The Unexpected Connection Between Coding and Interpretive Dance
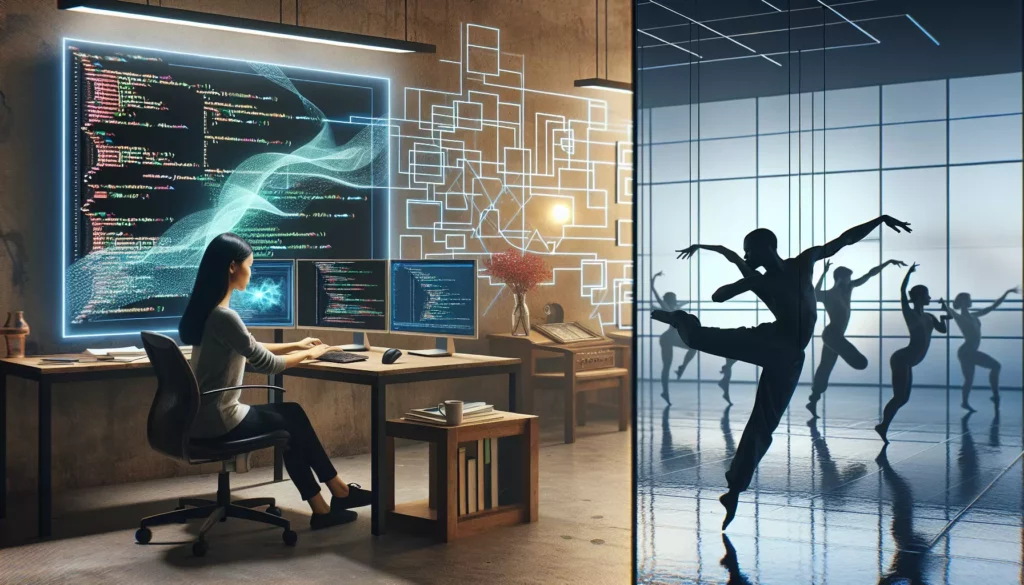
At first glance, coding and interpretive dance might seem like two completely unrelated disciplines. One involves sitting at a computer, meticulously typing lines of code, while the other is a form of artistic expression through movement. However, upon closer examination, these two fields share surprising similarities and interconnections that can enhance both the programmer’s and the dancer’s craft. In this article, we’ll explore the unexpected parallels between coding and interpretive dance, and how understanding these connections can benefit aspiring coders and dancers alike.
The Art of Expression
Both coding and interpretive dance are forms of expression, albeit in different mediums. Programmers use programming languages to express logic, algorithms, and functionality, while dancers use their bodies to convey emotions, stories, and abstract concepts. In both cases, the goal is to communicate ideas effectively.
Consider the following code snippet that calculates the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
for i in range(10):
print(fibonacci(i))
This code expresses the mathematical concept of the Fibonacci sequence through a recursive function. Similarly, a dancer might use a series of fluid, interconnected movements to represent the same concept, with each pose flowing into the next, mirroring the recursive nature of the sequence.
Structure and Syntax
Both coding and interpretive dance rely heavily on structure and syntax. In programming, the syntax of a language determines how code should be written and organized. Similarly, dance has its own set of rules and structures that govern movement and composition.
For example, in Python, proper indentation is crucial for defining code blocks:
def greet(name):
if name:
print(f"Hello, {name}!")
else:
print("Hello, stranger!")
greet("Alice")
greet("")
In dance, the structure might be defined by the music’s rhythm, the sequence of movements, or the spatial arrangement of dancers on stage. Both disciplines require practitioners to adhere to these structures while also finding creative ways to work within and sometimes challenge these constraints.
Problem-Solving and Creativity
Coding and interpretive dance both involve a significant amount of problem-solving and creativity. Programmers often face challenges when developing software and must find innovative solutions to complex problems. Similarly, dancers must solve problems related to choreography, spatial awareness, and physical limitations.
In coding, a programmer might need to optimize an algorithm for better performance:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
# Optimized version using memoization
def memoized_factorial(n, memo={}):
if n in memo:
return memo[n]
if n == 0:
return 1
result = n * memoized_factorial(n-1, memo)
memo[n] = result
return result
In dance, a choreographer might need to find a creative way to represent a complex emotion or concept through movement, perhaps by combining traditional techniques in a novel way or inventing entirely new movements.
Iteration and Refinement
Both coding and interpretive dance involve iterative processes of creation and refinement. Programmers rarely write perfect code on the first try; instead, they write, test, debug, and refine their code multiple times. Similarly, dancers and choreographers go through numerous rehearsals and revisions before a performance is ready.
In coding, this might involve refactoring code for better readability or efficiency:
# Initial version
def is_prime(n):
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
# Refactored version
def is_prime_optimized(n):
if n < 2:
return False
if n == 2:
return True
if n % 2 == 0:
return False
for i in range(3, int(n**0.5) + 1, 2):
if n % i == 0:
return False
return True
In dance, refinement might involve adjusting the timing of movements, refining gestures, or reworking entire sections of choreography to better convey the intended message or emotion.
Abstraction and Modularity
Both coding and interpretive dance make use of abstraction and modularity. In programming, complex systems are broken down into smaller, manageable modules or functions. Similarly, in dance, complex choreographies are often composed of smaller, reusable movement phrases or sequences.
In coding, this might look like:
def validate_input(data):
# Input validation logic
def process_data(data):
# Data processing logic
def generate_output(processed_data):
# Output generation logic
def main():
raw_data = get_user_input()
if validate_input(raw_data):
processed_data = process_data(raw_data)
output = generate_output(processed_data)
display_result(output)
else:
display_error("Invalid input")
In dance, a choreographer might create a series of movement phrases that can be combined and recombined in different ways to create a full performance, much like how functions in programming can be called and combined to create a complete program.
Collaboration and Teamwork
Both coding and interpretive dance often involve collaboration and teamwork. In software development, programmers frequently work in teams, collaborating on different aspects of a project. Similarly, dance performances often involve multiple dancers working together, as well as collaboration between dancers, choreographers, and other artists.
In coding, collaboration might involve using version control systems like Git:
git clone https://github.com/example/project.git
git checkout -b new-feature
# Make changes to the code
git add .
git commit -m "Implement new feature"
git push origin new-feature
# Create a pull request for team review
In dance, collaboration might involve dancers learning and practicing choreography together, providing feedback to each other, and working with musicians and costume designers to create a cohesive performance.
The Role of Patterns
Patterns play a crucial role in both coding and interpretive dance. In programming, design patterns are reusable solutions to common problems. In dance, patterns emerge in choreography, rhythm, and spatial arrangements.
In coding, an example of a design pattern might be the Observer pattern:
class Subject:
def __init__(self):
self._observers = []
self._state = None
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self._state)
def set_state(self, state):
self._state = state
self.notify()
class Observer:
def update(self, state):
pass
class ConcreteObserver(Observer):
def update(self, state):
print(f"State updated to: {state}")
In dance, patterns might emerge in the repetition and variation of movement phrases, the use of canonical structures, or the arrangement of dancers in geometric formations.
The Importance of Practice and Muscle Memory
Both coding and interpretive dance require extensive practice to master. Programmers spend countless hours writing code to internalize syntax, algorithms, and problem-solving techniques. Dancers similarly practice movements and sequences repeatedly to develop muscle memory and refine their technique.
In coding, this might involve solving coding challenges or working on personal projects:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Practice using the function
test_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = bubble_sort(test_array)
print(sorted_array)
In dance, practice might involve repeating a specific movement or sequence until it becomes second nature, allowing the dancer to focus on expression and emotion rather than the mechanics of the movement.
The Power of Visualization
Both coders and dancers use visualization techniques to enhance their craft. Programmers often use flowcharts, diagrams, and mental models to understand and design complex systems. Dancers visualize their movements, the space around them, and the emotions they want to convey.
In coding, visualization might involve creating a flowchart for an algorithm:
┌─────────────â”
│ Start │
└─────┬───────┘
│
┌─────▼───────â”
│ Input array │
└─────┬───────┘
│
┌─────▼───────â”
│ i = 0 │
└─────┬───────┘
│
┌─────▼───────â”
│ i < n-1 ◄─────â”
└─────┬───────┘ │
Yes │ No │
│ ┌────┴────â”
┌─────▼───────┠│ End │
│ j = 0 │ └────────┘
└─────┬───────┘
│
┌─────▼───────â”
│ j < n-i-1 ◄─────â”
└─────┬───────┘ │
Yes │ No │
│ ┌────┴────â”
┌─────▼───────┠│ i++ │
│arr[j]>arr[j+1│ └────┬────┘
└─────┬───────┘ │
Yes │ No │
│ ┌─────▼────â”
┌─────▼───────┠│ j++ │
│ Swap │ └─────────┘
└─────┬───────┘
│
└───────────────────┘
In dance, visualization might involve imagining the path of a movement through space or the energy flowing through the body during a performance.
The Role of Feedback and Iteration
Both coding and interpretive dance rely heavily on feedback and iteration. Programmers receive feedback through compiler errors, test results, and code reviews. Dancers receive feedback from mirrors, video recordings, instructors, and audience reactions.
In coding, feedback might come in the form of unit tests:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_zero(self):
self.assertEqual(add(0, 5), 5)
if __name__ == '__main__':
unittest.main()
In dance, feedback might involve watching video recordings of rehearsals or performances and making adjustments based on what is observed.
The Importance of Documentation and Notation
Both coding and interpretive dance have systems for documentation and notation. In programming, this includes code comments, documentation strings, and technical specifications. In dance, choreographers use dance notation systems like Labanotation or Benesh Movement Notation to record and communicate choreography.
In coding, documentation might look like this:
def calculate_area(length, width):
"""
Calculate the area of a rectangle.
Args:
length (float): The length of the rectangle.
width (float): The width of the rectangle.
Returns:
float: The area of the rectangle.
Raises:
ValueError: If length or width is negative.
"""
if length < 0 or width < 0:
raise ValueError("Length and width must be non-negative")
return length * width
In dance, notation might involve symbols and diagrams representing body positions, movements, and spatial relationships.
The Connection to Mathematics and Logic
Both coding and interpretive dance have strong connections to mathematics and logic. Programming is inherently mathematical, involving algorithms, data structures, and logical operations. Dance, particularly in its more abstract forms, can also express mathematical concepts through movement and spatial relationships.
In coding, mathematical concepts are often directly implemented:
import math
def calculate_circle_properties(radius):
area = math.pi * radius ** 2
circumference = 2 * math.pi * radius
return area, circumference
radius = 5
area, circumference = calculate_circle_properties(radius)
print(f"Circle with radius {radius}:")
print(f"Area: {area:.2f}")
print(f"Circumference: {circumference:.2f}")
In dance, mathematical concepts might be expressed through geometric formations, symmetrical movements, or rhythmic patterns based on numerical sequences.
The Importance of Continuous Learning
Both coding and interpretive dance require a commitment to lifelong learning. Technology is constantly evolving, and programmers must stay updated with new languages, frameworks, and best practices. Similarly, dancers continually refine their technique, learn new styles, and push the boundaries of their art form.
In coding, continuous learning might involve exploring new technologies:
import tensorflow as tf
# Creating a simple neural network
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(10,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(1)
])
model.compile(optimizer='adam', loss='mse')
# Training the model
model.fit(x_train, y_train, epochs=100, batch_size=32)
In dance, continuous learning might involve attending workshops, learning from other dancers, or experimenting with fusion styles that combine different dance traditions.
The Role of Community and Culture
Both coding and interpretive dance have vibrant communities and unique cultures. The tech community has its own events, conferences, and online forums where knowledge is shared and collaborations are formed. Similarly, the dance community has performances, competitions, and shared spaces where dancers come together to learn and create.
In coding, community involvement might include contributing to open-source projects:
git clone https://github.com/open-source-project/repo.git
cd repo
git checkout -b feature-branch
# Make changes and improvements
git add .
git commit -m "Add new feature"
git push origin feature-branch
# Create a pull request on GitHub
In dance, community involvement might include participating in dance jams, attending workshops, or organizing collaborative performances.
Conclusion: The Synergy Between Coding and Dance
As we’ve explored throughout this article, the connections between coding and interpretive dance are numerous and profound. Both disciplines require creativity, problem-solving skills, attention to detail, and a commitment to continuous improvement. They both involve expression through structured languages or movements, rely on patterns and abstraction, and benefit from collaboration and community involvement.
Understanding these connections can be valuable for both programmers and dancers. Programmers can draw inspiration from the fluidity and expressiveness of dance to write more elegant and creative code. Dancers can apply the logical thinking and problem-solving approaches of programming to develop innovative choreographies and improve their technical skills.
Moreover, recognizing these parallels can help break down the perceived barriers between STEM fields and the arts. It highlights the fact that creativity and logical thinking are not mutually exclusive but rather complementary skills that can enhance each other.
Whether you’re a coder looking to add some fluidity to your programming or a dancer seeking to bring more structure to your choreography, exploring the intersection of these two disciplines can lead to new insights and innovations in both fields. The unexpected connection between coding and interpretive dance serves as a reminder of the interconnectedness of human creativity and the potential for cross-disciplinary learning and growth.