The Role of Hash Functions and Collision Handling in Modern Programming
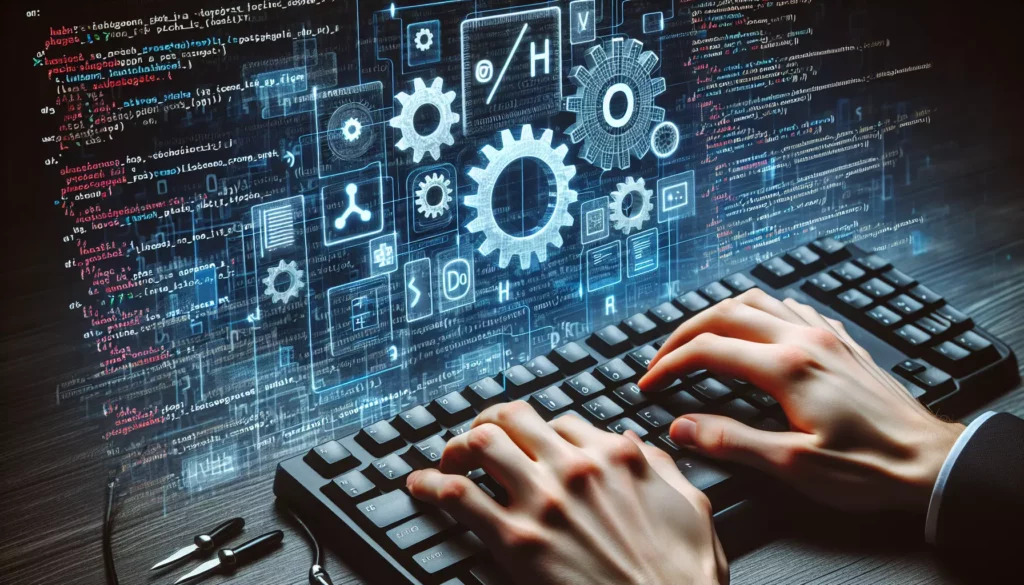
In the world of computer science and programming, hash functions and collision handling play a crucial role in various applications, from data structures to cryptography. Understanding these concepts is essential for any aspiring programmer, especially those preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll dive deep into the world of hash functions and collision handling, exploring their importance, implementations, and real-world applications.
What are Hash Functions?
Hash functions are mathematical algorithms that take an input (or “message”) and return a fixed-size string of bytes. The output, called a hash value or digest, is typically a fixed-length string that appears random and is (ideally) unique to the input data. Hash functions are designed to be fast to compute and deterministic, meaning the same input will always produce the same output.
Key properties of hash functions include:
- Determinism: The same input always produces the same output
- Fixed output size: Regardless of input size, the output is always the same length
- Efficiency: Hash functions should be quick to compute
- Uniformity: The output should be evenly distributed across the possible range of values
- One-way function: It should be computationally infeasible to reverse the hash to obtain the original input
Common Hash Functions
There are numerous hash functions in use today, each with its own strengths and applications. Some of the most common include:
1. MD5 (Message Digest algorithm 5)
MD5 is a widely used hash function that produces a 128-bit hash value. While it’s still used in some non-cryptographic applications, it’s considered cryptographically broken and unsuitable for security purposes.
2. SHA (Secure Hash Algorithm) family
The SHA family includes several hash functions designed by the NSA. SHA-256 is currently one of the most popular and secure options, producing a 256-bit hash value.
3. bcrypt
bcrypt is a password hashing function designed to be slow and computationally expensive, making it resistant to brute-force attacks. It’s widely used for securely storing passwords.
4. MurmurHash
MurmurHash is a non-cryptographic hash function known for its simplicity, speed, and good distribution properties. It’s often used in hash tables and bloom filters.
Implementing a Simple Hash Function
Let’s implement a basic hash function in Python to illustrate the concept:
def simple_hash(input_string, table_size):
hash_value = 0
for char in input_string:
hash_value = (hash_value * 31 + ord(char)) % table_size
return hash_value
# Example usage
table_size = 1000
input_string = "Hello, World!"
hash_result = simple_hash(input_string, table_size)
print(f"Hash of '{input_string}': {hash_result}")
This simple hash function uses the polynomial rolling hash method. It iterates through each character in the input string, multiplying the current hash value by a prime number (31 in this case) and adding the ASCII value of the character. The result is then taken modulo the table size to ensure it fits within the desired range.
The Importance of Collision Handling
Despite our best efforts to create perfect hash functions, collisions are inevitable. A collision occurs when two different inputs produce the same hash value. This is especially problematic in hash tables, where we use hash functions to determine the storage location of key-value pairs.
There are two main approaches to handling collisions:
1. Separate Chaining
In separate chaining, each bucket in the hash table contains a linked list (or another data structure) of elements that hash to that bucket. When a collision occurs, the new element is simply added to the list at that bucket.
Here’s a simple implementation of separate chaining in Python:
class HashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(self.size)]
def _hash(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
item[1] = value
return
self.table[index].append([key, value])
def get(self, key):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
return item[1]
raise KeyError(key)
def remove(self, key):
index = self._hash(key)
for i, item in enumerate(self.table[index]):
if item[0] == key:
del self.table[index][i]
return
raise KeyError(key)
# Example usage
ht = HashTable(10)
ht.insert("apple", 5)
ht.insert("banana", 7)
ht.insert("cherry", 3)
print(ht.get("banana")) # Output: 7
ht.remove("apple")
print(ht.get("apple")) # Raises KeyError
2. Open Addressing
In open addressing, when a collision occurs, we search for the next available slot in the hash table. There are several probing techniques used in open addressing:
- Linear Probing: Check the next slot sequentially until an empty slot is found.
- Quadratic Probing: Use a quadratic function to determine the next slot to check.
- Double Hashing: Use a second hash function to determine the step size for probing.
Here’s an example of linear probing in Python:
class HashTable:
def __init__(self, size):
self.size = size
self.keys = [None] * self.size
self.values = [None] * self.size
def _hash(self, key):
return hash(key) % self.size
def _find_slot(self, key):
index = self._hash(key)
while self.keys[index] is not None and self.keys[index] != key:
index = (index + 1) % self.size
return index
def insert(self, key, value):
index = self._find_slot(key)
self.keys[index] = key
self.values[index] = value
def get(self, key):
index = self._find_slot(key)
if self.keys[index] is None:
raise KeyError(key)
return self.values[index]
def remove(self, key):
index = self._find_slot(key)
if self.keys[index] is None:
raise KeyError(key)
self.keys[index] = None
self.values[index] = None
# Example usage
ht = HashTable(10)
ht.insert("apple", 5)
ht.insert("banana", 7)
ht.insert("cherry", 3)
print(ht.get("banana")) # Output: 7
ht.remove("apple")
print(ht.get("apple")) # Raises KeyError
Applications of Hash Functions and Collision Handling
Hash functions and collision handling techniques have numerous applications in computer science and software development. Some of the most common use cases include:
1. Hash Tables
Hash tables are one of the most important data structures in computer science, offering O(1) average-case time complexity for insertions, deletions, and lookups. They’re used in various applications, including:
- Implementing associative arrays and dictionaries
- Caching mechanisms in databases and web applications
- Symbol tables in compilers and interpreters
- Implementing sets with fast membership tests
2. Cryptography
Cryptographic hash functions play a crucial role in various security applications:
- Password storage: Storing hashed passwords instead of plaintext
- Digital signatures: Verifying the integrity and authenticity of messages
- Blockchain technology: Creating unique identifiers for blocks and transactions
- File integrity checking: Verifying that files haven’t been tampered with
3. Load Balancing
Hash functions are used in distributed systems to determine which server should handle a particular request, ensuring an even distribution of load across multiple servers.
4. Bloom Filters
Bloom filters are probabilistic data structures that use hash functions to test whether an element is a member of a set. They’re used in various applications, including:
- Spell checkers
- Cache filtering
- Network routers for packet routing
- Database systems to reduce disk lookups
5. Data Deduplication
Hash functions are used to identify duplicate data in storage systems, allowing for efficient deduplication and storage optimization.
Best Practices and Considerations
When working with hash functions and implementing collision handling strategies, keep the following best practices in mind:
1. Choose the Right Hash Function
Select a hash function appropriate for your use case. For cryptographic applications, use well-established and secure hash functions like SHA-256 or bcrypt. For non-cryptographic uses, faster hash functions like MurmurHash may be more suitable.
2. Consider Load Factor
The load factor of a hash table (the ratio of occupied slots to total slots) affects performance. Keep the load factor below 0.7 to 0.8 to maintain good performance, resizing the table when necessary.
3. Implement Resizing
Implement a resizing mechanism for your hash table to maintain performance as the number of elements grows. Typically, this involves doubling the size of the table and rehashing all elements when the load factor exceeds a certain threshold.
4. Test for Collisions
When implementing a custom hash function, thoroughly test it with various inputs to ensure it produces a uniform distribution and minimizes collisions.
5. Security Considerations
For security-sensitive applications, be aware of potential attacks like hash collision attacks or timing attacks. Use cryptographically secure hash functions and implement additional security measures as needed.
Conclusion
Hash functions and collision handling are fundamental concepts in computer science with wide-ranging applications. From optimizing data structures to securing sensitive information, these techniques play a crucial role in modern software development. As you prepare for technical interviews and advance your programming skills, a solid understanding of hash functions and collision handling will serve you well in solving complex problems and designing efficient systems.
Remember that mastering these concepts requires practice and hands-on experience. Implement your own hash tables, experiment with different hash functions, and explore real-world applications to deepen your understanding. With dedication and persistence, you’ll be well-equipped to tackle challenging coding problems and excel in your programming career.