The Science of Algorithm Benchmarking: Measuring Performance and Efficiency in Code
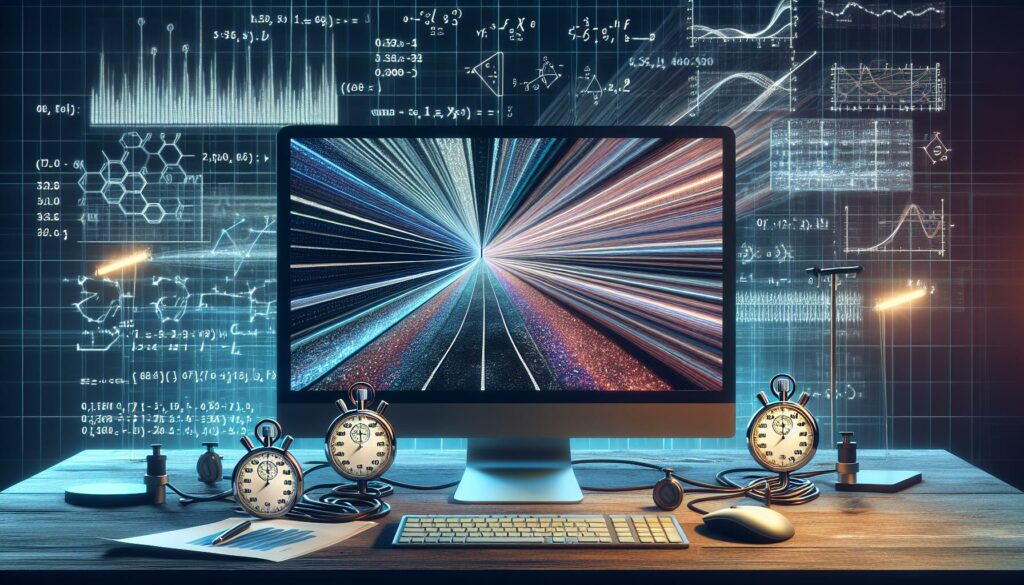
In the world of computer science and software engineering, algorithms are the building blocks of efficient and effective programs. As developers, we strive to create algorithms that not only solve problems correctly but also do so in the most optimal way possible. This is where the science of algorithm benchmarking comes into play. By systematically measuring and comparing the performance of different algorithms, we can make informed decisions about which solutions are best suited for specific problems and contexts.
In this comprehensive guide, we’ll explore the intricacies of algorithm benchmarking, its importance in coding education and skills development, and how it relates to preparing for technical interviews at major tech companies. We’ll delve into the methods, tools, and best practices used in benchmarking, and discuss how this knowledge can be applied to improve your coding skills and advance your career in the tech industry.
Understanding Algorithm Benchmarking
Algorithm benchmarking is the process of measuring and comparing the performance of different algorithms in terms of various metrics such as time complexity, space complexity, and efficiency. This practice is crucial for several reasons:
- Identifying the most efficient solution for a given problem
- Understanding the trade-offs between different algorithmic approaches
- Optimizing code for better performance
- Predicting how algorithms will scale with larger inputs
- Making informed decisions in algorithm selection for real-world applications
To effectively benchmark algorithms, we need to consider various factors and follow a structured approach. Let’s break down the key components of algorithm benchmarking.
Key Metrics in Algorithm Benchmarking
When benchmarking algorithms, several metrics are commonly used to evaluate performance:
1. Time Complexity
Time complexity is a measure of how the runtime of an algorithm grows as the input size increases. It’s typically expressed using Big O notation, which provides an upper bound on the growth rate of the algorithm’s runtime.
For example, an algorithm with O(n) time complexity will have a linear growth rate, while an algorithm with O(n^2) will grow quadratically. Common time complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
2. Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s often expressed using Big O notation. Common space complexities include:
- O(1) – Constant space
- O(n) – Linear space
- O(n^2) – Quadratic space
3. Execution Time
While time complexity provides a theoretical measure of an algorithm’s performance, actual execution time is a practical metric that can be measured directly. This is typically done by running the algorithm multiple times with various input sizes and recording the time taken for each run.
4. Memory Usage
Similar to execution time, actual memory usage can be measured to provide a concrete understanding of an algorithm’s space requirements in practice.
5. Scalability
Scalability measures how well an algorithm’s performance holds up as the input size grows. This is particularly important for algorithms that need to handle large datasets or high-traffic scenarios.
Benchmarking Techniques and Tools
To effectively benchmark algorithms, developers use a variety of techniques and tools. Here are some common approaches:
1. Manual Timing
The simplest form of benchmarking involves manually timing the execution of an algorithm using built-in language features or system clocks. While not the most precise method, it can provide a quick estimate of performance.
Here’s an example in Python:
import time
def algorithm_to_benchmark(input_data):
# Algorithm implementation here
pass
start_time = time.time()
result = algorithm_to_benchmark(input_data)
end_time = time.time()
execution_time = end_time - start_time
print(f"Execution time: {execution_time} seconds")
2. Profiling Tools
Most programming languages have built-in or third-party profiling tools that can provide detailed information about code execution, including time spent in different functions, memory usage, and more. For example:
- Python: cProfile, line_profiler
- Java: JProfiler, YourKit
- C++: Valgrind, gprof
- JavaScript: Chrome DevTools Profiler
3. Benchmarking Frameworks
Specialized benchmarking frameworks exist for various programming languages, providing standardized ways to measure and compare algorithm performance. Some popular ones include:
- Python: timeit, pytest-benchmark
- Java: JMH (Java Microbenchmark Harness)
- C++: Google Benchmark
- JavaScript: Benchmark.js
These frameworks often handle the complexities of accurate timing, statistical analysis, and reporting, making it easier to conduct reliable benchmarks.
4. Big O Analysis
While not a direct measurement technique, analyzing the Big O complexity of algorithms is crucial for understanding their theoretical performance characteristics. This involves examining the algorithm’s structure and determining how its runtime or space usage grows with input size.
Best Practices for Algorithm Benchmarking
To ensure accurate and meaningful benchmarks, consider the following best practices:
1. Use Realistic Inputs
Test your algorithms with input data that closely resembles what they’ll encounter in real-world scenarios. This includes varying input sizes and considering edge cases.
2. Repeat Measurements
Run your benchmarks multiple times to account for variations in system performance and obtain statistically significant results.
3. Control the Environment
Minimize interference from other processes or background tasks that could affect benchmark results. Run benchmarks on a consistent hardware and software configuration.
4. Consider Different Input Sizes
Test algorithms with various input sizes to understand how they scale. This is particularly important for identifying performance differences that may not be apparent with small inputs.
5. Compare Apples to Apples
When comparing different algorithms, ensure they’re solving the same problem and producing equivalent outputs. Be aware of any trade-offs between time and space complexity.
6. Use Appropriate Metrics
Choose benchmarking metrics that are relevant to your specific use case. For example, if memory usage is a critical concern, focus on space complexity and actual memory consumption.
Algorithm Benchmarking in Coding Education
Understanding and applying algorithm benchmarking techniques is crucial for developing strong coding skills and preparing for technical interviews, especially when targeting positions at major tech companies. Here’s how benchmarking fits into coding education:
1. Developing Algorithmic Thinking
By benchmarking different algorithms for the same problem, students can develop a deeper understanding of algorithmic efficiency and the trade-offs involved in different approaches. This fosters critical thinking and problem-solving skills.
2. Optimizing Code
Benchmarking helps identify performance bottlenecks in code, encouraging students to optimize their solutions. This skill is invaluable in real-world software development scenarios.
3. Preparing for Technical Interviews
Many technical interviews, especially at FAANG companies, involve discussing the time and space complexity of proposed solutions. Being familiar with benchmarking concepts and Big O notation is crucial for success in these interviews.
4. Understanding Scalability
Benchmarking different input sizes helps students grasp how algorithms scale, which is essential for designing systems that can handle large-scale data and high traffic.
5. Practical Application of Theoretical Concepts
Benchmarking bridges the gap between theoretical computer science concepts and practical programming. It allows students to see firsthand how asymptotic analysis translates to real-world performance.
Benchmarking in Practice: A Case Study
Let’s examine a practical example of algorithm benchmarking by comparing two sorting algorithms: Bubble Sort and Merge Sort. We’ll implement these algorithms in Python and use the timeit
module for benchmarking.
import random
import timeit
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Benchmarking function
def benchmark_sorting(sort_func, arr):
return timeit.timeit(lambda: sort_func(arr.copy()), number=1)
# Generate random arrays of different sizes
sizes = [100, 1000, 10000]
arrays = {size: [random.randint(1, 1000) for _ in range(size)] for size in sizes}
# Run benchmarks
for size, arr in arrays.items():
bubble_time = benchmark_sorting(bubble_sort, arr)
merge_time = benchmark_sorting(merge_sort, arr)
print(f"Array size: {size}")
print(f"Bubble Sort time: {bubble_time:.6f} seconds")
print(f"Merge Sort time: {merge_time:.6f} seconds")
print(f"Merge Sort is {bubble_time / merge_time:.2f}x faster")
print()
This script compares the performance of Bubble Sort (O(n^2) time complexity) with Merge Sort (O(n log n) time complexity) for different input sizes. The results might look something like this:
Array size: 100
Bubble Sort time: 0.000302 seconds
Merge Sort time: 0.000201 seconds
Merge Sort is 1.50x faster
Array size: 1000
Bubble Sort time: 0.031245 seconds
Merge Sort time: 0.002514 seconds
Merge Sort is 12.43x faster
Array size: 10000
Bubble Sort time: 3.152687 seconds
Merge Sort time: 0.030125 seconds
Merge Sort is 104.65x faster
This benchmark clearly demonstrates how Merge Sort’s superior time complexity translates to significantly better performance, especially as the input size grows. It also illustrates the importance of choosing the right algorithm for larger datasets.
Advanced Benchmarking Considerations
As you delve deeper into algorithm benchmarking, consider these advanced topics:
1. Amortized Analysis
Some data structures and algorithms have operations that occasionally take longer but are balanced out by more frequent, faster operations. Amortized analysis considers the average performance over a sequence of operations, providing a more accurate picture of real-world performance.
2. Cache Efficiency
Modern computer architectures rely heavily on caching to improve performance. Algorithms that exhibit good cache locality (accessing memory locations that are close together) can significantly outperform those that don’t, even if their theoretical time complexity is worse.
3. Parallel and Distributed Algorithms
With the prevalence of multi-core processors and distributed systems, benchmarking parallel and distributed algorithms introduces new challenges and considerations, such as communication overhead and load balancing.
4. Algorithm Portfolios
In some cases, the best approach is to have a portfolio of algorithms and choose the most appropriate one based on input characteristics or runtime conditions. Benchmarking can help determine the optimal selection criteria.
5. Energy Efficiency
As energy consumption becomes an increasingly important concern, especially in mobile and cloud computing, benchmarking algorithms for energy efficiency is gaining prominence.
Conclusion
Algorithm benchmarking is a crucial skill for any serious programmer or computer scientist. It provides the tools and methodologies needed to objectively compare different algorithmic approaches, optimize code performance, and make informed decisions in algorithm selection.
For students and professionals aiming to excel in coding interviews and land positions at top tech companies, a solid understanding of benchmarking techniques is invaluable. It not only helps in solving coding challenges efficiently but also demonstrates a deep understanding of algorithmic performance and optimization—qualities highly valued in the industry.
As you continue your journey in coding education and skills development, make algorithm benchmarking an integral part of your learning process. Practice implementing and benchmarking different algorithms, analyze their performance characteristics, and strive to understand the underlying reasons for performance differences. This approach will not only make you a better problem solver but also a more effective and efficient programmer in real-world scenarios.
Remember, the goal of benchmarking is not just to find the fastest algorithm but to understand the trade-offs involved and make informed decisions based on specific requirements and constraints. By mastering the science of algorithm benchmarking, you’ll be well-equipped to tackle complex coding challenges, optimize software systems, and excel in your programming career.