The Secret Life of Semicolons: A Deep Dive into Punctuation Psychology
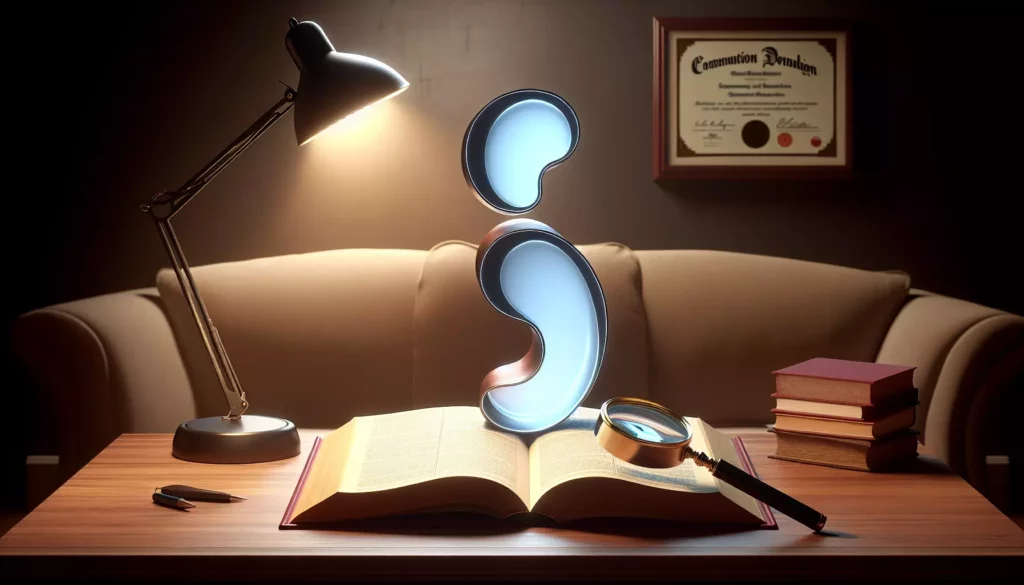
In the vast landscape of programming languages, punctuation marks play a crucial role in shaping the structure and meaning of our code. Among these, the semicolon stands out as a particularly intriguing character. Often overlooked or misunderstood, semicolons wield significant power in the world of coding. This article will explore the psychology behind semicolons, their usage across different programming languages, and how understanding their nuances can make you a more effective programmer.
The Semicolon’s Role in Programming
Before we delve into the psychological aspects of semicolons, let’s first establish their primary function in programming. In many languages, semicolons serve as statement terminators, indicating the end of a complete instruction or expression. They act as the full stop (period) of the programming world, helping to separate distinct thoughts and actions within our code.
For example, in languages like Java, C++, and JavaScript, you’ll often see code structures like this:
int x = 5;
System.out.println("The value of x is: " + x);
Here, the semicolons clearly demarcate the end of each statement, providing both the compiler and human readers with a clear understanding of where one instruction ends and another begins.
The Psychology of Semicolon Usage
Now that we’ve established the basic role of semicolons, let’s explore the psychological aspects of their usage in programming:
1. Cognitive Load and Code Readability
One of the primary psychological effects of semicolons is their impact on cognitive load and code readability. When used consistently, semicolons can significantly reduce the mental effort required to parse and understand code. They provide clear visual cues that help programmers quickly identify the boundaries of statements and expressions.
Consider the following JavaScript code snippets:
// Without semicolons
let x = 5
let y = 10
let sum = x + y
console.log(sum)
// With semicolons
let x = 5;
let y = 10;
let sum = x + y;
console.log(sum);
While both snippets are valid in JavaScript (due to its automatic semicolon insertion feature), the version with semicolons is generally considered more readable. The semicolons provide a clear visual break between statements, reducing the cognitive load required to understand the code’s structure.
2. The Semicolon as a Rhythm Marker
In the realm of programming psychology, semicolons can be viewed as rhythm markers in code. Just as punctuation in written language helps to establish the rhythm and flow of text, semicolons in code create a cadence that can influence how programmers read and interpret the code.
This rhythmic aspect of semicolons is particularly evident in languages that require them, such as Java or C++. Experienced programmers in these languages often develop an internal “code rhythm” that relies on the presence of semicolons. When a semicolon is missing, it can feel like a missed beat in a musical composition, immediately alerting the programmer to a potential issue.
3. Semicolons and Code Confidence
The use of semicolons can also have a psychological impact on a programmer’s confidence in their code. In languages where semicolons are required, their presence serves as a form of self-verification. Each semicolon acts as a small checkpoint, reassuring the programmer that they’ve completed a valid statement.
This confidence-building aspect of semicolons is particularly valuable for beginner programmers. As they learn to write code, the habit of ending each statement with a semicolon reinforces their understanding of program structure and helps prevent certain types of syntax errors.
4. The Debate: Semicolon Lovers vs. Haters
Interestingly, the use of semicolons has become a topic of debate in the programming community, particularly in languages where they are optional (like JavaScript). This debate reveals interesting psychological divides among programmers:
- Semicolon Lovers: Those who prefer to always use semicolons often cite reasons such as consistency, clarity, and the prevention of potential errors. They may feel that code without semicolons looks “incomplete” or “sloppy”.
- Semicolon Haters: On the other hand, some programmers prefer to omit semicolons when possible, arguing that they add unnecessary visual clutter. These developers may feel that code without semicolons looks “cleaner” or more “modern”.
This divide often reflects deeper psychological preferences related to code aesthetics, personal coding style, and even attitudes towards programming language design.
Semicolons Across Different Programming Languages
To fully understand the psychology of semicolons, it’s important to consider how they’re used (or not used) in different programming languages. Let’s explore some popular languages and their relationship with semicolons:
1. Java
In Java, semicolons are mandatory at the end of most statements. This strict requirement aligns with Java’s philosophy of explicit and unambiguous code. Psychologically, this can create a sense of structure and completeness for Java programmers.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
2. Python
Python takes the opposite approach, using indentation to define code blocks and newlines to separate statements. Semicolons are rarely used in Python, except when putting multiple statements on a single line. This approach aligns with Python’s philosophy of readability and simplicity.
def greet(name):
print(f"Hello, {name}!")
greet("World")
The absence of semicolons in Python can be psychologically freeing for some programmers, allowing them to focus more on the logic of their code rather than syntax details.
3. JavaScript
JavaScript presents an interesting case study in semicolon psychology. While semicolons are technically optional in many cases due to Automatic Semicolon Insertion (ASI), their use is still widely debated in the JavaScript community.
// With semicolons
let greeting = "Hello";
let name = "World";
console.log(greeting + ", " + name + "!");
// Without semicolons (relying on ASI)
let greeting = "Hello"
let name = "World"
console.log(greeting + ", " + name + "!")
The optional nature of semicolons in JavaScript has led to interesting psychological effects, including the development of strong personal preferences and coding styles.
4. Go (Golang)
Go takes a unique approach to semicolons. While they are technically part of the language’s grammar, they are automatically inserted by the lexer in most cases, meaning programmers rarely need to type them explicitly. This approach aims to combine the readability benefits of semicolon-free code with the precision of semicolon-terminated statements.
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Psychologically, this approach can provide a sense of simplicity similar to Python, while still maintaining a level of structure that semicolon-loving programmers might appreciate.
The Impact of Semicolons on Learning and Teaching Programming
The role of semicolons in programming languages can have significant implications for how we learn and teach coding. Let’s explore some of these effects:
1. Beginner-Friendly vs. Strict Syntax
Languages that require semicolons, like Java or C++, often have a steeper initial learning curve for beginners. The need to remember semicolons can be an additional cognitive burden for new programmers who are already struggling with fundamental concepts.
On the other hand, languages like Python, which don’t use semicolons, are often considered more beginner-friendly. The absence of this syntax requirement allows new learners to focus more on logic and problem-solving rather than worrying about syntax errors caused by missing semicolons.
2. Developing Good Habits
For educators, teaching languages with mandatory semicolons can be an opportunity to instill good coding habits early on. The discipline of always ending statements with semicolons can translate into other good practices, such as proper indentation and consistent code formatting.
3. Transitioning Between Languages
As programmers learn multiple languages, the presence or absence of semicolons can be a point of confusion. Developers moving from a semicolon-mandatory language to one where they’re optional (or vice versa) often go through an adjustment period. This transition can be an excellent opportunity to discuss the deeper reasons behind language design choices and to develop a more nuanced understanding of syntax across different programming paradigms.
4. Code Review and Collaboration
In collaborative environments, semicolon usage (or lack thereof) can become a point of discussion in code reviews. This can be an opportunity to develop critical thinking about code style and to learn how to articulate preferences and rationales for coding decisions.
Semicolons and Code Optimization
While semicolons primarily serve a syntactic purpose, their use (or omission) can have subtle effects on code optimization and performance in certain scenarios:
1. Minification in JavaScript
In JavaScript, the presence of semicolons can affect how code is minified. Minification is the process of reducing code size by removing unnecessary characters without changing functionality. In some cases, relying on Automatic Semicolon Insertion (ASI) without explicit semicolons can lead to unexpected behavior when the code is minified.
// Original code without semicolons
return
{
result: value
}
// Minified version (which may not behave as expected)
return{result:value}
In this example, the lack of a semicolon after `return` could lead to the function returning `undefined` instead of the object, as ASI would insert a semicolon after `return`.
2. Parse Time in Compiled Languages
In compiled languages like Java or C++, the presence of semicolons can slightly affect parse times. While the difference is usually negligible for small to medium-sized projects, in very large codebases, the cumulative effect of parsing semicolons could theoretically impact compilation times.
3. Runtime Performance
It’s important to note that in most modern languages, the presence or absence of semicolons has no impact on runtime performance. Once the code is compiled or interpreted, semicolons don’t affect how the program executes.
The Future of Semicolons in Programming
As programming languages continue to evolve, it’s interesting to consider the future role of semicolons:
1. Trend Towards Optional Semicolons
There seems to be a trend in newer languages towards making semicolons optional or eliminating them altogether. Languages like Kotlin, Swift, and Rust have adopted more flexible approaches to statement termination, often using newlines as natural separators.
2. Balancing Readability and Flexibility
Future language designs may continue to explore ways to balance the readability benefits of clear statement separation with the flexibility and cleanliness of semicolon-free code. We might see more languages adopting approaches similar to Go, where semicolons are part of the language grammar but are inserted automatically in most cases.
3. Personalization and IDE Support
As development environments become more sophisticated, we might see increased support for personal preferences regarding semicolons. IDEs could offer features to automatically add or remove semicolons based on user preferences, or to enforce team-wide styling rules.
Conclusion: The Psychological Power of a Tiny Symbol
The semicolon, despite its small size, carries significant psychological weight in the world of programming. From its role in reducing cognitive load and establishing code rhythm to its impact on learning and collaboration, this tiny symbol influences how we write, read, and think about code.
Understanding the psychology behind semicolons and other syntactic elements can make us more thoughtful and effective programmers. It encourages us to consider not just the functional aspects of our code, but also its readability, maintainability, and even its aesthetic qualities.
Whether you’re a semicolon enthusiast or prefer your code sans-semicolon, being aware of these psychological aspects can help you make more informed decisions about your coding style and practices. It can also foster empathy and understanding when collaborating with developers who may have different preferences or backgrounds.
As we continue to push the boundaries of what’s possible with code, let’s not forget the humble semicolon and the outsized role it plays in shaping our programming experiences. After all, in the intricate dance of logic and syntax that is programming, even the smallest steps can have profound impacts.
So the next time you type (or deliberately omit) a semicolon, take a moment to appreciate the complex psychology behind this simple punctuation mark. In the end, whether you use semicolons or not, the most important thing is writing clear, effective, and maintainable code that solves real-world problems. And that’s a goal we can all agree on, regardless of our punctuation preferences.