The Role of Problem Solving in Senior-Level Tech Interviews
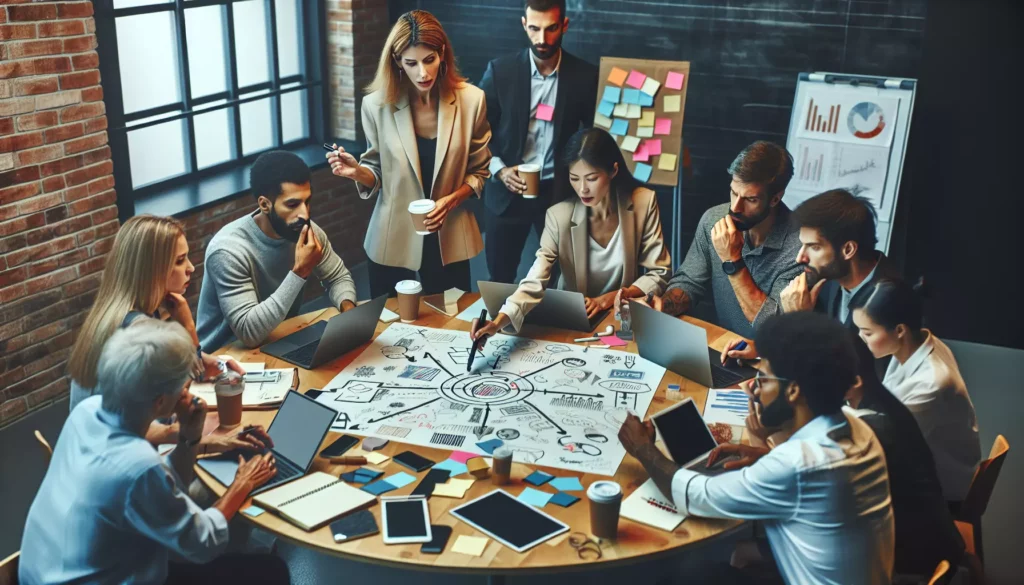
In the competitive landscape of the tech industry, senior-level positions are highly sought after, and the interview process for these roles is notoriously challenging. One of the most critical components of these interviews is problem-solving, which serves as a litmus test for a candidate’s technical prowess, creativity, and ability to handle complex scenarios. This article delves into the significance of problem-solving in senior-level tech interviews, exploring why it’s crucial, how it’s assessed, and how aspiring senior developers can prepare for this aspect of the interview process.
Why Problem Solving Matters in Senior-Level Tech Interviews
Problem-solving skills are paramount in senior-level tech positions for several reasons:
- Complexity of Work: Senior developers often tackle the most challenging and intricate problems within an organization. Their ability to break down complex issues and devise effective solutions is crucial.
- Leadership and Mentorship: Senior roles often involve guiding junior developers. Strong problem-solving skills enable senior developers to provide valuable insights and direction to their teams.
- Innovation: Companies rely on senior developers to drive innovation. The ability to think creatively and solve novel problems is essential for pushing technological boundaries.
- Adaptability: The tech industry is ever-evolving. Senior developers need to adapt quickly to new technologies and challenges, which requires robust problem-solving abilities.
- System Design: Senior roles often involve designing large-scale systems. This requires a holistic approach to problem-solving, considering factors like scalability, performance, and maintainability.
How Problem Solving is Assessed in Senior-Level Tech Interviews
Interviewers employ various methods to evaluate a candidate’s problem-solving skills:
1. Algorithmic Challenges
While not always the primary focus for senior roles, algorithmic problems are still common. These challenges assess a candidate’s ability to devise efficient solutions to computational problems. For example:
// Example of a common algorithmic challenge:
// Implement a function to find the longest palindromic substring in a given string
function longestPalindromicSubstring(s) {
if (!s || s.length < 2) return s;
let start = 0, maxLength = 1;
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
if (right - left + 1 > maxLength) {
start = left;
maxLength = right - left + 1;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
expandAroundCenter(i, i); // Odd length palindromes
expandAroundCenter(i, i + 1); // Even length palindromes
}
return s.substring(start, start + maxLength);
}
This problem tests a candidate’s ability to recognize patterns, optimize for efficiency, and handle edge cases.
2. System Design Questions
These questions are particularly important for senior roles. Candidates might be asked to design a large-scale system, considering aspects like:
- Scalability
- Load balancing
- Database design
- Caching strategies
- API design
- Microservices architecture
For example, a candidate might be asked to design a distributed file storage system like Dropbox or Google Drive. This tests their ability to think about complex systems holistically and make trade-offs between different design choices.
3. Open-ended Problem Solving
Interviewers often present real-world scenarios or past challenges the company has faced. For instance:
“Our e-commerce platform experiences significant slowdowns during peak holiday shopping periods. How would you approach diagnosing and solving this issue?”
This type of question assesses a candidate’s ability to:
- Ask clarifying questions
- Break down complex problems
- Propose and evaluate multiple solutions
- Consider business impact alongside technical feasibility
4. Debugging Exercises
Senior developers are often tasked with solving the most perplexing bugs. Interviewers might present a piece of code with subtle errors and ask the candidate to identify and fix the issues. For example:
// Find and fix the bug in this JavaScript code
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
console.log(fibonacci(50)); // This will take a very long time to execute
The bug here isn’t syntactical but performance-related. A senior developer should recognize the inefficiency of the naive recursive approach and suggest optimizations like memoization or an iterative solution.
5. Architecture and Design Patterns
Knowledge of software architecture and design patterns is crucial for senior roles. Candidates might be asked to discuss:
- The appropriate use of design patterns like Singleton, Factory, or Observer
- Architectural styles like microservices, event-driven architecture, or serverless computing
- Trade-offs between different architectural choices
For instance, a candidate might be asked to explain when they would choose a microservices architecture over a monolithic one, and how they would handle the challenges that come with distributed systems.
Key Problem-Solving Skills Assessed in Senior-Level Tech Interviews
Interviewers are looking for several key skills when evaluating a candidate’s problem-solving abilities:
1. Analytical Thinking
The ability to break down complex problems into manageable components is crucial. Senior developers should demonstrate:
- Systematic approach to problem analysis
- Identification of core issues and their relationships
- Ability to prioritize sub-problems effectively
2. Creativity
While adhering to best practices is important, senior developers should also show creativity in their problem-solving approach. This might involve:
- Proposing innovative solutions to longstanding problems
- Combining different technologies or approaches in novel ways
- Thinking outside conventional paradigms when necessary
3. Communication
The ability to articulate complex ideas clearly is essential. Candidates should demonstrate:
- Clear explanation of their thought process
- Effective use of diagrams or pseudocode to illustrate concepts
- Ability to discuss technical concepts with both technical and non-technical stakeholders
4. Trade-off Analysis
Senior developers often need to make decisions that balance various competing factors. Candidates should show:
- Understanding of the pros and cons of different approaches
- Ability to make informed decisions based on given constraints (time, resources, scalability requirements, etc.)
- Consideration of both short-term and long-term implications of design choices
5. Scalability Thinking
Given the large-scale nature of many tech projects, the ability to think about scalability is crucial. This involves:
- Understanding how solutions will perform under increased load
- Identifying potential bottlenecks in system designs
- Proposing strategies for horizontal and vertical scaling
How to Prepare for Problem Solving in Senior-Level Tech Interviews
Preparing for the problem-solving component of senior-level tech interviews requires a multifaceted approach:
1. Strengthen Your Fundamentals
While senior roles often focus more on high-level problem solving, a strong grasp of fundamentals is still crucial:
- Review data structures and algorithms
- Practice implementing common algorithms from scratch
- Understand time and space complexity analysis
Resources like AlgoCademy can be invaluable for refreshing these skills, offering interactive tutorials and problem sets tailored for interview preparation.
2. Study System Design
System design is a critical component of senior-level interviews:
- Read books on distributed systems and scalable architectures
- Study case studies of how large tech companies have solved scaling problems
- Practice designing high-level architectures for popular services (e.g., design Twitter, design a URL shortener)
3. Stay Current with Technology Trends
Senior developers are expected to have a broad understanding of the tech landscape:
- Follow tech blogs and news sites
- Experiment with new technologies and frameworks
- Attend conferences or webinars on emerging tech trends
4. Practice Articulating Your Thought Process
The ability to communicate your problem-solving approach is as important as the solution itself:
- Practice explaining your solutions out loud
- Participate in mock interviews where you can get feedback on your communication
- Join coding discussion groups or forums to practice explaining concepts to others
5. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can be beneficial. Here’s an example framework:
- Understand the problem: Ask clarifying questions, identify constraints and requirements.
- Break down the problem: Identify the main components or steps needed to solve the problem.
- Propose potential solutions: Brainstorm multiple approaches.
- Analyze trade-offs: Consider the pros and cons of each approach.
- Choose and implement a solution: Select the best approach and start implementing.
- Test and refine: Consider edge cases and potential improvements.
6. Work on Real-World Projects
Practical experience is invaluable:
- Contribute to open-source projects
- Build side projects that solve real problems
- Take on challenging projects at work and document your problem-solving process
7. Practice With Mock Interviews
Simulate the interview experience:
- Use platforms that offer mock technical interviews
- Practice with peers or mentors in your network
- Record yourself solving problems to review your performance
Common Pitfalls to Avoid in Problem Solving During Interviews
Even experienced developers can fall into certain traps during interviews. Here are some common pitfalls to be aware of:
1. Rushing to Code
Many candidates feel pressured to start coding immediately. However, it’s crucial to take time to understand the problem and plan your approach. Interviewers often value the thought process as much as the final solution.
2. Neglecting Edge Cases
Senior developers are expected to think comprehensively about potential scenarios. Always consider edge cases and discuss how your solution handles them.
3. Overlooking Scalability
A solution that works for small inputs might fail at scale. Always consider how your solution would perform with large datasets or high user loads.
4. Poor Communication
Failing to explain your thought process clearly can be detrimental. Practice articulating your ideas, even when you’re unsure or stuck.
5. Ignoring Trade-offs
Every solution has pros and cons. Demonstrating an understanding of these trade-offs shows maturity and real-world problem-solving skills.
6. Not Asking for Clarification
Don’t hesitate to ask questions to fully understand the problem. This shows thoughtfulness and attention to detail.
7. Stubbornness
If you realize your initial approach isn’t optimal, be willing to pivot. Adaptability is a valuable trait in senior developers.
The Future of Problem Solving in Tech Interviews
As the tech industry evolves, so do interview practices. Here are some trends that may shape the future of problem-solving assessments in senior-level tech interviews:
1. Emphasis on Real-World Scenarios
There’s a growing trend towards using actual problems that companies have faced, rather than abstract algorithmic puzzles. This approach better reflects the day-to-day challenges of senior roles.
2. Focus on Soft Skills
While technical skills remain crucial, there’s increasing recognition of the importance of soft skills like communication, leadership, and adaptability in problem-solving scenarios.
3. Remote Collaboration Challenges
With the rise of remote work, interviews may include more challenges that assess a candidate’s ability to solve problems collaboratively in a distributed environment.
4. Ethical Considerations
As tech’s impact on society grows, interviews may include more questions about ethical problem-solving, considering factors like privacy, bias, and social responsibility.
5. Interdisciplinary Problem Solving
With tech permeating various industries, senior developers may be expected to solve problems that require knowledge beyond pure computer science, incorporating fields like biology, finance, or environmental science.
Conclusion
Problem-solving skills are at the heart of what makes a senior developer valuable to an organization. In senior-level tech interviews, these skills are put to the test through a variety of challenges, from algorithmic puzzles to system design questions and real-world scenarios.
To excel in these interviews, candidates need to cultivate a broad skill set that includes strong analytical thinking, creativity, effective communication, and a deep understanding of scalability and system architecture. Preparation involves not just coding practice, but also studying system design, staying current with technology trends, and developing a structured approach to tackling complex problems.
Remember that the goal of these interviews is not just to find someone who can code, but to identify individuals who can lead teams, drive innovation, and solve the most challenging problems a company faces. By focusing on developing robust problem-solving skills and practicing their application in interview-like scenarios, aspiring senior developers can position themselves for success in even the most demanding tech interviews.
As the tech industry continues to evolve, so too will the nature of these interviews. However, the fundamental importance of strong problem-solving skills is likely to remain constant. By continually honing these skills and adapting to new challenges, senior developers can ensure they remain at the forefront of the tech industry, ready to tackle whatever problems the future may bring.