The Ultimate Guide to DApp Development: Building Decentralized Applications on the Blockchain
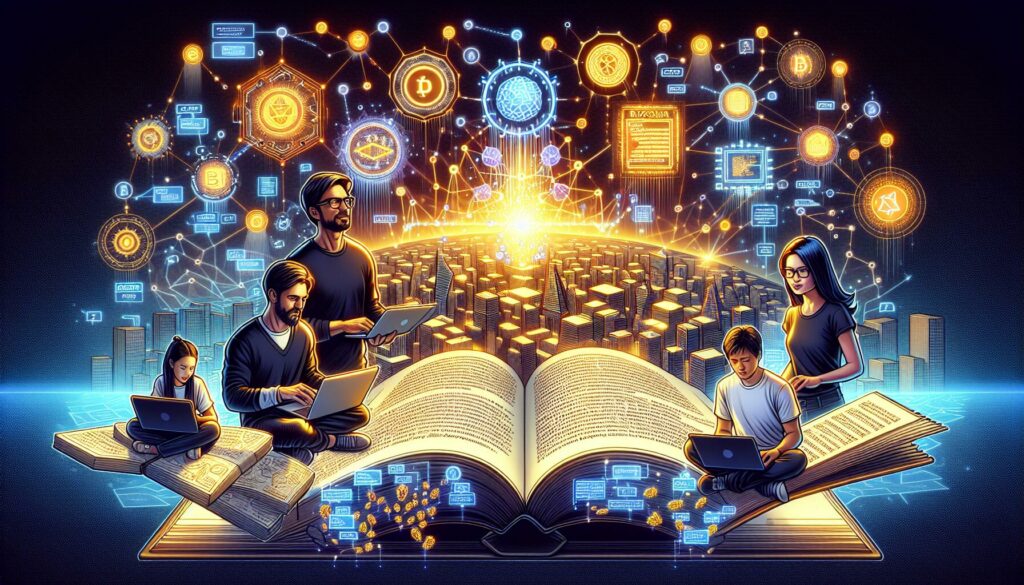
Table of Contents
- Introduction to DApps
- Understanding Blockchain Technology
- Key Components of a DApp
- Choosing the Right Blockchain Platform
- Smart Contracts: The Backbone of DApps
- Essential Development Tools and Frameworks
- Step-by-Step Guide to Building a DApp
- Frontend Development for DApps
- Testing and Deploying Your DApp
- Security Considerations in DApp Development
- Scalability Challenges and Solutions
- Improving User Experience in DApps
- Monetization Strategies for DApps
- Future Trends in DApp Development
- Conclusion
1. Introduction to DApps
Decentralized Applications, or DApps, have emerged as a revolutionary concept in the world of software development. Unlike traditional applications that run on centralized servers, DApps operate on a decentralized network of computers, typically a blockchain. This paradigm shift brings numerous advantages, including increased security, transparency, and resistance to censorship.
At its core, a DApp consists of two main components:
- A backend smart contract running on a blockchain network
- A frontend user interface that interacts with the smart contract
The decentralized nature of DApps means that no single entity has control over the application’s operation, making them ideal for scenarios where trust and transparency are paramount. From financial services to supply chain management, DApps are disrupting various industries and opening up new possibilities for innovation.
2. Understanding Blockchain Technology
Before diving into DApp development, it’s crucial to have a solid understanding of blockchain technology. A blockchain is a distributed ledger that records transactions across a network of computers. Each block in the chain contains a cryptographic hash of the previous block, a timestamp, and transaction data, making it virtually impossible to alter past records without detection.
Key features of blockchain technology include:
- Decentralization: No central authority controls the network
- Transparency: All transactions are visible to network participants
- Immutability: Once recorded, data cannot be altered without consensus
- Security: Cryptographic techniques ensure data integrity
Blockchain networks can be public (like Bitcoin or Ethereum) or private (permissioned networks for enterprises). Understanding the differences between these types and their consensus mechanisms (e.g., Proof of Work, Proof of Stake) is essential for choosing the right platform for your DApp.
3. Key Components of a DApp
A typical DApp consists of several key components:
- Smart Contracts: Self-executing code that runs on the blockchain
- Frontend Interface: The user-facing part of the application
- Web3 Library: Enables interaction between the frontend and the blockchain
- Blockchain Network: The underlying infrastructure where the DApp operates
- Storage Solution: For storing large amounts of data off-chain (e.g., IPFS)
Each of these components plays a crucial role in the functioning of a DApp. Smart contracts handle the business logic and data storage on the blockchain, while the frontend provides a user-friendly interface for interacting with the DApp. The Web3 library (such as Web3.js or ethers.js) acts as a bridge between the frontend and the blockchain, allowing developers to send transactions and interact with smart contracts.
4. Choosing the Right Blockchain Platform
Selecting the appropriate blockchain platform is a critical decision in DApp development. While Ethereum remains the most popular choice, several other platforms have emerged, each with its own strengths and weaknesses. Here are some factors to consider:
- Ethereum: Largest ecosystem, robust tooling, but faces scalability issues
- Binance Smart Chain: High performance, low fees, but more centralized
- Polkadot: Interoperability focused, allows custom blockchains (parachains)
- Cardano: Academic approach, focus on sustainability and scalability
- Solana: High throughput, low fees, but newer ecosystem
Consider factors such as transaction speed, cost, developer community, and the specific requirements of your DApp when making this decision. It’s also worth noting that some platforms offer cross-chain compatibility, allowing your DApp to operate on multiple blockchains.
5. Smart Contracts: The Backbone of DApps
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They are the backbone of most DApps, handling the business logic and data storage on the blockchain. Here’s what you need to know about smart contract development:
Languages for Smart Contract Development
- Solidity: The most popular language for Ethereum and EVM-compatible chains
- Vyper: A contract-oriented, pythonic programming language for Ethereum
- Rust: Used for developing on platforms like Solana and NEAR
Key Concepts in Smart Contract Development
- State variables and data types
- Functions and modifiers
- Events and logging
- Gas optimization
- Inheritance and interfaces
Here’s a simple example of a Solidity smart contract:
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 private storedData;
function set(uint256 x) public {
storedData = x;
}
function get() public view returns (uint256) {
return storedData;
}
}
This contract allows storing and retrieving a single unsigned integer value. While simple, it demonstrates the basic structure of a smart contract.
6. Essential Development Tools and Frameworks
To streamline the DApp development process, several tools and frameworks have been created. Here are some essential ones:
Development Environments
- Remix: Browser-based IDE for Solidity development
- Truffle Suite: Development framework for Ethereum
- Hardhat: Ethereum development environment for professionals
Libraries and Frameworks
- Web3.js: JavaScript library for interacting with Ethereum
- ethers.js: Complete Ethereum library and wallet implementation
- OpenZeppelin: Library for secure smart contract development
Testing Tools
- Ganache: Personal blockchain for Ethereum development
- Mocha: JavaScript test framework
- Chai: BDD / TDD assertion library for node
Familiarizing yourself with these tools will significantly enhance your productivity and the quality of your DApps.
7. Step-by-Step Guide to Building a DApp
Let’s walk through the process of building a simple DApp:
- Set up the development environment: Install Node.js, Truffle, and Ganache
- Create a new Truffle project: Run
truffle init
in your project directory - Write the smart contract: Create a new Solidity file in the
contracts/
directory - Compile the smart contract: Run
truffle compile
- Set up migration: Create a migration script in the
migrations/
directory - Deploy to local blockchain: Start Ganache and run
truffle migrate
- Develop the frontend: Create HTML, CSS, and JavaScript files
- Connect frontend to smart contract: Use Web3.js or ethers.js
- Test the DApp: Write and run tests using Mocha and Chai
- Deploy to testnet: Use a network like Rinkeby or Ropsten for testing
- Deploy to mainnet: Once thoroughly tested, deploy to the main network
This process may vary depending on the specific blockchain platform and tools you’re using, but the general flow remains similar.
8. Frontend Development for DApps
While the backend of a DApp runs on the blockchain, the frontend is typically a web application that interacts with the smart contracts. Here are some considerations for frontend development:
Web3 Integration
To interact with the blockchain, you’ll need to integrate a Web3 library into your frontend. This allows you to connect to a user’s wallet (like MetaMask) and send transactions to the blockchain.
Here’s a simple example of connecting to MetaMask using Web3.js:
if (typeof window.ethereum !== 'undefined') {
console.log('MetaMask is installed!');
window.ethereum.request({ method: 'eth_requestAccounts' })
.then((accounts) => {
console.log('Connected to account:', accounts[0]);
})
.catch((error) => {
console.error('Error connecting to MetaMask', error);
});
} else {
console.log('Please install MetaMask!');
}
UI Frameworks
Popular JavaScript frameworks like React, Vue.js, or Angular can be used to build the user interface of your DApp. These frameworks provide robust tools for creating interactive and responsive web applications.
Design Considerations
When designing the frontend of a DApp, consider the following:
- Provide clear feedback for blockchain transactions, which can take time to process
- Implement proper error handling for failed transactions
- Design for different wallet states (connected, disconnected, wrong network)
- Ensure responsiveness for both desktop and mobile devices
9. Testing and Deploying Your DApp
Thorough testing is crucial in DApp development due to the immutable nature of blockchain transactions. Here’s an overview of the testing and deployment process:
Testing
- Unit Testing: Test individual functions in your smart contracts
- Integration Testing: Test the interaction between multiple contracts
- Frontend Testing: Test the user interface and its interaction with smart contracts
- Network Testing: Test on testnets to simulate real-world conditions
Here’s an example of a simple Truffle test:
const SimpleStorage = artifacts.require("SimpleStorage");
contract("SimpleStorage", (accounts) => {
it("should store the value 89", async () => {
const simpleStorageInstance = await SimpleStorage.deployed();
await simpleStorageInstance.set(89, { from: accounts[0] });
const storedData = await simpleStorageInstance.get.call();
assert.equal(storedData, 89, "The value 89 was not stored.");
});
});
Deployment
- Local Deployment: Deploy to a local blockchain (like Ganache) for development
- Testnet Deployment: Deploy to a public testnet for more realistic testing
- Mainnet Deployment: Deploy to the main network when ready for production
Remember to thoroughly test your DApp on a testnet before deploying to mainnet, as mistakes on the mainnet can be costly and irreversible.
10. Security Considerations in DApp Development
Security is paramount in DApp development due to the financial nature of many blockchain applications. Here are some key security considerations:
- Smart Contract Audits: Have your smart contracts audited by professionals
- Reentrancy Guards: Protect against reentrancy attacks
- Access Control: Implement proper access controls in your smart contracts
- Gas Limitations: Be aware of gas limits and potential DOS attacks
- Avoid Common Pitfalls: Such as integer overflow/underflow, unchecked return values
- Use Established Libraries: Leverage audited libraries like OpenZeppelin
- Keep Private Keys Secure: Never expose private keys in your frontend code
Here’s an example of a simple reentrancy guard in Solidity:
contract ReentrancyGuard {
bool private _notEntered;
constructor () internal {
_notEntered = true;
}
modifier nonReentrant() {
require(_notEntered, "Reentrant call");
_notEntered = false;
_;
_notEntered = true;
}
}
11. Scalability Challenges and Solutions
Scalability remains one of the biggest challenges in blockchain technology and DApp development. Here are some common scalability issues and potential solutions:
Challenges
- High transaction fees during network congestion
- Slow transaction confirmation times
- Limited throughput (transactions per second)
Solutions
- Layer 2 Solutions: Off-chain scaling solutions like state channels and sidechains
- Sharding: Splitting the network into multiple shards to process transactions in parallel
- Optimistic Rollups: Bundling multiple transactions into a single on-chain transaction
- Plasma Chains: Child chains that periodically commit to the main chain
When developing a DApp, consider integrating with Layer 2 solutions or choosing a blockchain platform that addresses these scalability concerns.
12. Improving User Experience in DApps
User experience (UX) is crucial for the adoption of DApps. Here are some tips to improve the UX of your DApp:
- Simplify Onboarding: Make it easy for new users to get started
- Abstract Complexity: Hide blockchain complexities from the user where possible
- Provide Clear Feedback: Keep users informed about the status of their transactions
- Optimize Gas Usage: Implement strategies to minimize transaction costs
- Support Multiple Wallets: Allow users to connect with various wallet providers
- Responsive Design: Ensure your DApp works well on both desktop and mobile devices
Consider using tools like WalletConnect to provide a seamless wallet connection experience across different devices and platforms.
13. Monetization Strategies for DApps
While many DApps are open-source and free to use, developers need sustainable monetization strategies. Here are some approaches:
- Transaction Fees: Charge a small fee for each transaction processed by your DApp
- Token Model: Create a utility token for your DApp ecosystem
- Subscription Model: Offer premium features for a recurring fee
- NFTs: Sell unique digital assets related to your DApp
- Advertising: Display ads in your DApp (though this should be done carefully)
- Freemium Model: Offer basic features for free and charge for advanced functionality
Choose a monetization strategy that aligns with your DApp’s purpose and user base. Remember that transparency is key in the blockchain world, so be clear about your monetization approach.
14. Future Trends in DApp Development
The world of DApp development is rapidly evolving. Here are some trends to watch:
- Interoperability: Cross-chain DApps that can operate on multiple blockchains
- DeFi Integration: More traditional applications integrating DeFi functionality
- NFT Utility: Expanding use cases for NFTs beyond digital art
- DAO Frameworks: Tools for creating and managing Decentralized Autonomous Organizations
- Privacy Solutions: Implementations of zero-knowledge proofs and other privacy-enhancing technologies
- Improved Developer Tools: More sophisticated IDEs and debugging tools for blockchain development
Staying informed about these trends can help you build more innovative and future-proof DApps.
15. Conclusion
DApp development is an exciting and rapidly evolving field at the intersection of blockchain technology and software development. By leveraging the power of decentralized networks, DApps have the potential to revolutionize various industries and create new paradigms for user interaction and value exchange.
As you embark on your journey in DApp development, remember these key points:
- Understand the fundamentals of blockchain technology
- Choose the right blockchain platform for your needs
- Master smart contract development and security best practices
- Create user-friendly interfaces that abstract blockchain complexities
- Thoroughly test your DApps before deploying to mainnet
- Stay informed about the latest trends and technologies in the space
With dedication and continuous learning, you can create innovative DApps that contribute to the growing ecosystem of decentralized applications. The future of DApps is bright, and the possibilities are limitless. Happy building!