The Role of Algorithms in Problem Solving: A Comprehensive Guide
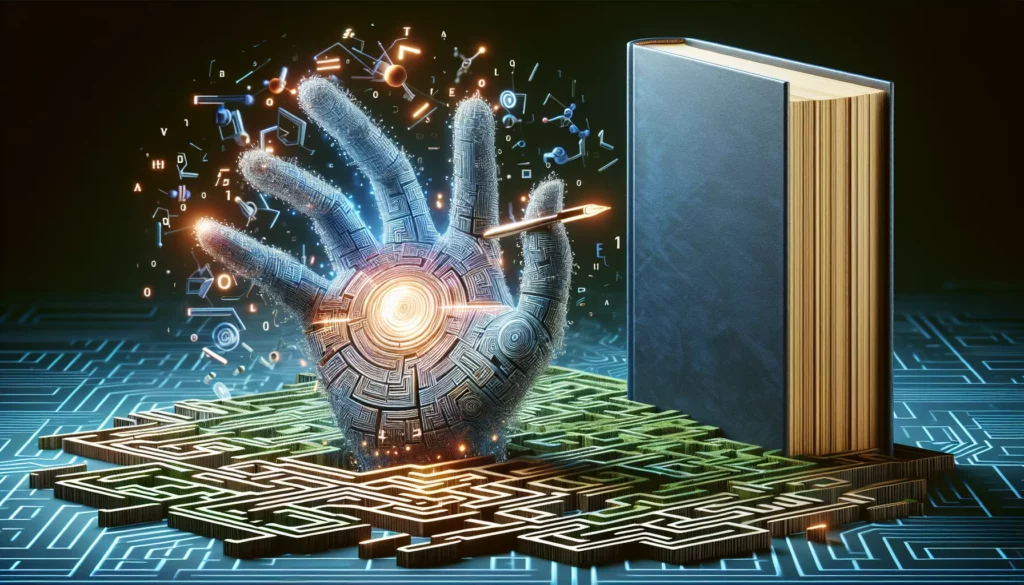
In the ever-evolving world of technology and computer science, algorithms play a pivotal role in problem-solving. Whether you’re a beginner coder or an experienced developer preparing for technical interviews at major tech companies, understanding the importance of algorithms is crucial. This comprehensive guide will explore the role of algorithms in problem-solving, their significance in coding education, and how they contribute to the development of essential programming skills.
What Are Algorithms?
Before delving into the role of algorithms in problem-solving, it’s essential to understand what algorithms are. An algorithm is a step-by-step procedure or formula for solving a problem or accomplishing a task. In computer science, algorithms are the foundation for all programming and are used to manipulate data, sort information, make calculations, and perform various other tasks.
Algorithms can be simple, like a recipe for baking a cake, or complex, like the algorithms used by search engines to return relevant results. Regardless of their complexity, all algorithms share some common characteristics:
- They have a clearly defined set of inputs and outputs
- Each step in the algorithm is precisely defined
- They produce a result in a finite amount of time
- They are effective, meaning they solve the problem they were designed to address
The Importance of Algorithms in Problem Solving
Algorithms are essential in problem-solving for several reasons:
1. Efficiency
One of the primary reasons algorithms are crucial in problem-solving is their ability to improve efficiency. A well-designed algorithm can significantly reduce the time and resources required to solve a problem. This is particularly important when dealing with large datasets or complex calculations.
For example, consider the task of finding a specific name in a phonebook. A naive approach would be to start from the beginning and check each name until you find the one you’re looking for. However, an efficient algorithm like binary search can dramatically reduce the number of steps required by repeatedly dividing the search area in half.
2. Consistency
Algorithms provide a consistent approach to problem-solving. Once an algorithm is developed and proven to work, it can be applied repeatedly to similar problems with consistent results. This consistency is crucial in many fields, from computer science to engineering and beyond.
3. Scalability
Well-designed algorithms can often scale to handle larger and more complex problems. This scalability is essential in today’s world, where we’re dealing with increasingly large datasets and complex systems. An algorithm that works efficiently for a small dataset should ideally be able to handle much larger datasets without a significant decrease in performance.
4. Problem Decomposition
Algorithms help break down complex problems into smaller, more manageable parts. This process of problem decomposition is a crucial skill in problem-solving and is particularly important in software development, where large projects are often broken down into smaller, more manageable modules or functions.
Algorithms in Coding Education
In the context of coding education, algorithms play a central role. Understanding and implementing algorithms is a fundamental skill that every programmer needs to develop. Here’s why algorithms are so important in coding education:
1. Foundational Knowledge
Algorithms form the foundation of computer science and programming. Learning about algorithms helps students understand how computers process information and solve problems. This foundational knowledge is crucial for developing more advanced programming skills.
2. Problem-Solving Skills
Studying algorithms helps develop problem-solving skills. By learning different algorithmic approaches, students learn how to analyze problems, break them down into smaller parts, and develop efficient solutions. These skills are transferable to many areas of life, not just programming.
3. Efficient Coding
Understanding algorithms helps programmers write more efficient code. By knowing which algorithm to use in different situations, developers can create programs that run faster and use fewer resources. This is particularly important when working on large-scale projects or with limited computing resources.
4. Preparation for Technical Interviews
Many technical interviews, especially at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), focus heavily on algorithms and data structures. Having a strong understanding of algorithms is often crucial for success in these interviews.
Common Types of Algorithms in Problem Solving
There are many types of algorithms used in problem-solving. Here are some of the most common:
1. Sorting Algorithms
Sorting algorithms are used to arrange data in a particular order, typically ascending or descending. Some common sorting algorithms include:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
Each of these algorithms has its own advantages and disadvantages in terms of time complexity and space complexity. For example, Bubble Sort is simple to implement but inefficient for large datasets, while Quick Sort is more complex but generally more efficient.
2. Searching Algorithms
Searching algorithms are used to find a particular item in a dataset. Common searching algorithms include:
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
The choice of searching algorithm often depends on whether the data is sorted and the size of the dataset. For example, Binary Search is very efficient for sorted data, while DFS and BFS are commonly used for graph traversal.
3. Dynamic Programming
Dynamic Programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s often used for optimization problems. The key idea is to store the results of subproblems so that we don’t have to recompute them when needed later.
A classic example of a problem solved using Dynamic Programming is the Fibonacci sequence. Instead of calculating each number in the sequence recursively (which can be very inefficient), we can use Dynamic Programming to store previously calculated values and reuse them.
4. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step with the hope of finding a global optimum. While they don’t always yield the best solution, they are often used for optimization problems where finding the exact solution would be too time-consuming.
An example of a problem often solved with a greedy algorithm is the “Coin Change” problem, where the goal is to make change for a given amount using the minimum number of coins.
5. Divide and Conquer
Divide and Conquer algorithms work by recursively breaking down a problem into two or more sub-problems of the same or related type, until these become simple enough to be solved directly. The solutions to the sub-problems are then combined to give a solution to the original problem.
Merge Sort and Quick Sort are examples of sorting algorithms that use the divide and conquer approach.
Implementing Algorithms: A Practical Example
To better understand how algorithms work in practice, let’s look at an implementation of the Binary Search algorithm in Python. Binary Search is an efficient algorithm for searching a sorted array by repeatedly dividing the search interval in half.
def binary_search(arr, x):
low = 0
high = len(arr) - 1
mid = 0
while low <= high:
mid = (high + low) // 2
# If x is greater, ignore left half
if arr[mid] < x:
low = mid + 1
# If x is smaller, ignore right half
elif arr[mid] > x:
high = mid - 1
# means x is present at mid
else:
return mid
# If we reach here, then the element was not present
return -1
# Test array
arr = [2, 3, 4, 10, 40]
x = 10
# Function call
result = binary_search(arr, x)
if result != -1:
print("Element is present at index", str(result))
else:
print("Element is not present in array")
This implementation of Binary Search demonstrates several key concepts:
- Problem decomposition: The problem of searching is broken down into smaller steps of comparing and adjusting the search range.
- Efficiency: By halving the search range in each step, Binary Search is much more efficient than a linear search for large datasets.
- Algorithm design: The algorithm is designed to handle all cases, including when the element is not in the array.
The Role of AI in Algorithm Learning and Problem Solving
Artificial Intelligence (AI) is playing an increasingly important role in algorithm learning and problem-solving. AI-powered tools can provide several benefits:
1. Personalized Learning
AI can analyze a student’s performance and learning style to provide personalized recommendations and exercises. This can help students focus on areas where they need the most improvement and learn at their own pace.
2. Immediate Feedback
AI-powered coding platforms can provide immediate feedback on code submissions. This includes not just identifying errors, but also suggesting improvements and more efficient solutions.
3. Problem Generation
AI can generate new problems based on a student’s skill level and learning goals. This ensures that students are always challenged with relevant and appropriately difficult problems.
4. Solution Analysis
AI can analyze submitted solutions to identify patterns and common mistakes. This can help educators understand where students are struggling and adjust their teaching accordingly.
Preparing for Technical Interviews with Algorithmic Thinking
For those preparing for technical interviews, particularly at major tech companies, a strong grasp of algorithms and problem-solving techniques is crucial. Here are some tips for preparing:
1. Practice Regularly
Consistent practice is key to improving your algorithmic thinking skills. Platforms like AlgoCademy, LeetCode, and HackerRank offer a wide range of problems to practice on.
2. Understand Time and Space Complexity
It’s not enough to solve the problem; you need to understand how efficient your solution is. Learn to analyze the time and space complexity of your algorithms using Big O notation.
3. Learn Multiple Approaches
For each problem, try to think of multiple ways to solve it. This will help you develop flexibility in your problem-solving approach.
4. Explain Your Thought Process
Practice explaining your thought process out loud as you solve problems. This is a crucial skill for technical interviews, where interviewers are often as interested in your problem-solving approach as they are in your final solution.
5. Review Core Data Structures
Make sure you have a solid understanding of core data structures like arrays, linked lists, trees, graphs, stacks, and queues. Many algorithmic problems involve manipulating these data structures.
The Future of Algorithms in Problem Solving
As technology continues to advance, the role of algorithms in problem-solving is likely to become even more important. Here are some trends to watch:
1. Machine Learning Algorithms
Machine learning algorithms are becoming increasingly important in many fields. These algorithms can learn from data and improve their performance over time without being explicitly programmed.
2. Quantum Algorithms
As quantum computing develops, new algorithms are being created to take advantage of the unique properties of quantum systems. These could potentially solve certain problems much faster than classical algorithms.
3. Blockchain Algorithms
With the rise of blockchain technology, there’s increasing focus on algorithms for consensus mechanisms, cryptographic hashing, and other blockchain-related problems.
4. Ethical Algorithms
As algorithms play an increasingly important role in decision-making systems, there’s growing emphasis on developing algorithms that are fair, transparent, and ethically sound.
Conclusion
Algorithms are at the heart of computer science and play a crucial role in problem-solving across many fields. From sorting and searching to machine learning and quantum computing, algorithms provide the frameworks we use to process information and make decisions.
For those learning to code or preparing for technical interviews, a strong understanding of algorithms is essential. It not only helps in writing efficient code but also develops critical thinking and problem-solving skills that are valuable in many areas of life.
As we look to the future, the importance of algorithms in problem-solving is only set to increase. By mastering algorithmic thinking, we equip ourselves with the tools to tackle the complex challenges of tomorrow’s technological landscape.
Remember, the journey to mastering algorithms is ongoing. Keep practicing, stay curious, and don’t be afraid to tackle challenging problems. With persistence and the right resources, you can develop the algorithmic thinking skills that are so valued in today’s tech industry.