The Ultimate Big O Notation Cheat Sheet: Mastering Algorithm Efficiency
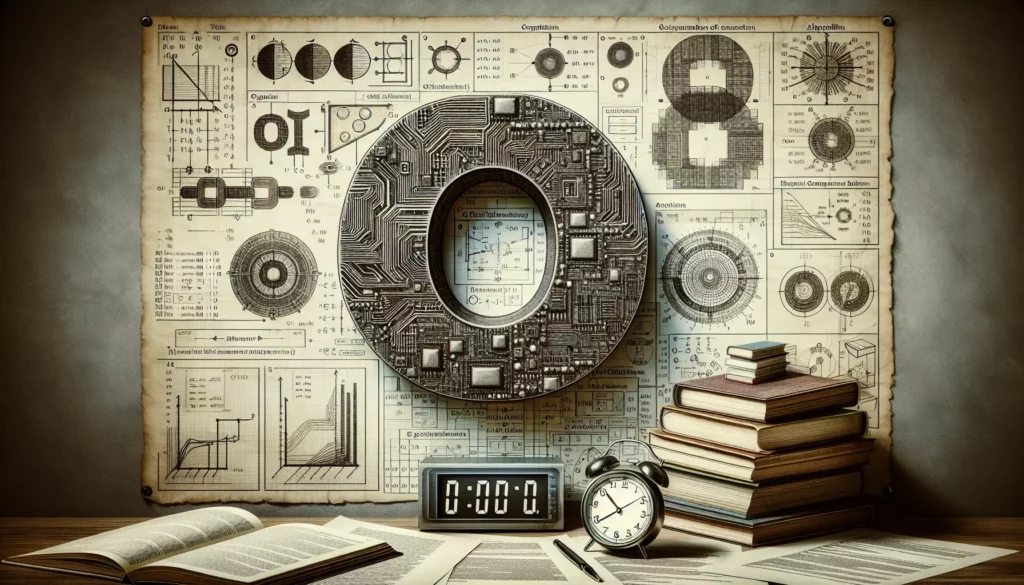
Welcome to AlgoCademy’s comprehensive guide to Big O notation! If you’re preparing for technical interviews at top tech companies or simply want to level up your coding skills, understanding Big O is crucial. This cheat sheet will help you grasp the fundamentals of algorithm efficiency and give you the tools to analyze and optimize your code.
What is Big O Notation?
Big O notation is a mathematical notation used in computer science to describe the performance or complexity of an algorithm. Specifically, it describes the worst-case scenario, or the maximum time it takes to execute as a function of the input size. Big O is essential for several reasons:
- It helps you understand how your algorithm’s performance scales with input size
- It allows you to compare different algorithms and choose the most efficient one
- It’s a common language for discussing algorithm efficiency in technical interviews
Common Big O Complexities
Let’s dive into the most common Big O complexities you’ll encounter, from best to worst:
O(1) – Constant Time
This is the holy grail of algorithm efficiency. No matter how large the input, the algorithm always takes the same amount of time to execute.
Example: Accessing an array element by index
function getElement(arr, index) {
return arr[index];
}
O(log n) – Logarithmic Time
These algorithms are highly efficient, especially for large datasets. The time complexity grows logarithmically with the input size.
Example: Binary search in a sorted array
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
O(n) – Linear Time
The execution time grows linearly with the input size. These algorithms are generally considered efficient for small to medium-sized inputs.
Example: Linear search in an unsorted array
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
O(n log n) – Linearithmic Time
This complexity is common in efficient sorting algorithms. It’s more efficient than O(n^2) but less efficient than O(n).
Example: Merge Sort
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
O(n^2) – Quadratic Time
These algorithms become inefficient as the input size grows. They’re often a result of nested loops.
Example: Bubble Sort
function bubbleSort(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
O(2^n) – Exponential Time
These algorithms have a runtime that doubles with each addition to the input. They’re often used in recursive algorithms solving complex problems.
Example: Recursive Fibonacci sequence
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
O(n!) – Factorial Time
This is the least efficient Big O complexity. The algorithm’s runtime grows factorially with the input size.
Example: Generating all permutations of a string
function getPermutations(str) {
if (str.length <= 1) return [str];
let permutations = [];
for (let i = 0; i < str.length; i++) {
let char = str[i];
let remainingChars = str.slice(0, i) + str.slice(i + 1);
let innerPermutations = getPermutations(remainingChars);
for (let perm of innerPermutations) {
permutations.push(char + perm);
}
}
return permutations;
}
Tips for Optimizing Algorithm Efficiency
Now that you understand Big O notation, here are some tips to help you write more efficient algorithms:
- Avoid nested loops when possible: Nested loops often lead to O(n^2) complexity or worse.
- Use appropriate data structures: Different data structures have different time complexities for various operations. Choose wisely based on your needs.
- Consider space-time tradeoffs: Sometimes, you can improve time complexity by using more memory, or vice versa.
- Divide and conquer: Break down complex problems into smaller, manageable parts. This often leads to more efficient solutions.
- Use memoization: For recursive algorithms, store previously computed results to avoid redundant calculations.
- Understand the problem constraints: Sometimes, a theoretically less efficient algorithm might perform better for small inputs or specific use cases.
Common Data Structure Operations and Their Big O Complexities
Understanding the time complexities of common data structure operations is crucial for writing efficient code. Here’s a quick reference:
Array
- Access: O(1)
- Search: O(n)
- Insertion: O(n)
- Deletion: O(n)
Linked List
- Access: O(n)
- Search: O(n)
- Insertion (at beginning): O(1)
- Deletion (at beginning): O(1)
Hash Table
- Search: O(1) average, O(n) worst case
- Insertion: O(1) average, O(n) worst case
- Deletion: O(1) average, O(n) worst case
Binary Search Tree
- Search: O(log n) average, O(n) worst case
- Insertion: O(log n) average, O(n) worst case
- Deletion: O(log n) average, O(n) worst case
Big O in Practice: Real-World Examples
Let’s look at some real-world scenarios where understanding Big O can make a significant difference:
1. Social Media Feed
Imagine you’re designing a social media feed that displays posts from a user’s friends. A naive approach might be to loop through all posts and check if each one is from a friend:
function getFriendPosts(allPosts, friends) {
return allPosts.filter(post => friends.includes(post.author));
}
This has a time complexity of O(n * m), where n is the number of posts and m is the number of friends. For users with many friends and posts, this could be slow.
A more efficient approach would be to use a Set for constant-time lookups:
function getFriendPosts(allPosts, friends) {
const friendSet = new Set(friends);
return allPosts.filter(post => friendSet.has(post.author));
}
This improves the time complexity to O(n + m), which is much more scalable.
2. E-commerce Product Search
In an e-commerce platform, users often search for products. If you have a large catalog, a linear search through all products would be inefficient:
function findProduct(products, name) {
return products.find(product => product.name === name);
}
This has a time complexity of O(n), where n is the number of products.
Instead, you could use a hash table (object in JavaScript) to achieve O(1) lookup time:
function createProductIndex(products) {
const index = {};
for (let product of products) {
index[product.name] = product;
}
return index;
}
function findProduct(productIndex, name) {
return productIndex[name];
}
While this uses more memory, it drastically improves search time, especially for large catalogs.
Common Pitfalls and Misconceptions
As you work with Big O notation, be aware of these common pitfalls:
1. Focusing Only on Time Complexity
While time complexity is crucial, don’t forget about space complexity. Sometimes, an algorithm with better time complexity might use significantly more memory, which could be problematic in memory-constrained environments.
2. Ignoring Constants
Big O notation ignores constants, but in practice, they can matter. For small inputs, an O(n) algorithm might outperform an O(log n) algorithm if the constant factors are significantly different.
3. Worst-Case vs. Average-Case
Big O typically describes worst-case scenarios. However, average-case performance might be more relevant in some real-world applications. For example, QuickSort has a worst-case time complexity of O(n^2), but its average-case complexity is O(n log n), which is why it’s often used in practice.
4. Overcomplicating Solutions
Sometimes, in an attempt to optimize, developers might overcomplicate their code. A simpler O(n) solution might be preferable to a complex O(log n) solution, especially if n is typically small.
Advanced Big O Concepts
As you become more comfortable with basic Big O analysis, consider these advanced concepts:
1. Amortized Analysis
Some data structures, like dynamic arrays, have operations that occasionally take longer but are generally fast. Amortized analysis considers the average time taken over a sequence of operations. For example, while appending to a dynamic array occasionally requires resizing (O(n)), the amortized time complexity for append operations is O(1).
2. Multi-Variable Time Complexity
Sometimes, an algorithm’s performance depends on multiple input variables. For example, a graph algorithm might have a complexity of O(V + E), where V is the number of vertices and E is the number of edges.
3. Recursive Time Complexity
Analyzing the time complexity of recursive algorithms can be tricky. The master theorem is often used to analyze divide-and-conquer algorithms. For example, the time complexity of Merge Sort is derived using this theorem.
Preparing for Technical Interviews
Understanding Big O notation is crucial for technical interviews, especially at top tech companies. Here are some tips to help you prepare:
- Practice analyzing different algorithms: Go through common sorting, searching, and data structure algorithms and practice determining their time and space complexities.
- Solve coding problems with efficiency in mind: When solving coding challenges, always consider the efficiency of your solution and be prepared to discuss trade-offs.
- Learn to optimize: Practice taking a working solution and optimizing it for better time or space complexity.
- Verbalize your thought process: During interviews, explain your reasoning as you analyze the efficiency of your code.
- Know your data structures: Understanding the time complexities of operations on different data structures will help you choose the right tool for the job.
Conclusion
Big O notation is a fundamental concept in computer science and a crucial skill for any software developer. By understanding and applying these principles, you’ll be able to write more efficient code, optimize existing algorithms, and ace those technical interviews.
Remember, the goal isn’t always to achieve the lowest possible Big O complexity. Sometimes, readability, maintainability, or other factors might be more important. The key is to understand the trade-offs and make informed decisions based on your specific requirements.
Keep practicing, analyzing, and optimizing your code. With time and experience, thinking in terms of Big O will become second nature, making you a more effective and efficient programmer.
Happy coding, and may your algorithms always run in O(1) time!