The Secret Art of Procrastinating on Your Coding Projects While Still Learning
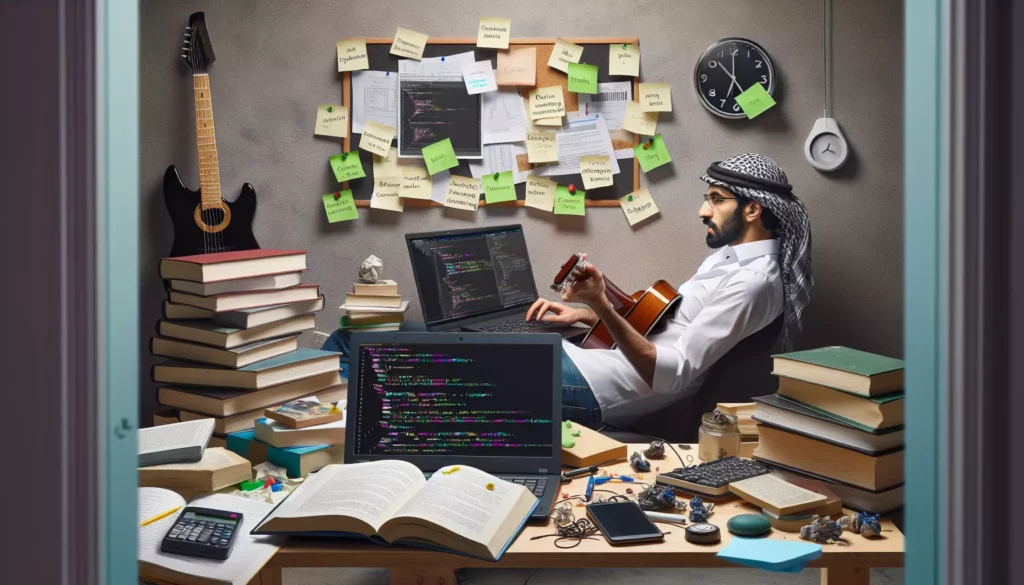
We’ve all been there. You sit down at your computer, ready to dive into that coding project you’ve been meaning to start (or finish), but suddenly, the allure of watching cat videos or reorganizing your sock drawer becomes irresistible. Welcome to the world of procrastination, a realm where coders often find themselves trapped. But what if I told you there’s a way to procrastinate on your coding projects while still learning and improving your skills? Intrigued? Let’s dive into the secret art of productive procrastination for programmers.
Understanding the Procrastination Paradox
Before we delve into the techniques of productive procrastination, it’s essential to understand why we procrastinate in the first place. Procrastination isn’t just about being lazy; it’s often rooted in perfectionism, fear of failure, or feeling overwhelmed by the task at hand. As a coder, you might find yourself putting off projects because:
- You’re intimidated by the complexity of the project
- You’re unsure where to start
- You fear your code won’t be good enough
- The project seems too time-consuming
- You’re stuck on a particular problem and don’t know how to proceed
Recognizing these reasons can help you address the root causes of your procrastination and find more productive ways to spend your time, even when you’re not actively working on your main project.
The Art of Productive Procrastination
Now that we understand why we procrastinate, let’s explore how we can turn this habit into a learning opportunity. Here are some strategies to help you procrastinate productively:
1. Explore New Programming Languages
Instead of working on your main project, why not dabble in a new programming language? This can broaden your skill set and potentially give you fresh ideas for your primary project. For instance, if you’re primarily a Python developer, you could explore JavaScript or Rust. Here’s a simple “Hello, World!” in Rust to get you started:
fn main() {
println!("Hello, World!");
}
By exploring new languages, you’re expanding your programming horizons and potentially discovering more efficient ways to tackle problems in your main project.
2. Solve Coding Challenges
Platforms like LeetCode, HackerRank, or CodeWars offer a plethora of coding challenges that can help you sharpen your problem-solving skills. These challenges often mimic the types of questions asked in technical interviews, so you’re not just procrastinating – you’re preparing for your future career!
Here’s a simple example of a coding challenge you might encounter:
def is_palindrome(s: str) -> bool:
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(c.lower() for c in s if c.isalnum())
# Check if the string is equal to its reverse
return s == s[::-1]
# Test the function
print(is_palindrome("A man, a plan, a canal: Panama")) # Should return True
print(is_palindrome("race a car")) # Should return False
By regularly solving these types of challenges, you’re building a strong foundation in algorithmic thinking and coding efficiency.
3. Contribute to Open Source Projects
Open source contributions are a fantastic way to procrastinate productively. Not only do you get to work on real-world projects, but you also collaborate with other developers and potentially make a meaningful impact. Sites like GitHub have a “good first issue” tag that can help you find beginner-friendly contributions.
Here’s how you might start contributing to an open source project:
- Find a project you’re interested in on GitHub
- Fork the repository
- Clone your fork to your local machine
- Create a new branch for your changes
- Make your changes and commit them
- Push your changes to your fork
- Create a pull request to the original repository
This process not only helps you learn about version control and collaboration but also exposes you to different coding styles and project structures.
4. Watch Coding Tutorials and Talks
YouTube, Coursera, and other platforms are treasure troves of coding knowledge. Instead of binge-watching your favorite TV show, why not binge-watch coding tutorials? You might discover new techniques, best practices, or even inspiration for your main project.
Some popular coding channels on YouTube include:
- Traversy Media
- The Coding Train
- freeCodeCamp
- Fireship
- CS Dojo
Remember, watching tutorials is most effective when you code along or immediately apply what you’ve learned to a small project.
5. Refactor Old Code
We all have that old project lurking in our GitHub repositories, filled with code that makes us cringe now. Instead of starting something new, why not revisit and refactor that old code? This practice can help you see how much you’ve improved and reinforce good coding practices.
For example, you might take this old JavaScript function:
function getSum(a, b) {
var result = a + b;
return result;
}
And refactor it to use modern ES6+ syntax:
const getSum = (a, b) => a + b;
This process of refactoring helps you internalize best practices and can often lead to more efficient, readable code.
6. Explore New Tools and Libraries
The tech world is constantly evolving, with new tools and libraries emerging all the time. Spend some time exploring these new technologies. You might find something that could significantly improve your workflow or inspire new project ideas.
For instance, if you’re a front-end developer, you might explore the latest features of React or try out a new state management library like Recoil:
import { atom, useRecoilState } from 'recoil';
const countState = atom({
key: 'countState',
default: 0,
});
function Counter() {
const [count, setCount] = useRecoilState(countState);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
By staying up-to-date with the latest tools and libraries, you’re ensuring that your skills remain relevant and marketable.
7. Write Technical Blog Posts
One of the best ways to solidify your understanding of a concept is to explain it to others. Start a technical blog where you write about coding concepts, project walkthroughs, or your experiences as a developer. This not only helps you learn but also builds your personal brand and can lead to networking opportunities.
Here’s a simple template for a technical blog post:
- Introduction: What problem are you addressing?
- Background: Any necessary context or prerequisites
- Main Content: Step-by-step explanation or tutorial
- Code Examples: Practical demonstrations
- Conclusion: Recap and next steps
- Further Resources: Links for readers to learn more
Remember, the act of organizing your thoughts and explaining concepts in writing can significantly enhance your own understanding.
8. Participate in Coding Forums and Communities
Platforms like Stack Overflow, Reddit’s programming subreddits, or Discord coding channels are great places to procrastinate productively. By answering questions or engaging in discussions, you’re reinforcing your own knowledge and potentially learning new things from others in the community.
Here are some tips for effective participation in coding communities:
- Be respectful and follow community guidelines
- Provide detailed, well-explained answers
- Include code examples when relevant
- Be open to feedback and corrections
- Ask thoughtful questions when you need help
Remember, even if you’re not an expert, explaining what you do know can help solidify your understanding and identify areas where you need to learn more.
9. Set Up a Better Development Environment
Sometimes, procrastination can be turned into productivity by improving your tools and workflow. Spend some time optimizing your development environment. This could involve:
- Customizing your IDE or text editor
- Setting up helpful aliases and scripts
- Configuring your terminal for better productivity
- Exploring new productivity tools or extensions
For example, you might set up some Git aliases to streamline your workflow:
git config --global alias.co checkout
git config --global alias.br branch
git config --global alias.ci commit
git config --global alias.st status
These small improvements can add up to significant time savings and a more enjoyable coding experience in the long run.
10. Practice Typing and Shortcuts
Improving your typing speed and mastering keyboard shortcuts can significantly boost your coding efficiency. Websites like TypeRacer or 10FastFingers offer coding-specific typing practice. Additionally, take some time to learn and practice the keyboard shortcuts for your IDE or text editor.
Here are some common VS Code shortcuts to get you started:
- Ctrl+P (Cmd+P on Mac): Quick Open, Go to File
- Ctrl+Shift+P (Cmd+Shift+P on Mac): Show Command Palette
- Ctrl+/ (Cmd+/ on Mac): Toggle line comment
- Alt+Up/Down (Option+Up/Down on Mac): Move line up/down
- Ctrl+D (Cmd+D on Mac): Select next occurrence of current word
Mastering these shortcuts can save you countless hours over the course of your coding career.
Balancing Productive Procrastination with Project Progress
While these strategies can help you learn and improve your skills even when you’re procrastinating, it’s important to remember that they shouldn’t completely replace work on your main projects. The key is to find a balance between productive procrastination and focused project work.
Here are some tips to help you strike that balance:
1. Use the Pomodoro Technique
The Pomodoro Technique involves working in focused 25-minute intervals (called “Pomodoros”), followed by short breaks. You could use this technique to alternate between your main project and productive procrastination activities. For example:
- Pomodoro 1: Work on your main project
- Break: 5 minutes
- Pomodoro 2: Solve a coding challenge
- Break: 5 minutes
- Pomodoro 3: Back to the main project
- And so on…
This approach helps you make progress on your project while still allowing time for other learning activities.
2. Set Clear Goals and Deadlines
For both your main project and your productive procrastination activities, set clear, achievable goals and deadlines. This can help you stay motivated and ensure that you’re making progress in all areas. For example:
- Main Project: Complete the user authentication system by Friday
- Productive Procrastination: Solve three LeetCode problems this week
- Learning Goal: Watch two React tutorial videos by Wednesday
By setting these goals, you create a structure that allows for both focused work and productive exploration.
3. Use a Project Management Tool
Tools like Trello, Asana, or even a simple Kanban board can help you visualize your tasks and progress. Create separate columns for your main project tasks and your productive procrastination activities. This can help you ensure you’re maintaining a healthy balance between the two.
4. Reflect and Adjust
Regularly reflect on how you’re spending your time and whether your productive procrastination is actually benefiting your overall growth as a developer. Are you learning new skills that could be applied to your main project? Are you making enough progress on your primary goals? Be honest with yourself and adjust your approach as needed.
Conclusion: Embracing Productive Procrastination
Procrastination doesn’t have to be the enemy of productivity. By channeling your procrastination tendencies into activities that enhance your coding skills and knowledge, you can turn what was once wasted time into valuable learning experiences. The secret art of procrastinating on your coding projects while still learning is all about finding creative ways to engage with programming concepts, even when you’re not ready to tackle your main project.
Remember, the goal isn’t to avoid your primary project indefinitely, but to create a balanced approach to coding that allows for both focused project work and exploratory learning. By incorporating these productive procrastination techniques into your routine, you can ensure that even your “off” time is contributing to your growth as a developer.
So the next time you find yourself procrastinating, don’t beat yourself up about it. Instead, choose one of these productive activities and make the most of your procrastination time. Who knows? The skills you pick up during these “productive procrastination” sessions might just be the key to unlocking a solution in your main project.
Happy coding, and may your procrastination always be productive!