The Role of Algorithms in Natural Language Processing: Unlocking the Power of Human-Computer Communication
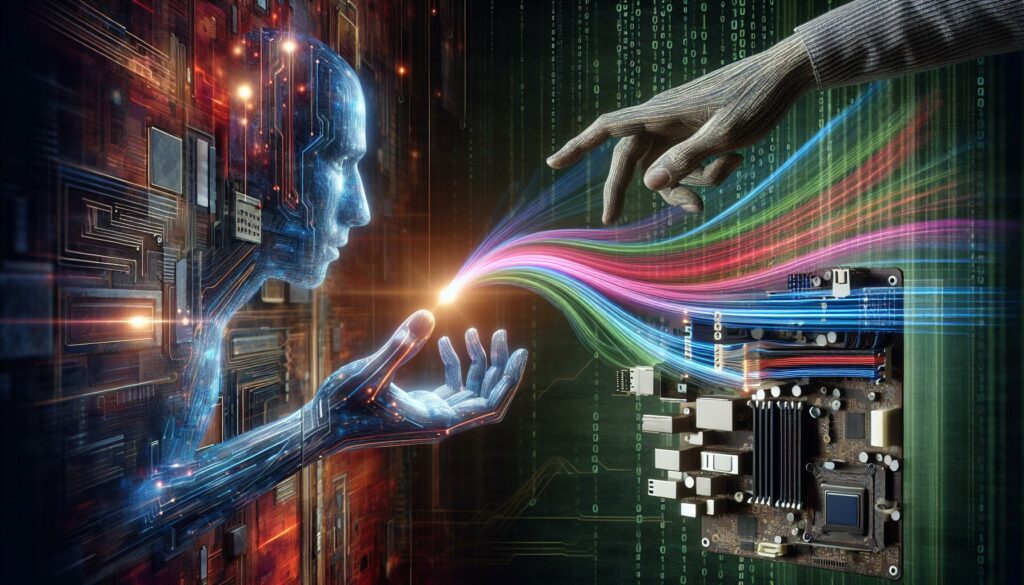
In the rapidly evolving world of technology, Natural Language Processing (NLP) has emerged as a crucial field that bridges the gap between human communication and computer understanding. At the heart of NLP lie powerful algorithms that enable machines to comprehend, interpret, and generate human language. This article delves deep into the role of algorithms in NLP, exploring their significance, types, and applications in various domains.
Understanding Natural Language Processing
Before we dive into the algorithms, it’s essential to grasp the concept of Natural Language Processing. NLP is a subfield of artificial intelligence (AI) and linguistics that focuses on the interaction between computers and human language. The ultimate goal of NLP is to enable computers to understand, interpret, and generate human language in a way that is both meaningful and useful.
NLP encompasses a wide range of tasks, including:
- Text classification
- Sentiment analysis
- Named entity recognition
- Machine translation
- Text summarization
- Question answering
- Speech recognition
- Text generation
To accomplish these tasks, NLP relies heavily on algorithms that can process and analyze large amounts of textual data efficiently.
The Importance of Algorithms in NLP
Algorithms play a crucial role in NLP for several reasons:
- Data Processing: NLP deals with vast amounts of unstructured text data. Algorithms help in efficiently processing and organizing this data for further analysis.
- Pattern Recognition: Algorithms can identify patterns and structures in language that might not be immediately apparent to humans.
- Scalability: With the exponential growth of digital text, algorithms allow NLP systems to scale and handle large volumes of data.
- Automation: NLP algorithms automate many language-related tasks, saving time and resources.
- Consistency: Unlike humans, algorithms can maintain consistency in their analysis and decision-making processes.
- Continuous Improvement: Machine learning algorithms in NLP can learn and improve their performance over time with more data and feedback.
Types of Algorithms Used in NLP
NLP employs a wide variety of algorithms, each suited for specific tasks or aspects of language processing. Let’s explore some of the most important types:
1. Tokenization Algorithms
Tokenization is often the first step in many NLP tasks. It involves breaking down text into smaller units called tokens, which can be words, characters, or subwords. Common tokenization algorithms include:
- Word tokenization
- Sentence tokenization
- Byte-Pair Encoding (BPE)
- WordPiece
Here’s a simple example of word tokenization in Python:
def simple_tokenize(text):
return text.split()
sentence = "Natural language processing is fascinating!"
tokens = simple_tokenize(sentence)
print(tokens)
# Output: ['Natural', 'language', 'processing', 'is', 'fascinating!']
2. Part-of-Speech (POS) Tagging Algorithms
POS tagging algorithms assign grammatical categories (such as noun, verb, adjective) to each word in a sentence. Popular algorithms for POS tagging include:
- Hidden Markov Models (HMM)
- Maximum Entropy Markov Models (MEMM)
- Conditional Random Fields (CRF)
3. Named Entity Recognition (NER) Algorithms
NER algorithms identify and classify named entities (e.g., person names, organizations, locations) in text. Common approaches include:
- Rule-based systems
- Machine learning-based methods (e.g., Conditional Random Fields)
- Deep learning models (e.g., Bidirectional LSTMs, Transformers)
4. Parsing Algorithms
Parsing algorithms analyze the grammatical structure of sentences. They are crucial for understanding the relationships between words and phrases. Types of parsing algorithms include:
- Constituency parsing (e.g., CKY algorithm, Earley parser)
- Dependency parsing (e.g., Transition-based parsing, Graph-based parsing)
5. Text Classification Algorithms
Text classification algorithms categorize text documents into predefined classes. Popular algorithms include:
- Naive Bayes
- Support Vector Machines (SVM)
- Random Forests
- Deep learning models (e.g., Convolutional Neural Networks, Recurrent Neural Networks)
Here’s a simple example of text classification using the Naive Bayes algorithm:
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.naive_bayes import MultinomialNB
from sklearn.model_selection import train_test_split
# Sample data
texts = ["I love this movie", "This film is terrible", "Great acting and plot"]
labels = ["positive", "negative", "positive"]
# Split the data
X_train, X_test, y_train, y_test = train_test_split(texts, labels, test_size=0.2)
# Vectorize the text
vectorizer = CountVectorizer()
X_train_vectorized = vectorizer.fit_transform(X_train)
X_test_vectorized = vectorizer.transform(X_test)
# Train the model
clf = MultinomialNB()
clf.fit(X_train_vectorized, y_train)
# Make predictions
predictions = clf.predict(X_test_vectorized)
print(predictions)
6. Word Embedding Algorithms
Word embedding algorithms transform words into dense vector representations, capturing semantic relationships. Popular word embedding techniques include:
- Word2Vec
- GloVe (Global Vectors for Word Representation)
- FastText
7. Language Model Algorithms
Language models predict the probability of a sequence of words. They are fundamental to many NLP tasks. Some important language model algorithms are:
- N-gram models
- Recurrent Neural Networks (RNNs)
- Long Short-Term Memory (LSTM) networks
- Transformer models (e.g., BERT, GPT)
8. Machine Translation Algorithms
Machine translation algorithms convert text from one language to another. Modern approaches include:
- Statistical Machine Translation (SMT)
- Neural Machine Translation (NMT) using sequence-to-sequence models
- Transformer-based models (e.g., Google’s Neural Machine Translation system)
9. Text Summarization Algorithms
Text summarization algorithms generate concise summaries of longer documents. There are two main approaches:
- Extractive summarization (selecting important sentences)
- Abstractive summarization (generating new sentences)
Algorithms used in text summarization include:
- TextRank (graph-based algorithm)
- Latent Semantic Analysis (LSA)
- Sequence-to-sequence models with attention
Deep Learning Algorithms in NLP
In recent years, deep learning algorithms have revolutionized the field of NLP. These algorithms, based on artificial neural networks, have achieved state-of-the-art performance on many NLP tasks. Some key deep learning architectures used in NLP include:
1. Recurrent Neural Networks (RNNs)
RNNs are designed to handle sequential data, making them well-suited for many NLP tasks. They process input sequences one element at a time, maintaining a hidden state that captures information from previous elements.
2. Long Short-Term Memory (LSTM) Networks
LSTMs are a type of RNN that addresses the vanishing gradient problem, allowing them to capture long-term dependencies in sequences. They are particularly effective for tasks like language modeling and machine translation.
3. Convolutional Neural Networks (CNNs)
While primarily used in computer vision, CNNs have also been applied to NLP tasks such as text classification and sentiment analysis. They can capture local patterns in text data.
4. Transformer Models
Transformer models, introduced in the paper “Attention Is All You Need,” have become the foundation for many state-of-the-art NLP systems. They use self-attention mechanisms to process input sequences in parallel, capturing long-range dependencies more efficiently than RNNs.
Some popular Transformer-based models include:
- BERT (Bidirectional Encoder Representations from Transformers)
- GPT (Generative Pre-trained Transformer)
- T5 (Text-to-Text Transfer Transformer)
Implementing NLP Algorithms: Practical Considerations
When implementing NLP algorithms, there are several practical considerations to keep in mind:
1. Data Preprocessing
Proper data preprocessing is crucial for the success of NLP algorithms. This may include:
- Lowercasing
- Removing punctuation and special characters
- Handling contractions
- Removing stop words
- Stemming or lemmatization
Here’s an example of basic text preprocessing in Python:
import re
import nltk
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
nltk.download('stopwords')
def preprocess_text(text):
# Lowercase the text
text = text.lower()
# Remove punctuation and special characters
text = re.sub(r'[^\w\s]', '', text)
# Tokenize the text
tokens = text.split()
# Remove stop words
stop_words = set(stopwords.words('english'))
tokens = [token for token in tokens if token not in stop_words]
# Stem the words
stemmer = PorterStemmer()
tokens = [stemmer.stem(token) for token in tokens]
return ' '.join(tokens)
# Example usage
text = "Natural Language Processing is fascinating! It's a powerful tool for analyzing text."
preprocessed_text = preprocess_text(text)
print(preprocessed_text)
# Output: natur languag process fascin power tool analyz text
2. Feature Engineering
Feature engineering involves creating meaningful representations of text data for machine learning algorithms. This may include:
- Bag-of-words representation
- TF-IDF (Term Frequency-Inverse Document Frequency)
- N-gram features
- Word embeddings
3. Model Selection and Hyperparameter Tuning
Choosing the right algorithm and tuning its hyperparameters is crucial for optimal performance. This often involves:
- Cross-validation
- Grid search or random search for hyperparameter optimization
- Ensemble methods
4. Handling Imbalanced Data
Many NLP tasks involve imbalanced datasets, where some classes are underrepresented. Techniques to address this include:
- Oversampling (e.g., SMOTE)
- Undersampling
- Class weighting
5. Evaluation Metrics
Choosing appropriate evaluation metrics is essential for assessing the performance of NLP algorithms. Common metrics include:
- Accuracy
- Precision, Recall, and F1-score
- BLEU score (for machine translation)
- ROUGE score (for text summarization)
- Perplexity (for language models)
Applications of NLP Algorithms
NLP algorithms have found applications in numerous domains, revolutionizing how we interact with technology and process information. Some key applications include:
1. Chatbots and Virtual Assistants
NLP algorithms power conversational AI systems, enabling them to understand user queries and generate appropriate responses. Companies like Apple (Siri), Google (Google Assistant), and Amazon (Alexa) heavily rely on NLP for their virtual assistants.
2. Machine Translation
Services like Google Translate use advanced NLP algorithms to provide real-time translation between hundreds of languages, breaking down language barriers in global communication.
3. Sentiment Analysis
Businesses use sentiment analysis algorithms to gauge public opinion about their products or services by analyzing social media posts, customer reviews, and other text data.
4. Information Retrieval
Search engines like Google employ sophisticated NLP algorithms to understand user queries and retrieve relevant information from vast amounts of web content.
5. Text Summarization
News aggregators and research tools use text summarization algorithms to provide concise overviews of long articles or documents, saving time for readers.
6. Content Recommendation
Streaming platforms like Netflix and content websites like Medium use NLP algorithms to analyze user preferences and recommend relevant content.
7. Healthcare
NLP is used in healthcare for tasks such as analyzing electronic health records, extracting information from medical literature, and assisting in clinical decision support systems.
8. Legal Document Analysis
Law firms and legal tech companies use NLP algorithms to analyze contracts, patents, and other legal documents, automating time-consuming manual reviews.
9. Automated Writing Assistance
Tools like Grammarly use NLP algorithms to provide writing suggestions, grammar corrections, and style improvements in real-time.
Challenges and Future Directions
While NLP has made significant strides, there are still several challenges and areas for future research:
1. Handling Ambiguity and Context
Human language is inherently ambiguous, and understanding context remains a significant challenge for NLP algorithms. Future research will focus on developing more context-aware models.
2. Multilingual and Cross-lingual NLP
Developing NLP systems that can work effectively across multiple languages and transfer knowledge between languages is an active area of research.
3. Common Sense Reasoning
Incorporating common sense knowledge into NLP models to enable more human-like understanding and reasoning is a major challenge.
4. Ethical Considerations
As NLP systems become more powerful, addressing ethical concerns such as bias, privacy, and the potential for misuse becomes increasingly important.
5. Efficiency and Resource Constraints
Developing more efficient NLP algorithms that can run on resource-constrained devices (e.g., mobile phones) is crucial for widespread adoption.
6. Explainability and Interpretability
Making complex NLP models more interpretable and explainable is essential for building trust and enabling their use in critical applications.
Conclusion
Algorithms play a pivotal role in Natural Language Processing, enabling machines to understand, interpret, and generate human language with increasing sophistication. From traditional statistical methods to cutting-edge deep learning approaches, NLP algorithms have revolutionized how we interact with technology and process vast amounts of textual data.
As the field continues to evolve, we can expect even more advanced algorithms that push the boundaries of what’s possible in human-computer communication. The future of NLP holds exciting possibilities, from more natural and context-aware language understanding to seamless multilingual communication and beyond.
For aspiring programmers and AI enthusiasts, mastering NLP algorithms is not just about learning technical skills; it’s about unlocking the potential to create technologies that can truly understand and interact with humans in meaningful ways. As we continue to refine and develop these algorithms, we move closer to a world where the language barrier between humans and machines becomes increasingly blurred, opening up new frontiers in artificial intelligence and human-computer interaction.