The Ultimate Roadmap to Becoming a Software Engineer: From Novice to FAANG-Ready
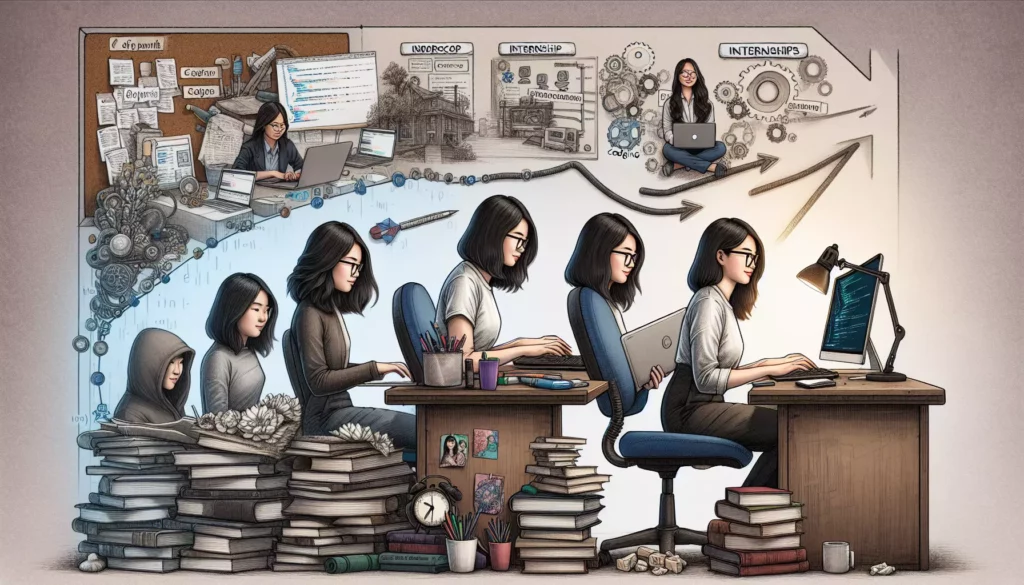
Embarking on the journey to become a software engineer can be both exciting and daunting. With the tech industry constantly evolving and the demand for skilled developers at an all-time high, it’s crucial to have a clear roadmap to guide you from your first lines of code to landing your dream job at a top tech company. In this comprehensive guide, we’ll walk you through the essential steps, skills, and resources you need to transform from a coding novice into a FAANG-ready software engineer.
1. Laying the Foundation: Understanding the Basics
Before diving into complex algorithms and advanced programming concepts, it’s essential to build a solid foundation in the basics of computer science and programming.
1.1. Choose Your First Programming Language
Start by selecting a beginner-friendly programming language. Popular choices include:
- Python: Known for its simplicity and readability
- JavaScript: Essential for web development
- Java: Widely used in enterprise applications
- C++: Preferred for system-level programming and game development
For beginners, Python is often recommended due to its straightforward syntax and versatility. Here’s a simple “Hello, World!” program in Python:
print("Hello, World!")
1.2. Master the Fundamentals
Regardless of the language you choose, focus on understanding these core concepts:
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
- Object-oriented programming (OOP) principles
- Basic data structures (arrays, lists, dictionaries)
1.3. Practice, Practice, Practice
Coding is a skill that improves with practice. Utilize platforms like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you solve problems and learn at your own pace.
2. Deepening Your Knowledge: Computer Science Fundamentals
While practical coding skills are important, a strong grasp of computer science fundamentals will set you apart and prepare you for more advanced topics.
2.1. Data Structures and Algorithms
Understanding data structures and algorithms is crucial for efficient problem-solving and optimizing code performance. Focus on:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
- Sorting and searching algorithms
- Dynamic programming
Here’s a simple implementation of a binary search algorithm in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
2.2. Operating Systems and Computer Architecture
Gain a basic understanding of how computers work at a lower level:
- Process management
- Memory management
- File systems
- CPU scheduling
- Concurrency and multithreading
2.3. Databases and SQL
Learn the basics of database management and SQL:
- Relational database concepts
- SQL queries (SELECT, INSERT, UPDATE, DELETE)
- Indexing and optimization
- NoSQL databases
3. Building Projects: Applying Your Knowledge
Theory alone isn’t enough. To truly understand and internalize concepts, you need to apply them in real-world projects.
3.1. Start Small
Begin with simple projects that reinforce the fundamentals:
- Calculator app
- To-do list manager
- Simple game (e.g., Tic-Tac-Toe)
- Weather app using an API
3.2. Gradually Increase Complexity
As you gain confidence, tackle more complex projects:
- Full-stack web application
- Mobile app
- Data analysis tool
- Machine learning project
3.3. Contribute to Open Source
Contributing to open-source projects is an excellent way to:
- Gain real-world experience
- Collaborate with other developers
- Build your portfolio
- Learn best practices and coding standards
4. Mastering Software Development Tools and Practices
To work efficiently and collaboratively, familiarize yourself with essential tools and practices used in professional software development.
4.1. Version Control
Learn to use Git and GitHub for version control. Key concepts include:
- Repositories
- Commits
- Branches
- Pull requests
- Merge conflicts
4.2. Integrated Development Environments (IDEs)
Become proficient in using IDEs like:
- Visual Studio Code
- IntelliJ IDEA
- PyCharm
- Eclipse
4.3. Agile Methodologies
Understand common software development methodologies:
- Scrum
- Kanban
- Extreme Programming (XP)
4.4. Continuous Integration and Continuous Deployment (CI/CD)
Learn the basics of automating the build, test, and deployment processes:
- Jenkins
- Travis CI
- GitLab CI
- GitHub Actions
5. Specializing: Choosing Your Path
As you progress, you may want to specialize in a particular area of software engineering. Some popular specializations include:
5.1. Web Development
- Front-end: HTML, CSS, JavaScript, React, Angular, or Vue.js
- Back-end: Node.js, Django, Ruby on Rails, or Spring Boot
- Full-stack: Combining front-end and back-end skills
5.2. Mobile Development
- iOS: Swift, Objective-C
- Android: Java, Kotlin
- Cross-platform: React Native, Flutter
5.3. Data Science and Machine Learning
- Python libraries: NumPy, Pandas, Scikit-learn
- Machine learning frameworks: TensorFlow, PyTorch
- Big data technologies: Hadoop, Spark
5.4. DevOps and Cloud Computing
- Cloud platforms: AWS, Google Cloud, Azure
- Containerization: Docker, Kubernetes
- Infrastructure as Code: Terraform, Ansible
6. Preparing for Technical Interviews
As you set your sights on top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), it’s crucial to prepare for their rigorous interview processes.
6.1. Master Data Structures and Algorithms
Revisit and deepen your understanding of data structures and algorithms. Practice solving complex problems on platforms like AlgoCademy, LeetCode, and HackerRank.
6.2. System Design
For more senior positions, you’ll need to understand system design principles:
- Scalability
- Load balancing
- Caching
- Database sharding
- Microservices architecture
6.3. Behavioral Interviews
Prepare for behavioral questions by reflecting on your experiences and using the STAR method (Situation, Task, Action, Result) to structure your responses.
6.4. Mock Interviews
Practice with peers or use platforms that offer mock interviews with experienced engineers. This will help you get comfortable with the interview format and receive valuable feedback.
7. Continuous Learning and Staying Up-to-Date
The field of software engineering is constantly evolving. To stay competitive and grow in your career, make continuous learning a habit.
7.1. Follow Industry Trends
- Subscribe to tech blogs and newsletters
- Attend conferences and meetups
- Follow influential developers and companies on social media
7.2. Online Courses and Certifications
Platforms like Coursera, edX, and Udacity offer advanced courses and specializations in various areas of software engineering.
7.3. Read Books and Research Papers
Deepen your knowledge by reading classic computer science books and staying current with research in your areas of interest.
7.4. Teach Others
Teaching is an excellent way to solidify your understanding of concepts. Consider:
- Writing blog posts or tutorials
- Giving presentations at local meetups
- Mentoring junior developers
Conclusion: Your Journey to Becoming a Software Engineer
Becoming a software engineer, especially one capable of working at top tech companies, is a challenging but rewarding journey. By following this roadmap and consistently putting in the effort to learn, practice, and grow, you’ll be well on your way to achieving your goals.
Remember, everyone’s path is unique, and it’s okay to adjust this roadmap to fit your personal goals and learning style. The key is to stay curious, persistent, and passionate about solving problems through code.
As you progress through your journey, platforms like AlgoCademy can be invaluable resources, offering structured learning paths, interactive coding challenges, and AI-powered assistance to help you tackle complex problems and prepare for technical interviews.
Whether you’re dreaming of building the next revolutionary app, contributing to cutting-edge AI research, or solving complex scalability issues at a tech giant, the skills and knowledge you gain along this roadmap will serve as a solid foundation for a successful career in software engineering.
So, take that first step, write your first line of code, and embark on this exciting journey to become a world-class software engineer. The tech world is waiting for your contributions!