The Stand-Up Comedian’s Approach to Technical Interviews: Timing, Delivery, and Punchlines
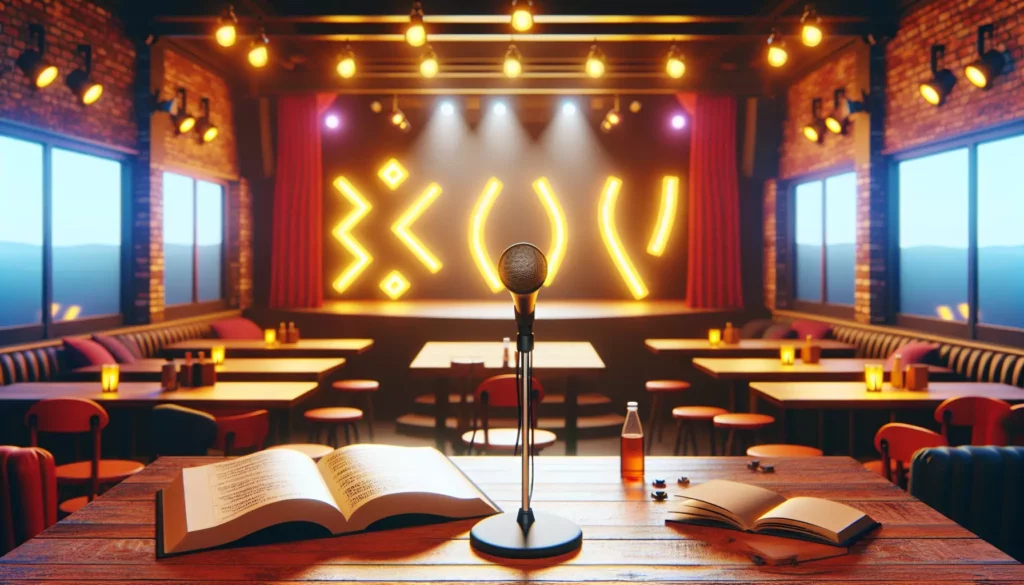
Picture this: you’re standing in front of a room full of people, palms sweaty, knees weak, arms heavy. No, it’s not your debut at the local comedy club – it’s your technical interview at a FAANG company. But what if I told you that the skills that make a great stand-up comedian could also make you a star in your next coding interview? Buckle up, aspiring devs, because we’re about to take a hilarious journey through the world of technical interviews, comedian-style!
Setting the Stage: The Similarities Between Comedy and Coding
At first glance, stand-up comedy and coding might seem as different as a knock-knock joke and a recursive algorithm. But dig a little deeper, and you’ll find some surprising parallels:
- Timing is everything: In comedy, it’s all about the pause before the punchline. In coding, it’s about optimizing your algorithm for the best time complexity.
- Structure matters: A good joke has a setup and a payoff. A good piece of code has a clear structure and a satisfying output.
- Practice makes perfect: Comedians rehearse their routines tirelessly. Coders? They solve problem after problem on platforms like AlgoCademy.
- Thinking on your feet: Improv skills help comedians handle hecklers. For coders, it’s all about adapting to unexpected edge cases.
Now that we’ve set the stage, let’s dive into how you can use a comedian’s toolkit to ace your next technical interview!
1. Know Your Audience: Reading the Room
Every good comedian knows that different crowds respond to different types of humor. Similarly, different interviewers and companies have their own cultures and expectations. Before you step into that interview room (or log into that Zoom call), do your homework:
- Research the company’s values and culture
- Look up your interviewer on LinkedIn (in a non-creepy way, of course)
- Understand the role you’re applying for and tailor your responses accordingly
Just as a comedian might adjust their material for a corporate gig versus a late-night club show, you should be prepared to shift your approach based on your audience. Maybe that means emphasizing your teamwork skills for a collaborative company culture, or focusing on your innovative problem-solving for a startup that values creativity.
2. Master Your Opening: The First Impression
A comedian’s opening line can make or break their set. In a technical interview, your introduction and how you approach the first problem can set the tone for the entire session. Here’s how to nail your opening:
- Start with confidence: Even if you’re nervous, project confidence. Stand-up comedians often use power poses before going on stage – try it before your interview!
- Break the ice: A light, professional comment can help ease tension. Just maybe avoid the classic “Why did the programmer quit his job? He didn’t get arrays!” (Unless you’re absolutely sure your interviewer will appreciate it.)
- Listen actively: Pay close attention to the problem statement. Good comedians are great listeners, picking up on audience cues. In an interview, understanding the nuances of the question is crucial.
Here’s an example of how you might approach the opening of a technical interview:
Interviewer: "Hello! How are you doing today?"
You: "Great, thank you! I'm excited to be here. I've been looking forward to this opportunity to discuss technology and problem-solving with you. Should we dive into the first question?"
Interviewer: "Sounds good. Let's start with a classic: Can you implement a function to reverse a string?"
You: "Absolutely! Before I start coding, would it be alright if I ask a few clarifying questions about the problem?"
This opening shows enthusiasm, professionalism, and a proactive approach to problem-solving – all great first impressions!
3. Timing is Everything: Pacing Your Problem-Solving
In comedy, timing can be the difference between a laugh and crickets. In coding interviews, it’s about managing your time effectively and knowing when to move on or ask for help. Here are some tips:
- Start with the brute force: Like a comedian testing new material, start with a straightforward solution. It shows you can approach the problem, even if it’s not optimal.
- Improve incrementally: Comedians refine their jokes over time. Similarly, iterate on your solution, explaining your thought process as you go.
- Know when to “wrap it up”: If you’re stuck, don’t spend the entire interview on one problem. Ask for a hint or move on if suggested.
Let’s see this in action with a common interview problem: finding the maximum subarray sum.
def max_subarray_sum(arr):
# Brute force approach
max_sum = float('-inf')
for i in range(len(arr)):
current_sum = 0
for j in range(i, len(arr)):
current_sum += arr[j]
max_sum = max(max_sum, current_sum)
return max_sum
# Improved solution (Kadane's algorithm)
def improved_max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
In this example, you’d start by explaining the brute force approach, then move on to the more efficient Kadane’s algorithm, discussing the time complexity improvement from O(n^2) to O(n).
4. Delivery is Key: Communicating Your Thoughts
A comedian’s delivery can elevate mediocre material or sabotage great jokes. In a technical interview, how you communicate your problem-solving process is just as important as solving the problem itself. Here’s how to deliver a stellar performance:
- Think out loud: Verbalize your thought process. This gives the interviewer insight into how you approach problems.
- Use analogies: Just as comedians use relatable situations to explain complex ideas, use analogies to clarify your coding concepts.
- Handle “hecklers” gracefully: If the interviewer challenges your approach, don’t get defensive. Treat it as an opportunity to demonstrate your ability to consider different perspectives.
Let’s see how this might play out in an interview:
Interviewer: "Can you implement a function to check if a binary tree is balanced?"
You: "Certainly! Let's think about this step by step. A balanced binary tree is one where the heights of the left and right subtrees of every node differ by no more than one. It's like a well-organized bookshelf where no stack of books is significantly taller than the others.
First, I'll define a helper function to calculate the height of a subtree:
def height(node):
if not node:
return 0
return 1 + max(height(node.left), height(node.right))
Now, for the main function:
def is_balanced(root):
if not root:
return True
left_height = height(root.left)
right_height = height(root.right)
if abs(left_height - right_height) > 1:
return False
return is_balanced(root.left) and is_balanced(root.right)
This solution recursively checks each node. It's like a librarian checking each shelf to ensure the books are evenly distributed. The time complexity would be O(n^2) in the worst case, where n is the number of nodes. We're potentially calculating the height for each node multiple times.
We could optimize this by calculating the height and checking balance in a single pass, returning -1 for unbalanced subtrees. Would you like me to implement that approach as well?"
Interviewer: "That's a great explanation! Yes, please go ahead with the optimized version."
This response demonstrates clear communication, use of analogies, and proactive suggestion of optimizations – all key elements of a strong interview performance.
5. Handle the Unexpected: Improv Skills for Curveball Questions
Even the most prepared comedian can face unexpected situations – a joke falls flat, or there’s a surprise interruption. Similarly, interviewers often throw curveball questions to see how you handle unfamiliar territory. Here’s how to improvise like a pro:
- Stay calm: Take a deep breath. Remember, it’s okay not to have an immediate answer.
- Break it down: Approach unfamiliar problems by relating them to concepts you do know.
- Ask questions: Seeking clarification shows you’re thoughtful and thorough.
- Think aloud: Even if you’re unsure, sharing your thought process can impress interviewers.
Let’s look at how you might handle an unexpected question:
Interviewer: "How would you design a system to detect if two rectangles on a 2D plane overlap?"
You: "Interesting question! I haven't encountered this exact problem before, but let's break it down. We're essentially dealing with geometric relationships in a 2D space.
First, let's define what we know:
1. A rectangle can be represented by its top-left and bottom-right coordinates.
2. Two rectangles overlap if there's any area shared between them.
Now, let's think about conditions where rectangles wouldn't overlap:
1. If one rectangle is entirely to the left of the other.
2. If one rectangle is entirely to the right of the other.
3. If one rectangle is entirely above the other.
4. If one rectangle is entirely below the other.
If none of these conditions are true, the rectangles must overlap.
Let's implement this logic:
def rectangles_overlap(rect1, rect2):
# rect1 and rect2 are tuples: (x1, y1, x2, y2)
# where (x1, y1) is top-left and (x2, y2) is bottom-right
# Check if one rectangle is on left side of other
if rect1[2] < rect2[0] or rect2[2] < rect1[0]:
return False
# Check if one rectangle is above other
if rect1[1] > rect2[3] or rect2[1] > rect1[3]:
return False
return True
This solution has a time complexity of O(1) since we're just doing constant-time comparisons.
Would you like me to explain any part of this in more detail or consider any edge cases?"
Interviewer: "That's a great approach! Can you think of any potential issues with floating-point precision in this context?"
You: "Absolutely, that's an important consideration. When dealing with floating-point numbers in geometric calculations, we can run into precision issues due to how computers represent these numbers.
For example, two rectangles that just barely touch at an edge might be incorrectly identified as overlapping or non-overlapping due to tiny rounding errors.
To mitigate this, we could introduce a small epsilon value for our comparisons:
def rectangles_overlap(rect1, rect2, epsilon=1e-9):
if rect1[2] + epsilon < rect2[0] or rect2[2] + epsilon < rect1[0]:
return False
if rect1[1] > rect2[3] + epsilon or rect2[1] > rect1[3] + epsilon:
return False
return True
This epsilon approach allows for a small margin of error in our calculations, making our function more robust against floating-point precision issues.
In a real-world scenario, the choice of epsilon would depend on the scale and precision requirements of the specific application."
This response demonstrates the ability to approach an unfamiliar problem methodically, communicate clearly, and even anticipate and address potential issues – all valuable skills in a technical interview.
6. Crafting Your Punchline: Optimizing Your Solution
In comedy, the punchline is what ties everything together and (hopefully) gets the big laugh. In coding interviews, your “punchline” is often the optimized solution you arrive at after discussing various approaches. Here’s how to make your coding punchline land:
- Start broad, then narrow down: Begin with a general approach, then refine it. This shows your problem-solving process.
- Explain trade-offs: Discuss the pros and cons of different solutions, demonstrating your analytical skills.
- Optimize incrementally: Show how you can improve your solution step by step, explaining the benefits of each optimization.
- Consider space-time trade-offs: Sometimes, using more memory can significantly speed up an algorithm. Explain these choices.
Let’s see this in action with a classic interview question: finding the kth largest element in an unsorted array.
def find_kth_largest(nums, k):
# Initial approach: Sort the array
# Time complexity: O(n log n), Space complexity: O(1)
nums.sort(reverse=True)
return nums[k-1]
# Optimized approach using QuickSelect algorithm
import random
def optimized_find_kth_largest(nums, k):
# Time complexity: O(n) average case, O(n^2) worst case
# Space complexity: O(1)
k = len(nums) - k
def quickselect(l, r):
pivot = random.randint(l, r)
nums[pivot], nums[r] = nums[r], nums[pivot]
store_index = l
for i in range(l, r):
if nums[i] <= nums[r]:
nums[store_index], nums[i] = nums[i], nums[store_index]
store_index += 1
nums[r], nums[store_index] = nums[store_index], nums[r]
if store_index == k:
return nums[k]
elif store_index < k:
return quickselect(store_index + 1, r)
else:
return quickselect(l, store_index - 1)
return quickselect(0, len(nums) - 1)
# Usage
nums = [3,2,1,5,6,4]
k = 2
print(optimized_find_kth_largest(nums, k)) # Output: 5
Here’s how you might explain this optimization:
“I started with a straightforward approach of sorting the array and returning the kth element from the end. This solution has a time complexity of O(n log n) due to the sorting step.
However, we can optimize this using the QuickSelect algorithm, which is based on the partitioning idea of QuickSort. This approach has an average time complexity of O(n), although the worst-case can still be O(n^2).
The key idea is that we don’t need to fully sort the array – we just need to find the correct position for the kth largest element. We randomly select a pivot, partition the array around it, and then recursively apply the algorithm to the appropriate partition.
This optimization significantly improves our average-case time complexity from O(n log n) to O(n), at the cost of a slightly more complex implementation. The space complexity remains O(1) as we’re doing the partitioning in-place.
In practice, this algorithm performs very well for large datasets where we need to find the kth largest (or smallest) element frequently.”
7. The Callback: Handling Follow-up Questions
Just as a comedian might have a callback to an earlier joke, interviewers often have follow-up questions to probe deeper into your understanding. Here’s how to handle them like a pro:
- Stay composed: Treat follow-ups as opportunities to showcase more of your knowledge, not as criticisms.
- Build on your previous answers: Reference your earlier solutions or explanations to show continuity in your thinking.
- Be honest about limitations: If you’re unsure, it’s okay to say so. Propose how you’d find the answer in a real work situation.
- Think out loud: Even if you’re not immediately sure of the answer, sharing your thought process can be valuable.
Let’s see how this might play out:
Interviewer: "Great job on implementing the QuickSelect algorithm. How would you modify this to find the median of an array?"
You: "Thank you! That's an interesting extension of the problem. Let's think about this step by step:
1. First, recall that the median is the middle value in a sorted array.
2. If the array has an odd number of elements, the median is the (n+1)/2 th element.
3. If the array has an even number of elements, the median is the average of the n/2 th and (n/2)+1 th elements.
We can modify our QuickSelect algorithm to find the median:
def find_median(nums):
n = len(nums)
if n % 2 == 1:
# Odd number of elements
return optimized_find_kth_largest(nums, (n+1)//2)
else:
# Even number of elements
left = optimized_find_kth_largest(nums, n//2)
right = optimized_find_kth_largest(nums, n//2 + 1)
return (left + right) / 2
This solution maintains the average O(n) time complexity of QuickSelect. However, in the even case, we're calling QuickSelect twice, which could be inefficient for large arrays.
An optimization could be to modify QuickSelect to find both elements in a single pass for even-length arrays, but that would increase the complexity of the implementation.
Would you like me to elaborate on any part of this solution?"
Interviewer: "That's a good approach. Can you think of any scenarios where this might not be the best solution?"
You: "Certainly! There are a few scenarios where this approach might not be ideal:
1. Streaming data: If we're dealing with a stream of numbers and need to continuously calculate the median, this approach wouldn't work well as it requires having all the data at once.
2. Very large datasets: While O(n) is generally good, for extremely large datasets that don't fit in memory, we might need a different approach.
3. Frequent updates: If the dataset is frequently updated and we need to recalculate the median often, this method would be inefficient as it requires reprocessing the entire dataset each time.
In these cases, other data structures like heaps (specifically, a max heap and a min heap) could be more appropriate. They allow for efficient insertions and deletions while maintaining the ability to quickly access the median.
For example, we could maintain two heaps: a max heap for the lower half of the numbers and a min heap for the upper half. This would allow us to find the median in O(1) time and insert/delete in O(log n) time, which could be more suitable for dynamic datasets.
Would you like me to sketch out how this heap-based solution might look?"
This response demonstrates the ability to build on previous answers, consider limitations of the proposed solution, and suggest alternative approaches for different scenarios – all valuable skills in a technical interview.
Conclusion: Bringing Down the House (or at Least Impressing the Interviewer)
Just as a stand-up comedian leaves their audience in stitches, your goal in a technical interview is to leave your interviewer impressed with your problem-solving skills, communication ability, and technical knowledge. By applying these comedian-inspired techniques, you can transform your interview from a nerve-wracking experience into a compelling performance:
- Know your audience and tailor your approach accordingly
- Start strong with a confident introduction
- Manage your timing, knowing when to move on or ask for help
- Deliver your solutions with clear communication and relatable analogies
- Handle unexpected questions with grace and creativity
- Build up to your optimized solution like a well-crafted punchline
- Be prepared for follow-up questions, treating them as opportunities to shine
Remember, just like in comedy, practice is key. Use platforms like AlgoCademy to hone your skills, work through countless problems, and get comfortable explaining your thought process. With time and effort, you’ll be ready to nail your technical interview and bring down the house – or at least secure that dream job offer!
Break a leg out there, code comics!