The Secret Language of LeetCode: Decoding What ‘Easy’ Really Means
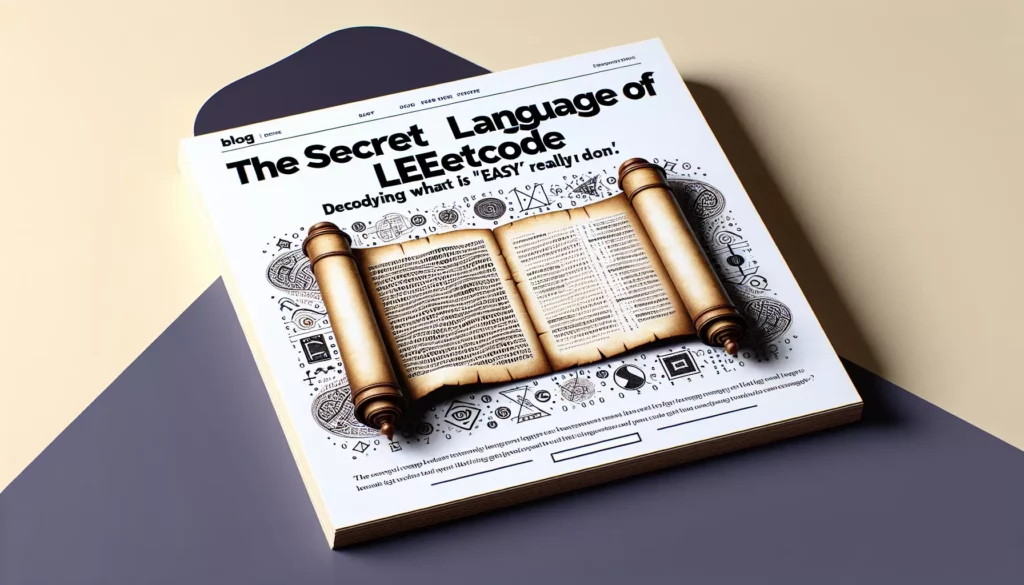
If you’ve ever ventured into the world of coding interviews or algorithmic problem-solving, chances are you’ve encountered LeetCode. This popular platform has become a cornerstone for developers preparing for technical interviews, especially those eyeing positions at major tech companies. But for many, especially beginners, there’s a puzzling aspect to LeetCode that often leaves them scratching their heads: the difficulty ratings. In this post, we’ll dive deep into the mysterious world of LeetCode’s “Easy” problems, decoding what they really mean and how to approach them effectively.
Understanding LeetCode’s Difficulty Levels
Before we delve into the specifics of “Easy” problems, let’s briefly overview LeetCode’s difficulty classification system:
- Easy: Supposedly straightforward problems that should be solvable with basic programming knowledge.
- Medium: More complex problems that may require some algorithmic thinking or data structure knowledge.
- Hard: Challenging problems that often involve advanced algorithms or complex problem-solving techniques.
At first glance, this system seems logical and helpful. However, many users quickly discover that “Easy” doesn’t always mean what they think it does.
The Reality of “Easy” Problems
For many LeetCode newcomers, tackling their first “Easy” problem can be a humbling experience. What’s marketed as straightforward can often feel like deciphering an ancient code. Here are some reasons why “Easy” problems might not feel so easy:
1. Assumed Knowledge
LeetCode’s “Easy” classification often assumes a certain level of programming knowledge and familiarity with common data structures and algorithms. For instance, an “Easy” problem might require understanding of:
- Basic data structures like arrays, linked lists, and hash tables
- Simple sorting and searching algorithms
- Fundamental programming concepts like loops, conditionals, and functions
For a complete beginner or someone transitioning from a different field, these “basic” concepts might not be so basic after all.
2. Problem-Solving Skills
Even if you understand the underlying concepts, translating a problem statement into code requires a specific set of problem-solving skills. “Easy” problems often test your ability to:
- Break down a problem into smaller, manageable parts
- Identify patterns and edge cases
- Translate your thought process into code
These skills take time and practice to develop, regardless of how “easy” a problem is supposed to be.
3. Time Pressure
In the context of interview preparation, solving a problem correctly is only part of the challenge. Many users feel pressured to solve “Easy” problems quickly, often within 15-20 minutes. This time constraint can make even simple problems feel more difficult than they actually are.
4. Optimal Solutions
While LeetCode allows you to submit any correct solution, there’s often an unspoken expectation to find the most efficient or “optimal” solution. This can turn an otherwise straightforward problem into a brain-teaser as you try to optimize for time and space complexity.
Decoding the “Easy” Label: A Case Study
Let’s look at a classic “Easy” LeetCode problem to understand what it really entails. Consider the “Two Sum” problem:
Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target. You may assume that each input would have exactly one solution, and you may not use the same element twice.
At first glance, this problem seems simple. Just find two numbers that add up to a target. Easy, right? Let’s break it down:
The Naive Approach
A beginner might approach this with a straightforward nested loop:
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
This solution works and is easy to understand. However, it has a time complexity of O(n^2), which isn’t optimal for large inputs.
The Optimal Approach
A more efficient solution uses a hash table:
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
This solution has a time complexity of O(n) and a space complexity of O(n), making it much more efficient for large inputs.
The Hidden Complexity
While both solutions solve the problem, the optimal solution requires:
- Understanding of hash tables and their time complexity benefits
- Ability to think in terms of complements rather than direct sums
- Knowledge of Python’s dictionary operations and enumeration
Suddenly, our “Easy” problem doesn’t seem so straightforward anymore, especially for someone new to these concepts.
Strategies for Tackling “Easy” Problems
Now that we’ve demystified what “Easy” really means in LeetCode terms, let’s explore some strategies to approach these problems more effectively:
1. Build a Strong Foundation
Before diving into LeetCode, ensure you have a solid grasp of fundamental programming concepts and basic data structures. Resources like AlgoCademy can provide interactive tutorials and step-by-step guidance to build this foundation.
2. Start with the Brute Force
When approaching a new problem, start with the simplest solution that comes to mind, even if it’s not optimal. This helps you understand the problem better and gives you a working solution to improve upon.
3. Learn to Identify Patterns
Many LeetCode problems, even “Easy” ones, follow common patterns. Learning to recognize these patterns can help you solve similar problems more quickly. Some common patterns include:
- Two-pointer technique
- Sliding window
- Hash table lookups
- Depth-first search (DFS) and Breadth-first search (BFS) for tree/graph problems
4. Practice Regularly
Consistency is key when it comes to improving your problem-solving skills. Set aside time each day to solve at least one problem, even if it’s just for 30 minutes.
5. Study Solutions
After solving a problem (or if you’re stuck), take the time to study other people’s solutions. LeetCode provides a discussion forum for each problem where users share their approaches. This can expose you to new techniques and ways of thinking.
6. Focus on Understanding, Not Memorization
While it’s tempting to try and memorize solutions to common problems, focus instead on understanding the underlying principles. This will serve you better in the long run, especially in actual interview situations.
7. Use External Resources
Don’t hesitate to use external resources to fill knowledge gaps. Websites like AlgoCademy offer targeted learning paths and AI-powered assistance to help you understand difficult concepts.
The Psychology of “Easy” Problems
Understanding the technical aspects of LeetCode problems is crucial, but it’s equally important to address the psychological impact of the “Easy” label:
Imposter Syndrome
Many developers, especially beginners, experience imposter syndrome when they struggle with “Easy” problems. It’s important to remember that difficulty is subjective and that everyone starts somewhere. Your worth as a developer isn’t determined by how quickly you can solve LeetCode problems.
The Learning Curve
Learning to solve algorithmic problems is a skill in itself, separate from general programming. Even experienced developers might find themselves struggling initially with LeetCode-style problems. Recognize that there’s a learning curve, and be patient with yourself as you climb it.
Comparison Trap
It’s easy to fall into the trap of comparing yourself to others, especially when you see people solving “Easy” problems in minutes. Remember that everyone’s journey is different, and focus on your own progress rather than how you measure up to others.
Beyond “Easy”: Preparing for Real Interviews
While mastering LeetCode’s “Easy” problems is a great start, it’s important to remember that real technical interviews often involve more than just solving algorithmic puzzles. Here are some additional aspects to consider in your preparation:
1. Communication Skills
In a real interview, you’ll need to explain your thought process as you solve problems. Practice talking through your solutions out loud, explaining your reasoning for each step.
2. System Design
Many interviews, especially for more senior positions, include system design questions. These go beyond algorithmic problem-solving and test your ability to design scalable, efficient systems.
3. Behavioral Questions
Don’t forget to prepare for behavioral questions that assess your soft skills, teamwork abilities, and past experiences.
4. Company-Specific Knowledge
Research the companies you’re interviewing with and be prepared to discuss their products, technologies, and recent developments.
5. Mock Interviews
Consider participating in mock interviews to simulate the pressure and environment of a real technical interview. Platforms like AlgoCademy often offer features to help with this.
Conclusion: Embracing the Journey
Decoding LeetCode’s “Easy” problems is just the beginning of a larger journey into the world of algorithmic problem-solving and technical interviews. While these problems may sometimes feel anything but easy, they serve as valuable stepping stones in developing your skills and confidence as a programmer.
Remember that the goal isn’t just to solve problems quickly, but to develop a deep understanding of fundamental concepts and problem-solving strategies. Embrace the challenges, celebrate your progress, and don’t be discouraged by initial difficulties. With persistence, practice, and the right resources, you’ll find yourself not just decoding LeetCode’s secret language, but fluently speaking it.
As you continue your journey, consider leveraging comprehensive learning platforms like AlgoCademy, which offer structured paths from basics to advanced topics, interactive coding environments, and AI-assisted learning. These tools can provide the guidance and practice you need to not just survive LeetCode’s “Easy” problems, but to thrive in your coding interview preparation and beyond.
Happy coding, and may your LeetCode journey be as rewarding as it is challenging!