The Ultimate Coding Interview Study Plan: Your Path to Success
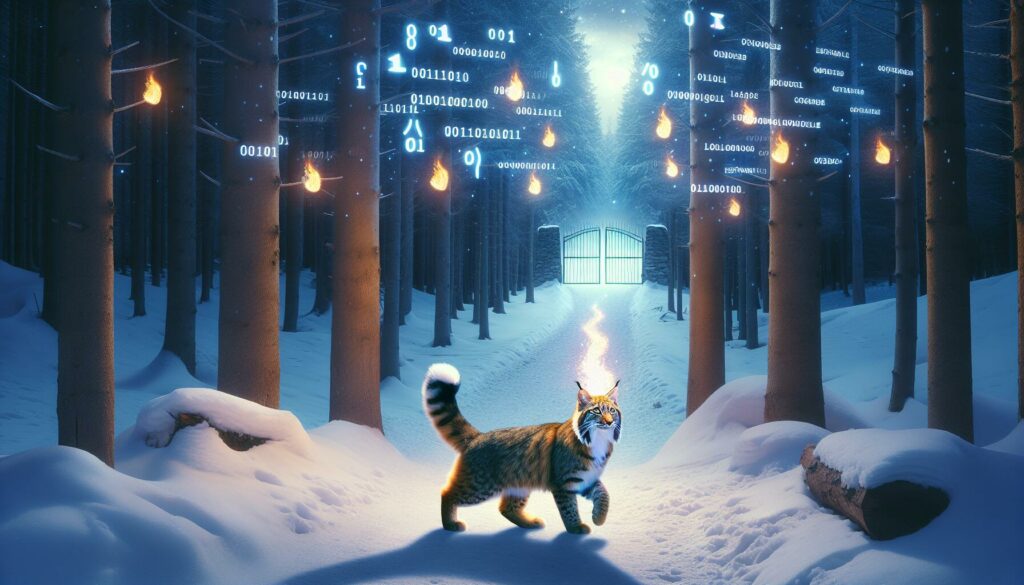
Preparing for a coding interview can be a daunting task, especially when you’re aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). However, with the right study plan and resources, you can significantly improve your chances of success. In this comprehensive guide, we’ll outline an effective coding interview study plan that will help you sharpen your skills and boost your confidence. Whether you’re a recent graduate or an experienced developer looking to make a career move, this plan will set you on the path to acing your next coding interview.
1. Assess Your Current Skills
Before diving into your study plan, it’s crucial to evaluate your current skill level. This assessment will help you identify areas that need improvement and allow you to tailor your study plan accordingly.
Steps for Self-Assessment:
- Take online coding assessments on platforms like HackerRank or LeetCode
- Review job descriptions for positions you’re interested in and compare your skills to the requirements
- Reflect on your past projects and identify areas where you struggled or excelled
- Seek feedback from peers or mentors on your coding abilities
2. Master the Fundamentals
Strong foundational knowledge is essential for success in coding interviews. Ensure you have a solid grasp of the following core concepts:
Key Areas to Focus On:
- Data Structures: Arrays, Linked Lists, Stacks, Queues, Trees, Graphs, Hash Tables
- Algorithms: Sorting, Searching, Recursion, Dynamic Programming
- Time and Space Complexity Analysis (Big O notation)
- Object-Oriented Programming (OOP) principles
- Basic design patterns
Resources for mastering fundamentals:
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- Online courses on platforms like Coursera, edX, or Udacity
- AlgoCademy’s interactive tutorials and problem-solving exercises
3. Practice, Practice, Practice
Consistent practice is key to improving your problem-solving skills and becoming more comfortable with coding under pressure. Aim to solve a variety of problems regularly.
Effective Practice Strategies:
- Solve at least one coding problem daily
- Use platforms like LeetCode, HackerRank, or CodeSignal for a wide range of problems
- Focus on problems commonly asked in interviews (e.g., array manipulation, string operations, tree traversals)
- Time yourself while solving problems to simulate interview conditions
- Review and learn from others’ solutions after solving a problem
Pro Tip: Use AlgoCademy’s AI-powered assistance to get hints and explanations when you’re stuck on a problem. This feature can help you learn new approaches and optimize your solutions.
4. Learn and Implement Efficient Algorithms
Understanding and implementing efficient algorithms is crucial for solving complex problems quickly. Familiarize yourself with common algorithmic techniques and their applications.
Key Algorithmic Techniques to Master:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Graph Algorithms (BFS, DFS, Dijkstra’s, etc.)
- Bit Manipulation
Practice implementing these algorithms from scratch to deepen your understanding. AlgoCademy offers guided tutorials on these techniques, helping you grasp the concepts and apply them to various problems.
5. Develop Your Problem-Solving Approach
Having a structured approach to problem-solving can significantly improve your performance in coding interviews. Follow these steps when tackling a problem:
- Understand the problem: Ask clarifying questions and identify constraints
- Break down the problem into smaller, manageable parts
- Consider multiple approaches and discuss trade-offs
- Choose the most appropriate solution
- Plan your implementation before coding
- Write clean, well-commented code
- Test your solution with various inputs, including edge cases
- Optimize your solution if time permits
Practice verbalizing your thought process while solving problems. This skill is crucial during interviews, as interviewers want to understand your reasoning and problem-solving approach.
6. Study System Design and Scalability
For more senior positions or roles at larger companies, system design questions are often a significant part of the interview process. Familiarize yourself with key concepts and best practices in designing large-scale systems.
Topics to Cover in System Design:
- Scalability and Load Balancing
- Database Design and Optimization
- Caching Strategies
- Microservices Architecture
- API Design
- Distributed Systems
- Security and Authentication
Resources for system design preparation:
- “Designing Data-Intensive Applications” by Martin Kleppmann
- “System Design Interview – An Insider’s Guide” by Alex Xu
- Online courses focused on system design and architecture
- AlgoCademy’s advanced modules on system design principles and case studies
7. Mock Interviews and Peer Practice
Simulating real interview conditions is an excellent way to prepare for the actual experience. Engage in mock interviews and peer coding sessions to improve your skills and gain confidence.
Ways to Practice Interview-Style Coding:
- Use platforms like Pramp or InterviewBit for mock interviews with peers
- Ask friends or colleagues to conduct mock interviews for you
- Record yourself solving problems and review your performance
- Participate in coding competitions or hackathons to practice under time pressure
- Utilize AlgoCademy’s interview simulation feature to experience realistic interview scenarios
Remember to practice both the technical aspects and soft skills, such as communication and problem-solving under pressure.
8. Stay Updated with Industry Trends
The tech industry is constantly evolving, and staying informed about current trends and technologies can give you an edge in interviews. Keep yourself updated on the latest developments in your field of interest.
Ways to Stay Informed:
- Follow tech blogs and news websites
- Attend industry conferences or webinars
- Participate in online developer communities and forums
- Contribute to open-source projects
- Experiment with new technologies and frameworks in personal projects
AlgoCademy regularly updates its content to reflect current industry trends and interview patterns, ensuring you’re always prepared with relevant knowledge.
9. Brush Up on Computer Science Fundamentals
While practical coding skills are crucial, many interviews also test your understanding of computer science fundamentals. Review key concepts in areas such as:
- Operating Systems
- Computer Networks
- Database Management Systems
- Software Engineering Principles
- Programming Language Concepts
Resources for reviewing CS fundamentals:
- “Computer Science Distilled” by Wladston Ferreira Filho
- Online computer science courses from reputable universities
- AlgoCademy’s comprehensive CS fundamentals module
10. Develop a Consistent Study Routine
Creating and sticking to a consistent study routine is crucial for long-term success in your interview preparation. Here’s a sample weekly study plan:
Sample Weekly Study Plan:
- Monday-Friday: Solve 1-2 coding problems daily (1-2 hours)
- Tuesday & Thursday: Study algorithms and data structures (1 hour each day)
- Wednesday: System design practice or study (1-2 hours)
- Saturday: Mock interview or longer problem-solving session (2-3 hours)
- Sunday: Review and reflect on the week’s progress, plan for the upcoming week (1 hour)
Adjust this plan based on your schedule and learning style. Consistency is more important than the exact number of hours spent studying.
11. Learn to Optimize Your Code
Interviewers often ask candidates to optimize their initial solutions. Practice identifying inefficiencies in your code and applying optimization techniques.
Common Optimization Techniques:
- Using appropriate data structures (e.g., hash tables for O(1) lookup)
- Eliminating unnecessary computations
- Applying caching or memoization
- Utilizing space-time trade-offs
- Implementing more efficient algorithms
AlgoCademy’s step-by-step guidance feature can help you learn these optimization techniques by showing you how to improve your initial solutions.
12. Familiarize Yourself with Coding Interview Formats
Different companies have varying interview formats. Research and prepare for the specific types of interviews you might encounter.
Common Coding Interview Formats:
- Whiteboard coding
- Live coding with an interviewer
- Take-home coding assignments
- Pair programming sessions
- Online coding assessments
Practice with different formats to feel comfortable regardless of the interview style you encounter.
13. Develop Your Soft Skills
While technical skills are crucial, soft skills can often make or break an interview. Focus on improving:
- Communication: Clearly explain your thought process and solutions
- Collaboration: Show your ability to work well with others
- Problem-solving: Demonstrate a structured approach to tackling challenges
- Adaptability: Show willingness to learn and adapt to new information
- Time management: Practice working efficiently under time constraints
AlgoCademy’s interview simulation feature includes feedback on both technical skills and soft skills, helping you improve your overall interview performance.
14. Create a GitHub Portfolio
A well-maintained GitHub profile can showcase your coding skills and projects to potential employers. Consider the following:
- Contribute to open-source projects
- Showcase personal projects that demonstrate your skills
- Maintain clean, well-documented code in your repositories
- Include a detailed README for each project
- Keep your contributions graph active
15. Prepare for Behavioral Questions
Many coding interviews also include behavioral questions to assess your fit within the company culture. Prepare for questions like:
- “Tell me about a time when you faced a significant technical challenge.”
- “How do you handle disagreements with team members?”
- “Describe a project you’re particularly proud of.”
Use the STAR method (Situation, Task, Action, Result) to structure your responses to behavioral questions.
16. Review Company-Specific Information
Before each interview, research the company thoroughly:
- Understand the company’s products, services, and recent news
- Review the job description and required skills
- Research the company’s tech stack and development practices
- Prepare questions to ask your interviewers about the company and role
17. Practice Coding in a Simple Text Editor
While IDEs are helpful in day-to-day coding, many interviews require coding without such tools. Practice writing code in a simple text editor to simulate interview conditions.
18. Learn to Handle Ambiguity
Interviewers often present vague problems to see how you handle uncertainty. Practice:
- Asking clarifying questions
- Making and stating assumptions
- Breaking down ambiguous problems into manageable parts
19. Develop a Pre-Interview Routine
Establish a routine to follow before each interview:
- Get a good night’s sleep
- Review key concepts and common problems
- Prepare your interview space (for remote interviews)
- Do a quick warm-up coding problem
- Practice deep breathing or meditation to calm nerves
20. Post-Interview Reflection and Improvement
After each interview, take time to reflect:
- Note questions you found challenging
- Identify areas for improvement
- Research correct solutions for problems you struggled with
- Adjust your study plan based on your performance
Conclusion
Preparing for coding interviews is a challenging but rewarding process. By following this comprehensive study plan and utilizing resources like AlgoCademy, you can significantly improve your chances of success. Remember that consistent practice, a structured approach to problem-solving, and continuous learning are key to performing well in coding interviews.
As you progress through your study plan, take advantage of AlgoCademy’s features such as AI-powered assistance, step-by-step guidance, and interview simulations to enhance your learning experience. With dedication and the right resources, you’ll be well-equipped to tackle even the most challenging coding interviews at top tech companies.
Good luck with your interview preparation, and remember that each interview, regardless of the outcome, is an opportunity to learn and grow as a developer!