The Secret Language of For Loops: What They’re Really Saying About You
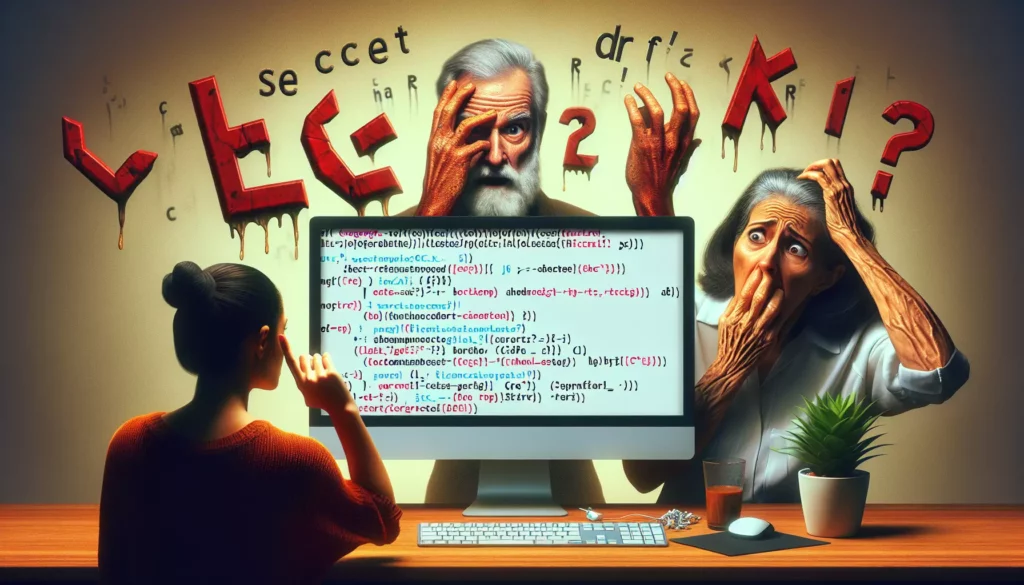
Have you ever wondered what your for loops say about you as a programmer? It might sound strange, but the way you write and use for loops can reveal a lot about your coding style, problem-solving approach, and even your personality. In this deep dive into the world of for loops, we’ll uncover the hidden messages behind these fundamental programming constructs and explore how mastering them can elevate your coding skills to new heights.
The Basics: What is a For Loop?
Before we decode the secret language of for loops, let’s quickly recap what they are and why they’re so important in programming. A for loop is a control flow statement that allows you to execute a block of code repeatedly for a specified number of iterations. It’s one of the most commonly used loop structures in programming and is supported by virtually all modern programming languages.
The basic syntax of a for loop typically consists of three parts:
- Initialization: Where you set up the initial value of the loop counter
- Condition: The condition that determines when the loop should stop
- Iteration: How the loop counter changes after each iteration
Here’s a simple example of a for loop in Python:
for i in range(5):
print(i)
This loop will print the numbers 0 through 4. Simple, right? But there’s so much more to for loops than meets the eye.
The Loop Whisperer: Decoding Your For Loop Style
Now that we’ve covered the basics, let’s dive into what your for loops might be saying about you as a programmer. Are you ready to have your coding soul laid bare?
1. The Traditionalist
If you find yourself frequently writing for loops like this:
for (int i = 0; i < n; i++) {
// Your code here
}
You’re likely a traditionalist. You appreciate the classic C-style for loop and stick to what works. This approach shows that you have a solid foundation in programming fundamentals and value clarity in your code. However, it might also suggest that you’re hesitant to embrace newer, more concise loop constructs available in modern languages.
2. The Pythonista
If your for loops often look like this:
for item in iterable:
# Your code here
You’re probably a Pythonista at heart, even if you’re coding in another language. You value readability and simplicity, and you’re not afraid to embrace more modern, expressive ways of looping. This style indicates that you’re adaptable and quick to adopt language-specific features that can make your code more elegant and maintainable.
3. The Functional Enthusiast
Do you find yourself reaching for map(), filter(), or list comprehensions instead of traditional for loops?
squared_numbers = [x**2 for x in range(10)]
If so, you’re likely a functional programming enthusiast. You appreciate the conciseness and expressiveness of functional constructs and are always looking for ways to make your code more declarative. This approach suggests that you’re open to different programming paradigms and are constantly seeking to improve your code’s efficiency and readability.
4. The Optimizer
If your for loops often include performance optimizations like this:
for (int i = 0, len = array.length; i < len; i++) {
// Your code here
}
You’re probably an optimizer at heart. You’re always thinking about performance and are willing to sacrifice a bit of readability for the sake of efficiency. This style indicates that you have a deep understanding of how loops work under the hood and are mindful of the impact your code can have on system resources.
5. The Nested Loop Ninja
If you find yourself frequently writing nested loops like this:
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
// Your code here
}
}
You might be a nested loop ninja. You’re comfortable dealing with multi-dimensional data structures and complex algorithms. This approach shows that you can handle intricate logic and aren’t afraid to tackle challenging problems. However, it might also suggest that you need to be cautious about code complexity and consider alternative approaches when possible.
Leveling Up Your For Loop Game
Now that we’ve decoded the secret language of for loops, let’s explore how you can level up your loop game and become a true loop master. Here are some advanced techniques and best practices to consider:
1. Embrace Language-Specific Features
Many modern programming languages offer specialized loop constructs that can make your code more readable and efficient. For example, in JavaScript, you can use forEach() for arrays:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(number => console.log(number));
Or in Python, you can use enumerate() when you need both the index and value:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(f"{index}: {fruit}")
2. Know When to Use Different Loop Types
While for loops are versatile, sometimes other loop types might be more appropriate. For example, when you don’t know the number of iterations in advance, a while loop might be a better choice:
while not done:
# Process data
done = check_condition()
3. Consider Loop Unrolling for Performance
In performance-critical sections of your code, you might consider loop unrolling. This technique can reduce the overhead of loop control and potentially allow for better instruction pipelining:
for (int i = 0; i < n; i += 4) {
// Process four elements at once
process(array[i]);
process(array[i+1]);
process(array[i+2]);
process(array[i+3]);
}
4. Use Iterator Methods for Cleaner Code
Many languages offer iterator methods that can replace explicit loops, leading to more declarative and often more readable code. For example, in JavaScript:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(n => n % 2 === 0);
const doubledNumbers = numbers.map(n => n * 2);
5. Be Mindful of Loop Invariants
Identifying and optimizing loop invariants can significantly improve your loop’s performance. A loop invariant is a condition that remains true throughout all iterations of a loop. By moving invariant computations outside the loop, you can reduce unnecessary calculations:
const len = array.length; // Loop invariant
for (let i = 0; i < len; i++) {
// Use len instead of recalculating array.length each iteration
}
The Psychology of Loops: What Your For Loops Really Reveal
Now that we’ve explored various loop styles and techniques, let’s delve deeper into what your for loops might reveal about your problem-solving approach and coding personality.
The Meticulous Planner
If your for loops are always precisely structured with clear initialization, condition, and iteration steps, you’re likely a meticulous planner. You approach problems systematically and prefer to have a clear roadmap before diving into implementation. This trait can be incredibly valuable when working on complex projects that require careful coordination and planning.
The Iterative Thinker
Do you find yourself frequently using nested loops or loops within loops? You might be an iterative thinker who excels at breaking down complex problems into smaller, manageable steps. This approach can be powerful when dealing with multi-dimensional data or intricate algorithms, but it’s important to balance it with considerations for code readability and maintainability.
The Efficiency Enthusiast
If you’re always looking for ways to optimize your loops, whether through loop unrolling, minimizing operations within the loop body, or using language-specific optimizations, you’re likely an efficiency enthusiast. You have a deep appreciation for performance and are always striving to make your code run faster and use fewer resources. This mindset is crucial for developing high-performance applications, but remember to balance it with code readability and maintainability.
The Abstraction Lover
Do you prefer using higher-order functions like map() and filter() over traditional for loops? You might be an abstraction lover who enjoys working at a higher level of abstraction. This approach can lead to more concise and expressive code, but it’s important to ensure that your team is comfortable with these abstractions and that they don’t obscure the underlying logic.
The Pragmatic Adaptor
If you find yourself using different loop styles depending on the context – traditional for loops in some cases, list comprehensions in others, and iterator methods elsewhere – you’re likely a pragmatic adaptor. You understand that different situations call for different approaches and are flexible in your coding style. This adaptability is a valuable trait in a diverse coding environment, allowing you to work effectively across different projects and teams.
Mastering the Art of Looping: Best Practices and Pitfalls to Avoid
As we’ve seen, for loops are more than just a simple programming construct – they’re a reflection of your coding style and problem-solving approach. To truly master the art of looping, consider the following best practices and common pitfalls to avoid:
Best Practices
- Keep it Simple: Strive for simplicity in your loops. If a loop becomes too complex, consider breaking it down into smaller, more manageable pieces.
- Use Meaningful Variable Names: Instead of using single-letter variables like ‘i’, ‘j’, or ‘k’, use descriptive names that convey the purpose of the loop.
- Consider the Readability-Performance Trade-off: While optimizing for performance is important, don’t sacrifice readability unless absolutely necessary. In most cases, clear, maintainable code is more valuable than micro-optimizations.
- Use the Appropriate Loop Construct: Choose the right type of loop for the job. For known iterations, use a for loop. For conditional looping, use a while loop. For iterating over collections, use language-specific constructs like foreach loops.
- Be Mindful of Loop Boundaries: Pay attention to off-by-one errors, especially when dealing with array indices. Use < for exclusive upper bounds and <= for inclusive upper bounds.
Common Pitfalls to Avoid
- Infinite Loops: Always ensure that your loop has a clear termination condition that will eventually be met.
- Modifying Loop Variables Within the Loop: Changing the loop counter inside the loop body can lead to unexpected behavior and make the code harder to understand.
- Unnecessary Complexity: Avoid overcomplicating your loops. If you find yourself writing deeply nested loops or loops with multiple exit conditions, consider refactoring your approach.
- Ignoring Performance in Critical Sections: While premature optimization is often discouraged, be aware of the performance implications of your loops, especially in performance-critical sections of your code.
- Neglecting Edge Cases: Always consider how your loop will behave with empty collections, single-element collections, or other edge cases.
The Future of Looping: Trends and Innovations
As programming languages and paradigms evolve, so too does the way we approach looping. Here are some trends and innovations that are shaping the future of looping in programming:
1. Increased Abstraction
Many modern programming languages are moving towards higher levels of abstraction when it comes to looping. This trend is evident in the popularity of functional programming constructs and the increasing use of iterator methods. For example, in JavaScript, the introduction of async/await has led to new ways of handling asynchronous loops:
for await (const item of asyncIterable) {
console.log(item);
}
2. Parallelization and Concurrency
As multi-core processors become ubiquitous, there’s an increasing focus on parallelizing loops for better performance. Many languages now offer constructs for parallel looping. For instance, C# provides the Parallel.For method:
Parallel.For(0, 1000, i =>
{
// Parallel processing here
});
3. Machine Learning and Adaptive Loops
With the rise of machine learning, we’re starting to see the emergence of “smart” loops that can adapt their behavior based on data patterns or runtime conditions. While still in its early stages, this could lead to more efficient and context-aware looping constructs in the future.
4. Domain-Specific Loop Optimizations
As programming languages become more specialized, we’re likely to see more domain-specific loop optimizations. For example, languages designed for data science or scientific computing might offer specialized looping constructs optimized for matrix operations or large dataset processing.
Conclusion: Embracing Your Loop Personality
As we’ve explored in this deep dive into the world of for loops, these fundamental programming constructs reveal much more than just a way to repeat code. They offer insights into your problem-solving approach, your coding style, and even your personality as a programmer.
Whether you’re a traditionalist who sticks to classic C-style loops, a Pythonista who embraces language-specific features, a functional enthusiast who prefers higher-order functions, an optimizer always looking for performance gains, or a nested loop ninja tackling complex algorithms, your approach to loops is a unique reflection of your programming journey.
The key to mastering loops isn’t just about knowing the syntax or understanding the mechanics. It’s about recognizing when and how to use different looping constructs effectively, being mindful of performance implications, and always striving for code that is not only functional but also readable and maintainable.
As you continue your programming journey, embrace your loop personality while remaining open to new techniques and approaches. The world of programming is constantly evolving, and so too should your approach to loops. By staying curious, adaptable, and always eager to learn, you’ll be well-equipped to tackle any looping challenge that comes your way.
Remember, in the end, the best loop is the one that solves the problem efficiently and clearly. So go forth and loop with confidence, knowing that each for loop you write is not just a piece of code, but a reflection of your unique approach to problem-solving in the vast and exciting world of programming.