The Science of Asking Yourself the Right Questions Before Writing a Line of Code
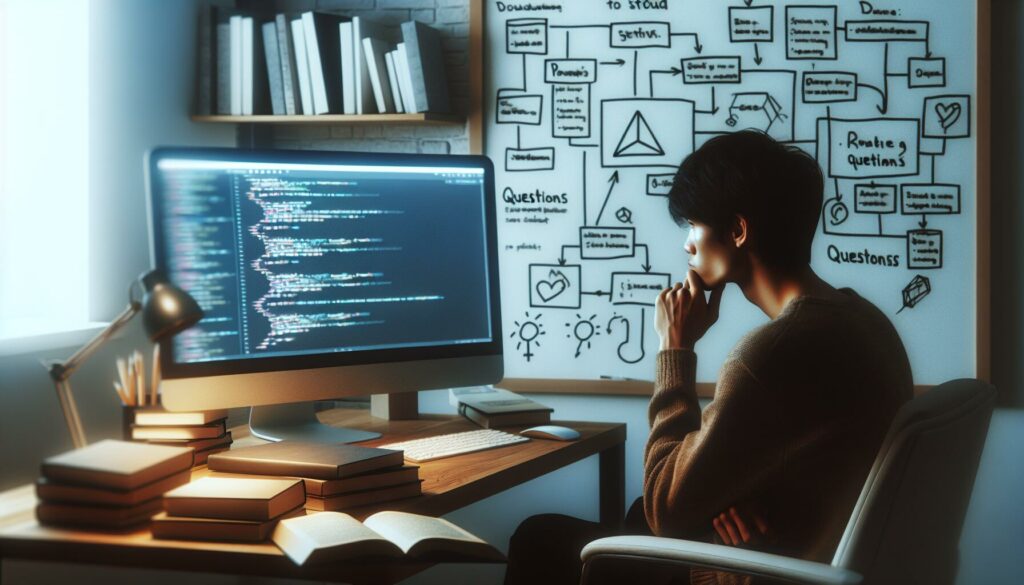
In the world of programming, it’s easy to get caught up in the excitement of diving straight into coding. However, experienced developers know that the key to efficient and effective programming lies in the preparation phase. This crucial step involves asking yourself the right questions before you even write a single line of code. In this comprehensive guide, we’ll explore the science behind this approach and how it can dramatically improve your coding skills, especially when tackling complex algorithms and preparing for technical interviews at top tech companies.
Why Questioning Matters in Programming
Before we delve into the specific questions you should ask, let’s understand why this process is so important:
- Clarity of Purpose: Questions help you clarify the problem you’re trying to solve, ensuring you don’t waste time on unnecessary features.
- Efficiency: By addressing potential issues upfront, you can avoid time-consuming rewrites and debugging sessions later.
- Better Design: Thoughtful questions lead to better-structured code that’s easier to maintain and scale.
- Problem-Solving Skills: This practice enhances your analytical thinking, a crucial skill for technical interviews and real-world programming challenges.
The Right Questions to Ask
Let’s break down the key questions you should ask yourself, categorized by different aspects of the programming process:
1. Understanding the Problem
- What exactly am I trying to achieve with this code?
- Can I explain the problem in simple terms?
- What are the inputs and expected outputs?
- Are there any constraints or edge cases I need to consider?
Example: If you’re tasked with creating a function to find the longest substring without repeating characters, you might ask:
- What should the function return if the string is empty?
- How should I handle uppercase and lowercase characters?
- What's the maximum possible length of the input string?
2. Planning the Approach
- What algorithms or data structures might be useful for this problem?
- Can I break this problem down into smaller, manageable sub-problems?
- Is there more than one way to solve this? What are the trade-offs?
For the longest substring problem, you might consider:
- Would a sliding window approach work here?
- Should I use a hash set to keep track of characters?
- Is there a way to optimize this for space complexity?
3. Considering Efficiency
- What’s the time complexity of my proposed solution?
- Is there a more efficient algorithm I could use?
- How does the space complexity look?
Efficiency questions for our example might include:
- Can I achieve O(n) time complexity?
- Is it possible to solve this with constant space?
- How would my solution perform with very large input strings?
4. Anticipating Edge Cases
- What are the possible edge cases for this problem?
- How should my code handle unexpected inputs?
- Are there any potential overflow or underflow scenarios?
For the substring problem, consider:
- How should the function behave with an empty string input?
- What if all characters in the string are the same?
- How about strings with non-ASCII characters?
5. Testing Strategy
- What test cases should I use to validate my solution?
- How can I ensure my code handles all the edge cases?
- What kind of stress testing might be useful?
Testing considerations:
- Should I create test cases for strings of various lengths?
- How can I test for performance with very long strings?
- What about testing with strings containing special characters?
The Science Behind the Questions
The process of asking these questions isn’t just a best practice—it’s grounded in cognitive science and problem-solving theory. Here’s why it works:
Activating Prior Knowledge
When you ask yourself questions, you’re actively recalling and applying your existing knowledge. This process, known as retrieval practice, strengthens your understanding and helps you make connections between different concepts.
Metacognition
Questioning is a form of metacognition—thinking about your thinking. This higher-order cognitive process helps you monitor your understanding and identify gaps in your knowledge, leading to more effective learning and problem-solving.
Reducing Cognitive Load
By breaking down the problem and addressing potential issues upfront, you’re reducing the cognitive load when you actually start coding. This allows you to focus more on implementation details rather than grappling with the problem itself.
Fostering Creativity
Open-ended questions can stimulate creative thinking, helping you come up with novel solutions or approaches you might not have considered otherwise.
Implementing the Questioning Process
Now that we understand the importance and science behind asking the right questions, let’s look at how to implement this process effectively:
1. Create a Pre-Coding Checklist
Develop a personalized checklist of questions to go through before you start coding. This might include:
- Problem understanding questions
- Algorithm selection questions
- Efficiency considerations
- Edge case identification
- Testing strategy questions
2. Use the Rubber Duck Method
Explain your problem and proposed solution to an inanimate object (like a rubber duck) or an imaginary person. This process often helps you identify gaps in your understanding or logic.
3. Write Pseudocode
Before diving into actual code, write out your algorithm in plain language. This can help you spot potential issues and refine your approach.
4. Time-Box Your Questioning Phase
Set a specific amount of time for this pre-coding phase to ensure you don’t get stuck in analysis paralysis. For smaller problems, 5-10 minutes might suffice, while more complex issues might require 20-30 minutes.
5. Document Your Questions and Answers
Keep a record of the questions you ask and the answers you come up with. This can be invaluable for future reference and for explaining your thought process in interviews.
Applying This Approach to Technical Interviews
The questioning technique is particularly valuable when preparing for technical interviews at top tech companies. Here’s how to leverage it:
1. Practice with Real Interview Questions
Use platforms like LeetCode, HackerRank, or AlgoCademy to access real interview questions. Before coding, go through your questioning process for each problem.
2. Verbalize Your Thought Process
In mock interviews or when practicing with a friend, verbalize your questions and thought process. This mimics the actual interview scenario where you’ll need to communicate your approach.
3. Time Your Questioning Phase
In interviews, time is limited. Practice efficiently going through your questioning process within 2-3 minutes before starting to code.
4. Anticipate Interviewer Questions
Try to predict what questions an interviewer might ask about your approach. This helps you be better prepared to explain and justify your decisions.
5. Use Questions to Guide Your Problem-Solving
If you’re stuck during an interview, fall back on your questioning technique. It can help you break down the problem and approach it systematically.
Common Pitfalls to Avoid
While asking questions is crucial, there are some pitfalls to be aware of:
1. Analysis Paralysis
Don’t get stuck in an endless loop of questioning. Set a time limit and be prepared to move forward with the best information you have.
2. Ignoring Intuition
While systematic questioning is important, don’t completely disregard your intuitive understanding or experience. Sometimes, your initial instincts can guide you to efficient solutions.
3. Overlooking Simple Solutions
In your quest to anticipate all scenarios, don’t overcomplicate things. Sometimes, the simplest solution is the best.
4. Failing to Prioritize
Not all questions are equally important. Learn to prioritize questions that have the most significant impact on your solution.
5. Neglecting to Act on Answers
Asking questions is only valuable if you use the insights gained. Make sure to incorporate what you learn into your coding approach.
Tools and Techniques to Enhance Your Questioning Skills
To further refine your ability to ask the right questions, consider these tools and techniques:
1. Mind Mapping
Use mind mapping tools to visually organize your thoughts and questions. This can help you see connections and identify areas that need more consideration.
2. The Five Whys Technique
Originated by Sakichi Toyoda, this technique involves asking “Why?” five times to get to the root of a problem or to deeply understand a concept.
3. SWOT Analysis
While typically used in business, a SWOT (Strengths, Weaknesses, Opportunities, Threats) analysis can be adapted to evaluate different coding approaches.
4. Decision Matrices
When faced with multiple possible solutions, use a decision matrix to objectively compare them based on criteria like efficiency, readability, and maintainability.
5. Peer Review
Regularly engage in peer review sessions where you present your questions and approach to colleagues. Their input can help you identify blind spots in your questioning process.
Conclusion
The science of asking yourself the right questions before writing code is a powerful tool in any programmer’s arsenal. It’s not just about avoiding errors or writing efficient code; it’s about developing a mindset that approaches problems systematically and thoughtfully. This skill is invaluable whether you’re working on personal projects, tackling complex algorithms, or preparing for technical interviews at top tech companies.
By incorporating this questioning process into your coding routine, you’re not just improving your code—you’re enhancing your overall problem-solving abilities and analytical thinking. Remember, the best programmers aren’t just those who can write code quickly, but those who can think deeply about problems and approach them strategically.
As you continue to develop your skills on platforms like AlgoCademy, make the pre-coding questioning phase an integral part of your learning process. With practice, you’ll find that this approach not only improves the quality of your code but also boosts your confidence in tackling even the most challenging programming problems.
So, the next time you’re faced with a coding challenge, take a step back, ask the right questions, and watch as your solutions become more elegant, efficient, and effective. Happy coding!