The Role of Pair Programming in Learning as a Junior Developer
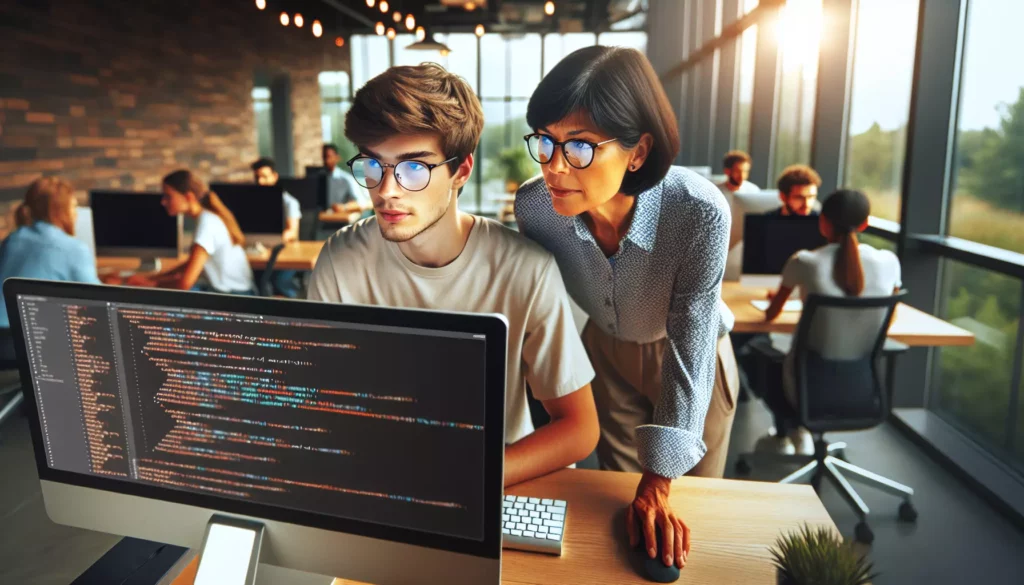
As a junior developer embarking on your coding journey, you’re likely to encounter various learning methodologies and collaborative practices. One such practice that has gained significant traction in both educational settings and professional environments is pair programming. This approach to coding not only accelerates learning but also enhances problem-solving skills and fosters a collaborative mindset essential for success in the tech industry. In this comprehensive guide, we’ll explore the role of pair programming in learning as a junior developer, its benefits, challenges, and how it fits into the broader landscape of coding education and skills development.
What is Pair Programming?
Pair programming is a software development technique where two programmers work together at a single workstation. One, the “driver,” writes code while the other, the “navigator” or “observer,” reviews each line of code as it’s typed in. The two programmers switch roles frequently, typically every 30 minutes to an hour.
This collaborative approach to coding was popularized by Extreme Programming (XP) methodologies and has since become a staple in many agile development environments. However, its application extends beyond professional settings and into the realm of coding education, where it serves as a powerful tool for junior developers to accelerate their learning and skill acquisition.
The Benefits of Pair Programming for Junior Developers
1. Accelerated Learning
One of the primary advantages of pair programming for junior developers is the accelerated learning curve it provides. When paired with a more experienced developer, juniors can gain insights into best practices, coding patterns, and problem-solving strategies that might take much longer to discover on their own.
For example, a junior developer might struggle with implementing a complex algorithm. Through pair programming, they can observe how a senior developer approaches the problem, breaks it down, and implements a solution. This real-time learning experience is invaluable and often more effective than traditional learning methods.
2. Improved Code Quality
Pair programming naturally leads to higher code quality. With two sets of eyes on the code, errors are caught earlier, and solutions are often more elegant. For junior developers, this means learning to write cleaner, more efficient code from the outset.
Consider this simple example of how pair programming can improve code quality:
// Before pair programming (written by junior developer)
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total = total + items[i].price;
}
return total;
}
// After pair programming (improved with senior developer input)
function calculateTotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
In this example, the pair programming session resulted in a more concise and efficient solution using functional programming concepts that the junior developer might not have been familiar with initially.
3. Enhanced Problem-Solving Skills
Pair programming encourages verbal communication of thought processes. As junior developers articulate their ideas and reasoning, they develop stronger problem-solving skills. Moreover, exposure to different problem-solving approaches from their partners broadens their perspective and enhances their ability to tackle complex coding challenges.
4. Increased Confidence
Working alongside another developer, especially a more experienced one, can significantly boost a junior developer’s confidence. As they contribute ideas, write code, and solve problems collaboratively, they gain validation of their skills and knowledge. This confidence is crucial for junior developers as they progress in their careers and take on more challenging projects.
5. Improved Communication Skills
Effective communication is a critical skill in the tech industry. Pair programming provides junior developers with ample opportunities to practice explaining their thought processes, defending their ideas, and giving and receiving feedback. These communication skills are invaluable not only for coding but also for future roles that may involve leadership or client interactions.
6. Exposure to Different Coding Styles and Tools
Every developer has their unique coding style and preferred set of tools. Through pair programming, junior developers are exposed to a variety of approaches, IDEs, shortcuts, and debugging techniques. This exposure broadens their skill set and helps them develop their own efficient workflow.
Challenges of Pair Programming for Junior Developers
While the benefits of pair programming are numerous, it’s important to acknowledge and address the challenges that junior developers might face:
1. Imposter Syndrome
Junior developers may feel intimidated when paired with more experienced programmers, leading to imposter syndrome. It’s crucial to create a supportive environment where juniors feel comfortable making mistakes and asking questions.
2. Unequal Participation
There’s a risk of the more experienced developer dominating the session, potentially reducing the learning opportunity for the junior. Establishing clear roles and encouraging equal participation is essential to mitigate this issue.
3. Differing Pace and Learning Styles
Junior developers may require more time to process information or prefer different learning approaches. Pair programming sessions should be flexible enough to accommodate various learning styles and paces.
4. Overreliance on the Partner
There’s a potential for junior developers to become overly dependent on their programming partners. It’s important to balance pair programming with individual coding time to ensure juniors develop self-reliance and problem-solving skills.
Implementing Pair Programming in Learning Environments
To maximize the benefits of pair programming for junior developers, consider the following strategies:
1. Structured Pairing Sessions
Implement structured pair programming sessions with clear objectives and time limits. This helps maintain focus and ensures both partners have the opportunity to contribute meaningfully.
2. Rotate Partners
Regularly rotating programming partners exposes junior developers to diverse perspectives and coding styles. This variety enhances their adaptability and broadens their skill set.
3. Gradual Complexity Increase
Start with simple tasks and gradually increase complexity as junior developers become more comfortable with pair programming. This approach builds confidence and prevents overwhelming newcomers to the practice.
4. Encourage Teaching Moments
Promote a culture where more experienced developers actively explain their thought processes and decision-making. This transforms pair programming sessions into rich learning experiences for junior developers.
5. Incorporate Code Reviews
Follow pair programming sessions with code reviews. This additional step reinforces learning and provides an opportunity for junior developers to receive broader feedback on their work.
Pair Programming in the Context of Coding Education Platforms
Platforms like AlgoCademy, which focus on coding education and programming skills development, can greatly benefit from incorporating pair programming into their curriculum. Here’s how pair programming aligns with and enhances the goals of such platforms:
1. Interactive Learning
Pair programming naturally complements the interactive nature of coding tutorials. Platforms can implement virtual pair programming features, allowing learners to collaborate in real-time on coding challenges.
2. AI-Powered Assistance
While AI can provide valuable guidance, pair programming with human partners offers a level of interaction and spontaneity that AI cannot fully replicate. However, AI can be used to suggest optimal pairings based on skill levels and learning objectives.
3. Preparation for Technical Interviews
Many technical interviews, especially for major tech companies, involve collaborative problem-solving. Pair programming experiences prepare junior developers for these scenarios by improving their ability to think aloud and collaborate on complex problems.
4. Algorithmic Thinking and Problem-Solving
Pair programming sessions focused on algorithmic challenges can enhance a junior developer’s problem-solving skills. The collaborative nature of pair programming often leads to more creative and efficient solutions to complex algorithmic problems.
5. Community Building
Incorporating pair programming into online learning platforms can foster a sense of community among learners. This community aspect can increase engagement and motivation, crucial factors in the learning process.
Real-World Application: Pair Programming in Tech Companies
Many leading tech companies, including those often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), utilize pair programming in their development processes. Understanding how pair programming is applied in these professional settings can help junior developers prepare for their future careers:
1. Onboarding New Developers
Companies often use pair programming to onboard new hires, including junior developers. This approach helps newcomers familiarize themselves with the codebase, development practices, and team dynamics more quickly.
2. Tackling Complex Problems
When faced with particularly challenging issues or architectural decisions, even experienced developers in these companies often turn to pair programming. This collaborative approach can lead to more robust and innovative solutions.
3. Code Reviews and Quality Assurance
While not traditional pair programming, many companies implement a form of asynchronous collaboration through thorough code review processes. Junior developers can learn a lot from these reviews, which often involve discussions similar to those in pair programming sessions.
4. Knowledge Sharing
In large organizations with diverse projects, pair programming serves as an effective knowledge sharing tool. It allows for cross-pollination of ideas and techniques across different teams and projects.
Tools and Platforms for Remote Pair Programming
As remote work becomes more prevalent, tools that facilitate remote pair programming are increasingly important. Here are some popular options that junior developers should be familiar with:
1. Visual Studio Code Live Share
This extension for Visual Studio Code allows real-time collaborative editing and debugging. It’s particularly useful for its seamless integration with a widely-used IDE.
2. Tuple
Designed specifically for remote pair programming, Tuple offers low-latency screen sharing and remote control features optimized for developers.
3. Replit
A browser-based IDE that supports real-time collaboration, Replit is excellent for quick pair programming sessions without the need for extensive setup.
4. CodePen
While primarily known for front-end development, CodePen’s Collab Mode allows real-time collaboration, making it a great tool for pair programming on web development projects.
5. GitDuck
This tool combines video chat with code sharing, creating an immersive pair programming experience that closely mimics in-person collaboration.
Best Practices for Junior Developers in Pair Programming
To make the most of pair programming experiences, junior developers should keep these best practices in mind:
1. Be Active and Engaged
Whether you’re the driver or navigator, stay actively involved in the process. Ask questions, offer suggestions, and be present in the moment.
2. Communicate Clearly
Practice articulating your thoughts and reasoning. Clear communication is key to effective pair programming and is a valuable skill in any development role.
3. Be Open to Feedback
View feedback as an opportunity for growth rather than criticism. Embrace the chance to learn from your programming partner.
4. Take Regular Breaks
Pair programming can be mentally intensive. Take short breaks to maintain focus and prevent burnout.
5. Reflect on Sessions
After each pair programming session, take time to reflect on what you learned, what worked well, and areas for improvement.
6. Practice Both Roles
Ensure you get experience as both the driver and navigator. Each role offers unique learning opportunities and skill development.
Conclusion
Pair programming plays a crucial role in the learning journey of junior developers. It offers a unique blend of collaborative problem-solving, real-time feedback, and exposure to diverse coding practices. By accelerating the learning process, improving code quality, and enhancing communication skills, pair programming prepares junior developers not just for coding challenges but for the collaborative nature of the tech industry as a whole.
As coding education platforms like AlgoCademy continue to evolve, incorporating pair programming into their curriculum can provide learners with a more holistic and industry-aligned learning experience. For junior developers, embracing pair programming is not just about improving coding skills—it’s about developing the collaborative mindset and communication abilities that are essential for a successful career in software development.
Whether you’re just starting your coding journey or looking to enhance your skills, consider seeking out pair programming opportunities. Engage with peers, mentor others when you can, and don’t shy away from pairing with more experienced developers. Remember, every line of code written together is an opportunity to learn, grow, and become a better developer.