The Role of Graph Algorithms in Solving Complex Coding Problems
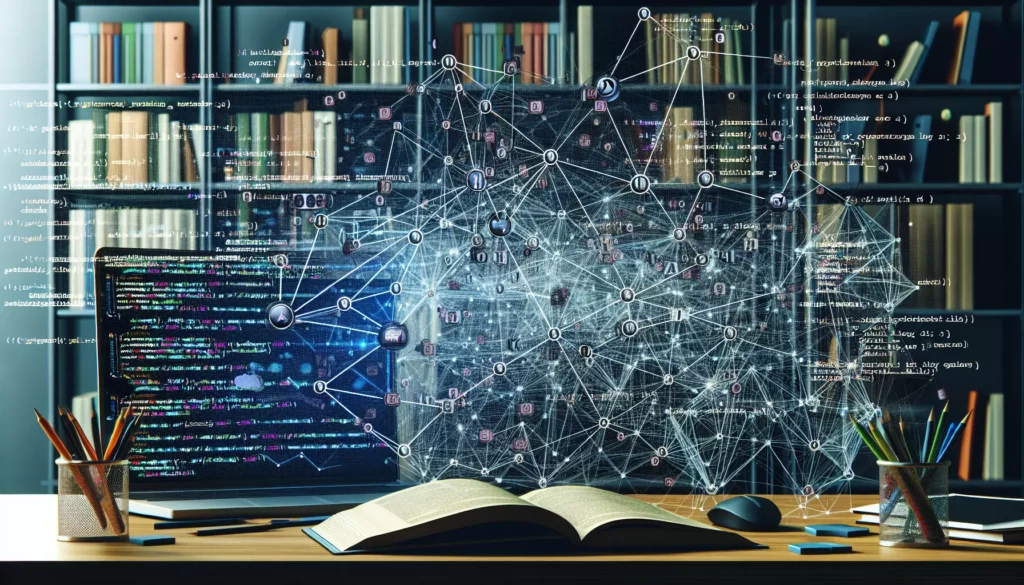
In the world of computer science and programming, graph algorithms play a crucial role in solving complex coding problems. These algorithms are essential tools for tackling a wide range of challenges, from optimizing network flows to finding the shortest path between two points. As aspiring developers and coding enthusiasts progress in their journey, understanding and mastering graph algorithms becomes increasingly important, especially when preparing for technical interviews at major tech companies.
In this comprehensive guide, we’ll explore the significance of graph algorithms in problem-solving, discuss various types of graph algorithms, and provide practical examples of how they can be applied to real-world coding challenges. By the end of this article, you’ll have a solid understanding of how graph algorithms can enhance your problem-solving skills and help you tackle complex coding problems with confidence.
Understanding Graphs and Their Importance
Before diving into specific algorithms, it’s essential to understand what graphs are and why they’re so important in computer science.
What is a Graph?
A graph is a data structure consisting of a set of vertices (also called nodes) and a set of edges that connect these vertices. Graphs can be used to represent a wide variety of relationships and connections in the real world, such as:
- Social networks (where vertices represent people and edges represent friendships)
- Road networks (where vertices represent intersections and edges represent roads)
- Computer networks (where vertices represent devices and edges represent connections)
- Dependency relationships in software projects
- State machines in game development
Why are Graphs Important in Coding?
Graphs are versatile data structures that can model complex relationships and interactions. Many real-world problems can be represented as graph problems, making graph algorithms essential for solving a wide range of coding challenges. Some key reasons why graphs are important in coding include:
- Modeling relationships: Graphs can represent various types of relationships between entities, making them ideal for solving problems involving connections or dependencies.
- Optimization: Many optimization problems, such as finding the shortest path or minimizing costs, can be solved efficiently using graph algorithms.
- Network analysis: Graphs are crucial for analyzing and optimizing network structures, whether they’re social networks, computer networks, or transportation networks.
- Data organization: Graphs can be used to organize and structure data in ways that make it easier to process and analyze.
- Algorithm design: Understanding graph algorithms can help in designing efficient solutions for complex problems that may not initially appear to be graph-related.
Common Types of Graph Algorithms
Now that we understand the importance of graphs, let’s explore some common types of graph algorithms and their applications in solving coding problems.
1. Breadth-First Search (BFS)
Breadth-First Search is a graph traversal algorithm that explores all the vertices of a graph in breadth-first order, i.e., it visits all the neighbors of a vertex before moving to the next level.
Applications:
- Finding the shortest path in an unweighted graph
- Web crawling
- Social network analysis (e.g., finding degrees of separation)
- Puzzle solving (e.g., solving a Rubik’s cube)
Example Implementation:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=" ")
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal starting from vertex 'A':")
bfs(graph, 'A')
2. Depth-First Search (DFS)
Depth-First Search is another graph traversal algorithm that explores as far as possible along each branch before backtracking. It goes deep into the graph before exploring other branches.
Applications:
- Detecting cycles in a graph
- Topological sorting
- Solving maze problems
- Finding connected components in a graph
Example Implementation:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=" ")
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("DFS traversal starting from vertex 'A':")
dfs(graph, 'A')
3. Dijkstra’s Algorithm
Dijkstra’s algorithm is used to find the shortest path between nodes in a graph with non-negative edge weights. It’s particularly useful for solving problems involving weighted graphs.
Applications:
- Finding the shortest route in a road network
- Network routing protocols
- Solving problems involving minimum cost or time
Example Implementation:
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'A': 4, 'D': 3, 'E': 1},
'C': {'A': 2, 'F': 5},
'D': {'B': 3},
'E': {'B': 1, 'F': 2},
'F': {'C': 5, 'E': 2}
}
print("Shortest distances from 'A':")
print(dijkstra(graph, 'A'))
4. Bellman-Ford Algorithm
The Bellman-Ford algorithm is used to find the shortest paths from a single source vertex to all other vertices in a weighted graph. Unlike Dijkstra’s algorithm, it can handle graphs with negative edge weights.
Applications:
- Detecting negative cycles in a graph
- Solving problems involving arbitrage in currency exchange
- Network routing protocols that can handle negative weights
Example Implementation:
def bellman_ford(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
for _ in range(len(graph) - 1):
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
distances[neighbor] = distances[node] + weight
# Check for negative cycles
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
print("Graph contains a negative cycle")
return None
return distances
# Example usage
graph = {
'A': {'B': -1, 'C': 4},
'B': {'C': 3, 'D': 2, 'E': 2},
'C': {},
'D': {'B': 1, 'C': 5},
'E': {'D': -3}
}
print("Shortest distances from 'A':")
print(bellman_ford(graph, 'A'))
5. Floyd-Warshall Algorithm
The Floyd-Warshall algorithm is used to find the shortest paths between all pairs of vertices in a weighted graph. It can handle both positive and negative edge weights, but not negative cycles.
Applications:
- Finding the transitive closure of a graph
- Solving the all-pairs shortest path problem
- Inversion of real matrices
Example Implementation:
def floyd_warshall(graph):
distances = {node: {inner_node: float('infinity') for inner_node in graph} for node in graph}
for node in graph:
distances[node][node] = 0
for neighbor, weight in graph[node].items():
distances[node][neighbor] = weight
for k in graph:
for i in graph:
for j in graph:
distances[i][j] = min(distances[i][j], distances[i][k] + distances[k][j])
return distances
# Example usage
graph = {
'A': {'B': 3, 'C': 6, 'D': 15},
'B': {'A': 3, 'C': 2, 'D': 4},
'C': {'A': 6, 'B': 2, 'D': 5},
'D': {'A': 15, 'B': 4, 'C': 5}
}
print("All-pairs shortest distances:")
result = floyd_warshall(graph)
for node in result:
print(f"{node}: {result[node]}")
Applying Graph Algorithms to Solve Complex Coding Problems
Now that we’ve covered some of the most common graph algorithms, let’s explore how they can be applied to solve complex coding problems. We’ll look at a few examples that demonstrate the power of graph algorithms in tackling real-world challenges.
Problem 1: Social Network Analysis
Imagine you’re working on a social networking platform and need to implement a “friend suggestion” feature. You want to suggest friends of friends who are not already connected to the user.
This problem can be solved using a combination of Breadth-First Search (BFS) and set operations:
from collections import deque
def suggest_friends(graph, user, max_distance=2):
visited = set()
queue = deque([(user, 0)])
visited.add(user)
suggestions = set()
while queue:
current_user, distance = queue.popleft()
if distance > max_distance:
break
for friend in graph[current_user]:
if friend not in visited:
visited.add(friend)
queue.append((friend, distance + 1))
if distance > 0:
suggestions.add(friend)
# Remove direct friends from suggestions
suggestions -= set(graph[user])
return suggestions
# Example usage
social_graph = {
'Alice': ['Bob', 'Charlie'],
'Bob': ['Alice', 'David', 'Eve'],
'Charlie': ['Alice', 'Frank'],
'David': ['Bob', 'George'],
'Eve': ['Bob', 'Frank'],
'Frank': ['Charlie', 'Eve'],
'George': ['David']
}
user = 'Alice'
print(f"Friend suggestions for {user}:")
print(suggest_friends(social_graph, user))
In this example, we use BFS to explore the user’s social network up to a certain distance (in this case, friends of friends). We then use set operations to remove direct friends and return the suggestions.
Problem 2: Optimal Route Planning
Suppose you’re developing a navigation app and need to find the optimal route between two locations, considering both distance and traffic conditions.
This problem can be solved using Dijkstra’s algorithm with a custom weight function that takes into account both distance and traffic:
import heapq
def optimal_route(graph, start, end, traffic_multiplier=1.5):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
previous = {node: None for node in graph}
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
break
if current_distance > distances[current_node]:
continue
for neighbor, (distance, traffic) in graph[current_node].items():
weight = distance * (1 + (traffic_multiplier - 1) * traffic)
total_distance = current_distance + weight
if total_distance < distances[neighbor]:
distances[neighbor] = total_distance
previous[neighbor] = current_node
heapq.heappush(pq, (total_distance, neighbor))
# Reconstruct the path
path = []
current = end
while current:
path.append(current)
current = previous[current]
path.reverse()
return path, distances[end]
# Example usage
road_network = {
'A': {'B': (10, 0.2), 'C': (15, 0.1)},
'B': {'A': (10, 0.2), 'D': (12, 0.3), 'E': (15, 0.4)},
'C': {'A': (15, 0.1), 'F': (10, 0.5)},
'D': {'B': (12, 0.3), 'F': (1, 0.9)},
'E': {'B': (15, 0.4), 'F': (5, 0.1)},
'F': {'C': (10, 0.5), 'D': (1, 0.9), 'E': (5, 0.1)}
}
start, end = 'A', 'F'
optimal_path, total_cost = optimal_route(road_network, start, end)
print(f"Optimal route from {start} to {end}:")
print(f"Path: {' -> '.join(optimal_path)}")
print(f"Total cost: {total_cost:.2f}")
In this example, we use Dijkstra’s algorithm with a custom weight function that considers both distance and traffic conditions. The traffic_multiplier
parameter allows us to adjust the importance of traffic in route planning.
Problem 3: Detecting Cycles in a Dependency Graph
In software development, it’s crucial to detect circular dependencies in a project’s module structure. This problem can be solved using Depth-First Search (DFS) to detect cycles in a directed graph.
def has_cycle(graph):
visited = set()
recursion_stack = set()
def dfs(node):
visited.add(node)
recursion_stack.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
if dfs(neighbor):
return True
elif neighbor in recursion_stack:
return True
recursion_stack.remove(node)
return False
for node in graph:
if node not in visited:
if dfs(node):
return True
return False
# Example usage
dependency_graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': ['E'],
'E': ['B'] # This creates a cycle: B -> D -> E -> B
}
print("Does the dependency graph have a cycle?")
print(has_cycle(dependency_graph))
In this example, we use DFS to traverse the graph and keep track of nodes in the current recursion stack. If we encounter a node that’s already in the recursion stack, we’ve found a cycle.
Advanced Graph Algorithms and Techniques
As you progress in your coding journey and tackle more complex problems, you may encounter situations that require more advanced graph algorithms and techniques. Here are a few examples:
1. Minimum Spanning Trees
Minimum Spanning Tree (MST) algorithms, such as Kruskal’s and Prim’s algorithms, are used to find a subset of edges that connect all vertices in a weighted, undirected graph with the minimum possible total edge weight.
Applications:
- Network design (e.g., designing efficient computer or electrical networks)
- Clustering algorithms
- Image segmentation in computer vision
2. Maximum Flow Algorithms
Maximum flow algorithms, such as the Ford-Fulkerson algorithm and its improved version, the Edmonds-Karp algorithm, are used to find the maximum flow in a flow network.
Applications:
- Transportation and logistics optimization
- Bipartite matching problems
- Network capacity planning
3. Strongly Connected Components
Algorithms for finding strongly connected components in a directed graph, such as Kosaraju’s algorithm or Tarjan’s algorithm, are useful for analyzing the structure of complex networks.
Applications:
- Analyzing social networks
- Solving 2-satisfiability problems
- Identifying cycles in directed graphs
4. Graph Coloring
Graph coloring algorithms are used to assign colors to vertices or edges of a graph such that no two adjacent vertices or edges have the same color.
Applications:
- Scheduling problems (e.g., exam timetabling)
- Register allocation in compiler optimization
- Solving Sudoku puzzles
Conclusion
Graph algorithms play a crucial role in solving complex coding problems across various domains. By understanding and mastering these algorithms, you’ll be better equipped to tackle a wide range of challenges in your coding journey and technical interviews.
As you continue to develop your skills, remember that graph algorithms are just one piece of the puzzle. To become a well-rounded programmer, it’s essential to:
- Practice implementing these algorithms from scratch to deepen your understanding
- Solve diverse coding problems that require applying graph algorithms in creative ways
- Study the time and space complexity of different algorithms to make informed choices
- Explore real-world applications of graph algorithms in various industries
- Stay updated with new developments in graph theory and algorithms
By incorporating graph algorithms into your problem-solving toolkit, you’ll be better prepared to tackle complex coding challenges and excel in technical interviews at major tech companies. Remember that mastering these concepts takes time and practice, so be patient with yourself and enjoy the learning process.
As you continue your coding education journey, consider exploring additional resources, such as interactive coding tutorials and AI-powered assistance tools, to further enhance your skills in graph algorithms and other essential programming concepts. With dedication and consistent practice, you’ll be well on your way to becoming a proficient problem solver and a valuable asset to any development team.