The Psychological Stages of Grief After Failing a LeetCode Easy
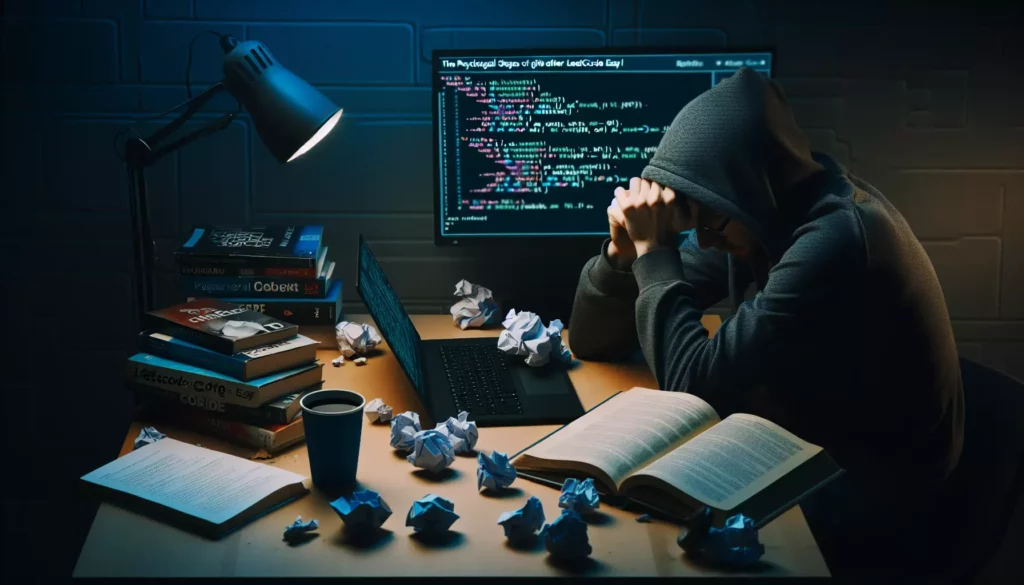
Picture this: You’ve been coding for months, maybe even years. You’ve tackled complex projects, debugged gnarly issues, and even impressed your peers with your programming prowess. Feeling confident, you decide it’s time to take on the legendary LeetCode, the proving ground for aspiring software engineers. You click on an “Easy” problem, thinking, “I’ve got this in the bag.” But then… disaster strikes. Your code fails, your confidence crumbles, and you’re left staring at the screen in disbelief. Welcome to the psychological rollercoaster of failing a LeetCode Easy problem.
In this deep dive, we’ll explore the emotional journey that many programmers experience when faced with unexpected challenges in their coding journey. We’ll walk through the stages of grief, offer coping strategies, and ultimately show you how to turn this seemingly devastating experience into a powerful learning opportunity.
The Five Stages of LeetCode Grief
Just as with any significant loss, failing a LeetCode Easy problem can trigger a grieving process. Let’s break down these stages and see how they manifest in the world of coding challenges:
1. Denial: “This Can’t Be Happening”
The first stage hits you like a ton of bricks. You’re in disbelief. Thoughts race through your mind:
- “There must be a mistake in the test cases.”
- “My code is perfect; the problem must be broken.”
- “Maybe I accidentally clicked on a Medium or Hard problem?”
You frantically double-check the problem description, convinced that you’ve missed some crucial detail. You run your code locally, and it works fine. So why is LeetCode telling you it’s wrong?
This denial stage is a natural defense mechanism. Your brain is trying to protect your ego from the harsh reality that you’ve stumbled on what should have been an easy hurdle. It’s okay to spend a little time here, but don’t get stuck – acceptance is the key to moving forward.
2. Anger: “This is Ridiculous!”
As the reality of the situation sets in, denial gives way to anger. You might find yourself:
- Cursing at your computer screen
- Ranting to your rubber duck (or any unfortunate soul within earshot)
- Leaving passive-aggressive comments on the problem discussion board
Your internal monologue might sound something like this: “Who designed this stupid problem anyway? I’ve been coding for years; I don’t need this!” The anger stage is fueled by frustration and a sense of injustice. After all, you’re a competent programmer, right? How dare a simple problem make you feel this way!
While it’s natural to feel angry, it’s important to channel this energy productively. Instead of lashing out, try to use this passion to fuel your determination to solve the problem.
3. Bargaining: “If Only…”
As the anger subsides, you enter the bargaining stage. This is where you start making deals with yourself or the coding gods:
- “If I can solve this in the next 30 minutes, I’ll never procrastinate on my coding practice again.”
- “Maybe if I just try one more time with a slight tweak, it’ll work.”
- “I’ll watch every LeetCode tutorial video if I can just figure this out.”
This stage is characterized by a desperate attempt to regain control over the situation. You might find yourself obsessively tweaking your code, hoping that the next minor change will be the magical solution. It’s during this stage that many programmers fall into the trap of “solution tunneling” – getting so fixated on their original approach that they can’t see alternative solutions.
4. Depression: “I’m Just Not Cut Out for This”
When bargaining fails to yield results, depression sets in. This is the low point of your LeetCode journey, where self-doubt creeps in and negative thoughts dominate:
- “Maybe I’m not as good at programming as I thought.”
- “I’ll never pass a technical interview if I can’t even solve an Easy problem.”
- “Why did I even think I could be a software engineer?”
During this stage, you might feel tempted to give up on LeetCode altogether. The challenge that once seemed exciting now feels insurmountable. It’s crucial to remember that these feelings are temporary and not reflective of your true abilities or potential.
5. Acceptance: “Okay, Let’s Figure This Out”
Finally, you reach the stage of acceptance. This doesn’t mean you’re happy about failing the problem, but you’re ready to approach it with a clear head. In this stage, you might find yourself thinking:
- “Alright, I missed something. Let’s break this down step by step.”
- “This is an opportunity to learn and improve my skills.”
- “Everyone struggles sometimes. It’s part of the learning process.”
Acceptance is where real growth begins. You’re now in a mental state where you can objectively analyze the problem, seek help if needed, and learn from the experience.
Turning Failure into a Learning Opportunity
Now that we’ve explored the emotional journey, let’s talk about how to transform this seemingly negative experience into a powerful catalyst for growth and learning.
1. Analyze Your Approach
Take a step back and critically examine your initial solution. Ask yourself:
- What assumptions did I make about the problem?
- Did I consider all possible edge cases?
- Is there a more efficient algorithm I could have used?
Sometimes, the simplest problems can trip us up because we overcomplicate them or miss a crucial detail. By analyzing your approach, you can identify areas for improvement in your problem-solving process.
2. Study the Problem Discussion
LeetCode’s problem discussions are a goldmine of information. After giving the problem your best shot, dive into the discussions to see how others approached it. You might discover:
- Alternative algorithms you hadn’t considered
- Clever optimizations that improve runtime or memory usage
- Common pitfalls that many programmers encounter
Remember, there’s no shame in learning from others. Even experienced programmers regularly consult problem discussions to broaden their knowledge and improve their skills.
3. Practice Debugging Skills
Failing a problem is an excellent opportunity to sharpen your debugging skills. Instead of immediately looking at the solution, try to debug your code:
- Use print statements or a debugger to track variable values
- Test your code with different inputs, including edge cases
- Break your solution down into smaller functions and test each one individually
These debugging techniques won’t just help you solve the current problem; they’ll make you a more effective programmer in all your future endeavors.
4. Revisit Fundamental Concepts
Sometimes, failing an Easy problem can reveal gaps in your fundamental knowledge. Use this as an opportunity to revisit and strengthen your understanding of core concepts:
- Review data structures like arrays, linked lists, and hash tables
- Brush up on basic algorithms such as searching and sorting
- Practice time and space complexity analysis
By solidifying your foundation, you’ll be better equipped to tackle not just this problem, but future challenges as well.
5. Embrace the Growth Mindset
Perhaps the most important takeaway from this experience is the cultivation of a growth mindset. Remember:
- Every failure is an opportunity to learn and improve
- Your current abilities are not fixed; with practice, you can enhance your skills
- Challenges are not threats, but chances to grow stronger
By reframing your perspective on failure, you can turn each setback into a stepping stone towards success.
Practical Steps to Overcome LeetCode Challenges
Now that we’ve addressed the emotional aspects and the learning opportunities, let’s look at some practical steps you can take to overcome LeetCode challenges, especially when you’re feeling stuck:
1. Break Down the Problem
Often, the key to solving a tricky problem is to break it down into smaller, manageable parts. Try this approach:
- Clearly define the input and expected output
- Identify the main components or steps needed to solve the problem
- Tackle each component individually
- Combine the solutions to these sub-problems into a complete solution
Here’s an example of how you might break down a simple string manipulation problem:
Problem: Reverse words in a string
1. Split the string into words
2. Reverse each word
3. Join the reversed words back into a string
# Python implementation
def reverse_words(s):
# Step 1: Split the string into words
words = s.split()
# Step 2: Reverse each word
reversed_words = [word[::-1] for word in words]
# Step 3: Join the reversed words back into a string
return " ".join(reversed_words)
# Test the function
print(reverse_words("Hello World")) # Output: "olleH dlroW"
2. Use Pseudocode
Before diving into coding, write out your algorithm in pseudocode. This helps you focus on the logic without getting bogged down in syntax details. For example:
Problem: Find the maximum subarray sum
Pseudocode:
1. Initialize variables:
- max_sum = first element of array
- current_sum = first element of array
2. Iterate through the array starting from the second element:
- Add current element to current_sum
- If current_sum is greater than max_sum, update max_sum
- If current_sum becomes negative, reset it to 0
3. Return max_sum
# Python implementation
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
print(max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4])) # Output: 6
3. Start with a Brute Force Solution
If you’re struggling to come up with an optimal solution, start with a brute force approach. While it may not be efficient, it can help you understand the problem better and potentially lead to insights for optimization. Here’s an example:
Problem: Find two numbers in an array that add up to a target sum
# Brute force solution
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
# More efficient solution using a hash table
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the functions
nums = [2, 7, 11, 15]
target = 9
print(two_sum_brute_force(nums, target)) # Output: [0, 1]
print(two_sum_optimized(nums, target)) # Output: [0, 1]
4. Learn to Use LeetCode’s Built-in Tools
LeetCode provides several tools to help you debug and optimize your code:
- Custom Test Cases: Use these to test your code with different inputs, including edge cases.
- Runtime and Memory Usage Analysis: After submitting a solution, review these metrics to understand how your code performs compared to others.
- Code Playground: Experiment with different approaches without affecting your submission history.
Familiarizing yourself with these tools can significantly enhance your problem-solving efficiency on the platform.
The Importance of Consistency and Patience
As you navigate the challenges of LeetCode and coding interviews, it’s crucial to remember that improvement takes time and consistent effort. Here are some tips to help you stay motivated and make steady progress:
1. Establish a Routine
Set aside dedicated time each day or week for LeetCode practice. Consistency is key to building and maintaining your skills. Even 30 minutes a day can lead to significant improvements over time.
2. Track Your Progress
Keep a log of the problems you’ve attempted, noting:
- The problem name and difficulty level
- Whether you solved it independently or needed help
- Key concepts or techniques used in the solution
- Areas for improvement or further study
Reviewing this log periodically can help you see your progress and identify areas that need more attention.
3. Join a Community
Engaging with other learners can provide motivation, support, and valuable insights. Consider:
- Joining LeetCode discussion forums
- Participating in coding challenges or contests
- Finding a study buddy or forming a study group
Sharing your experiences and learning from others can make the journey more enjoyable and productive.
4. Celebrate Small Wins
Don’t just focus on the problems you can’t solve. Celebrate your successes, no matter how small. Solved an Easy problem without help? That’s worth celebrating! Improved your runtime on a Medium problem? Pat yourself on the back!
5. Take Breaks and Manage Stress
Burnout is real, especially when tackling challenging problems. Remember to:
- Take regular breaks to rest your mind
- Practice stress-management techniques like deep breathing or meditation
- Engage in other activities you enjoy to maintain a balanced lifestyle
A refreshed mind is more capable of tackling complex problems and retaining new information.
Conclusion: Embracing the Journey
Failing a LeetCode Easy problem can be a humbling experience, but it’s also an invaluable part of your growth as a programmer. By understanding and navigating the psychological stages of grief that often accompany such failures, you can transform these moments of frustration into powerful learning opportunities.
Remember, every experienced programmer has faced similar challenges. What sets successful developers apart is not their ability to solve every problem instantly, but their resilience, curiosity, and commitment to continuous learning.
As you continue your coding journey, embrace each challenge as a chance to grow. Celebrate your progress, learn from your mistakes, and keep pushing forward. With persistence and the right mindset, you’ll not only conquer LeetCode problems but also develop the problem-solving skills and resilience that are invaluable in the ever-evolving world of software development.
So the next time you face a seemingly insurmountable coding challenge, take a deep breath, remember the stages we’ve discussed, and approach the problem with renewed determination. Your future self will thank you for the skills and perseverance you’re developing now.
Happy coding, and may your LeetCode journey be filled with growth, discovery, and eventual triumph!