The Secret Language of Error Messages: What Your Computer is Really Saying
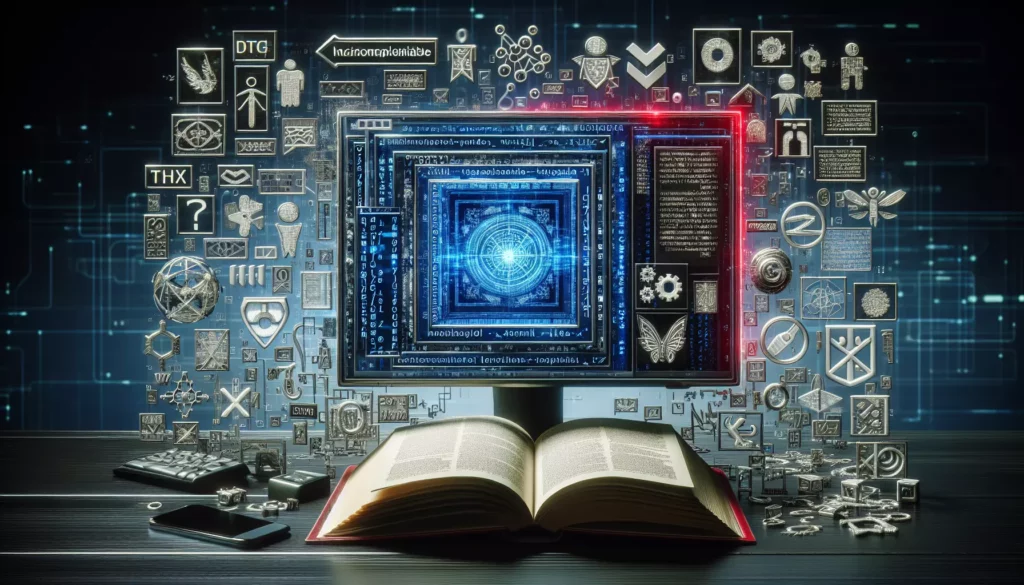
As aspiring programmers and coding enthusiasts, we’ve all been there: staring at the screen, scratching our heads, and wondering what on earth our computer is trying to tell us. Error messages can be cryptic, frustrating, and sometimes downright intimidating. But fear not! In this comprehensive guide, we’ll decode the secret language of error messages and help you understand what your computer is really saying. By the end of this article, you’ll be equipped with the knowledge to tackle common error messages head-on and become a more efficient problem-solver in your coding journey.
Why Understanding Error Messages Matters
Before we dive into the nitty-gritty of error messages, let’s take a moment to understand why deciphering them is crucial for your growth as a programmer:
- Faster Debugging: When you can quickly interpret error messages, you’ll spend less time scratching your head and more time fixing the issue.
- Improved Problem-Solving Skills: Learning to read error messages effectively enhances your analytical thinking and helps you approach problems more systematically.
- Better Code Quality: Understanding errors leads to writing more robust and error-free code in the future.
- Increased Confidence: As you become more adept at handling errors, you’ll gain confidence in your coding abilities and tackle more complex projects.
The Anatomy of an Error Message
Error messages may seem like a jumble of words and numbers, but they typically follow a structure. Let’s break down the common components of an error message:
- Error Type: This indicates the category of the error (e.g., SyntaxError, TypeError, ValueError).
- Error Message: A brief description of what went wrong.
- File Name and Line Number: The location in your code where the error occurred.
- Traceback: A step-by-step recount of the function calls that led to the error.
Here’s an example of a typical error message in Python:
Traceback (most recent call last):
File "example.py", line 5, in <module>
result = 10 / 0
ZeroDivisionError: division by zero
In this example, we can identify:
- Error Type: ZeroDivisionError
- Error Message: “division by zero”
- File Name and Line Number: example.py, line 5
- Traceback: The sequence of events leading to the error
Common Error Types and What They Mean
Now that we understand the structure of error messages, let’s explore some common error types you’re likely to encounter in your coding journey:
1. SyntaxError
A SyntaxError occurs when your code violates the rules of the programming language. It’s like a grammatical error in written language.
Example:
print("Hello, World!"
SyntaxError: unexpected EOF while parsing
What it’s really saying: “Hey, you forgot to close your parentheses! Double-check your syntax and make sure all your brackets, quotes, and parentheses are properly matched.”
2. IndentationError
In Python, proper indentation is crucial. An IndentationError occurs when your code’s indentation is inconsistent or incorrect.
Example:
def greet(name):
print(f"Hello, {name}!")
IndentationError: expected an indented block
What it’s really saying: “Oops! Your code’s formatting is off. Make sure you’re using consistent indentation, especially after colons in function definitions, loops, and conditional statements.”
3. NameError
A NameError pops up when you try to use a variable or function that hasn’t been defined yet.
Example:
print(undefined_variable)
NameError: name 'undefined_variable' is not defined
What it’s really saying: “I can’t find this variable or function you’re trying to use. Did you forget to define it, or maybe you misspelled its name?”
4. TypeError
TypeErrors occur when you perform an operation on an incompatible data type.
Example:
result = "5" + 10
TypeError: can only concatenate str (not "int") to str
What it’s really saying: “Hold up! You’re trying to mix apples and oranges here. Make sure you’re using compatible data types in your operations, or convert them if necessary.”
5. ValueError
A ValueError is raised when a function receives an argument of the correct type but an inappropriate value.
Example:
int("hello")
ValueError: invalid literal for int() with base 10: 'hello'
What it’s really saying: “I understand what you’re trying to do, but the value you provided doesn’t make sense in this context. Double-check your input and make sure it’s appropriate for the operation you’re performing.”
6. IndexError
An IndexError occurs when you try to access an index that doesn’t exist in a sequence (like a list or string).
Example:
my_list = [1, 2, 3]
print(my_list[3])
IndexError: list index out of range
What it’s really saying: “Whoa there! You’re trying to access an element that doesn’t exist. Remember, list indices start at 0, so a list with 3 elements has indices 0, 1, and 2.”
7. KeyError
A KeyError is raised when you try to access a dictionary with a key that doesn’t exist.
Example:
my_dict = {"apple": 1, "banana": 2}
print(my_dict["cherry"])
KeyError: 'cherry'
What it’s really saying: “I looked everywhere, but I can’t find the key you’re asking for in this dictionary. Are you sure it exists, or did you maybe misspell it?”
Strategies for Decoding Error Messages
Now that we’re familiar with common error types, let’s explore some strategies to help you become a pro at decoding error messages:
1. Read the Error Message Carefully
It might seem obvious, but many programmers skip this crucial step. Take the time to read the entire error message, including the traceback. Often, the information you need is right there in plain sight.
2. Identify the Error Type
Knowing the error type (SyntaxError, TypeError, etc.) can immediately narrow down the possible causes of the problem. Use this as your starting point for debugging.
3. Locate the Error in Your Code
Most error messages will point you to the exact line where the error occurred. Go to that line in your code and examine it closely. Sometimes, the issue might be in the surrounding lines, so expand your search if necessary.
4. Use Print Statements for Debugging
If you’re unsure about the values of variables or the flow of your program, insert print statements at strategic points in your code. This can help you trace the execution and pinpoint where things go wrong.
5. Google the Error Message
If you’re still stumped, don’t hesitate to search for the error message online. Chances are, someone else has encountered the same issue and found a solution. Websites like Stack Overflow can be invaluable resources.
6. Use a Debugger
For more complex issues, learning to use a debugger can be a game-changer. Debuggers allow you to step through your code line by line, examine variable values, and track the flow of your program in real-time.
7. Take a Break and Come Back Later
Sometimes, the best thing you can do is step away from your code for a while. A fresh perspective can often lead to a breakthrough when you return to the problem.
Advanced Error Handling Techniques
As you progress in your coding journey, you’ll encounter more complex scenarios where simple error messages might not be enough. Here are some advanced techniques to handle errors more effectively:
1. Try-Except Blocks
Try-except blocks allow you to gracefully handle exceptions that might occur in your code. Instead of crashing your program, you can catch the error and decide how to respond.
Example:
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: Cannot divide by zero!")
result = None
2. Custom Exceptions
Creating your own custom exceptions can make your code more readable and maintainable, especially in larger projects.
Example:
class InvalidAgeError(Exception):
pass
def set_age(age):
if age < 0:
raise InvalidAgeError("Age cannot be negative")
return age
try:
my_age = set_age(-5)
except InvalidAgeError as e:
print(f"Error: {e}")
3. Logging
Instead of using print statements for debugging, consider using Python’s logging module. It provides a more flexible and powerful way to track what’s happening in your code.
Example:
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
def divide(a, b):
logger.debug(f"Dividing {a} by {b}")
try:
result = a / b
except ZeroDivisionError:
logger.error("Division by zero attempted")
result = None
return result
print(divide(10, 0))
4. Assertions
Assertions are a way to ensure that certain conditions are met in your code. They can help catch bugs early and make your code more robust.
Example:
def calculate_average(numbers):
assert len(numbers) > 0, "List cannot be empty"
return sum(numbers) / len(numbers)
print(calculate_average([])) # This will raise an AssertionError
Error Messages in Different Programming Languages
While we’ve primarily focused on Python in our examples, it’s worth noting that error messages can vary across different programming languages. Let’s take a quick look at how error messages might appear in some other popular languages:
JavaScript
JavaScript error messages often appear in the browser’s console and include the error type, message, and a stack trace.
Example:
Uncaught TypeError: Cannot read property 'length' of undefined
at calculateAverage (script.js:3)
at <anonymous>:1:1
Java
Java error messages typically include the exception type, message, and a detailed stack trace.
Example:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 3
at Main.main(Main.java:5)
C++
C++ error messages can be quite verbose, especially when dealing with template errors. They often include the error type, location, and additional context.
Example:
error: no match for 'operator[]' (operand types are 'std::string' {aka 'std::basic_string<char>'} and 'int')
cout << myString[5] << endl;
^
Conclusion: Embracing the Error Message
As we’ve explored in this comprehensive guide, error messages are not your enemy. They’re valuable tools that can help you become a better programmer. By understanding the secret language of error messages, you’ll be able to:
- Debug your code more efficiently
- Write more robust and error-free programs
- Develop stronger problem-solving skills
- Gain confidence in your coding abilities
Remember, every experienced programmer has faced countless error messages on their journey. The key is to approach each one as a learning opportunity rather than a setback. Embrace the challenge, stay curious, and keep coding!
As you continue your coding education with AlgoCademy, don’t be afraid of error messages. Use the knowledge you’ve gained here to tackle them head-on, whether you’re working through interactive coding tutorials, preparing for technical interviews, or developing your algorithmic thinking skills. With practice and persistence, you’ll soon find yourself fluent in the secret language of error messages, ready to take on any coding challenge that comes your way.
Happy coding, and may your error messages always be informative and your bugs easy to squash!