The Ultimate Strategy for Navigating the Most Difficult Data Structure Questions
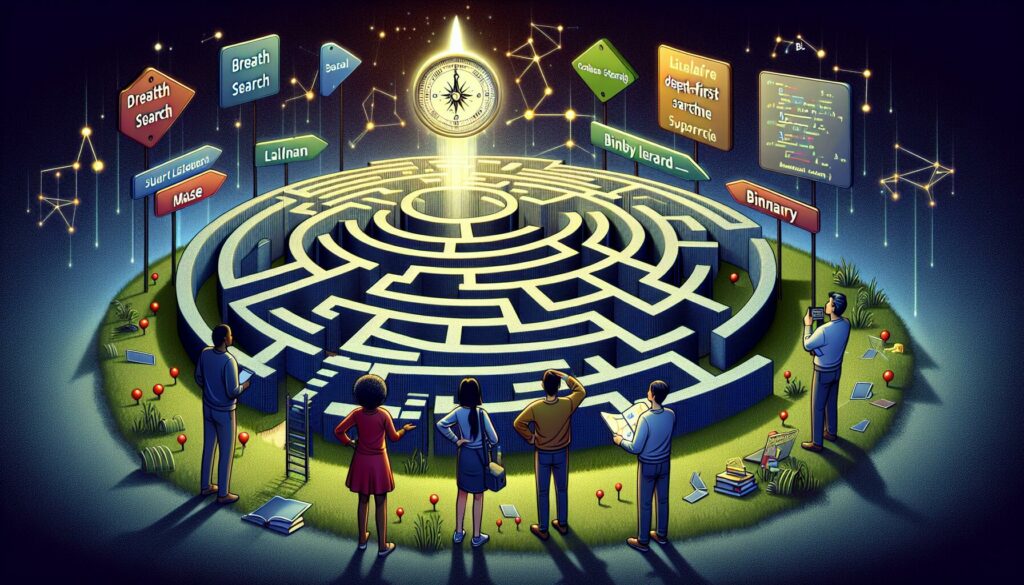
In the world of coding interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), data structure questions often pose the most significant challenge for candidates. These questions are designed to test not just your knowledge of various data structures but also your ability to apply them creatively to solve complex problems. In this comprehensive guide, we’ll explore strategies to tackle even the most difficult data structure questions, helping you navigate your way to success in technical interviews.
1. Build a Strong Foundation
Before diving into advanced problem-solving techniques, it’s crucial to have a solid understanding of fundamental data structures. This includes:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Ensure you understand the basic operations, time complexities, and use cases for each of these structures. Platforms like AlgoCademy offer interactive tutorials and exercises to help you master these fundamentals.
2. Develop a Systematic Approach
When faced with a difficult data structure question, follow these steps:
- Understand the problem: Read the question carefully and ask clarifying questions if needed.
- Identify the constraints: Note any time or space complexity requirements.
- Break down the problem: Divide the problem into smaller, manageable sub-problems.
- Choose appropriate data structures: Based on the problem requirements, select the most suitable data structures.
- Design the algorithm: Outline your approach before diving into coding.
- Implement the solution: Write clean, efficient code.
- Test and optimize: Run through test cases and refine your solution.
3. Master Common Patterns
Many difficult data structure questions follow certain patterns. Recognizing these patterns can significantly speed up your problem-solving process. Some common patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a Linked List
- Tree Breadth-First Search (BFS)
- Tree Depth-First Search (DFS)
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Practice problems that utilize these patterns to build your pattern recognition skills.
4. Optimize Your Solutions
Interviewers often look for candidates who can not only solve problems but also optimize their solutions. Here are some optimization techniques:
- Space-time tradeoff: Sometimes, using extra space can significantly reduce time complexity.
- Preprocessing: Organize data in a way that makes subsequent operations more efficient.
- Caching: Store frequently accessed results to avoid redundant computations.
- Amortized analysis: Some data structures (like dynamic arrays) have operations that are occasionally expensive but cheap on average.
5. Practice, Practice, Practice
Consistent practice is key to mastering difficult data structure questions. Here’s how to make your practice sessions effective:
- Solve diverse problems: Don’t stick to one type of problem or data structure.
- Time yourself: Practice under time constraints to simulate interview conditions.
- Review solutions: After solving a problem, look at other solutions to learn different approaches.
- Explain your solutions: Practice articulating your thought process, as you’ll need to do this in interviews.
6. Leverage Advanced Data Structures
While basic data structures form the foundation, knowledge of advanced structures can give you an edge in solving complex problems. Some advanced data structures to study include:
- Trie (Prefix Tree)
- Segment Tree
- Fenwick Tree (Binary Indexed Tree)
- Disjoint Set Union (Union-Find)
- Suffix Array and Suffix Tree
- Bloom Filter
Understanding these structures and their applications can help you tackle niche problems more efficiently.
7. Understand Time and Space Complexity
A crucial aspect of navigating difficult data structure questions is having a deep understanding of time and space complexity. This knowledge helps you:
- Evaluate the efficiency of your solutions
- Choose the most appropriate data structures for a given problem
- Optimize your algorithms effectively
Make sure you can analyze the time and space complexity of your solutions and explain your analysis to the interviewer.
8. Learn to Handle Edge Cases
Difficult data structure questions often come with tricky edge cases. To handle these effectively:
- Always consider empty or null inputs
- Think about boundary conditions (e.g., first or last elements in an array)
- Consider cases with minimal data (e.g., arrays with one or two elements)
- Test your solution with large inputs to check for scalability issues
9. Develop Problem-Solving Strategies
When faced with a particularly challenging question, consider these problem-solving strategies:
- Simplify the problem: Solve a simpler version first, then build up to the full solution.
- Use visualization: Draw diagrams or use tools to visualize the problem and potential solutions.
- Think aloud: Verbalize your thought process to engage the interviewer and potentially receive hints.
- Consider multiple approaches: Don’t fixate on your first idea; explore different solutions before coding.
10. Master Recursion and Dynamic Programming
Many difficult data structure questions involve recursion or can be optimized using dynamic programming. To excel in these areas:
- Practice identifying base cases and recursive relations
- Learn to transform recursive solutions into iterative ones
- Understand memoization and tabulation techniques for dynamic programming
- Study classic dynamic programming problems and their solutions
11. Implement Common Data Structure Operations
Being able to implement basic operations on data structures from scratch can be crucial. Practice implementing:
- Insertion, deletion, and search in linked lists
- Balancing operations in binary search trees
- BFS and DFS traversals on graphs
- Heap operations (heapify, insert, extract)
Here’s an example of implementing a basic operation on a binary search tree in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def insert_into_bst(root, val):
if not root:
return TreeNode(val)
if val < root.val:
root.left = insert_into_bst(root.left, val)
else:
root.right = insert_into_bst(root.right, val)
return root
12. Learn to Combine Multiple Data Structures
Some of the most challenging questions require combining multiple data structures. For example:
- Using a hash table with a linked list for LRU cache implementation
- Combining a stack with a hash table for certain parsing problems
- Using a trie with a heap for autocomplete functionality
Practice problems that require you to use multiple data structures in tandem.
13. Understand Trade-offs Between Different Data Structures
Each data structure has its strengths and weaknesses. Understanding these trade-offs is crucial for choosing the right structure for a given problem. For instance:
- Arrays offer constant-time access but linear-time insertion/deletion
- Linked lists provide constant-time insertion/deletion but linear-time access
- Hash tables offer average constant-time operations but can degrade to linear time in worst-case scenarios
Be prepared to discuss these trade-offs in your interviews.
14. Master String Manipulation Techniques
String manipulation is a common theme in data structure questions. Key techniques to master include:
- Sliding window for substring problems
- KMP algorithm for pattern matching
- Rabin-Karp algorithm for string searching
- Trie data structure for prefix-based operations
Here’s a simple implementation of the sliding window technique in Python:
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(n - k):
window_sum = window_sum - arr[i] + arr[i + k]
max_sum = max(max_sum, window_sum)
return max_sum
15. Develop Intuition for Data Structure Selection
As you practice, you’ll develop an intuition for which data structure to use for different types of problems. Some general guidelines:
- Use hash tables for problems involving frequency counting or quick lookup
- Consider using stacks for problems involving matching parentheses or undo operations
- Use heaps for problems involving finding the k-th largest/smallest element
- Consider using trees for hierarchical data or range queries
16. Learn to Implement Custom Data Structures
Sometimes, a problem may require you to implement a custom data structure. This could involve:
- Modifying an existing data structure to fit specific requirements
- Combining multiple data structures to create a new one
- Implementing a lesser-known data structure from scratch
Practice implementing structures like LRU Cache, Trie, or UnionFind to prepare for such scenarios.
17. Understand the Concept of Amortized Analysis
Some data structures, like dynamic arrays, have operations that seem expensive but are actually efficient when averaged over time. Understanding amortized analysis helps you:
- Accurately assess the true efficiency of certain data structures
- Explain why some seemingly expensive operations are actually efficient in practice
- Make informed decisions about data structure selection in complex scenarios
18. Practice Explaining Your Thought Process
In interviews, your thought process is often as important as your final solution. Practice:
- Explaining your approach before you start coding
- Discussing trade-offs between different potential solutions
- Walking through your code step-by-step after implementation
- Analyzing the time and space complexity of your solution
19. Learn to Handle System Design Questions
While not strictly data structure questions, system design problems often involve choosing and implementing appropriate data structures at scale. To prepare:
- Study how different data structures behave with very large datasets
- Learn about distributed data structures and their applications
- Understand how to scale data structures across multiple machines
20. Stay Updated with New Developments
The field of computer science is constantly evolving. Stay updated with:
- New data structures and their applications
- Improvements to existing data structures
- Emerging patterns in coding interviews
Platforms like AlgoCademy regularly update their content to reflect these changes, so make use of such resources to stay current.
Conclusion
Mastering difficult data structure questions is a journey that requires dedication, practice, and a systematic approach. By building a strong foundation, developing problem-solving strategies, and consistently practicing with diverse problems, you can significantly improve your ability to tackle even the most challenging questions in coding interviews.
Remember, the key is not just to memorize solutions, but to understand the underlying principles and develop the ability to apply them creatively to new problems. With persistence and the right approach, you can navigate the most difficult data structure questions with confidence and success.
Keep practicing, stay curious, and don’t be afraid to tackle problems that seem challenging at first. Every difficult question you solve is a step towards mastery. Good luck with your coding journey!