The Streetfighter’s Guide to Coding Interviews: Combos, Special Moves, and Finishing Techniques
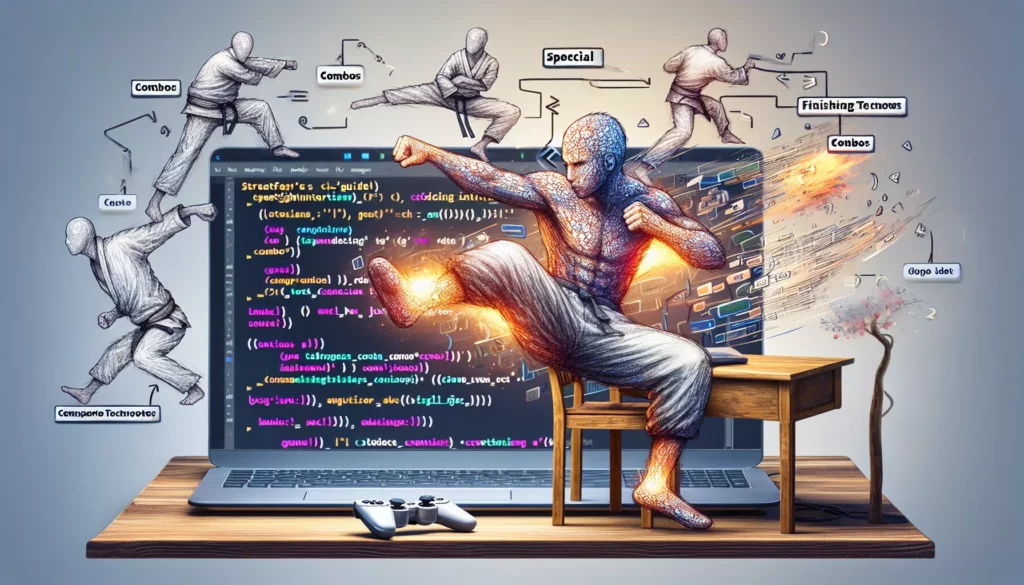
In the high-stakes arena of coding interviews, success often feels like winning a fierce battle in a fighting game. Just as a streetfighter must master combos, special moves, and finishing techniques to emerge victorious, aspiring developers need to arm themselves with a powerful arsenal of coding skills and strategies. This comprehensive guide will transform you from a button-mashing novice into a coding interview champion, ready to face even the toughest FAANG (Facebook, Amazon, Apple, Netflix, Google) opponents.
Round 1: Understanding the Battlefield
Before you can start throwing punches (or writing code), it’s crucial to understand the landscape of coding interviews. These technical assessments are designed to evaluate your problem-solving skills, coding proficiency, and ability to think on your feet. Like different fighting game stages, each company may have its unique interview style, but they all share common elements:
- Algorithmic problem-solving
- Data structure manipulation
- System design questions
- Behavioral interviews
Your goal is to prove that you’re not just a one-trick pony, but a well-rounded fighter capable of adapting to any challenge thrown your way.
Round 2: Training Your Coding Muscles
Just as a streetfighter spends countless hours in the dojo perfecting their moves, you need to dedicate time to honing your coding skills. Here’s how to build your strength:
1. Master the Fundamentals
Before attempting flashy combos, ensure you have a solid grasp of the basics. This includes:
- Core programming concepts (variables, loops, conditionals)
- Object-oriented programming principles
- Time and space complexity analysis
- Basic data structures (arrays, linked lists, stacks, queues)
These are your light punches and kicks – simple, but essential for building more complex techniques.
2. Level Up Your Data Structures
As you progress, you’ll need to master more advanced data structures. These are your special moves:
- Trees (binary trees, binary search trees, balanced trees)
- Graphs (directed, undirected, weighted)
- Hash tables
- Heaps
- Tries
Understanding when and how to use these structures efficiently can give you a significant advantage in solving complex problems.
3. Algorithm Dojo
Algorithms are the combos of the coding world. Practice implementing and analyzing these common algorithms:
- Sorting (quicksort, mergesort, heapsort)
- Searching (binary search, depth-first search, breadth-first search)
- Dynamic programming
- Greedy algorithms
- Divide and conquer
Remember, it’s not just about memorizing these algorithms, but understanding their underlying principles and when to apply them.
Round 3: Mastering Combos – Problem-Solving Techniques
In a streetfight, stringing together the right combination of moves can lead to devastating effects. Similarly, in coding interviews, combining different problem-solving techniques can help you tackle even the most challenging questions. Here are some powerful combos to add to your repertoire:
1. The Two-Pointer Technique
This technique is particularly useful for array and string problems. It involves using two pointers that either move towards each other or in the same direction to solve the problem efficiently.
Example problem: Given a sorted array, remove duplicates in-place.
def remove_duplicates(nums):
if not nums:
return 0
# Initialize two pointers
slow = 0
# Move fast pointer through the array
for fast in range(1, len(nums)):
if nums[fast] != nums[slow]:
slow += 1
nums[slow] = nums[fast]
return slow + 1
# Test the function
arr = [1, 1, 2, 2, 3, 4, 4, 5]
new_length = remove_duplicates(arr)
print(f"Array after removing duplicates: {arr[:new_length]}")
print(f"New length: {new_length}")
2. The Sliding Window Technique
This technique is excellent for solving substring or subarray problems with a specific condition. It involves maintaining a “window” that expands or contracts based on certain criteria.
Example problem: Find the longest substring with at most k distinct characters.
from collections import defaultdict
def longest_substring_with_k_distinct(s, k):
char_count = defaultdict(int)
max_length = 0
start = 0
for end in range(len(s)):
char_count[s[end]] += 1
while len(char_count) > k:
char_count[s[start]] -= 1
if char_count[s[start]] == 0:
del char_count[s[start]]
start += 1
max_length = max(max_length, end - start + 1)
return max_length
# Test the function
s = "aabacbebebe"
k = 3
result = longest_substring_with_k_distinct(s, k)
print(f"Longest substring with at most {k} distinct characters: {result}")
3. The Divide and Conquer Combo
This powerful technique involves breaking down a problem into smaller subproblems, solving them independently, and then combining the results. It’s particularly useful for problems involving arrays, trees, and graphs.
Example problem: Implement the Merge Sort algorithm.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Test the function
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(f"Sorted array: {sorted_arr}")
Round 4: Special Moves – Advanced Techniques
Every great fighter has a set of special moves that can turn the tide of battle. In the coding interview world, these are the advanced techniques that can help you solve particularly tricky problems or optimize your solutions.
1. The Bit Manipulation Hadouken
Bit manipulation can lead to highly efficient solutions for certain problems. Mastering bitwise operations can give you an edge in scenarios involving binary numbers, flags, or optimization.
Example: Count the number of set bits in an integer.
def count_set_bits(n):
count = 0
while n:
count += n & 1
n >>= 1
return count
# Test the function
num = 13 # Binary: 1101
result = count_set_bits(num)
print(f"Number of set bits in {num}: {result}")
2. The Dynamic Programming Dragon Punch
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into simpler subproblems. It’s particularly useful for problems involving finding the maximum, minimum, or optimal solution.
Example: Solve the classic “Climbing Stairs” problem.
def climb_stairs(n):
if n <= 2:
return n
dp = [0] * (n + 1)
dp[1] = 1
dp[2] = 2
for i in range(3, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
n = 5
ways = climb_stairs(n)
print(f"Number of ways to climb {n} stairs: {ways}")
3. The Graph Traversal Spinning Bird Kick
Many complex problems can be modeled as graphs. Mastering graph traversal algorithms like Depth-First Search (DFS) and Breadth-First Search (BFS) is crucial for solving these types of problems efficiently.
Example: Implement DFS to detect a cycle in a directed graph.
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def is_cyclic_util(self, v, visited, rec_stack):
visited[v] = True
rec_stack[v] = True
for neighbor in self.graph[v]:
if not visited[neighbor]:
if self.is_cyclic_util(neighbor, visited, rec_stack):
return True
elif rec_stack[neighbor]:
return True
rec_stack[v] = False
return False
def is_cyclic(self):
visited = {v: False for v in self.graph}
rec_stack = {v: False for v in self.graph}
for node in self.graph:
if not visited[node]:
if self.is_cyclic_util(node, visited, rec_stack):
return True
return False
# Test the function
g = Graph()
g.add_edge(0, 1)
g.add_edge(1, 2)
g.add_edge(2, 3)
g.add_edge(3, 1) # This edge creates a cycle
result = g.is_cyclic()
print(f"Does the graph contain a cycle? {result}")
Round 5: Finishing Moves – System Design and Behavioral Questions
To truly excel in coding interviews, especially at FAANG companies, you need to master the art of system design and nail the behavioral questions. These are your finishing moves – the techniques that will set you apart from other candidates and clinch your victory.
1. The System Design Shoryuken
System design questions test your ability to architect large-scale distributed systems. To excel in this area:
- Understand key concepts: scalability, load balancing, caching, database sharding
- Practice designing popular systems: URL shortener, social media feed, chat application
- Learn to make appropriate trade-offs based on requirements
Example approach for designing a URL shortener:
- Clarify requirements (e.g., scale, features like custom URLs)
- Estimate scale (e.g., requests per second, storage needs)
- Design the high-level system components:
- API Gateway
- Application servers
- Database (consider NoSQL for scalability)
- Cache (e.g., Redis for frequently accessed URLs)
- Discuss the URL shortening algorithm (e.g., base62 encoding)
- Address scalability concerns (e.g., database sharding, load balancing)
- Consider additional features (e.g., analytics, rate limiting)
2. The Behavioral Interview Sonic Boom
Behavioral questions assess your soft skills, problem-solving approach, and cultural fit. To prepare:
- Use the STAR method (Situation, Task, Action, Result) to structure your responses
- Prepare stories that highlight your strengths and align with the company’s values
- Practice common questions like “Tell me about a time you faced a challenge at work”
Example STAR response:
Question: “Describe a situation where you had to work with a difficult team member.”
Answer:
- Situation: During a critical project at my previous job, I was paired with a team member who consistently missed deadlines and communicated poorly.
- Task: My goal was to ensure the project’s success while improving our working relationship.
- Action: I initiated a one-on-one meeting to understand their challenges. We agreed on a more structured communication plan and I offered to help them with time management techniques.
- Result: Our improved collaboration led to the project being completed on time. The team member’s performance improved, and they thanked me for my support.
Final Round: Training Regimen and Resources
To become a true coding interview champion, you need a structured training regimen. Here’s a plan to help you level up your skills:
1. Daily Coding Practice
- Solve at least one coding problem every day
- Use platforms like LeetCode, HackerRank, or AlgoCademy for a variety of problems
- Focus on different topics each week (e.g., arrays, strings, trees, graphs)
2. Mock Interviews
- Practice with a friend or use services like Pramp for realistic interview experiences
- Time yourself to simulate real interview conditions
- Get feedback on your problem-solving approach and communication skills
3. System Design Practice
- Read books like “Designing Data-Intensive Applications” by Martin Kleppmann
- Watch system design videos on YouTube (e.g., Gaurav Sen, Success in Tech)
- Practice designing popular systems and discuss your solutions with peers
4. Behavioral Interview Preparation
- Reflect on your past experiences and prepare relevant stories
- Practice answering common behavioral questions out loud
- Research the company’s values and culture to tailor your responses
5. Continuous Learning
- Stay updated with the latest technologies and industry trends
- Contribute to open-source projects to gain real-world experience
- Attend coding workshops or hackathons to challenge yourself
The Final Showdown: Interview Day
As you step into the interview arena, remember these final tips to emerge victorious:
- Warm-up: Do a few easy coding problems to get your mind in the right state.
- Stay calm: Take deep breaths and remember your training.
- Communicate: Explain your thought process clearly as you solve problems.
- Ask questions: Clarify any ambiguities in the problem statement.
- Test your code: Always run through test cases, including edge cases.
- Be adaptable: If you’re stuck, be open to hints and be ready to pivot your approach.
- Show enthusiasm: Demonstrate your passion for coding and the company’s mission.
Remember, like any great fighter, the key to success in coding interviews is consistent practice, adaptability, and the confidence that comes from thorough preparation. With this streetfighter’s guide, you’re now equipped with the combos, special moves, and finishing techniques needed to take on even the toughest FAANG opponents. Step into the ring with confidence, and may your code be bug-free and your algorithms optimal. It’s time to ace that interview and land your dream job. Game on!