The Role of Latency and Throughput in System Design
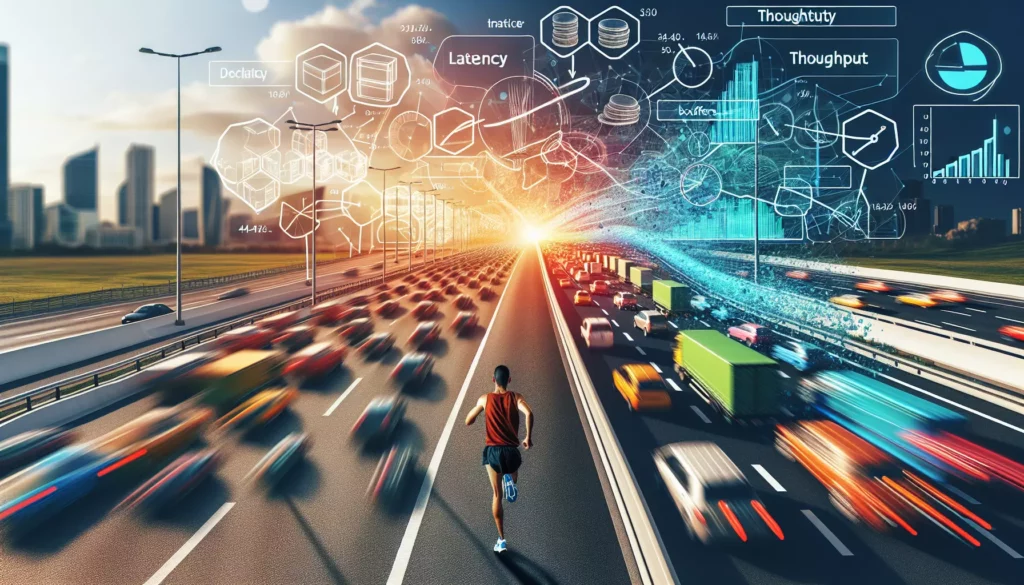
In the world of software engineering and system design, two critical concepts often take center stage: latency and throughput. These performance metrics play a crucial role in determining the efficiency and effectiveness of any system, from small applications to large-scale distributed systems. As aspiring software engineers and coding enthusiasts, understanding these concepts is essential for designing robust and high-performing systems. In this comprehensive guide, we’ll dive deep into the intricacies of latency and throughput, exploring their significance, measurement, and optimization techniques in system design.
Understanding Latency
Latency refers to the time delay between an input or request and the corresponding output or response. In simpler terms, it’s the time it takes for data to travel from its source to its destination. Latency is typically measured in units of time, such as milliseconds (ms) or microseconds (μs).
Types of Latency
- Network Latency: The delay in data transmission over a network.
- Processing Latency: The time taken by a system to process a request or perform a computation.
- Storage Latency: The delay in reading or writing data to storage devices.
Factors Affecting Latency
- Physical distance between nodes
- Network congestion
- Processing power of systems
- Data size and complexity
- Storage technology (e.g., SSD vs. HDD)
Understanding Throughput
Throughput is the rate at which a system can process or transmit data. It represents the amount of work that can be done in a given time period. Throughput is typically measured in units of data per unit time, such as bits per second (bps) or transactions per second (TPS).
Types of Throughput
- Network Throughput: The rate at which data can be transmitted over a network.
- Processing Throughput: The rate at which a system can process requests or perform computations.
- Storage Throughput: The rate at which data can be read from or written to storage devices.
Factors Affecting Throughput
- Available bandwidth
- System resources (CPU, memory, etc.)
- Concurrency and parallelism
- Data size and complexity
- System architecture and design
The Relationship Between Latency and Throughput
Latency and throughput are closely related but distinct concepts. While it’s tempting to think that reducing latency will automatically increase throughput (or vice versa), the relationship is more nuanced. In some cases, optimizing for one metric may come at the expense of the other.
Key Points to Consider:
- Trade-offs: Improving latency might reduce throughput, and vice versa. For example, batching requests can increase throughput but may introduce additional latency.
- System Bottlenecks: Identifying and addressing bottlenecks can improve both latency and throughput simultaneously.
- Workload Characteristics: The nature of the workload (e.g., read-heavy vs. write-heavy) can influence the balance between latency and throughput optimization.
Measuring Latency and Throughput
Accurate measurement of latency and throughput is crucial for understanding system performance and identifying areas for improvement. Here are some common methods and tools for measuring these metrics:
Measuring Latency
- Ping: A simple tool for measuring network latency.
- Traceroute: Helps identify the path and latency between network nodes.
- Application Performance Monitoring (APM) tools: Provide detailed insights into application-level latency.
Measuring Throughput
- iperf: A tool for measuring network throughput.
- Benchmark tools: Specialized tools for measuring system-specific throughput (e.g., database benchmarks).
- Load testing tools: Simulate real-world scenarios to measure system throughput under various conditions.
Example: Measuring Network Latency with Ping
Here’s a simple example of using the ping command to measure network latency:
$ ping google.com
PING google.com (172.217.16.142) 56(84) bytes of data.
64 bytes from lhr25s10-in-f14.1e100.net (172.217.16.142): icmp_seq=1 ttl=117 time=8.43 ms
64 bytes from lhr25s10-in-f14.1e100.net (172.217.16.142): icmp_seq=2 ttl=117 time=8.39 ms
64 bytes from lhr25s10-in-f14.1e100.net (172.217.16.142): icmp_seq=3 ttl=117 time=8.40 ms
64 bytes from lhr25s10-in-f14.1e100.net (172.217.16.142): icmp_seq=4 ttl=117 time=8.38 ms
--- google.com ping statistics ---
4 packets transmitted, 4 received, 0% packet loss, time 3004ms
rtt min/avg/max/mdev = 8.382/8.401/8.430/0.019 ms
In this example, we can see that the average round-trip time (latency) to google.com is approximately 8.4 ms.
Optimization Techniques for Latency and Throughput
Optimizing latency and throughput is an ongoing process in system design. Here are some common techniques to improve these metrics:
Latency Optimization
- Caching: Implement caching mechanisms to reduce the need for repeated computations or data retrieval.
- Content Delivery Networks (CDNs): Use CDNs to bring content closer to end-users, reducing network latency.
- Asynchronous Processing: Implement asynchronous operations to prevent blocking and improve responsiveness.
- Database Optimization: Use indexing, query optimization, and denormalization techniques to improve database performance.
- Load Balancing: Distribute traffic across multiple servers to prevent overload and reduce processing latency.
Throughput Optimization
- Horizontal Scaling: Add more servers or nodes to increase overall system capacity.
- Vertical Scaling: Upgrade hardware resources (CPU, memory, storage) to improve single-node performance.
- Parallelization: Implement parallel processing techniques to handle multiple tasks simultaneously.
- Batching: Group multiple operations or requests to reduce overhead and improve efficiency.
- Compression: Use data compression techniques to reduce the amount of data transferred over the network.
Example: Implementing Caching in Python
Here’s a simple example of how caching can be implemented in Python to improve latency for expensive computations:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
# First call (not cached)
print(fibonacci(100))
# Second call (cached result)
print(fibonacci(100))
In this example, the @lru_cache
decorator is used to cache the results of the Fibonacci function. The first call will compute the result, while subsequent calls for the same input will return the cached value, significantly reducing latency.
Case Studies: Latency and Throughput in Real-World Systems
To better understand the practical implications of latency and throughput optimization, let’s examine a few real-world case studies:
1. Google Search
Google’s search engine is renowned for its low latency and high throughput. Some key strategies they employ include:
- Distributed indexing and serving infrastructure
- Extensive use of caching at various levels
- Predictive pre-fetching of results
- Continuous optimization of search algorithms
2. Netflix Streaming
Netflix has optimized its streaming service for both latency and throughput:
- Use of adaptive bitrate streaming to adjust video quality based on network conditions
- Extensive CDN infrastructure to bring content closer to users
- Predictive caching of popular content on local ISP servers
- Efficient video encoding techniques to reduce data transfer
3. High-Frequency Trading Systems
In the world of financial trading, ultra-low latency is critical:
- Co-location of servers with exchange data centers
- Use of specialized hardware and network equipment
- Optimization of trading algorithms for minimal processing time
- Custom low-latency protocols for data transmission
Challenges in Balancing Latency and Throughput
While optimizing for both latency and throughput is ideal, it’s not always straightforward. Here are some common challenges:
- Resource Constraints: Limited hardware resources may force trade-offs between latency and throughput optimizations.
- Scalability: Maintaining low latency while scaling for higher throughput can be challenging.
- Consistency vs. Performance: In distributed systems, ensuring data consistency often comes at the cost of increased latency.
- Network Limitations: Physical network constraints (e.g., speed of light) can impose limits on latency reduction.
- Workload Variability: Optimizing for specific workload patterns may lead to suboptimal performance for other patterns.
Future Trends in Latency and Throughput Optimization
As technology continues to evolve, new approaches to latency and throughput optimization are emerging:
- Edge Computing: Moving computation closer to data sources and end-users to reduce network latency.
- 5G Networks: Leveraging high-speed, low-latency 5G networks for improved mobile and IoT performance.
- Quantum Computing: Exploring quantum algorithms for certain problems that could dramatically improve processing speed.
- AI-Driven Optimization: Using machine learning techniques to dynamically optimize system performance based on real-time data.
- New Hardware Architectures: Developing specialized hardware (e.g., GPUs, TPUs) for specific high-performance computing tasks.
Best Practices for Latency and Throughput Optimization
To wrap up our discussion, here are some best practices to keep in mind when optimizing systems for latency and throughput:
- Measure and Monitor: Continuously measure and monitor latency and throughput metrics to identify issues and improvement opportunities.
- Understand Your Workload: Analyze your system’s workload characteristics to determine the most appropriate optimization strategies.
- Set Clear Goals: Define specific latency and throughput targets based on your application’s requirements and user expectations.
- Optimize Incrementally: Start with the most significant bottlenecks and optimize incrementally, measuring the impact of each change.
- Consider Trade-offs: Be aware of the potential trade-offs between latency, throughput, cost, and other system characteristics.
- Design for Scalability: Ensure your system architecture can scale horizontally to handle increased load while maintaining performance.
- Use Appropriate Tools: Leverage profiling tools, APM solutions, and benchmarking frameworks to gain insights into system performance.
- Stay Updated: Keep abreast of new technologies and techniques in the field of performance optimization.
Conclusion
Latency and throughput are fundamental concepts in system design that significantly impact the performance and user experience of software applications. By understanding these metrics, their relationship, and various optimization techniques, developers and system architects can create more efficient and scalable systems.
As you continue your journey in software engineering and prepare for technical interviews, remember that a deep understanding of latency and throughput will not only help you design better systems but also demonstrate your expertise to potential employers. Practice analyzing and optimizing systems for these metrics, and you’ll be well-prepared to tackle complex system design challenges in your future career.
Keep exploring, experimenting, and pushing the boundaries of what’s possible in system performance. The field of latency and throughput optimization is constantly evolving, and there’s always more to learn and discover. Happy coding!