The Tutorial-to-Application Pipeline: How to Transform What You Watch Into Code You Write
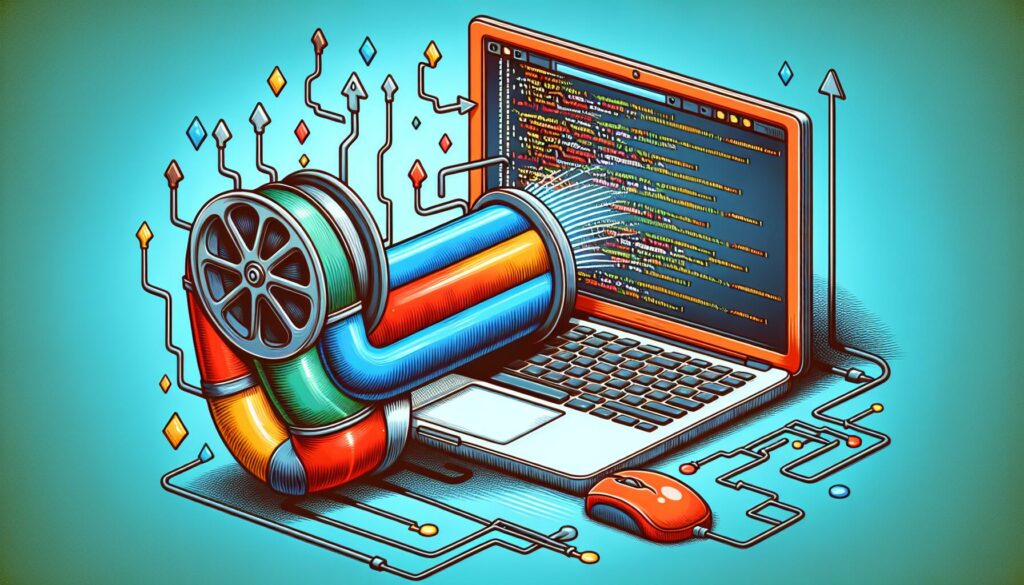
In the vast landscape of coding education, there’s a common challenge that many aspiring developers face: the gap between watching tutorials and actually writing code. This phenomenon, often referred to as the “tutorial purgatory,” can leave learners feeling stuck, overwhelmed, and unsure of how to progress. But fear not! In this comprehensive guide, we’ll explore the Tutorial-to-Application Pipeline, a strategic approach to transform the knowledge you gain from tutorials into practical, hands-on coding skills. Whether you’re a beginner just starting your coding journey or an intermediate learner looking to level up, this article will provide you with actionable strategies to bridge the gap between passive learning and active coding.
Understanding the Tutorial-to-Application Gap
Before we dive into the solutions, it’s essential to understand why this gap exists in the first place. There are several reasons why learners often struggle to transition from watching tutorials to writing their own code:
- Passive vs. Active Learning: Watching tutorials is largely a passive activity, while coding requires active engagement and problem-solving.
- Lack of Context: Tutorials often present isolated concepts or specific solutions, making it challenging to see the bigger picture.
- Overwhelming Information: The sheer volume of tutorials and resources available can lead to information overload and decision paralysis.
- Fear of Failure: The blank screen can be intimidating, and the fear of making mistakes can prevent learners from taking the first step.
- Tutorial Dependency: Relying too heavily on tutorials can create a false sense of understanding without developing independent problem-solving skills.
Recognizing these challenges is the first step toward overcoming them. Now, let’s explore the Tutorial-to-Application Pipeline and how it can help you transform your learning experience.
The Tutorial-to-Application Pipeline: A Step-by-Step Approach
The Tutorial-to-Application Pipeline is a structured process designed to help you move from passive consumption of tutorials to active creation of your own code. Here’s a breakdown of each step in the pipeline:
1. Active Watching: Engage with the Tutorial
The first step in the pipeline is to transform your tutorial-watching experience from passive to active. Here are some strategies to make your viewing more engaging and productive:
- Take Notes: Jot down key concepts, code snippets, and your own thoughts as you watch. This helps reinforce learning and creates a personal reference guide.
- Pause and Predict: Before the instructor reveals a solution, pause the video and try to predict what comes next. This engages your problem-solving skills and helps you think like a programmer.
- Code Along: Instead of just watching, open your code editor and type along with the instructor. This hands-on approach helps build muscle memory and familiarizes you with the coding environment.
- Ask Questions: Even if you’re watching a pre-recorded tutorial, write down questions that come to mind. You can research these later or ask in coding communities.
By actively engaging with tutorials, you’re laying the groundwork for better retention and understanding of the material.
2. Reflection and Synthesis: Connect the Dots
After watching a tutorial, take some time to reflect on what you’ve learned and how it fits into the bigger picture of programming. This step is crucial for developing a deeper understanding and preparing to apply your knowledge. Consider the following activities:
- Summarize the Key Points: In your own words, write a brief summary of the main concepts covered in the tutorial. This helps reinforce your understanding and identifies any areas that might need further clarification.
- Draw Connections: Think about how the new information relates to concepts you’ve learned previously. Creating mental links between different programming ideas helps build a more comprehensive understanding of coding.
- Identify Real-World Applications: Consider how the concepts from the tutorial could be applied to real-world problems or projects. This helps bridge the gap between theory and practice.
- Create a Mind Map: Visualize the relationships between different concepts by creating a mind map. This can help you see the bigger picture and how various programming ideas interconnect.
The reflection and synthesis stage helps solidify your learning and prepares you for the next, more hands-on steps in the pipeline.
3. Experimentation: Tinker with the Code
Now that you’ve actively engaged with the tutorial and reflected on its content, it’s time to start experimenting. This stage is all about playing with the code and exploring its possibilities. Here’s how to approach this step:
- Modify Existing Code: Take the code from the tutorial and start making small changes. What happens if you alter a variable? Can you add a new feature or functionality?
- Break Things on Purpose: Intentionally introduce errors into the code and try to fix them. This helps you understand how the code works and improves your debugging skills.
- Combine Concepts: Try to incorporate ideas from previous tutorials into the current project. This helps you see how different programming concepts can work together.
- Use Different Inputs: If the tutorial used specific data or inputs, try running the code with different values. This helps you understand the code’s behavior under various conditions.
Experimentation is a low-stakes way to gain hands-on experience and build confidence in your coding abilities. Don’t be afraid to make mistakes – they’re an essential part of the learning process!
4. Mini-Projects: Apply Knowledge to Small Tasks
After experimenting with the tutorial code, it’s time to take your first steps towards independent coding. Mini-projects are small, focused tasks that allow you to apply what you’ve learned in a more structured way. Here’s how to approach this stage:
- Set Clear Objectives: Define a small, achievable goal for your mini-project. It should be related to the concepts covered in the tutorial but different enough to require some independent problem-solving.
- Start from Scratch: Instead of modifying existing code, start with a blank file. This forces you to recall and apply the concepts you’ve learned.
- Use Pseudocode: Before diving into actual coding, write out the logic of your mini-project in plain language. This helps organize your thoughts and creates a roadmap for your code.
- Embrace the Challenge: You’ll likely encounter obstacles and moments of confusion. This is normal and an important part of the learning process. Use these challenges as opportunities to develop your problem-solving skills.
Here’s an example of how you might approach a mini-project after watching a tutorial on basic Python functions:
# Mini-Project: Temperature Converter
# Pseudocode:
# 1. Define a function to convert Celsius to Fahrenheit
# 2. Define a function to convert Fahrenheit to Celsius
# 3. Ask the user for input (temperature and unit)
# 4. Call the appropriate function based on the input
# 5. Display the result
def celsius_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
temperature = float(input("Enter the temperature: "))
unit = input("Enter the unit (C for Celsius, F for Fahrenheit): ").upper()
if unit == "C":
result = celsius_to_fahrenheit(temperature)
print(f"{temperature}°C is equal to {result:.2f}°F")
elif unit == "F":
result = fahrenheit_to_celsius(temperature)
print(f"{temperature}°F is equal to {result:.2f}°C")
else:
print("Invalid unit. Please enter C or F.")
This mini-project applies the concept of functions learned in the tutorial to create a practical temperature converter. It also introduces new elements like user input and conditional statements, encouraging you to expand your knowledge.
5. Problem-Solving: Tackle Coding Challenges
As you gain confidence through mini-projects, it’s time to challenge yourself with more complex problems. Coding challenges and algorithmic problems are excellent ways to hone your skills and prepare for real-world programming scenarios. Here’s how to approach this stage:
- Start with Simple Problems: Begin with easy coding challenges and gradually increase the difficulty. Platforms like LeetCode, HackerRank, or AlgoCademy offer a wide range of problems sorted by difficulty.
- Focus on Problem-Solving Process: Don’t just aim for the correct answer. Pay attention to how you approach the problem, break it down, and develop a solution.
- Practice Regularly: Set aside time each day or week to work on coding challenges. Consistent practice is key to improving your skills.
- Analyze Multiple Solutions: After solving a problem, look at other people’s solutions. This exposes you to different approaches and coding styles.
- Implement Time and Space Complexity: As you progress, start considering the efficiency of your solutions in terms of time and space complexity.
Here’s an example of how you might approach a common coding challenge:
# Problem: Given an array of integers, return indices of the two numbers
# such that they add up to a specific target.
def two_sum(nums, target):
# Create a dictionary to store complements
complement_dict = {}
# Iterate through the array
for i, num in enumerate(nums):
complement = target - num
# If the complement exists in the dictionary, return the indices
if complement in complement_dict:
return [complement_dict[complement], i]
# Otherwise, add the current number and its index to the dictionary
complement_dict[num] = i
# If no solution is found, return an empty list
return []
# Test the function
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(f"Indices of the two numbers that add up to {target}: {result}")
This problem-solving exercise applies concepts like array manipulation, dictionary usage, and efficient algorithm design. It’s a step up from the mini-projects and helps develop more advanced programming skills.
6. Personal Projects: Build Something Meaningful
The final stage of the Tutorial-to-Application Pipeline is to embark on personal projects. This is where you truly transition from a tutorial follower to an independent developer. Personal projects allow you to apply everything you’ve learned in a real-world context and create something meaningful. Here’s how to approach this stage:
- Choose a Project You’re Passionate About: Select an idea that excites you and solves a problem you care about. This motivation will help you push through challenges.
- Start Small and Iterate: Begin with a minimal viable product (MVP) and gradually add features. This approach helps prevent overwhelm and allows you to see progress quickly.
- Plan Before You Code: Outline the project’s structure, features, and potential challenges before you start coding. This planning phase can save you time and frustration later.
- Embrace New Technologies: Use your project as an opportunity to learn new tools, libraries, or frameworks that are relevant to your goals.
- Seek Feedback: Share your project with others, whether it’s peers, mentors, or online communities. Their feedback can provide valuable insights and improvement suggestions.
- Document Your Process: Keep a development log or blog about your project. This helps reinforce your learning and can be valuable for future reference or even for your portfolio.
Here’s a high-level example of how you might start planning a personal project:
# Personal Project: Book Recommendation System
# Project Outline:
# 1. Data Collection:
# - Scrape book information from Goodreads or use an existing dataset
# - Store data in a database (e.g., SQLite)
# 2. Data Processing:
# - Clean and preprocess the collected data
# - Extract relevant features (e.g., genre, author, rating)
# 3. Recommendation Algorithm:
# - Implement a content-based filtering algorithm
# - Use cosine similarity to find books with similar features
# 4. User Interface:
# - Create a simple web interface using Flask
# - Allow users to input their favorite books
# - Display recommended books based on user input
# 5. Deployment:
# - Deploy the application on a cloud platform (e.g., Heroku)
# Next Steps:
# - Research and choose appropriate libraries (e.g., pandas, scikit-learn, Flask)
# - Set up a GitHub repository for version control
# - Break down each component into smaller, manageable tasks
# - Start with data collection and gradually build up the system
This project outline demonstrates how you can apply various programming concepts and technologies to create a practical application. It incorporates data processing, machine learning, web development, and deployment – all skills that are valuable in real-world software development.
Overcoming Common Challenges in the Tutorial-to-Application Pipeline
As you work through the Tutorial-to-Application Pipeline, you’re likely to encounter some challenges. Here are some common obstacles and strategies to overcome them:
1. Tutorial Dependency
Challenge: Feeling lost without step-by-step guidance.
Solution: Gradually reduce your reliance on tutorials. Start by using them as a reference rather than a strict guide. As you progress, challenge yourself to solve problems before looking up solutions.
2. Imposter Syndrome
Challenge: Feeling like you’re not a “real” programmer or that you’re not good enough.
Solution: Remember that every developer started as a beginner. Focus on your progress rather than comparing yourself to others. Celebrate small victories and keep a record of your achievements to boost confidence.
3. Analysis Paralysis
Challenge: Getting stuck in the planning phase, unable to start coding due to overthinking.
Solution: Embrace the “just start” mentality. It’s okay if your first attempt isn’t perfect. You can always refactor and improve your code later. The important thing is to begin writing code.
4. Scope Creep
Challenge: Projects becoming too large and overwhelming.
Solution: Practice breaking down large projects into smaller, manageable tasks. Use project management tools or a simple to-do list to keep track of your progress and maintain focus.
5. Knowledge Gaps
Challenge: Encountering concepts or technologies you don’t understand.
Solution: Embrace these moments as learning opportunities. Use resources like documentation, Stack Overflow, or coding communities to fill in your knowledge gaps. Don’t be afraid to ask for help when needed.
Leveraging Tools and Resources
To maximize your success with the Tutorial-to-Application Pipeline, take advantage of the following tools and resources:
- Version Control: Use Git and GitHub to track changes in your code and collaborate with others.
- Integrated Development Environments (IDEs): Tools like Visual Studio Code, PyCharm, or IntelliJ IDEA can significantly enhance your coding experience with features like code completion and debugging tools.
- Online Coding Platforms: Websites like AlgoCademy, LeetCode, or HackerRank offer a wide range of coding challenges and tutorials to practice your skills.
- Documentation: Get comfortable reading official documentation for languages and libraries you’re using. It’s an essential skill for any developer.
- Coding Communities: Join platforms like Stack Overflow, Reddit’s programming subreddits, or Discord coding servers to connect with other learners and experienced developers.
- Blogs and Podcasts: Follow coding blogs and listen to programming podcasts to stay updated on industry trends and continue learning.
Conclusion: Embracing the Journey from Viewer to Creator
The transition from watching coding tutorials to writing your own code is a significant milestone in every programmer’s journey. The Tutorial-to-Application Pipeline provides a structured approach to bridge this gap, helping you transform from a passive consumer of coding content to an active creator of software.
Remember that this process is not linear, and it’s okay to move back and forth between the stages as needed. The key is to maintain a balance between learning new concepts and applying what you’ve learned. Embrace the challenges, celebrate your progress, and don’t be afraid to make mistakes – they’re an essential part of the learning process.
As you progress through the pipeline, you’ll not only improve your coding skills but also develop crucial problem-solving abilities, gain confidence in your capabilities, and cultivate a mindset of continuous learning. These qualities are invaluable in the ever-evolving field of software development.
So, the next time you watch a coding tutorial, remember that it’s just the beginning. Use the Tutorial-to-Application Pipeline to guide your learning journey, and soon you’ll find yourself not just understanding code, but creating innovative solutions and bringing your own ideas to life through programming.
Happy coding, and may your journey from viewer to creator be filled with exciting discoveries, rewarding challenges, and the joy of building something uniquely yours!