The Role of Test Cases in Verifying Your Solution: A Comprehensive Guide
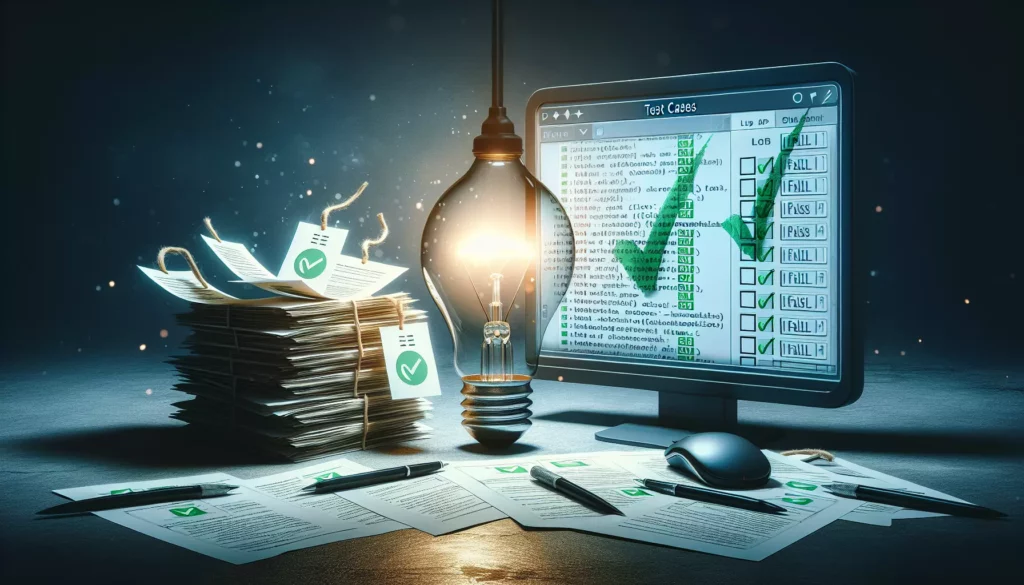
In the world of software development and coding interviews, the importance of test cases cannot be overstated. They serve as a crucial tool for verifying the correctness, efficiency, and robustness of your solutions. Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at top tech companies, understanding the role of test cases is essential for your success. In this comprehensive guide, we’ll explore the significance of test cases, how to create them, and how they can help you become a better programmer.
What Are Test Cases?
Test cases are specific scenarios or inputs designed to verify that a piece of code or a function behaves correctly under various conditions. They are an essential part of the software development process and play a crucial role in ensuring the quality and reliability of your code. Test cases typically consist of:
- Input data
- Expected output or behavior
- Steps to execute the test
- Preconditions (if any)
- Post-conditions (if any)
In the context of coding interviews and algorithmic problem-solving, test cases are often used to validate your solution against different scenarios, including edge cases and corner cases.
The Importance of Test Cases in Coding
Test cases serve several crucial purposes in the coding process:
1. Verifying Correctness
The primary purpose of test cases is to ensure that your code produces the correct output for various inputs. By running your solution against a set of well-designed test cases, you can quickly identify if your code is working as expected or if there are any bugs or logical errors.
2. Catching Edge Cases
Edge cases are scenarios that occur at the extreme ends of the input range or involve boundary conditions. Test cases help you identify and handle these edge cases, which are often overlooked during the initial implementation.
3. Improving Code Quality
Writing test cases encourages you to think critically about your code and consider different scenarios. This process often leads to more robust and well-structured solutions.
4. Facilitating Debugging
When your code fails a test case, it provides valuable information about where the problem might be, making it easier to debug and fix issues.
5. Enabling Refactoring
With a comprehensive set of test cases, you can confidently refactor your code to improve its efficiency or readability, knowing that you can quickly verify if the changes have introduced any bugs.
6. Demonstrating Understanding
In coding interviews, creating and discussing test cases demonstrates your thorough understanding of the problem and your attention to detail, which are highly valued skills in the software development industry.
Types of Test Cases
When verifying your solution, it’s important to consider different types of test cases:
1. Normal Cases
These are typical scenarios that cover the expected behavior of your code under normal conditions. They verify that your solution works correctly for common inputs.
2. Edge Cases
Edge cases test your code’s behavior at the boundaries of input ranges or under extreme conditions. Examples include:
- Empty inputs (e.g., empty strings, empty arrays)
- Minimum or maximum possible values
- Single-element inputs
3. Corner Cases
Corner cases are special scenarios that may not be immediately obvious but are still valid inputs. These often combine multiple edge cases or involve unique situations specific to the problem.
4. Error Cases
Error cases test how your code handles invalid inputs or unexpected situations. They ensure that your solution gracefully handles errors and doesn’t crash or produce unexpected results.
5. Performance Cases
These test cases focus on the efficiency of your solution, often involving large inputs or repeated operations to evaluate time and space complexity.
Creating Effective Test Cases
To create effective test cases for verifying your solution, follow these steps:
1. Analyze the Problem
Thoroughly understand the problem statement, including any constraints, input ranges, and expected outputs.
2. Identify Key Scenarios
Determine the main scenarios your solution needs to handle, including normal cases, edge cases, and potential error conditions.
3. Design Input-Output Pairs
Create specific input-output pairs for each scenario you’ve identified. Ensure that these pairs cover a wide range of possible inputs and expected outputs.
4. Consider Edge Cases
Pay special attention to edge cases and corner cases. Think about extreme values, empty inputs, and boundary conditions.
5. Include Error Handling
If applicable, create test cases for invalid inputs or error conditions to ensure your code handles them appropriately.
6. Test for Performance
If the problem has specific time or space complexity requirements, create test cases with large inputs to verify your solution’s efficiency.
7. Organize and Document
Organize your test cases in a clear and logical manner. Provide brief descriptions or comments for each test case to explain its purpose.
Implementing Test Cases in Your Code
Once you’ve created your test cases, it’s time to implement them in your code. Here’s an example of how you might structure your test cases using Python:
def solution(input_data):
# Your implementation here
pass
def run_tests():
test_cases = [
{
"input": [1, 2, 3],
"expected_output": 6,
"description": "Normal case: sum of positive integers"
},
{
"input": [],
"expected_output": 0,
"description": "Edge case: empty array"
},
{
"input": [-1, 0, 1],
"expected_output": 0,
"description": "Corner case: array with negative, zero, and positive integers"
},
# Add more test cases here
]
for i, test_case in enumerate(test_cases):
result = solution(test_case["input"])
if result == test_case["expected_output"]:
print(f"Test case {i + 1} passed: {test_case['description']}")
else:
print(f"Test case {i + 1} failed: {test_case['description']}")
print(f"Expected {test_case['expected_output']}, but got {result}")
if __name__ == "__main__":
run_tests()
This structure allows you to easily add new test cases and run them all at once to verify your solution.
Best Practices for Using Test Cases
To make the most of test cases in verifying your solution, consider the following best practices:
1. Start with Simple Cases
Begin with simple, normal cases to ensure your basic implementation is correct before moving on to more complex scenarios.
2. Incrementally Add Complexity
Gradually introduce more complex test cases, including edge cases and corner cases, to thoroughly test your solution.
3. Use a Variety of Inputs
Ensure your test cases cover a wide range of possible inputs, including different data types, sizes, and values.
4. Test Both Valid and Invalid Inputs
Include test cases for both valid and invalid inputs to verify that your solution handles errors appropriately.
5. Automate Test Execution
Use automated testing frameworks or scripts to run your test cases efficiently and consistently.
6. Regularly Update Test Cases
As you refine your solution or discover new edge cases, update your test suite accordingly.
7. Use Test-Driven Development (TDD)
Consider writing test cases before implementing your solution, following the principles of Test-Driven Development.
The Role of Test Cases in Coding Interviews
In coding interviews, particularly for positions at top tech companies, test cases play a crucial role in both the problem-solving process and the evaluation of candidates. Here’s how test cases are typically used in interview settings:
1. Problem Understanding
Interviewers often provide initial test cases to help you understand the problem requirements and expected behavior of the solution.
2. Solution Verification
As you develop your solution, you’ll be expected to run it against the provided test cases and any additional cases you create.
3. Edge Case Identification
Interviewers will assess your ability to identify and handle edge cases, often by asking you to come up with additional test cases.
4. Code Quality Evaluation
Your approach to creating and using test cases demonstrates your attention to detail and commitment to code quality.
5. Problem-Solving Skills
The way you use test cases to debug and refine your solution showcases your problem-solving skills and systematic approach.
6. Communication
Discussing test cases with your interviewer allows you to demonstrate your ability to communicate technical concepts clearly.
Advanced Test Case Techniques
As you become more proficient in using test cases, consider these advanced techniques to further improve your skills:
1. Parameterized Tests
Use parameterized testing to run the same test logic with different inputs, reducing code duplication and making it easier to add new test cases.
2. Property-Based Testing
Implement property-based testing to automatically generate a large number of test cases based on specified properties or invariants of your solution.
3. Mutation Testing
Use mutation testing tools to evaluate the quality of your test cases by introducing small changes (mutations) to your code and checking if the tests catch these changes.
4. Fuzz Testing
Employ fuzz testing techniques to generate random or semi-random inputs to stress-test your solution and uncover potential vulnerabilities or edge cases.
5. Coverage Analysis
Use code coverage tools to identify parts of your code that are not adequately covered by your test cases, helping you improve your test suite.
Conclusion
Test cases are an indispensable tool in the software development process and play a crucial role in coding interviews. By mastering the art of creating and using effective test cases, you’ll not only improve the quality and reliability of your code but also demonstrate your skills as a thorough and methodical programmer.
Remember that the process of creating and running test cases is not just about catching bugs; it’s about developing a systematic approach to problem-solving and code verification. This skill will serve you well throughout your coding journey, from learning new concepts to acing technical interviews at top tech companies.
As you continue to practice and refine your coding skills, make test cases an integral part of your problem-solving routine. Embrace the challenge of creating comprehensive test suites, and you’ll find that your solutions become more robust, your debugging skills sharpen, and your confidence as a programmer grows.
By leveraging the power of test cases, you’ll be well-equipped to tackle complex coding challenges, excel in technical interviews, and ultimately succeed in your software development career. So, the next time you sit down to solve a coding problem, remember the crucial role that test cases play in verifying your solution and take the time to create a thorough and effective test suite. Your future self (and your code) will thank you for it!