The Secret to Writing Code From Scratch: Start by Writing Pseudocode
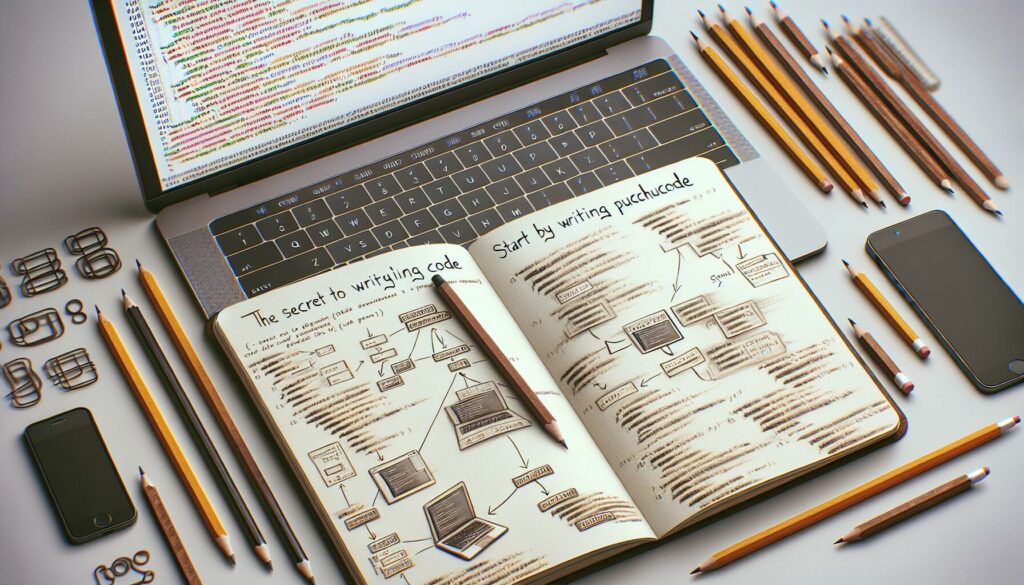
When faced with the challenge of writing code from scratch, many programmers, especially beginners, often find themselves staring at a blank screen, unsure of where to begin. The task can seem daunting, but there’s a powerful technique that can help break through this initial barrier and set you on the path to success: writing pseudocode. In this comprehensive guide, we’ll explore the art of pseudocode, its benefits, and how it can dramatically improve your coding process and outcomes.
What is Pseudocode?
Pseudocode is a informal, high-level description of a computer program or algorithm. It uses structural conventions of a normal programming language, but is intended for human reading rather than machine reading. Essentially, it’s a way of planning out your code in plain language before diving into the syntax-specific details of a particular programming language.
Think of pseudocode as a bridge between your initial idea and the final code. It allows you to outline the logic and structure of your program without getting bogged down in language-specific syntax or implementation details.
Why Start with Pseudocode?
Starting your coding process with pseudocode offers numerous advantages:
- Clarifies Thinking: Writing pseudocode forces you to think through the logic of your program step-by-step, helping you identify potential issues or gaps in your understanding early in the process.
- Language-Agnostic: Pseudocode is not tied to any specific programming language, allowing you to focus on the algorithm and logic rather than syntax.
- Easier Collaboration: Team members or mentors can easily understand and provide feedback on your approach, even if they’re not familiar with the specific programming language you’ll be using.
- Faster Development: By planning out your code in advance, you can often write the actual code more quickly and with fewer errors.
- Improved Documentation: Pseudocode can serve as a form of documentation, helping others (or your future self) understand the intent behind your code.
How to Write Effective Pseudocode
While there’s no strict standard for writing pseudocode, here are some guidelines to help you create clear and effective pseudocode:
1. Use Clear, Concise Language
Write your pseudocode in plain language that’s easy to understand. Avoid jargon or overly technical terms unless they’re necessary for clarity.
2. Keep it High-Level
Focus on the overall structure and logic of your program rather than getting into minute details. You can always add more specifics later if needed.
3. Use Indentation
Just like in real code, use indentation to show the structure and nesting of your program elements.
4. Include Control Structures
Use words like “IF”, “ELSE”, “WHILE”, “FOR” to indicate control structures in your pseudocode.
5. Be Consistent
Develop your own style and stick to it. Consistency makes your pseudocode easier to read and understand.
A Simple Pseudocode Example
Let’s look at a simple example of pseudocode for a program that finds the largest number in a list:
SET largest_number to first number in the list
FOR each number in the list
IF number > largest_number
SET largest_number to number
END IF
END FOR
PRINT largest_number
This pseudocode clearly outlines the logic of the program without getting into the specifics of how it would be implemented in any particular language.
From Pseudocode to Code
Once you have your pseudocode, translating it into actual code becomes much easier. Let’s take our pseudocode example and implement it in Python:
def find_largest_number(numbers):
largest_number = numbers[0]
for number in numbers:
if number > largest_number:
largest_number = number
return largest_number
# Example usage
number_list = [10, 5, 8, 12, 3]
result = find_largest_number(number_list)
print(f"The largest number is: {result}")
As you can see, the structure of the code closely follows the pseudocode, making the translation process straightforward.
Pseudocode for More Complex Problems
As problems become more complex, the value of pseudocode increases. Let’s look at a more involved example: implementing a basic bubble sort algorithm.
Here’s the pseudocode:
FUNCTION bubble_sort(list)
SET n to length of list
FOR i from 0 to n-1
SET swapped to false
FOR j from 0 to n-i-1
IF list[j] > list[j+1]
SWAP list[j] and list[j+1]
SET swapped to true
END IF
END FOR
IF not swapped
BREAK
END IF
END FOR
RETURN list
END FUNCTION
This pseudocode outlines the bubble sort algorithm, showing the nested loops and the swapping logic without getting bogged down in language-specific details.
Now, let’s implement this in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
if not swapped:
break
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print(f"Sorted list: {sorted_list}")
Again, you can see how closely the Python code mirrors the structure laid out in the pseudocode, demonstrating how pseudocode can serve as an effective blueprint for your actual code.
Pseudocode in Problem-Solving and Technical Interviews
Pseudocode isn’t just useful for personal projects or classroom assignments. It’s also a valuable tool in professional settings, particularly during technical interviews. Many interviewers appreciate seeing a candidate’s thought process through pseudocode before they start coding.
When faced with a coding problem in an interview, consider these steps:
- Clarify the problem and requirements with the interviewer
- Think through the logic and write pseudocode
- Explain your approach using the pseudocode
- Translate the pseudocode into actual code
- Test and refine your solution
This approach demonstrates your problem-solving skills and your ability to plan before coding, both of which are highly valued in the software development industry.
Common Pitfalls to Avoid
While pseudocode is a powerful tool, there are some common mistakes to watch out for:
1. Too Much Detail
Remember, pseudocode is meant to be a high-level outline. Don’t get bogged down in implementation details that belong in the actual code.
2. Too Little Detail
On the flip side, make sure your pseudocode provides enough information to guide your coding. Extremely vague pseudocode won’t be helpful when you start to implement.
3. Ignoring Edge Cases
Use pseudocode to think through potential edge cases or error conditions. This can save you time and headaches later in the development process.
4. Not Updating Pseudocode
If you make significant changes to your approach while coding, update your pseudocode to match. This keeps your planning and implementation in sync.
Tools and Techniques for Writing Pseudocode
While pseudocode can be written with any text editor, there are some tools and techniques that can enhance the process:
1. Flowcharts
Visual learners might find it helpful to combine pseudocode with flowcharts. Tools like draw.io or Lucidchart can be used to create flowcharts that complement your pseudocode.
2. Comment Blocks in IDEs
Many integrated development environments (IDEs) allow you to create collapsible comment blocks. You can use these to write your pseudocode directly in your code file, making it easy to reference as you implement.
3. Markdown Editors
Markdown editors like Typora or Visual Studio Code with a Markdown extension can be great for writing structured pseudocode, especially for larger projects.
4. Collaborative Tools
For team projects, collaborative tools like Google Docs or Notion can be useful for writing and discussing pseudocode together.
Pseudocode in Different Programming Paradigms
While the basic principles of pseudocode remain the same, the style might vary slightly depending on the programming paradigm you’re working in:
Procedural Programming
In procedural programming, pseudocode often focuses on describing the steps of the algorithm in a linear fashion, much like the examples we’ve seen so far.
Object-Oriented Programming (OOP)
For OOP, your pseudocode might include descriptions of classes, methods, and how objects interact. For example:
CLASS Car
PROPERTIES:
make
model
year
METHOD start_engine
PRINT "The [make] [model]'s engine is starting..."
METHOD drive
PRINT "The [year] [make] [model] is moving forward."
END CLASS
CREATE new Car with make="Toyota", model="Corolla", year=2022
CALL start_engine on new Car
CALL drive on new Car
Functional Programming
In functional programming, pseudocode might focus more on describing transformations and the flow of data. For instance:
FUNCTION process_data(data)
TRANSFORM data by doubling each number
FILTER transformed data to keep only even numbers
REDUCE filtered data to sum all numbers
RETURN result
INPUT_DATA = [1, 2, 3, 4, 5]
RESULT = process_data(INPUT_DATA)
PRINT RESULT
Pseudocode in Agile Development
In Agile development methodologies, pseudocode can play a crucial role in sprint planning and task breakdown. Here’s how you might use it:
- User Story Breakdown: Use pseudocode to break down user stories into smaller, implementable tasks.
- Sprint Planning: Write high-level pseudocode for features to be implemented in the upcoming sprint. This can help in estimating the complexity and time required for each task.
- Pair Programming: Use pseudocode as a communication tool when pair programming, allowing both developers to agree on the approach before coding begins.
- Code Reviews: Include pseudocode in code reviews to explain the intent behind complex algorithms or structures.
Teaching and Learning with Pseudocode
Pseudocode is an excellent tool for both teaching and learning programming concepts:
For Teachers
Use pseudocode to explain algorithms and programming concepts without the distraction of language-specific syntax. This allows students to focus on the logic and structure of programs.
For Students
Practice writing pseudocode for various problems to improve your problem-solving skills. Try to solve coding challenges by writing pseudocode before implementing the solution in a specific language.
Conclusion: Embracing Pseudocode in Your Coding Journey
Writing code from scratch doesn’t have to be an intimidating process. By starting with pseudocode, you can break down complex problems, clarify your thinking, and create a roadmap for your coding journey. Whether you’re a beginner just starting out or an experienced developer tackling a new challenge, pseudocode can be an invaluable tool in your programming toolkit.
Remember, the goal of pseudocode is to make the coding process smoother and more efficient. It’s not about creating a perfect blueprint, but rather about giving yourself a clear starting point and direction. As you become more comfortable with pseudocode, you’ll likely find that your coding becomes more organized, your debugging time decreases, and your overall productivity increases.
So the next time you’re faced with a blank screen and a coding task, take a step back, grab a pen and paper (or open a text editor), and start with pseudocode. You might be surprised at how quickly your ideas start flowing and how much easier the actual coding becomes. Happy coding!