The Silent Coder: Improving Problem-Solving by Explaining Solutions Without Words
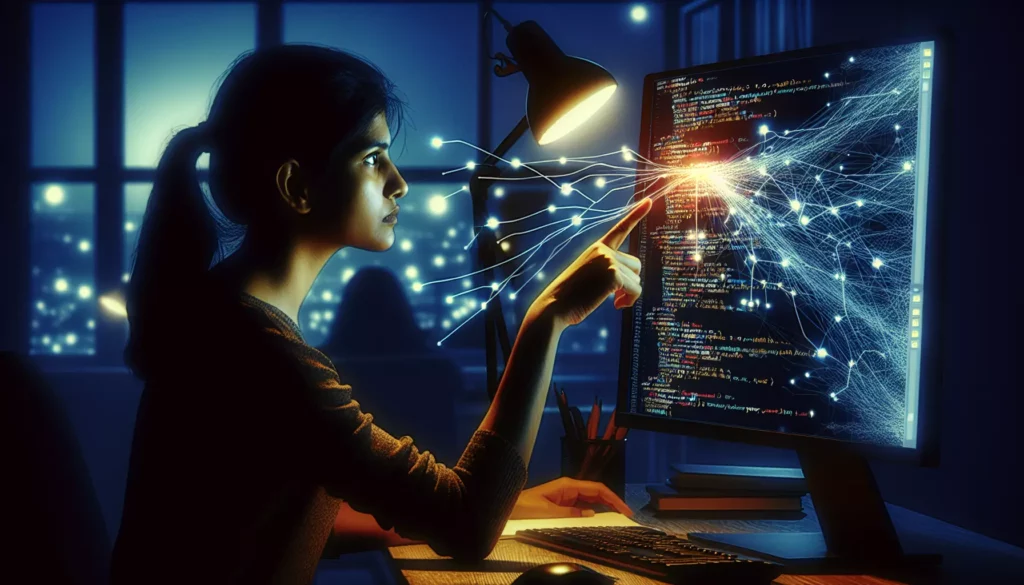
In the world of coding and software development, the ability to effectively communicate solutions is just as crucial as the ability to write them. However, a unique approach is gaining traction among developers and educators alike: explaining coding solutions without using words. This method, often referred to as “silent coding” or “wordless explanations,” is revolutionizing the way we think about problem-solving and knowledge transfer in the programming community.
The Power of Silent Coding
Silent coding is not about working in isolation or refraining from collaboration. Instead, it’s a technique that challenges developers to explain their solutions using only code, comments, and visual aids. This approach offers several benefits:
- Enhances clarity of thought
- Improves code readability
- Transcends language barriers
- Fosters deeper understanding of concepts
- Encourages more efficient problem-solving
By removing the crutch of verbal explanations, developers are forced to make their code speak for itself, resulting in cleaner, more intuitive solutions.
Techniques for Wordless Explanations
There are several effective techniques that developers can use to explain their solutions without relying on words:
1. Descriptive Variable Naming
One of the most powerful tools in a silent coder’s arsenal is the use of descriptive variable names. By choosing names that clearly convey the purpose and content of variables, developers can make their code self-explanatory. For example:
// Less descriptive
int x = 5;
int y = 10;
int z = x + y;
// More descriptive
int numberOfApples = 5;
int numberOfOranges = 10;
int totalFruits = numberOfApples + numberOfOranges;
In the second example, anyone reading the code can immediately understand what each variable represents without the need for additional explanation.
2. Meaningful Function Names
Similar to descriptive variable naming, using clear and meaningful function names can significantly improve code readability. Functions should be named to describe what they do, not how they do it. For instance:
// Less descriptive
function calc(a, b) {
return a * b;
}
// More descriptive
function calculateRectangleArea(length, width) {
return length * width;
}
The second function name immediately conveys its purpose, making the code more self-explanatory.
3. Commenting Strategy
While the goal is to make the code speak for itself, strategic use of comments can provide valuable context without resorting to wordy explanations. Comments should be used to explain the “why” behind complex logic or unconventional approaches, rather than describing what the code is doing. For example:
// Bad commenting
// Loop through the array
for (let i = 0; i < array.length; i++) {
// Do something with each element
doSomething(array[i]);
}
// Good commenting
// Use a for loop instead of forEach for better performance in this case
for (let i = 0; i < array.length; i++) {
doSomething(array[i]);
}
The second example provides insight into the reasoning behind the chosen approach, which is more valuable than simply describing what the code does.
4. Visual Aids and Diagrams
For complex algorithms or data structures, visual representations can be incredibly effective in explaining concepts without words. Tools like flowcharts, UML diagrams, or even simple ASCII art can convey relationships and processes more clearly than paragraphs of text. For instance, a binary search tree could be represented as:
50
/ \
30 70
/ \ / \
20 40 60 80
This simple visual representation immediately conveys the structure and relationships within the binary search tree.
5. Step-by-Step Code Evolution
When explaining a solution, showing the evolution of the code from a basic implementation to the final optimized version can be very effective. This technique allows others to follow the thought process and understand the reasoning behind each improvement. For example:
// Step 1: Basic implementation
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Step 2: Memoization to improve performance
function fibonacciMemo(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciMemo(n - 1, memo) + fibonacciMemo(n - 2, memo);
return memo[n];
}
// Step 3: Iterative solution for even better performance
function fibonacciIterative(n) {
if (n <= 1) return n;
let a = 0, b = 1;
for (let i = 2; i <= n; i++) {
[a, b] = [b, a + b];
}
return b;
}
By presenting the code in this way, the evolution of the solution becomes clear, showcasing the thought process behind each optimization.
Benefits of Silent Coding in Problem-Solving
Adopting a silent coding approach can significantly enhance problem-solving skills in several ways:
1. Improved Code Quality
When developers focus on making their code self-explanatory, it naturally leads to cleaner, more maintainable code. This approach encourages best practices such as:
- Proper code organization
- Modular design
- Consistent naming conventions
- Reduced code duplication
By striving to make code understandable without verbal explanations, developers often produce higher quality, more robust solutions.
2. Enhanced Logical Thinking
The process of explaining solutions without words forces developers to think more deeply about their code structure and logic. This leads to:
- More thorough problem analysis
- Consideration of edge cases
- Clearer algorithm design
- Better understanding of code complexity
As a result, developers become more adept at breaking down complex problems and designing efficient solutions.
3. Improved Collaboration
While it might seem counterintuitive, silent coding can actually enhance collaboration among team members. When code is self-explanatory:
- Code reviews become more efficient
- Onboarding new team members is easier
- Cross-team collaboration is smoother
- Knowledge sharing is more effective
Teams that practice silent coding often find that their collective problem-solving abilities improve as a result of clearer, more intuitive code.
4. Language-Agnostic Understanding
One of the most significant benefits of silent coding is its ability to transcend language barriers. When solutions are explained primarily through code and visual aids, developers from different linguistic backgrounds can more easily understand and learn from each other. This approach:
- Facilitates global collaboration
- Enhances learning from international resources
- Improves code portability across different programming languages
By focusing on the universal language of code, silent coding promotes a more inclusive and diverse programming community.
Implementing Silent Coding in Education
The principles of silent coding can be particularly beneficial in educational settings, both for teaching programming concepts and for assessing student understanding. Here are some ways to incorporate silent coding into coding education:
1. Code Reading Exercises
Encourage students to analyze and explain code without relying on verbal descriptions. This can be done through:
- Code comprehension quizzes
- Peer code review sessions
- Reverse engineering exercises
These activities help students develop their ability to understand code at a glance, a crucial skill for efficient problem-solving.
2. Visual Programming Challenges
Create coding challenges that require students to explain their solutions using only flowcharts, diagrams, or other visual representations. This approach:
- Enhances algorithmic thinking
- Improves problem decomposition skills
- Encourages creative problem-solving
By removing the option to rely on verbal explanations, students are forced to think more deeply about the structure and logic of their solutions.
3. Code Refactoring Exercises
Provide students with functional but poorly written code and challenge them to refactor it to be self-explanatory. This exercise:
- Teaches the importance of code readability
- Develops skills in code organization and structure
- Encourages critical thinking about code quality
Through these exercises, students learn to write cleaner, more maintainable code from the outset.
4. Silent Debugging Sessions
Conduct debugging sessions where students must identify and fix issues in code without verbal explanations. This can be done through:
- Pair programming with silent observation
- Written bug reports using only code snippets and comments
- Visual debugging tools and breakpoints
These sessions help students develop a keen eye for code analysis and improve their ability to quickly identify and resolve issues.
Challenges and Limitations of Silent Coding
While silent coding offers numerous benefits, it’s important to acknowledge its limitations and potential challenges:
1. Learning Curve
Adopting a silent coding approach can be challenging, especially for beginners. It requires:
- A solid foundation in programming concepts
- Practice in writing clear, self-explanatory code
- Patience and persistence in developing new habits
Educators and mentors should provide adequate support and guidance during this transition period.
2. Time Constraints
In some situations, particularly under tight deadlines, taking the time to make code entirely self-explanatory may not be feasible. Balancing code clarity with development speed is an important skill to develop.
3. Complex Concepts
Some advanced or highly abstract concepts may be difficult to explain solely through code and visual aids. In these cases, supplementary verbal or written explanations may be necessary.
4. Personal Learning Styles
Not all individuals learn or understand concepts in the same way. While silent coding can be beneficial for many, some learners may still prefer or require verbal explanations to fully grasp certain ideas.
Integrating Silent Coding with Traditional Methods
The most effective approach to problem-solving and coding education often involves a combination of silent coding techniques and traditional explanatory methods. Here are some ways to integrate silent coding into existing practices:
1. Documentation Best Practices
Encourage the use of self-documenting code alongside traditional documentation. This can include:
- Inline comments for complex logic
- README files with visual representations of system architecture
- API documentation that relies heavily on code examples
By combining these approaches, teams can create comprehensive, accessible documentation that caters to different learning styles.
2. Code Review Processes
Implement a two-stage code review process:
- Silent review: Reviewers first attempt to understand the code without any explanation from the author.
- Discussion: After the silent review, engage in a dialogue to address any remaining questions or concerns.
This approach encourages both clear code writing and effective communication skills.
3. Presentation Techniques
When presenting solutions or new features, start with a silent code walkthrough before providing verbal explanations. This can be done by:
- Sharing screens with code and allowing time for silent observation
- Using interactive coding environments for live demonstrations
- Providing visual aids alongside code snippets
This technique allows team members to form their own understanding before receiving additional context.
4. Learning Resources
Develop educational materials that incorporate both silent coding principles and traditional explanations. This can include:
- Interactive coding tutorials with minimal instructions
- Video courses that alternate between silent code demonstrations and verbal explanations
- Textbooks that emphasize code readability and visual representations
By offering diverse learning resources, educators can cater to different learning preferences while still promoting the benefits of silent coding.
Conclusion
The silent coder approach to problem-solving and explanation offers a powerful toolset for improving code quality, enhancing understanding, and fostering better collaboration among developers. By focusing on making code self-explanatory and leveraging visual aids, programmers can transcend language barriers and create more intuitive, maintainable solutions.
While silent coding presents certain challenges and may not be suitable for every situation, its principles can be effectively integrated with traditional coding practices to create a more comprehensive approach to software development and education. As the programming community continues to evolve, the ability to explain solutions without words will likely become an increasingly valuable skill for developers at all levels.
By embracing the techniques of silent coding, developers can not only improve their own problem-solving abilities but also contribute to a more accessible, efficient, and globally collaborative coding ecosystem. Whether you’re a seasoned professional or just starting your coding journey, incorporating elements of silent coding into your practice can lead to significant improvements in your skills and the overall quality of your work.