The Role of Critical Thinking in Software Development
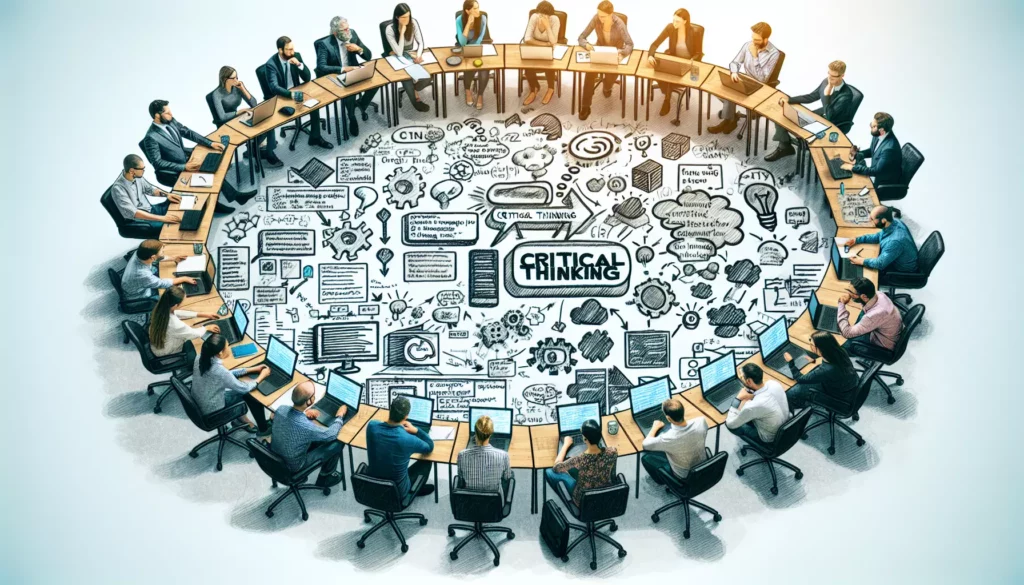
In the ever-evolving world of technology, software development stands as a cornerstone of innovation and progress. At the heart of this field lies a crucial skill that often separates great developers from good ones: critical thinking. As we delve into the intricate relationship between critical thinking and software development, we’ll explore how this cognitive skill not only enhances coding abilities but also shapes the entire development process.
Understanding Critical Thinking in the Context of Software Development
Critical thinking, in its essence, is the ability to analyze information objectively and make reasoned judgments. In software development, this translates to the capacity to approach problems methodically, evaluate multiple solutions, and make informed decisions that lead to efficient and effective code.
For developers, critical thinking encompasses several key aspects:
- Problem analysis and decomposition
- Logical reasoning and algorithmic thinking
- Evaluating trade-offs between different approaches
- Anticipating potential issues and edge cases
- Continuously questioning and improving existing solutions
These aspects of critical thinking are not just beneficial but essential in navigating the complex landscape of software development, especially when preparing for technical interviews at major tech companies like those in FAANG (Facebook, Amazon, Apple, Netflix, Google).
The Impact of Critical Thinking on Coding Skills
When it comes to writing code, critical thinking plays a pivotal role in several ways:
1. Problem-Solving Approach
Critical thinkers in software development don’t just jump into coding. They take a step back to analyze the problem at hand. This involves breaking down complex issues into smaller, manageable parts – a process known as decomposition. By doing so, developers can tackle each component systematically, leading to more comprehensive and robust solutions.
For example, when faced with a complex algorithm challenge, a developer might:
- Identify the core problem and desired outcome
- Break down the problem into smaller sub-problems
- Analyze each sub-problem individually
- Develop solutions for each part
- Integrate these solutions into a cohesive whole
2. Algorithmic Thinking
Critical thinking is closely tied to algorithmic thinking – the ability to create step-by-step solutions to problems. This skill is crucial in developing efficient algorithms, which are the backbone of any software application. By applying critical thinking, developers can:
- Evaluate the efficiency of different algorithms
- Optimize code for better performance
- Choose the most appropriate data structures for specific problems
Consider the following example of how critical thinking can lead to more efficient code:
// Less efficient approach
function findDuplicates(arr) {
let duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// More efficient approach using critical thinking
function findDuplicatesOptimized(arr) {
let seen = new Set();
let duplicates = new Set();
for (let num of arr) {
if (seen.has(num)) {
duplicates.add(num);
} else {
seen.add(num);
}
}
return Array.from(duplicates);
}
In this example, critical thinking led to the use of a Set data structure, reducing the time complexity from O(n^2) to O(n).
3. Code Quality and Maintainability
Critical thinkers in software development are not satisfied with code that merely works; they strive for code that is clean, efficient, and maintainable. This involves:
- Writing clear and self-documenting code
- Structuring code for readability and reusability
- Implementing proper error handling and edge case management
- Adhering to coding standards and best practices
By applying critical thinking to code quality, developers ensure that their solutions are not only functional but also sustainable in the long term.
Critical Thinking in the Software Development Lifecycle
The influence of critical thinking extends beyond just writing code. It plays a crucial role throughout the entire software development lifecycle:
1. Requirements Analysis
In the initial stages of a project, critical thinking helps developers:
- Ask the right questions to clarify project requirements
- Identify potential conflicts or inconsistencies in specifications
- Anticipate future needs and scalability issues
This foresight can save significant time and resources in later stages of development.
2. Design and Architecture
When designing software architecture, critical thinking enables developers to:
- Evaluate different architectural patterns and their suitability for the project
- Consider scalability, maintainability, and performance implications
- Make informed decisions about technology stack and frameworks
For instance, when deciding between a monolithic and microservices architecture, a developer would critically analyze factors such as team size, project complexity, scalability needs, and deployment strategies.
3. Testing and Quality Assurance
Critical thinking is essential in creating comprehensive test strategies. It helps in:
- Identifying potential edge cases and failure scenarios
- Designing effective unit tests and integration tests
- Analyzing test results and debugging complex issues
A critical thinker might approach testing with a mindset of “How can this break?” rather than just confirming that it works under ideal conditions.
4. Code Review and Collaboration
In team environments, critical thinking enhances the code review process by:
- Providing constructive feedback on code quality and design choices
- Identifying potential improvements or optimizations
- Fostering meaningful discussions about best practices and coding standards
This collaborative aspect of critical thinking contributes to the overall growth and skill development of the entire team.
Developing Critical Thinking Skills for Software Development
Given the importance of critical thinking in software development, it’s crucial for developers to actively cultivate this skill. Here are some strategies to enhance critical thinking abilities:
1. Practice Problem-Solving
Engaging in coding challenges and algorithmic problems is an excellent way to sharpen critical thinking skills. Platforms like AlgoCademy offer a wide range of problems that simulate real-world scenarios and technical interview questions from major tech companies.
When solving these problems, focus on:
- Understanding the problem thoroughly before coding
- Considering multiple approaches and their trade-offs
- Analyzing the time and space complexity of your solutions
2. Embrace Continuous Learning
The field of software development is constantly evolving. Stay updated with:
- New programming languages and frameworks
- Emerging design patterns and architectural styles
- Best practices in software engineering
This continuous learning process enhances your ability to think critically about new technologies and their applications.
3. Analyze and Reflect on Your Code
After completing a project or solving a problem, take time to reflect:
- Could the solution be more efficient?
- Is the code as clear and maintainable as it could be?
- What lessons can be applied to future projects?
This self-reflection is a crucial aspect of developing critical thinking skills.
4. Seek and Provide Code Reviews
Actively participating in code reviews, both as a reviewer and a reviewee, can significantly enhance critical thinking skills. It exposes you to different perspectives and approaches to problem-solving.
5. Study Design Patterns and Algorithms
A deep understanding of design patterns and algorithms provides a foundation for critical thinking in software development. It equips you with a toolkit of proven solutions and the knowledge to apply them judiciously.
Critical Thinking in Technical Interviews
For those aspiring to work at major tech companies, critical thinking skills are particularly crucial during technical interviews. These interviews often focus on assessing a candidate’s problem-solving abilities and thought processes rather than just their coding skills.
Approaching Interview Questions
When faced with a technical interview question, apply critical thinking by:
- Clarifying the problem and asking relevant questions
- Discussing multiple approaches before settling on a solution
- Explaining your thought process as you work through the problem
- Analyzing the efficiency of your solution and considering optimizations
For example, consider this common interview question:
// Question: Given an array of integers, find two numbers such that they add up to a specific target number.
function findTwoSum(nums, target) {
// Your solution here
}
A critical thinker might approach this problem as follows:
- Clarify: “Can the array contain duplicate numbers? Can we assume the array is sorted?”
- Consider approaches:
- Brute force: nested loops (O(n^2) time complexity)
- Sorting and two-pointer technique (O(n log n) time complexity)
- Hash map approach (O(n) time complexity, O(n) space complexity)
- Choose and implement the most efficient solution:
function findTwoSum(nums, target) { let map = new Map(); for (let i = 0; i < nums.length; i++) { let complement = target - nums[i]; if (map.has(complement)) { return [map.get(complement), i]; } map.set(nums[i], i); } return []; // No solution found }
- Explain the choice: “This solution uses a hash map to achieve O(n) time complexity, trading some space for improved time efficiency.”
- Discuss potential optimizations or variations of the problem.
The Future of Critical Thinking in Software Development
As technology continues to advance, the role of critical thinking in software development is likely to become even more crucial. Here are some trends that underscore its importance:
1. Artificial Intelligence and Machine Learning
With the rise of AI and ML, developers need to think critically about:
- Ethical implications of AI algorithms
- Bias in training data and model outputs
- Interpreting and explaining complex ML models
2. Increased Complexity of Software Systems
As software systems grow more complex, critical thinking skills will be essential for:
- Managing and integrating diverse technologies
- Designing scalable and resilient architectures
- Troubleshooting intricate, distributed systems
3. Rapid Technological Change
The fast-paced evolution of technology requires developers to:
- Quickly evaluate and adopt new tools and frameworks
- Critically assess the long-term viability of emerging technologies
- Balance innovation with stability in software projects
Conclusion
Critical thinking is not just a valuable skill in software development; it’s an essential component of a developer’s toolkit. From writing efficient code to navigating complex system architectures, from acing technical interviews to shaping the future of technology, critical thinking underpins every aspect of a software developer’s journey.
As we’ve explored, critical thinking enhances problem-solving abilities, improves code quality, and drives innovation in software development. It’s a skill that distinguishes exceptional developers and is highly sought after by leading tech companies.
For those looking to advance their careers in software development, particularly those aiming for positions at major tech companies, honing critical thinking skills should be a top priority. Platforms like AlgoCademy offer valuable resources and challenges that can help developers sharpen these skills, preparing them for the complex problems they’ll face in their careers.
Remember, critical thinking in software development is not just about finding solutions; it’s about finding the best solutions. It’s about asking the right questions, challenging assumptions, and continuously striving for improvement. As you continue your journey in software development, make critical thinking a cornerstone of your approach, and watch as it opens doors to new opportunities and innovations in your career.