The Role of Pseudocode in Problem Solving: A Comprehensive Guide
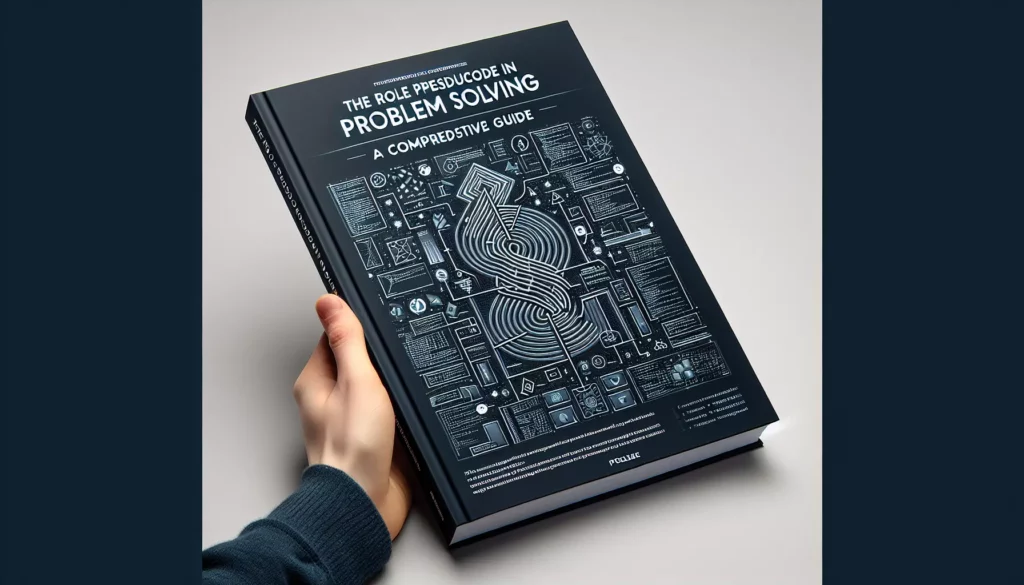
In the world of programming and software development, problem-solving is a crucial skill that separates great developers from the rest. One of the most effective tools in a programmer’s arsenal for tackling complex problems is pseudocode. This powerful technique bridges the gap between human thinking and computer code, allowing developers to plan and structure their solutions before diving into actual coding. In this comprehensive guide, we’ll explore the role of pseudocode in problem-solving, its benefits, and how to use it effectively in your coding journey.
What is Pseudocode?
Pseudocode is a informal, high-level description of a computer program or algorithm. It uses structural conventions of a normal programming language, but is intended for human reading rather than machine reading. Essentially, it’s a way of planning out your code in plain language before writing it in a specific programming language.
The beauty of pseudocode lies in its flexibility. It doesn’t adhere to the strict syntax of any particular programming language, allowing developers to focus on the logic and structure of their solution without getting bogged down in language-specific details.
The Importance of Pseudocode in Problem Solving
Pseudocode plays a crucial role in the problem-solving process for several reasons:
- Clarifies thinking: Writing pseudocode forces you to think through your solution step-by-step, helping to identify potential issues or gaps in your logic early in the process.
- Improves communication: Pseudocode can be easily understood by other developers, making it an excellent tool for discussing and sharing ideas within a team.
- Facilitates planning: It allows you to outline the structure and flow of your program before committing to a specific implementation.
- Saves time: By identifying potential issues early, pseudocode can save significant time in the actual coding and debugging phases.
- Language-independent: Pseudocode is not tied to any specific programming language, making it a versatile tool for problem-solving across different platforms and technologies.
How to Write Effective Pseudocode
While there’s no strict syntax for pseudocode, following some general guidelines can make your pseudocode more effective:
- Use clear, concise language: Write in plain English (or your preferred language) using simple, straightforward statements.
- Be consistent: Use consistent terminology and structure throughout your pseudocode.
- Use indentation: Properly indent your pseudocode to show the structure and nesting of your algorithm.
- Keep it high-level: Focus on the overall logic and flow rather than getting bogged down in low-level details.
- Use common programming constructs: Incorporate familiar programming concepts like loops, conditionals, and functions in your pseudocode.
Example of Pseudocode in Action
Let’s look at an example of how pseudocode can be used to solve a common programming problem: finding the largest number in an array.
FUNCTION find_largest_number(numbers):
SET largest = first number in the array
FOR EACH number in the array:
IF number > largest THEN
SET largest = number
END IF
END FOR
RETURN largest
END FUNCTION
This pseudocode outlines the basic logic for finding the largest number in an array. It’s easy to understand and can be translated into any programming language. Let’s see how this might look in Python:
def find_largest_number(numbers):
largest = numbers[0]
for number in numbers:
if number > largest:
largest = number
return largest
As you can see, the Python code closely follows the structure laid out in the pseudocode, demonstrating how pseudocode can serve as a blueprint for your actual code.
Pseudocode in Different Programming Paradigms
Pseudocode can be adapted to fit different programming paradigms. Let’s look at how the same problem might be approached using different paradigms:
Object-Oriented Approach
CLASS NumberArray
PROPERTY numbers
CONSTRUCTOR(numbers)
SET this.numbers = numbers
METHOD find_largest()
SET largest = first number in this.numbers
FOR EACH number in this.numbers
IF number > largest THEN
SET largest = number
END IF
END FOR
RETURN largest
END CLASS
Functional Approach
FUNCTION find_largest_number(numbers)
RETURN REDUCE numbers WITH (accumulator, current) =>
IF current > accumulator THEN
RETURN current
ELSE
RETURN accumulator
END IF
STARTING WITH first number in numbers
END FUNCTION
These examples demonstrate how pseudocode can be adapted to reflect different programming styles and approaches, making it a versatile tool for problem-solving across various paradigms.
Pseudocode in Technical Interviews
Pseudocode is particularly valuable in technical interviews, especially when applying for positions at major tech companies like those in the FAANG group (Facebook, Amazon, Apple, Netflix, Google). Here’s why:
- Demonstrates problem-solving skills: Using pseudocode shows interviewers that you can break down complex problems into manageable steps.
- Facilitates discussion: Pseudocode allows you to quickly sketch out your approach, making it easier to discuss and refine your solution with the interviewer.
- Reduces pressure: Starting with pseudocode can help alleviate the pressure of having to write perfect, syntactically correct code right away.
- Shows planning ability: Using pseudocode demonstrates that you plan before you code, a valuable skill in professional software development.
Common Pitfalls to Avoid When Using Pseudocode
While pseudocode is a powerful tool, there are some common mistakes to avoid:
- Being too vague: While pseudocode should be high-level, it shouldn’t be so vague that it doesn’t provide a clear path to implementation.
- Being too detailed: On the flip side, getting bogged down in too much detail defeats the purpose of pseudocode. Strike a balance between clarity and brevity.
- Ignoring edge cases: Even in pseudocode, it’s important to consider and account for potential edge cases in your algorithm.
- Not considering efficiency: While pseudocode doesn’t need to be optimized, it’s good practice to think about efficiency even at this stage.
- Forgetting to update: If you make changes to your implementation, remember to update your pseudocode to keep it in sync with your actual code.
Tools and Techniques for Writing Pseudocode
While pseudocode can be written with any text editor, there are some tools and techniques that can enhance the process:
- Flowcharts: Visual representations can complement pseudocode, especially for complex algorithms. Tools like Draw.io or Lucidchart can be useful for creating flowcharts.
- Mind mapping software: Tools like MindMeister or XMind can help you brainstorm and organize your thoughts before writing pseudocode.
- Collaborative platforms: If you’re working in a team, using collaborative tools like Google Docs or Notion can facilitate real-time collaboration on pseudocode.
- IDEs with pseudocode support: Some IDEs, like PSeInt, are specifically designed for writing and working with pseudocode.
- Comment-driven development: Writing your pseudocode as comments in your actual code file can help you seamlessly transition from planning to implementation.
Pseudocode and Algorithmic Thinking
Pseudocode is closely tied to algorithmic thinking, which is the ability to define clear steps to solve a problem or achieve a goal. Here’s how pseudocode supports and enhances algorithmic thinking:
- Breaking down problems: Pseudocode encourages you to break complex problems into smaller, manageable steps.
- Identifying patterns: As you write pseudocode for different problems, you’ll start to recognize common patterns and algorithms.
- Improving logical thinking: The process of writing pseudocode helps strengthen your ability to think logically and sequentially.
- Facilitating abstraction: Pseudocode allows you to abstract away implementation details and focus on the core logic of your algorithm.
- Encouraging modularity: By breaking your solution into functions or modules in pseudocode, you’re practicing good software design principles.
Pseudocode in Different Domains
While we’ve primarily discussed pseudocode in the context of general programming, it’s a versatile tool that can be applied in various domains of computer science and software development:
1. Database Design
Pseudocode can be used to outline database operations and queries:
FUNCTION get_user_orders(user_id):
CONNECT TO database
QUERY "SELECT * FROM orders WHERE user_id = user_id"
FETCH results
CLOSE database connection
RETURN results
END FUNCTION
2. Machine Learning Algorithms
Pseudocode can help in planning complex machine learning algorithms:
FUNCTION train_neural_network(data, labels):
INITIALIZE neural network with random weights
FOR EACH epoch in number_of_epochs:
FOR EACH batch in data:
forward_propagation(batch)
calculate_loss()
backward_propagation()
update_weights()
END FOR
evaluate_accuracy()
END FOR
RETURN trained_model
END FUNCTION
3. Game Development
Game logic can be outlined using pseudocode:
FUNCTION game_loop():
WHILE game is running:
handle_user_input()
update_game_state()
check_for_collisions()
update_score()
render_graphics()
END WHILE
END FUNCTION
4. Web Development
Even in web development, pseudocode can be useful for planning out application logic:
FUNCTION handle_user_registration(user_data):
VALIDATE user_data
IF validation_successful THEN
HASH password
STORE user in database
SEND confirmation email
RETURN success message
ELSE
RETURN error messages
END IF
END FUNCTION
Pseudocode and Code Documentation
Pseudocode can play a valuable role in code documentation. Here’s how:
- High-level overview: Pseudocode can provide a high-level overview of what a complex function or module does, making it easier for other developers (or your future self) to understand the code’s purpose and logic.
- Algorithm explanation: For complex algorithms, including pseudocode in the documentation can help explain the logic more clearly than the actual code.
- Planning record: Keeping the original pseudocode as part of the documentation provides insight into the thought process behind the implementation.
- Maintenance aid: When maintaining or updating code, referring back to the pseudocode can help you quickly understand and modify the underlying logic.
Here’s an example of how pseudocode might be used in a function’s documentation:
/**
* Finds the shortest path between two nodes in a graph using Dijkstra's algorithm.
*
* Pseudocode:
* FUNCTION find_shortest_path(graph, start, end):
* INITIALIZE distances with infinity for all nodes except start
* INITIALIZE priority queue with (start, 0)
* INITIALIZE previous nodes as empty map
*
* WHILE priority queue is not empty:
* GET node with minimum distance from queue
* IF node is end:
* BREAK
* FOR EACH neighbor of node:
* CALCULATE new distance
* IF new distance is less than recorded distance:
* UPDATE distance
* UPDATE previous node
* ADD (neighbor, new distance) to priority queue
*
* RECONSTRUCT path using previous nodes
* RETURN path
*
* @param {Object} graph - The graph represented as an adjacency list
* @param {string} start - The starting node
* @param {string} end - The ending node
* @returns {Array} The shortest path from start to end
*/
function findShortestPath(graph, start, end) {
// Implementation here...
}
Pseudocode in Agile Development
In Agile development methodologies, pseudocode can be a valuable tool at various stages of the development process:
- Sprint planning: Pseudocode can help break down user stories into more detailed tasks, giving the team a clearer idea of the work involved.
- Pair programming: When pair programming, writing pseudocode together can help align understanding and approach before diving into the actual coding.
- Code reviews: Including pseudocode in pull requests can help reviewers understand the logic behind the implementation more quickly.
- Refactoring: When planning to refactor complex code, writing pseudocode for the new approach can help validate the idea before committing to the changes.
Teaching and Learning with Pseudocode
Pseudocode is an excellent tool for both teaching and learning programming concepts:
- Introducing new concepts: Teachers can use pseudocode to introduce new programming concepts without the added complexity of language-specific syntax.
- Problem-solving practice: Students can practice problem-solving by writing pseudocode solutions before learning to implement them in a specific language.
- Algorithm study: Pseudocode is often used in computer science textbooks and courses to explain algorithms in a language-agnostic way.
- Self-study: When learning a new programming concept, writing pseudocode can help learners focus on understanding the logic before worrying about syntax.
Conclusion
Pseudocode is a powerful tool in a programmer’s arsenal, playing a crucial role in problem-solving, algorithm design, and software development. By bridging the gap between human thinking and computer code, pseudocode allows developers to plan and structure their solutions effectively, leading to clearer, more efficient, and more maintainable code.
Whether you’re a beginner just starting your coding journey, a student preparing for technical interviews, or an experienced developer tackling complex problems, mastering the art of writing good pseudocode can significantly enhance your problem-solving skills and overall effectiveness as a programmer.
Remember, the goal of pseudocode is not to write perfect, executable code, but to clarify your thinking and create a solid plan for your implementation. With practice, you’ll find that using pseudocode becomes a natural and invaluable part of your programming workflow, helping you tackle even the most challenging coding problems with confidence and clarity.