The Role of Pattern Recognition in Coding Challenges: Unlocking the Power of Algorithmic Thinking
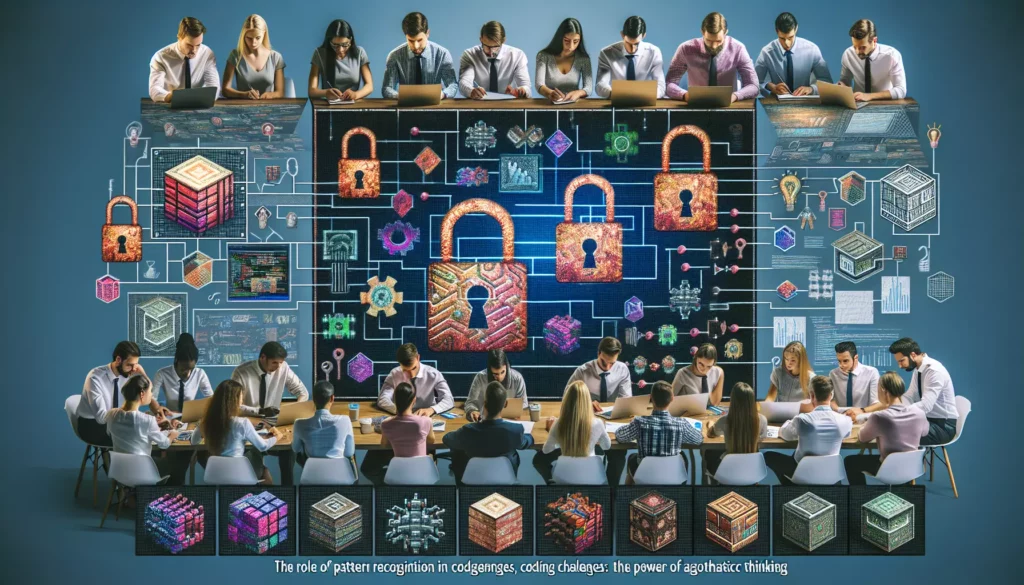
In the ever-evolving landscape of software development and computer science, the ability to recognize patterns and apply them to solve complex problems is a crucial skill. This skill, known as pattern recognition, plays a pivotal role in tackling coding challenges and developing efficient algorithms. As aspiring programmers and seasoned developers alike strive to enhance their problem-solving abilities, understanding the significance of pattern recognition becomes increasingly important.
In this comprehensive guide, we’ll explore the intricate relationship between pattern recognition and coding challenges, delving into how this cognitive skill can be harnessed to improve algorithmic thinking and overall programming prowess. We’ll examine various aspects of pattern recognition in coding, from its fundamental principles to practical applications in real-world scenarios.
Understanding Pattern Recognition in Coding
Pattern recognition in coding refers to the ability to identify recurring structures, behaviors, or problem-solving approaches within different programming challenges. It involves recognizing similarities between seemingly disparate problems and applying known solutions or techniques to new situations. This skill is essential for several reasons:
- Efficiency: Recognizing patterns allows developers to solve problems more quickly by applying proven solutions.
- Optimization: Pattern recognition often leads to more optimized code by identifying opportunities for improvement.
- Problem-solving: It enhances overall problem-solving skills by providing a framework for approaching new challenges.
- Code reusability: Recognizing patterns promotes the creation of reusable code components and design patterns.
- Algorithm design: It aids in the development of efficient algorithms by identifying common problem structures.
Types of Patterns in Coding Challenges
When it comes to coding challenges, several types of patterns emerge frequently. Understanding these patterns can significantly improve your ability to tackle a wide range of problems:
1. Algorithmic Patterns
Algorithmic patterns are recurring approaches to solving specific types of problems. Some common examples include:
- Divide and Conquer: Breaking down a problem into smaller, manageable sub-problems.
- Dynamic Programming: Solving complex problems by breaking them down into simpler subproblems.
- Greedy Algorithms: Making locally optimal choices at each step to find a global optimum.
- Backtracking: Building a solution incrementally and abandoning solutions that fail to satisfy the constraints.
2. Data Structure Patterns
Certain problems are best solved using specific data structures. Recognizing these patterns can help you choose the most appropriate data structure for a given problem:
- Stack-based problems: Often used for parsing expressions or managing function calls.
- Queue-based problems: Useful for breadth-first search algorithms or managing tasks in order.
- Tree-based problems: Common in hierarchical data representation and searching algorithms.
- Graph-based problems: Frequently used in network analysis, pathfinding, and social network algorithms.
3. Coding Technique Patterns
These patterns involve specific coding techniques that can be applied across various problems:
- Two-pointer technique: Often used in array or string manipulation problems.
- Sliding window: Useful for problems involving subarrays or substring calculations.
- Fast and slow pointers: Commonly used in linked list problems, especially for cycle detection.
- Bit manipulation: Applied in problems that can be optimized using bitwise operations.
The Process of Pattern Recognition in Coding
Developing strong pattern recognition skills in coding is a gradual process that involves several key steps:
1. Problem Analysis
The first step in recognizing patterns is to thoroughly analyze the problem at hand. This involves:
- Identifying the problem’s core requirements and constraints.
- Breaking down the problem into smaller components.
- Recognizing any familiar elements or structures within the problem description.
2. Pattern Identification
Once you’ve analyzed the problem, the next step is to identify any patterns that might be applicable:
- Compare the current problem with previously encountered challenges.
- Look for similarities in problem structure, data manipulation, or required output.
- Consider whether any known algorithmic or data structure patterns might be relevant.
3. Pattern Application
After identifying potential patterns, you need to apply them to the current problem:
- Adapt the pattern to fit the specific requirements of the problem.
- Combine multiple patterns if necessary to create a comprehensive solution.
- Iterate and refine the application of the pattern as needed.
4. Evaluation and Optimization
The final step involves evaluating the effectiveness of the applied pattern and optimizing the solution:
- Assess whether the chosen pattern leads to an efficient and correct solution.
- Identify any areas where the solution can be further optimized.
- Consider alternative patterns that might yield better results.
Practical Examples of Pattern Recognition in Coding Challenges
To better understand how pattern recognition works in practice, let’s examine a few common coding challenges and the patterns that can be applied to solve them:
Example 1: Two Sum Problem
Problem: Given an array of integers and a target sum, find two numbers in the array that add up to the target sum.
Pattern Recognition:
- This problem can be solved using the two-pointer technique or a hash table.
- The two-pointer approach works well for sorted arrays, while the hash table method is efficient for unsorted arrays.
Solution using a hash table:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
Example 2: Fibonacci Sequence
Problem: Generate the nth Fibonacci number.
Pattern Recognition:
- This problem exhibits a clear recursive pattern.
- It can be optimized using dynamic programming to avoid redundant calculations.
Solution using dynamic programming:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Example 3: Detecting a Cycle in a Linked List
Problem: Determine if a linked list contains a cycle.
Pattern Recognition:
- This problem can be solved using the fast and slow pointer technique (Floyd’s Cycle-Finding Algorithm).
- The pattern involves using two pointers moving at different speeds to detect a cycle.
Solution using fast and slow pointers:
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
Developing Pattern Recognition Skills
Improving your pattern recognition skills in coding is an ongoing process that requires practice and dedication. Here are some strategies to enhance your abilities:
1. Solve Diverse Problems
Expose yourself to a wide range of coding challenges across different domains and difficulty levels. This broadens your perspective and helps you recognize patterns in various contexts.
2. Study Classic Algorithms and Data Structures
Familiarize yourself with fundamental algorithms and data structures. Understanding these building blocks will help you identify their applications in more complex problems.
3. Analyze Multiple Solutions
For each problem you solve, explore alternative solutions. This practice helps you recognize different patterns that can be applied to the same problem.
4. Participate in Coding Competitions
Engage in coding competitions or online judge platforms. These environments often present problems that require pattern recognition and quick thinking.
5. Review and Reflect
After solving a problem, take time to reflect on the solution. Identify the patterns you used and consider how they might apply to future challenges.
6. Collaborate and Discuss
Engage in discussions with other programmers. Sharing insights and learning from others can significantly enhance your pattern recognition skills.
The Impact of Pattern Recognition on Coding Interviews
Pattern recognition plays a crucial role in coding interviews, especially for positions at major tech companies. Here’s how it can impact your performance:
1. Efficient Problem-Solving
Recognizing patterns allows you to quickly identify potential solutions, saving valuable time during interviews.
2. Demonstrating Algorithmic Thinking
By applying known patterns to new problems, you showcase your ability to think algorithmically and adapt existing knowledge to novel situations.
3. Optimizing Solutions
Pattern recognition often leads to more optimized solutions, which is highly valued in technical interviews.
4. Handling Time Pressure
In time-constrained interview settings, the ability to quickly recognize and apply patterns can be a significant advantage.
5. Tackling Unfamiliar Problems
Even when faced with unfamiliar problems, strong pattern recognition skills can help you draw parallels to known problems and devise effective solutions.
Common Pitfalls in Pattern Recognition
While pattern recognition is a powerful tool, it’s important to be aware of potential pitfalls:
1. Over-reliance on Patterns
Avoid forcing a pattern onto a problem where it doesn’t fit. Sometimes, a unique approach is required.
2. Neglecting Problem-Specific Details
Don’t overlook the unique aspects of each problem in your eagerness to apply a familiar pattern.
3. Ignoring Efficiency
Applying a pattern without considering its efficiency in the specific context can lead to suboptimal solutions.
4. Lack of Flexibility
Be prepared to adapt or combine patterns as needed. Rigid adherence to a single pattern may limit your problem-solving capabilities.
Advanced Pattern Recognition Techniques
As you progress in your coding journey, you can explore more advanced pattern recognition techniques:
1. Meta-patterns
Identify patterns that occur across different types of problems or algorithms. These high-level patterns can guide your overall approach to problem-solving.
2. Pattern Adaptation
Learn to adapt known patterns to fit unique problem requirements. This skill involves recognizing the core principles of a pattern and applying them in novel ways.
3. Pattern Creation
As you gain experience, you may start to create your own patterns based on recurring themes you observe in your problem-solving experiences.
4. Cross-domain Pattern Recognition
Look for patterns that extend beyond coding into other fields like mathematics, logic, or even real-world scenarios. This broader perspective can lead to innovative solutions.
Conclusion
Pattern recognition is a fundamental skill in the world of coding and algorithm design. It empowers developers to approach complex problems with confidence, leverage existing knowledge, and devise efficient solutions. By honing your pattern recognition abilities, you not only enhance your problem-solving skills but also prepare yourself for the challenges of technical interviews and real-world software development.
Remember that developing strong pattern recognition skills is an ongoing process that requires practice, patience, and a willingness to learn from each coding challenge you encounter. As you continue to solve diverse problems and analyze various solutions, you’ll find that your ability to recognize and apply patterns improves, making you a more effective and efficient programmer.
Embrace the journey of pattern discovery in your coding practice, and you’ll unlock new levels of problem-solving prowess that will serve you well throughout your programming career. Whether you’re preparing for technical interviews at top tech companies or working on complex software projects, the power of pattern recognition will be an invaluable asset in your developer toolkit.