The Procrastinator’s Guide to Cramming LeetCode: How I Learned 50 Algorithms in 48 Hours
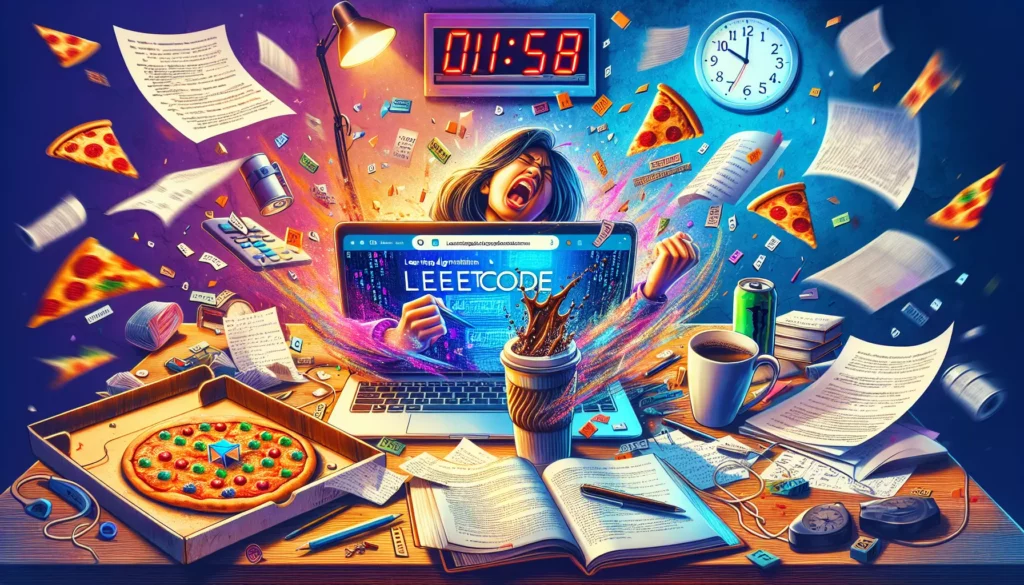
We’ve all been there. You’ve got a big tech interview coming up, and you’ve been putting off your LeetCode practice for weeks. Now, with just 48 hours to go, panic sets in. But fear not, fellow procrastinators! I’m about to share my wild journey of cramming 50 algorithms in just two days. It’s not the ideal way to prepare, but when you’re in a pinch, sometimes you’ve got to work with what you’ve got.
The Setup: My 48-Hour Algorithm Marathon
Before we dive into the nitty-gritty, let me set the scene. It was a typical Wednesday evening when I received an email confirming my interview with a FAANG company for that Friday afternoon. My heart raced as I realized I had severely underestimated my preparation time. With only 48 hours left, I knew I had to act fast.
I cleared my schedule, stocked up on energy drinks and snacks, and prepared for what would be the most intense coding sprint of my life. My goal? To cover 50 of the most common algorithms asked in technical interviews. Was it ambitious? Absolutely. Was it crazy? Probably. But desperate times call for desperate measures.
The Strategy: Breaking Down the Challenge
To tackle this Herculean task, I needed a solid game plan. Here’s how I broke it down:
- 25 algorithms per day
- Approximately 1 hour per algorithm
- 15-minute breaks every 2 hours
- 6 hours of sleep each night (I’m not completely insane)
I categorized the algorithms into groups to ensure I covered a wide range:
- Array and String Manipulation
- Linked Lists
- Trees and Graphs
- Dynamic Programming
- Sorting and Searching
- Miscellaneous (Bit Manipulation, Math, etc.)
Day 1: The Array and String Onslaught
I kicked off my algorithm marathon with the basics: arrays and strings. These are the bread and butter of coding interviews, and I knew I had to nail them.
1. Two Sum
Starting with the classic “Two Sum” problem felt like easing into a warm bath. The goal is to find two numbers in an array that add up to a target sum. Here’s a simple solution using a hash map:
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
This solution has a time complexity of O(n) and space complexity of O(n), making it efficient for large inputs.
2. Valid Parentheses
Moving on to strings, the “Valid Parentheses” problem is a great introduction to using stacks. The task is to determine if a string of brackets is valid (i.e., every opening bracket has a corresponding closing bracket in the correct order).
def is_valid(s):
stack = []
mapping = {")": "(", "}": "{", "]": "["}
for char in s:
if char in mapping:
if not stack or stack[-1] != mapping[char]:
return False
stack.pop()
else:
stack.append(char)
return len(stack) == 0
This elegant solution uses a stack to keep track of opening brackets and checks for matching closing brackets as it iterates through the string.
3. Reverse Linked List
As the day progressed, I moved onto linked lists. The “Reverse Linked List” problem is a classic that often comes up in interviews. Here’s an iterative solution:
def reverse_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
This solution reverses the links between nodes as it traverses the list, effectively reversing the entire list in one pass.
4. Merge Two Sorted Lists
Continuing with linked lists, I tackled the “Merge Two Sorted Lists” problem. This problem tests your ability to manipulate pointers and work with sorted data structures:
def merge_two_lists(l1, l2):
dummy = ListNode(0)
current = dummy
while l1 and l2:
if l1.val < l2.val:
current.next = l1
l1 = l1.next
else:
current.next = l2
l2 = l2.next
current = current.next
current.next = l1 if l1 else l2
return dummy.next
This solution uses a dummy node to simplify the merging process and handles the case where one list might be longer than the other.
5. Maximum Subarray
As the first day was winding down, I delved into dynamic programming with the “Maximum Subarray” problem. This problem asks you to find the contiguous subarray with the largest sum:
def max_subarray(nums):
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
This elegant solution uses Kadane’s algorithm, which has a time complexity of O(n) and space complexity of O(1).
Day 2: Diving Deeper into Data Structures
After a short night’s sleep, I dove back in, ready to tackle more complex problems involving trees, graphs, and advanced algorithms.
6. Binary Tree Inorder Traversal
Starting the day with trees, I implemented an inorder traversal of a binary tree. This is a fundamental operation that forms the basis for many tree-related algorithms:
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
This recursive solution elegantly captures the left-root-right order of inorder traversal.
7. Validate Binary Search Tree
Building on the previous problem, I moved on to validating a binary search tree. This problem tests your understanding of the BST property and recursive thinking:
def is_valid_bst(root):
def validate(node, low=float('-inf'), high=float('inf')):
if not node:
return True
if node.val <= low or node.val >= high:
return False
return (validate(node.left, low, node.val) and
validate(node.right, node.val, high))
return validate(root)
This solution uses a helper function with upper and lower bounds to ensure each node satisfies the BST property.
8. Course Schedule (Graph Cycle Detection)
Moving into graph territory, I tackled the “Course Schedule” problem, which is essentially cycle detection in a directed graph:
from collections import defaultdict
def can_finish(num_courses, prerequisites):
graph = defaultdict(list)
for course, prereq in prerequisites:
graph[course].append(prereq)
def is_cyclic(course, path):
if course in path:
return True
if course in visited:
return False
visited.add(course)
path.add(course)
for prereq in graph[course]:
if is_cyclic(prereq, path):
return True
path.remove(course)
return False
visited = set()
for course in range(num_courses):
if is_cyclic(course, set()):
return False
return True
This solution uses depth-first search to detect cycles in the graph, which would indicate that the course schedule is impossible.
9. Merge K Sorted Lists
As the day progressed, I moved onto more advanced problems. “Merge K Sorted Lists” is a step up from merging two lists and introduces the concept of using a heap for efficient merging:
import heapq
def merge_k_lists(lists):
heap = []
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
dummy = ListNode(0)
current = dummy
while heap:
val, i, node = heapq.heappop(heap)
current.next = node
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
This solution uses a min-heap to efficiently select the smallest element among the k lists at each step.
10. LRU Cache
As the final hours of my cramming session approached, I tackled the “LRU Cache” problem, which tests your ability to design a data structure with specific time complexity requirements:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key):
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
This solution uses Python’s OrderedDict to maintain the order of elements, allowing for O(1) time complexity for both get and put operations.
The Aftermath: Lessons Learned
As the clock struck 48 hours, I collapsed onto my bed, my brain swimming with algorithms and data structures. While I wouldn’t recommend this intense cramming method to anyone (seriously, start preparing earlier!), I did learn some valuable lessons:
- Pattern Recognition is Key: Many problems share similar patterns. Once you recognize these patterns, solving new problems becomes easier.
- Time Management is Crucial: Even with limited time, it’s important to allocate your efforts wisely. Focus on understanding the core concepts rather than memorizing specific solutions.
- Practice Active Recall: Instead of just reading solutions, try to implement them yourself. This helps solidify your understanding.
- Don’t Neglect the Basics: Even when tackling advanced problems, a strong grasp of fundamental data structures and algorithms is essential.
- Take Care of Your Health: Pulling all-nighters and surviving on caffeine is not sustainable. Regular breaks and adequate sleep are crucial for effective learning.
The Interview: How It Went
You’re probably wondering how the interview went after this intense cramming session. Well, I’m happy to report that I didn’t completely crash and burn! While I certainly wasn’t as prepared as I could have been with more time, the intense focus of the past 48 hours had sharpened my problem-solving skills.
During the interview, I was asked to solve two algorithmic problems:
- A variation of the “Two Sum” problem (thank goodness I started with that one!)
- A tree traversal problem that was similar to the Binary Tree Inorder Traversal I had practiced
While I didn’t come up with optimal solutions immediately, I was able to talk through my thought process, propose initial solutions, and then optimize them. The interviewer seemed impressed with my ability to communicate my ideas and iterate on my solutions.
The intense cramming had given me a broad overview of many algorithmic concepts, which helped me make connections and draw from different areas to solve the problems at hand. However, it was clear that deeper understanding and more practice would have made the process smoother.
Conclusion: The Right Way to Prepare
While my 48-hour algorithm marathon got me through the interview, it’s not a method I’d recommend. Proper preparation for coding interviews should involve:
- Consistent Practice: Solve a few problems each day over an extended period. This allows for better retention and deeper understanding.
- Focus on Fundamentals: Ensure you have a solid grasp of basic data structures and algorithms before moving on to more complex topics.
- Mock Interviews: Practice explaining your thought process out loud. Platforms like AlgoCademy offer mock interview experiences that can be invaluable.
- Review and Reflect: After solving a problem, take time to review other solutions and reflect on what you’ve learned.
- Build Projects: Apply your algorithmic knowledge to real-world projects. This helps solidify your understanding and gives you talking points for behavioral interviews.
Remember, the goal of technical interviews isn’t just to solve problems, but to demonstrate your problem-solving approach, communication skills, and ability to write clean, efficient code. These skills are developed over time, not crammed in 48 hours.
If you’re looking for a more structured and effective way to prepare for coding interviews, consider using platforms like AlgoCademy. With its interactive coding tutorials, AI-powered assistance, and comprehensive curriculum, it can help you build a solid foundation in algorithmic thinking and problem-solving skills.
In the end, while my cramming adventure made for an interesting story, the best approach to mastering algorithms and acing technical interviews is consistent, deliberate practice over time. Start early, stay curious, and happy coding!