The Role of Mock Interviews in Preparation: Mastering the Art of Technical Interviews
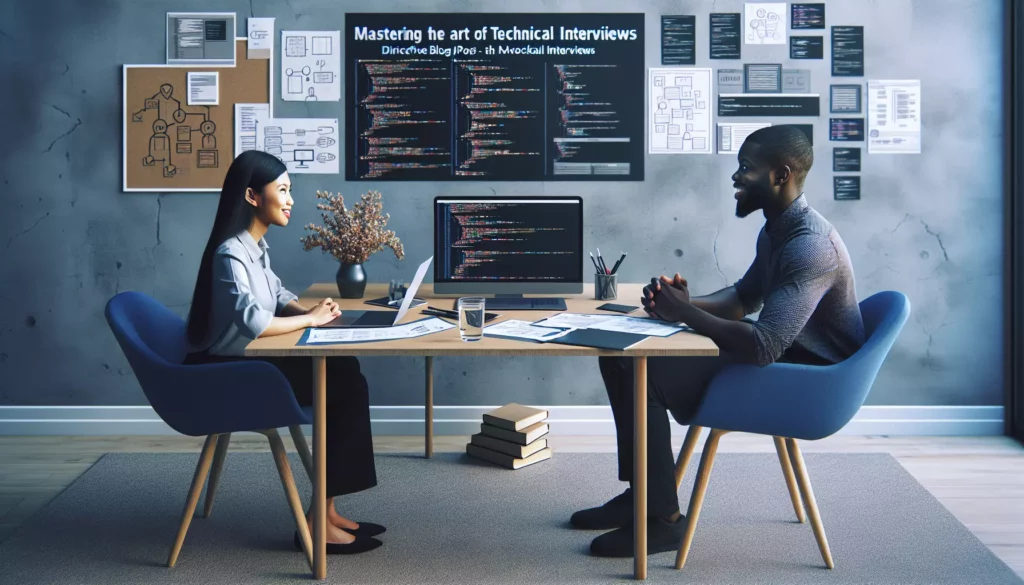
In the competitive world of software engineering, landing a job at a top tech company like Google, Amazon, or Facebook (often referred to as FAANG companies) is a dream for many aspiring developers. However, the road to success is paved with challenging technical interviews that require not just coding skills, but also the ability to think on your feet, communicate effectively, and solve complex problems under pressure. This is where mock interviews play a crucial role in preparation, acting as a bridge between theoretical knowledge and practical application.
Understanding the Importance of Mock Interviews
Mock interviews are simulated job interviews that mimic the conditions of real technical interviews. They provide a safe environment for candidates to practice their skills, receive feedback, and refine their approach. The importance of mock interviews in the preparation process cannot be overstated, especially when it comes to technical roles in the software industry.
Benefits of Mock Interviews
- Reduced Anxiety: By familiarizing yourself with the interview process, you can significantly reduce anxiety and nervousness on the actual day.
- Improved Communication: Practice articulating your thoughts and explaining complex concepts clearly and concisely.
- Feedback and Growth: Receive constructive criticism to identify areas for improvement and track your progress over time.
- Time Management: Learn to manage your time effectively during coding challenges and problem-solving sessions.
- Realistic Experience: Get a taste of what to expect in a real interview, including common questions and scenarios.
Types of Mock Interviews for Technical Roles
When preparing for technical interviews, especially for positions at FAANG companies, it’s important to engage in various types of mock interviews to cover all aspects of the assessment process.
1. Algorithmic Problem-Solving Interviews
These mock interviews focus on data structures and algorithms, often mirroring the style of LeetCode or HackerRank problems. They typically involve:
- Solving coding challenges in real-time
- Discussing time and space complexity
- Optimizing solutions
- Explaining thought processes and approaches
Example scenario:
Interviewer: "Given an array of integers, find two numbers such that they add up to a specific target number."
Candidate: "Okay, let's approach this step-by-step:
1. We can use a hash map to store the complement of each number as we iterate through the array.
2. For each number, we check if its complement exists in the hash map.
3. If it does, we've found our pair. If not, we add the current number to the map.
Here's a Python implementation:"
def two_sum(nums, target):
complement_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_map:
return [complement_map[complement], i]
complement_map[num] = i
return [] # No solution found
Interviewer: "Great! Can you explain the time and space complexity of this solution?"
Candidate: "Certainly. The time complexity is O(n) because we're iterating through the array once. The space complexity is also O(n) in the worst case, where we might need to store all elements in the hash map before finding a solution."
2. System Design Interviews
These mock interviews test your ability to design large-scale distributed systems. They often involve:
- Discussing high-level architecture
- Considering scalability and performance
- Addressing potential bottlenecks
- Making trade-offs between different design choices
Example scenario:
Interviewer: "Design a URL shortening service like bit.ly."
Candidate: "Let's break this down into components:
1. API Gateway: To handle incoming requests
2. Application Server: To process URL shortening and redirection
3. Database: To store long and short URL mappings
4. Cache: To improve read performance
For the URL shortening algorithm, we could use:
- A hash function to generate a short code
- A base62 encoding of an auto-incrementing ID
To handle scalability:
- We can use a load balancer in front of multiple application servers
- Implement database sharding based on the first few characters of the short URL
- Use a distributed cache like Redis for faster lookups
For analytics, we can:
- Use a separate analytics service
- Implement asynchronous logging to a data warehouse for processing"
Interviewer: "How would you handle potential hash collisions in your shortening algorithm?"
Candidate: "Good point. We could implement a collision resolution strategy:
1. If a collision occurs, append a small random string to the original URL before hashing again
2. Alternatively, use a combination of timestamp and random string to ensure uniqueness"
3. Behavioral Interviews
While technical skills are crucial, companies also value soft skills and cultural fit. Behavioral mock interviews help prepare for questions about:
- Past experiences and projects
- Teamwork and conflict resolution
- Leadership and initiative
- Handling pressure and deadlines
Example scenario:
Interviewer: "Tell me about a time when you had to deal with a difficult team member on a project."
Candidate: "In my last role, we were working on a critical feature with a tight deadline. One team member consistently missed meetings and delivered incomplete work. Here's how I handled it:
1. I first approached them privately to understand if there were any personal issues affecting their work.
2. We discovered they were struggling with some technical aspects of the project.
3. I organized pair programming sessions to help them catch up and improve their skills.
4. We also adjusted our team communication to include more frequent check-ins.
The result was a significant improvement in their performance, and we completed the project on time. This experience taught me the importance of open communication and supporting team members to achieve collective success."
Interviewer: "That's a great example. How did this experience influence your approach to teamwork in subsequent projects?"
Candidate: "It reinforced the importance of proactive communication and regular check-ins. In future projects, I implemented a system of brief daily stand-ups and weekly one-on-ones with team members to catch potential issues early and ensure everyone felt supported."
Preparing for Mock Interviews
To get the most out of mock interviews, it’s essential to approach them with the same seriousness as you would a real interview. Here are some tips to help you prepare:
1. Review Fundamental Concepts
Before diving into mock interviews, ensure you have a solid grasp of fundamental computer science concepts, including:
- Data structures (arrays, linked lists, trees, graphs, hash tables)
- Algorithms (sorting, searching, dynamic programming)
- Time and space complexity analysis
- Object-oriented programming principles
- Database concepts (SQL vs. NoSQL, indexing, transactions)
- Networking basics (TCP/IP, HTTP)
2. Practice Coding Problems
Regularly solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on:
- Implementing solutions in your preferred programming language
- Optimizing for both time and space complexity
- Writing clean, readable code
- Explaining your thought process as you solve problems
3. Study System Design Principles
For system design interviews, familiarize yourself with:
- Scalability concepts (vertical vs. horizontal scaling)
- Load balancing techniques
- Caching strategies
- Database sharding and replication
- Microservices architecture
- API design principles
4. Prepare Your Personal Stories
For behavioral interviews, reflect on your past experiences and prepare stories that demonstrate:
- Leadership and initiative
- Problem-solving skills
- Teamwork and collaboration
- Handling conflicts or challenges
- Learning from failures
5. Set Up a Proper Environment
Create an environment that mimics a real interview setting:
- Use a quiet, well-lit room
- Ensure a stable internet connection for video interviews
- Have a whiteboard or shared document ready for collaborative problem-solving
- Dress professionally, as you would for an actual interview
Conducting Effective Mock Interviews
To maximize the benefits of mock interviews, consider the following strategies:
1. Find Suitable Interview Partners
Look for mock interview partners who can provide valuable feedback:
- Peers with similar experience levels for mutual practice
- Senior developers or mentors for more challenging sessions
- Professional interview coaching services for expert guidance
2. Simulate Real Interview Conditions
Make the mock interview as realistic as possible:
- Set a specific time limit for each session
- Use a shared coding environment or whiteboard
- Avoid interruptions or breaks during the session
- Practice both video and phone interview formats
3. Rotate Interview Roles
If practicing with peers, take turns being the interviewer and the candidate. This helps you:
- Gain perspective on what interviewers look for
- Learn to ask probing questions
- Improve your ability to give constructive feedback
4. Record and Review Sessions
With permission, record your mock interviews to:
- Analyze your performance objectively
- Identify areas for improvement in communication and problem-solving
- Track your progress over time
5. Embrace Constructive Feedback
After each mock interview:
- Ask for specific feedback on your performance
- Discuss alternative approaches to problems
- Identify strengths to emphasize and weaknesses to address
- Create an action plan for improvement
Leveraging Technology in Mock Interviews
In today’s digital age, various tools and platforms can enhance your mock interview experience:
1. Online Coding Platforms
Utilize platforms that offer collaborative coding environments:
- CoderPad
- HackerRank’s CodePair
- LeetCode’s Mock Interview feature
These tools allow you to write, run, and share code in real-time, simulating the experience of a technical interview.
2. AI-Powered Interview Preparation
Explore AI-driven platforms that offer personalized interview practice:
- AlgoCademy’s AI-assisted coding tutorials
- Pramp’s peer-to-peer mock interviews with AI feedback
- InterviewBit’s guided interview preparation
These platforms can provide immediate feedback on your coding style, problem-solving approach, and communication skills.
3. Video Conferencing Tools
Familiarize yourself with popular video conferencing platforms used for remote interviews:
- Zoom
- Google Meet
- Microsoft Teams
Practice sharing your screen, using virtual whiteboards, and maintaining eye contact through the camera.
Common Pitfalls to Avoid in Mock Interviews
While mock interviews are incredibly beneficial, be aware of these potential pitfalls:
1. Over-Rehearsing Responses
While preparation is key, avoid memorizing scripted answers. Instead, focus on understanding concepts deeply so you can explain them naturally and adapt to different question phrasings.
2. Neglecting Non-Technical Skills
Don’t focus solely on coding skills. Pay attention to your communication, problem-solving approach, and ability to work through ambiguity.
3. Ignoring Time Management
In real interviews, time is limited. Practice managing your time effectively, including asking for clarification, thinking aloud, and knowing when to move on from a stuck point.
4. Failing to Learn from Mistakes
Each mock interview is a learning opportunity. Analyze your mistakes, understand why you made them, and develop strategies to avoid them in the future.
5. Neglecting to Practice Explaining Your Thought Process
Interviewers are often more interested in your problem-solving approach than the final solution. Practice articulating your thoughts clearly as you work through problems.
Conclusion: The Path to Interview Success
Mock interviews are an invaluable tool in your preparation arsenal for technical interviews, especially when aiming for positions at top tech companies. They provide a realistic simulation of the interview experience, helping you refine your technical skills, improve your communication, and build confidence.
Remember that consistent practice and honest self-reflection are key to making the most of mock interviews. Embrace the feedback you receive, continuously work on your weak areas, and approach each session as an opportunity to learn and grow.
As you progress through your mock interview journey, you’ll likely notice significant improvements in your problem-solving skills, coding abilities, and overall interview performance. This growth will not only prepare you for the interviews ahead but also contribute to your development as a skilled and confident software engineer.
Platforms like AlgoCademy offer comprehensive resources and AI-powered assistance to complement your mock interview practice, providing a well-rounded approach to interview preparation. By combining structured learning, practical coding exercises, and regular mock interviews, you’ll be well-equipped to tackle even the most challenging technical interviews and take a significant step towards landing your dream job in the competitive world of software engineering.