The Role of Code Refactoring in Continuous Improvement: A Comprehensive Guide
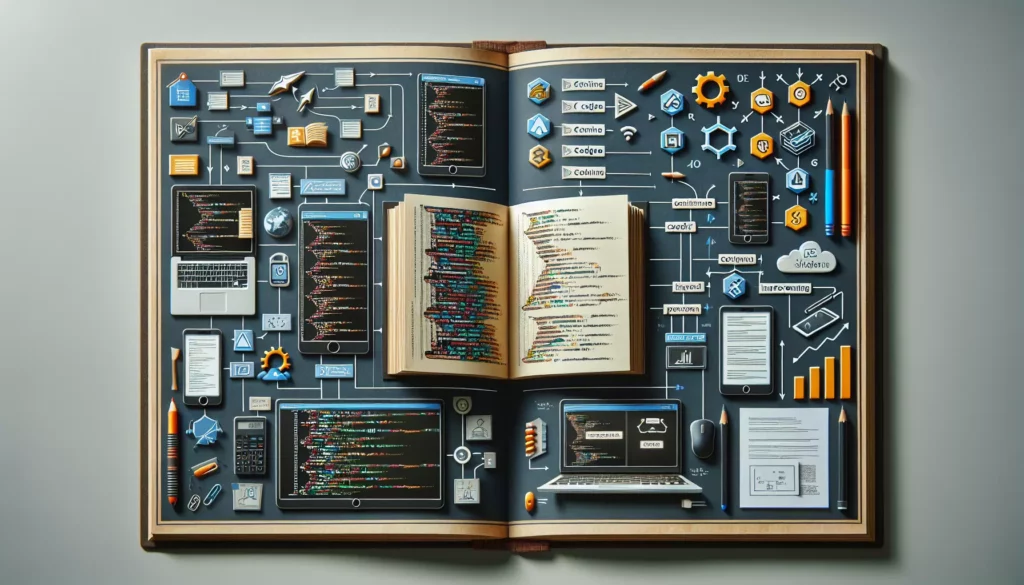
In the ever-evolving world of software development, the ability to write clean, efficient, and maintainable code is a crucial skill. As projects grow in complexity and scale, the need for continuous improvement becomes increasingly apparent. One of the most powerful tools in a developer’s arsenal for achieving this improvement is code refactoring. In this comprehensive guide, we’ll explore the role of code refactoring in continuous improvement, its benefits, techniques, and best practices.
What is Code Refactoring?
Code refactoring is the process of restructuring existing computer code without changing its external behavior. It’s about improving the internal structure of the code while preserving its functionality. The primary goal of refactoring is to enhance code quality, making it easier to understand, maintain, and extend.
Refactoring is not about fixing bugs or adding new features. Instead, it focuses on improving the design, structure, and implementation of the code. This process can involve simplifying complex logic, removing redundancies, improving naming conventions, and reorganizing code to enhance its overall clarity and efficiency.
The Importance of Continuous Improvement in Software Development
Continuous improvement is a fundamental principle in modern software development methodologies. It emphasizes the ongoing effort to enhance various aspects of the development process, including code quality, team productivity, and overall project efficiency. In the context of coding, continuous improvement involves regularly reviewing and refining code to ensure it remains clean, efficient, and adaptable to changing requirements.
The need for continuous improvement arises from several factors:
- Evolving technology and best practices
- Changing project requirements
- Accumulation of technical debt
- Growing complexity of software systems
- Need for better performance and scalability
Code refactoring plays a crucial role in this continuous improvement process by providing a systematic approach to enhancing code quality over time.
Benefits of Code Refactoring
Incorporating regular code refactoring into your development process offers numerous benefits:
1. Improved Code Readability and Maintainability
Refactoring helps in simplifying complex code structures, making them easier to read and understand. This improved readability directly translates to better maintainability, as developers can more quickly grasp the code’s purpose and make necessary changes or fixes.
2. Enhanced Performance
By optimizing code structure and eliminating redundancies, refactoring can lead to performance improvements. This is particularly important for large-scale applications where even small optimizations can have significant impacts on overall system performance.
3. Reduced Technical Debt
Technical debt accumulates when developers choose quick solutions over better approaches that would take longer to implement. Regular refactoring helps in addressing this debt by gradually improving the code quality and structure.
4. Easier Bug Detection and Fixing
Clean, well-structured code makes it easier to identify and fix bugs. Refactoring can help uncover hidden bugs and make the debugging process more efficient.
5. Improved Scalability
Refactored code is typically more modular and loosely coupled, making it easier to scale and extend the application as requirements evolve.
6. Better Team Collaboration
When code is clean and well-organized, it’s easier for team members to collaborate, understand each other’s work, and contribute to different parts of the project.
Common Code Refactoring Techniques
There are numerous techniques for refactoring code. Here are some of the most common ones:
1. Extract Method
This technique involves taking a code fragment that can be grouped together and turning it into a method. This improves code organization and reusability.
Before refactoring:
def calculate_total_price(items):
total = 0
for item in items:
if item.type == 'book':
total += item.price * 0.9 # 10% discount on books
else:
total += item.price
print(f"Total price: ${total}")
return total
After refactoring:
def calculate_item_price(item):
if item.type == 'book':
return item.price * 0.9 # 10% discount on books
return item.price
def calculate_total_price(items):
total = sum(calculate_item_price(item) for item in items)
print(f"Total price: ${total}")
return total
2. Rename Method
This involves changing the name of a method to better reflect its purpose. Good naming is crucial for code readability.
Before refactoring:
def process(data):
# Complex data processing logic
pass
After refactoring:
def normalize_and_filter_data(data):
# Complex data processing logic
pass
3. Replace Temp with Query
This technique involves replacing temporary variables with a method call. This can make the code more readable and reduce duplication.
Before refactoring:
base_price = quantity * item_price
if base_price > 1000:
discount = base_price * 0.95
else:
discount = base_price * 0.98
After refactoring:
def base_price():
return quantity * item_price
def discount_factor():
return 0.95 if base_price() > 1000 else 0.98
discount = base_price() * discount_factor()
4. Introduce Parameter Object
When a group of parameters are passed together frequently, they can be replaced with an object.
Before refactoring:
def create_order(customer_name, customer_address, product_id, quantity):
# Order creation logic
pass
After refactoring:
class OrderDetails:
def __init__(self, customer_name, customer_address, product_id, quantity):
self.customer_name = customer_name
self.customer_address = customer_address
self.product_id = product_id
self.quantity = quantity
def create_order(order_details):
# Order creation logic
pass
5. Replace Conditional with Polymorphism
This technique involves replacing complex conditional logic with polymorphic behavior, typically using inheritance or interfaces.
Before refactoring:
class Animal:
def make_sound(self, animal_type):
if animal_type == 'dog':
return 'Woof'
elif animal_type == 'cat':
return 'Meow'
elif animal_type == 'cow':
return 'Moo'
After refactoring:
class Animal:
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return 'Woof'
class Cat(Animal):
def make_sound(self):
return 'Meow'
class Cow(Animal):
def make_sound(self):
return 'Moo'
Best Practices for Code Refactoring
To ensure that your refactoring efforts are effective and don’t introduce new problems, consider the following best practices:
1. Refactor Incrementally
Make small, incremental changes rather than trying to refactor large portions of code at once. This approach reduces the risk of introducing errors and makes it easier to track and revert changes if necessary.
2. Maintain a Solid Test Suite
Before refactoring, ensure you have a comprehensive set of tests in place. These tests will help you verify that your refactoring hasn’t altered the code’s behavior.
3. Use Version Control
Always use version control systems like Git when refactoring. This allows you to easily track changes, create branches for experimental refactoring, and revert if needed.
4. Follow the Boy Scout Rule
The Boy Scout Rule states: “Always leave the code better than you found it.” Apply this principle by making small improvements whenever you work on a piece of code.
5. Understand the Code Before Refactoring
Make sure you fully understand the code and its context before attempting to refactor it. Refactoring without proper understanding can lead to unintended consequences.
6. Use Automated Refactoring Tools
Many modern IDEs offer automated refactoring tools. These can help perform common refactoring operations safely and efficiently.
7. Document Your Refactoring
Keep track of significant refactoring efforts, especially those that impact the overall architecture or design. This documentation can be valuable for team communication and future maintenance.
Challenges in Code Refactoring
While refactoring offers numerous benefits, it’s not without its challenges:
1. Time Constraints
Refactoring takes time, and in fast-paced development environments, it can be challenging to allocate sufficient time for this process.
2. Risk of Introducing Bugs
Without proper care and testing, refactoring can inadvertently introduce new bugs or alter existing functionality.
3. Resistance to Change
In team settings, there might be resistance to refactoring, especially if the code is currently working “well enough.”
4. Scope Creep
It’s easy for refactoring efforts to expand beyond their initial scope, potentially leading to unnecessary changes or delays.
5. Legacy Code
Refactoring legacy code can be particularly challenging, especially if it lacks proper documentation or tests.
Integrating Refactoring into the Development Process
To make refactoring a regular part of your development process:
1. Include Refactoring in Sprint Planning
Allocate time for refactoring tasks in your sprint planning sessions. This ensures that refactoring is given proper consideration and resources.
2. Implement Code Reviews
Use code reviews as an opportunity to identify areas that need refactoring. Encourage team members to suggest improvements during these reviews.
3. Set Coding Standards
Establish and enforce coding standards within your team. This can help prevent the need for extensive refactoring in the future.
4. Use Static Code Analysis Tools
Integrate static code analysis tools into your CI/CD pipeline to automatically identify areas that might benefit from refactoring.
5. Encourage Continuous Learning
Promote continuous learning within your team about new refactoring techniques and best practices in software design.
Measuring the Impact of Refactoring
To justify the time and effort spent on refactoring, it’s important to measure its impact. Some metrics to consider include:
- Code complexity metrics (e.g., cyclomatic complexity)
- Code duplication levels
- Test coverage
- Build and deployment times
- Number of bugs reported
- Time spent on maintenance tasks
Regularly tracking these metrics can help demonstrate the value of refactoring efforts over time.
Conclusion
Code refactoring is a crucial component of continuous improvement in software development. By regularly refining and optimizing code, developers can create more maintainable, efficient, and scalable software systems. While refactoring comes with its challenges, the long-term benefits in terms of code quality, developer productivity, and system performance are substantial.
As you continue your journey in software development, remember that refactoring is not a one-time task but an ongoing process. By integrating refactoring into your regular development workflow and following best practices, you can ensure that your codebase remains clean, efficient, and adaptable to changing requirements.
Whether you’re a beginner learning the ropes of coding or an experienced developer preparing for technical interviews at major tech companies, mastering the art of code refactoring will undoubtedly enhance your skills and make you a more valuable asset to any development team.