ethics and Professional Conduct, for instance, outlines principles that echo ancient philosophical virtues:
- Contribute to society and human well-being
- Avoid harm
- Be honest and trustworthy
- Respect privacy
- Honor confidentiality
These principles resonate with the ethical teachings of philosophers like Aristotle, who emphasized the importance of virtue in human conduct.
The Trolley Problem in AI Ethics
The ancient philosophical thought experiment known as the Trolley Problem has found new relevance in the age of artificial intelligence and autonomous vehicles. This ethical dilemma, which asks whether it’s morally permissible to divert a trolley to kill one person instead of five, now informs discussions about how self-driving cars should be programmed to respond in unavoidable accident scenarios.
Programmers working on AI systems must grapple with these ethical questions, much like the philosophers of old, to create systems that align with human values and ethical principles.
The Nature of Reality and Virtual Worlds
Ancient philosophers often pondered the nature of reality and our perception of it. In the digital age, programmers are literally creating virtual realities, bringing philosophical questions about the nature of existence into sharp focus.
Plato’s Cave and Virtual Reality
Plato’s Allegory of the Cave, which explores the relationship between perception and reality, finds a modern parallel in virtual reality (VR) technology. Just as the prisoners in Plato’s cave mistake shadows for reality, users of VR technology immerse themselves in artificial worlds that can feel compellingly real.
Programmers creating VR experiences must grapple with questions that echo Plato’s concerns:
- How do we create convincing illusions of reality?
- What is the relationship between the virtual and the real?
- How does immersion in virtual worlds affect our perception of reality?
These questions blur the lines between philosophy and technology, challenging programmers to think deeply about the nature of the experiences they create.
The Matrix and Simulation Theory
The philosophical idea that our reality might be a simulation, popularized by the movie “The Matrix” but with roots in ancient skepticism, has gained traction in the age of advanced computing. Some programmers and tech visionaries, like Elon Musk, have seriously considered the possibility that we might be living in a computer simulation.
This idea raises profound questions for programmers:
- If we can create increasingly realistic simulations, what are the limits of simulated reality?
- How can we determine if our own reality is “real” or simulated?
- What ethical considerations arise if we create sentient beings within our simulations?
These questions demonstrate how the work of programmers intersects with age-old philosophical inquiries about the nature of reality and consciousness.
The Pursuit of Knowledge and Learning
Both ancient philosophy and modern programming share a fundamental commitment to the pursuit of knowledge and continuous learning.
Socrates and the Growth Mindset
Socrates famously said, “I know that I know nothing,” embodying a humble approach to knowledge that emphasized constant questioning and learning. This attitude aligns perfectly with the growth mindset essential for success in programming.
In the rapidly evolving world of technology, programmers must constantly update their skills and knowledge. The Socratic approach of questioning assumptions and seeking deeper understanding is invaluable in this context.
For instance, when learning a new programming language or framework, a developer might approach it with these Socratic questions:
- What are the fundamental principles of this language?
- How does it differ from languages I already know?
- What problems is it particularly well-suited to solve?
- What are its limitations and potential pitfalls?
This questioning approach leads to a deeper, more nuanced understanding of the technology.
Aristotle’s Empiricism and Data-Driven Development
Aristotle’s emphasis on empirical observation and data collection as the basis for knowledge finds a modern counterpart in data-driven development practices. Just as Aristotle advocated for careful observation of the natural world, modern programmers rely on data to inform their decisions and improve their code.
Consider the practice of A/B testing in web development. This approach, which involves comparing two versions of a webpage to see which performs better, is essentially an application of Aristotelian empiricism to the digital realm.
A simple A/B test might look like this:
if (user.group === "A") {
showButtonVersion1();
} else {
showButtonVersion2();
}
trackUserBehavior();
By collecting and analyzing data on user behavior, developers can make informed decisions about which version of the interface is more effective, echoing Aristotle’s emphasis on observation-based knowledge.
The Concept of Time and Asynchronous Programming
Ancient philosophers grappled with the concept of time, a theme that finds surprising resonance in modern asynchronous programming paradigms.
Heraclitus and the Flow of Time
The pre-Socratic philosopher Heraclitus famously said, “No man ever steps in the same river twice, for it’s not the same river and he’s not the same man.” This concept of constant flux and the flow of time is mirrored in the asynchronous nature of many modern programming paradigms.
In asynchronous programming, operations don’t block the execution of the program but instead allow it to continue flowing, much like Heraclitus’s river. Consider this JavaScript example:
console.log("Start");
setTimeout(() => {
console.log("This happens later");
}, 1000);
console.log("End");
The program doesn’t wait for the setTimeout function to complete before moving on, embodying the Heraclitean concept of flow and change.
Zeno’s Paradoxes and Recursive Programming
Zeno’s paradoxes, which explore the nature of infinity and divisibility, find an unexpected parallel in recursive programming techniques. Just as Zeno’s Dichotomy paradox suggests an infinite series of steps before reaching a destination, recursive functions often involve breaking a problem down into smaller, self-similar parts.
Consider this recursive function to calculate factorial:
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
}
This function, like Zeno’s paradoxes, involves a series of steps that approach a final result, illustrating how ancient philosophical concepts can inform modern programming paradigms.
The Harmony of Opposites in Programming Paradigms
The ancient Greek philosopher Heraclitus proposed the unity of opposites, suggesting that seemingly contradictory forces can coexist in harmony. This concept finds a surprising parallel in the world of programming paradigms.
Object-Oriented vs. Functional Programming
The debate between object-oriented programming (OOP) and functional programming (FP) often presents them as opposing paradigms. However, modern programming practices increasingly recognize the value of combining these approaches, echoing Heraclitus’s unity of opposites.
Consider this hybrid approach in JavaScript:
// Object-Oriented approach
class Person {
constructor(name) {
this.name = name;
}
greet() {
return `Hello, ${this.name}!`;
}
}
// Functional approach
const upperCaseGreeting = (greetingFn) => (...args) =>
greetingFn(...args).toUpperCase();
// Combining OOP and FP
const person = new Person("Alice");
const loudGreeting = upperCaseGreeting(person.greet.bind(person));
console.log(loudGreeting()); // Outputs: HELLO, ALICE!
This example demonstrates how seemingly opposite programming paradigms can work together harmoniously, much like Heraclitus’s unity of opposites.
The Philosophical Foundations of Computer Science
Many fundamental concepts in computer science have their roots in philosophical ideas that date back to ancient times.
Leibniz’s Binary System and Modern Computing
While not strictly ancient, the philosopher and mathematician Gottfried Wilhelm Leibniz’s work on the binary system in the 17th century laid the groundwork for modern computing. Leibniz’s binary system, inspired by the I Ching, an ancient Chinese text, is the foundation of all digital technology today.
Every line of code we write ultimately translates to binary, a system that bridges ancient philosophical concepts with cutting-edge technology:
// The number 42 in binary
const binaryRepresentation = 0b101010;
console.log(binaryRepresentation); // Outputs: 42
Aristotle’s Categories and Data Structures
Aristotle’s work on categorization and classification finds its modern counterpart in the organization of data structures in programming. Just as Aristotle sought to categorize all things in the natural world, programmers use data structures to organize and categorize information in the digital realm.
Consider this simple implementation of a binary tree, a data structure that embodies Aristotelian categorization:
class TreeNode {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
function insertNode(root, value) {
if (root === null) {
return new TreeNode(value);
}
if (value < root.value) {
root.left = insertNode(root.left, value);
} else {
root.right = insertNode(root.right, value);
}
return root;
}
This binary tree structure allows for the efficient categorization and retrieval of data, echoing Aristotle’s philosophical work on categories.
Conclusion: The Enduring Relevance of Ancient Wisdom
As we’ve explored throughout this article, the connections between ancient philosophy and modern programming are numerous and profound. From logical thinking and ethical considerations to the nature of reality and the pursuit of knowledge, the wisdom of ancient philosophers continues to resonate in the world of coding.
These parallels offer several valuable insights:
- Timeless Principles: The enduring relevance of ancient philosophical concepts in modern programming underscores the timeless nature of certain principles of logic, ethics, and knowledge.
- Interdisciplinary Thinking: Recognizing these connections encourages programmers to think beyond the confines of their technical discipline, fostering creativity and innovation.
- Ethical Foundations: As technology’s impact on society grows, the ethical frameworks developed by ancient philosophers provide valuable guidance for navigating complex moral questions in the digital age.
- Holistic Understanding: Exploring these parallels can lead to a more holistic understanding of programming, situating it within the broader context of human intellectual history.
- Continuous Learning: The philosophical emphasis on questioning and continuous learning aligns perfectly with the ever-evolving nature of the tech industry.
For aspiring programmers, particularly those engaging with platforms like AlgoCademy, understanding these philosophical connections can enrich their learning experience. It can provide a broader context for the skills they’re developing, helping them see coding not just as a technical skill, but as part of a long tradition of human inquiry and problem-solving.
As we continue to push the boundaries of what’s possible with technology, the wisdom of ancient philosophers serves as a valuable guide. It reminds us to question our assumptions, seek elegance in our solutions, consider the ethical implications of our work, and never stop learning. In doing so, we honor the legacy of those ancient thinkers while blazing new trails in the digital frontier.
The unexpected parallels between coding and ancient philosophy reveal that, despite the millennia that separate us from the great thinkers of antiquity, the fundamental questions and challenges we grapple with remain remarkably similar. As programmers, embracing this philosophical heritage can deepen our understanding of our craft and inspire us to approach our work with greater wisdom and perspective.